- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Implementing Secure WebSockets (wss) in Golang
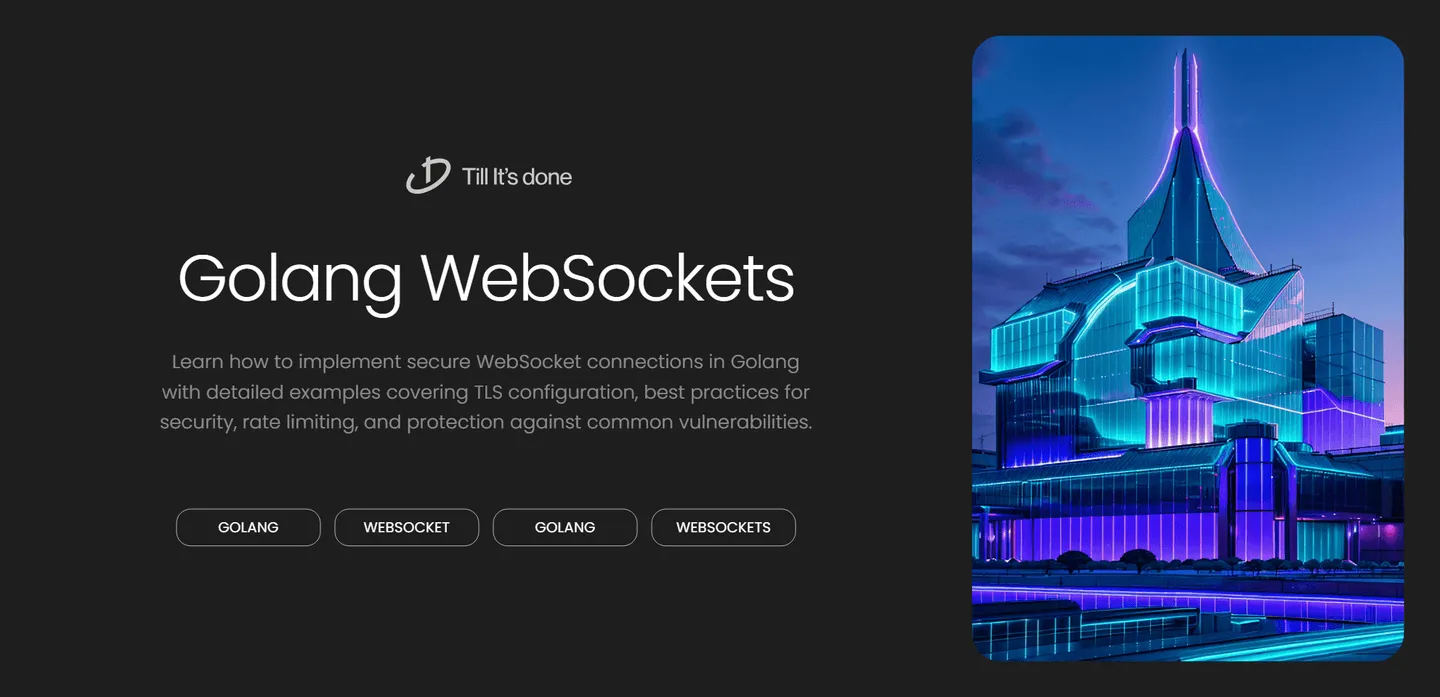
Implementing Secure WebSockets (wss) in Golang
In today’s real-time web applications, WebSocket connections have become crucial for enabling bidirectional communication between clients and servers. However, security should never be an afterthought. Let’s explore how to implement secure WebSockets (WSS) in Golang, ensuring your real-time applications remain protected against potential threats.
Understanding WebSocket Security
Before diving into the implementation, it’s essential to understand why we need secure WebSockets. Regular WebSocket connections (ws://) transmit data in plain text, making them vulnerable to man-in-the-middle attacks and eavesdropping. Secure WebSockets (wss://) use TLS/SSL encryption to protect the data in transit, similar to how HTTPS secures HTTP connections.
Setting Up the Project
First, let’s create a secure WebSocket server using Golang. We’ll use the popular gorilla/websocket
package for this implementation.
package main
import ( "log" "net/http" "github.com/gorilla/websocket")
var upgrader = websocket.Upgrader{ ReadBufferSize: 1024, WriteBufferSize: 1024, CheckOrigin: func(r *http.Request) bool { // In production, replace this with proper origin validation return true },}
func handleWebSocket(w http.ResponseWriter, r *http.Request) { conn, err := upgrader.Upgrade(w, r, nil) if err != nil { log.Printf("Failed to upgrade connection: %v", err) return } defer conn.Close()
// Implement connection handling logic here for { messageType, message, err := conn.ReadMessage() if err != nil { log.Printf("Error reading message: %v", err) break }
// Echo the message back if err := conn.WriteMessage(messageType, message); err != nil { log.Printf("Error writing message: %v", err) break } }}
Implementing TLS Security
To enable WSS, we need to configure TLS certificates. You can use Let’s Encrypt for production or generate self-signed certificates for development.
func main() { http.HandleFunc("/ws", handleWebSocket)
// Load TLS certificates certFile := "path/to/cert.pem" keyFile := "path/to/key.pem"
log.Printf("Starting secure WebSocket server on :8443") err := http.ListenAndServeTLS(":8443", certFile, keyFile, nil) if err != nil { log.Fatal("ListenAndServeTLS: ", err) }}
Best Practices for WebSocket Security
- Origin Validation: Implement proper origin checking to prevent unauthorized connections:
var upgrader = websocket.Upgrader{ CheckOrigin: func(r *http.Request) bool { origin := r.Header.Get("Origin") return origin == "https://yourtrustedorigin.com" },}
- Connection Timeouts: Implement read and write deadlines to prevent resource exhaustion:
const ( writeWait = 10 * time.Second pongWait = 60 * time.Second)
conn.SetReadDeadline(time.Now().Add(pongWait))conn.SetPongHandler(func(string) error { conn.SetReadDeadline(time.Now().Add(pongWait)) return nil})
Rate Limiting and DOS Protection
Implement rate limiting to protect your WebSocket server from denial of service attacks:
type Client struct { conn *websocket.Conn lastPing time.Time msgCount int rateLimit time.Duration}
func (c *Client) checkRateLimit() bool { now := time.Now() if now.Sub(c.lastPing) < c.rateLimit { c.msgCount++ if c.msgCount > 100 { // Max 100 messages per rate limit window return false } } else { c.lastPing = now c.msgCount = 0 } return true}
Conclusion
Implementing secure WebSockets in Golang requires careful attention to both encryption and additional security measures. By following these best practices and implementing proper security controls, you can build robust real-time applications that protect your users’ data and maintain the integrity of your communication channels.
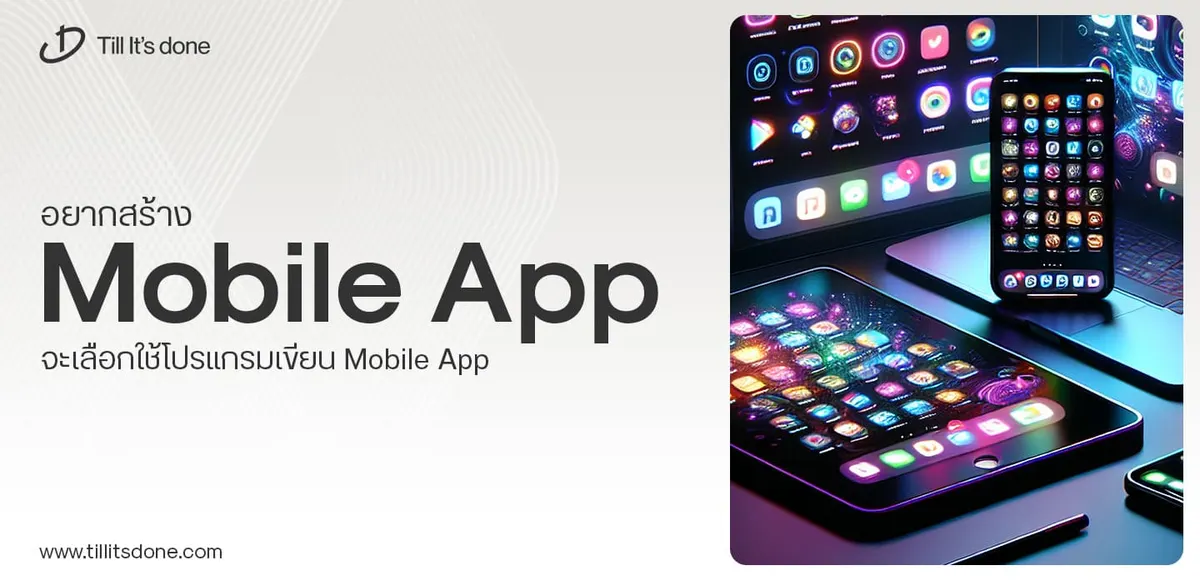
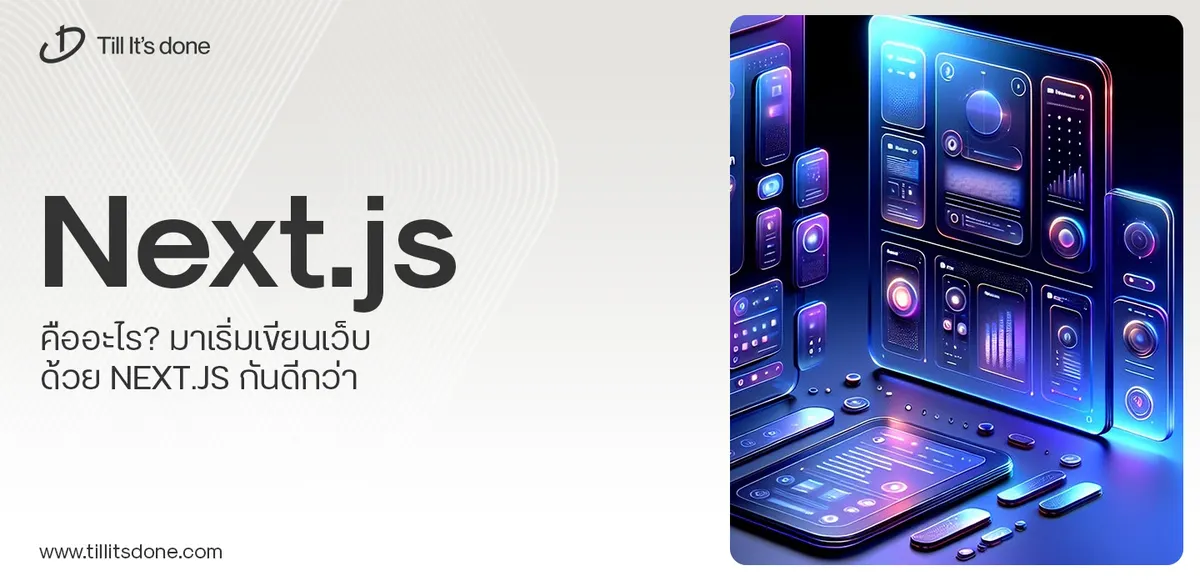
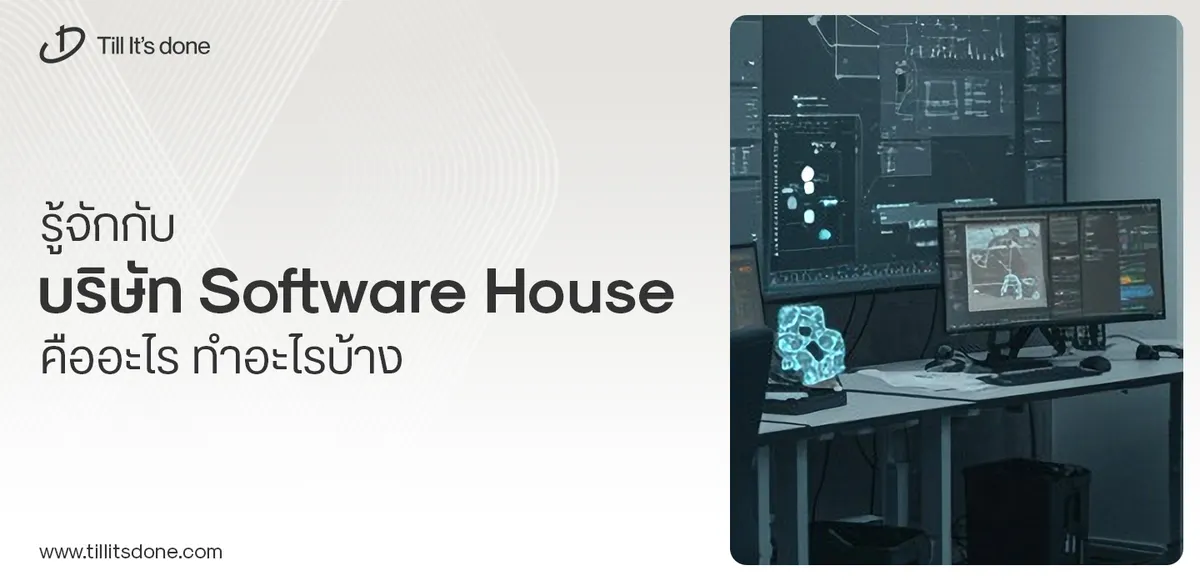
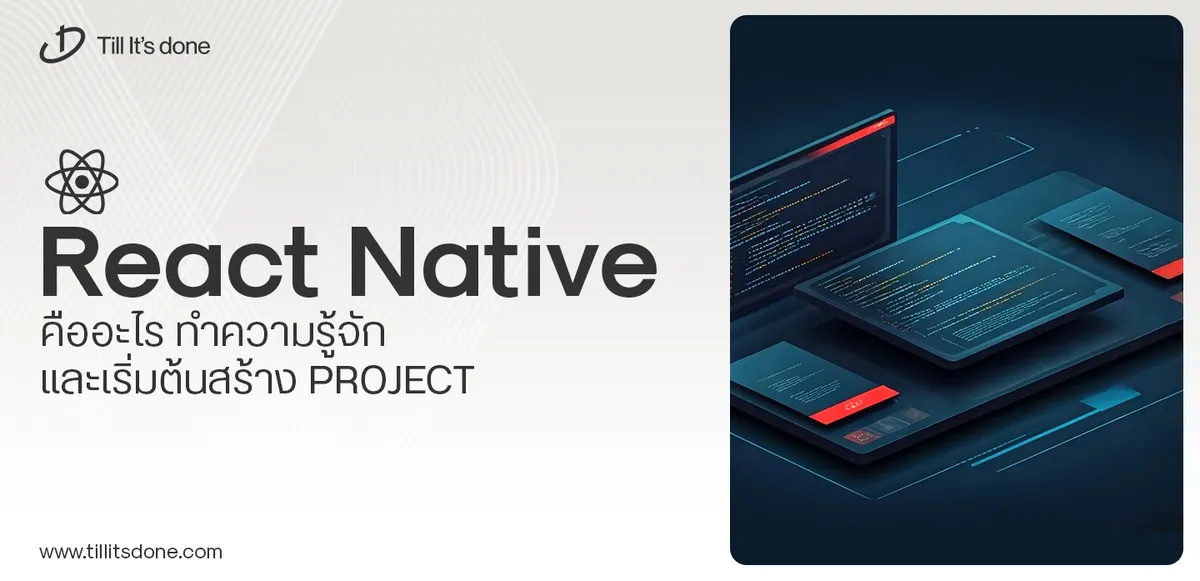
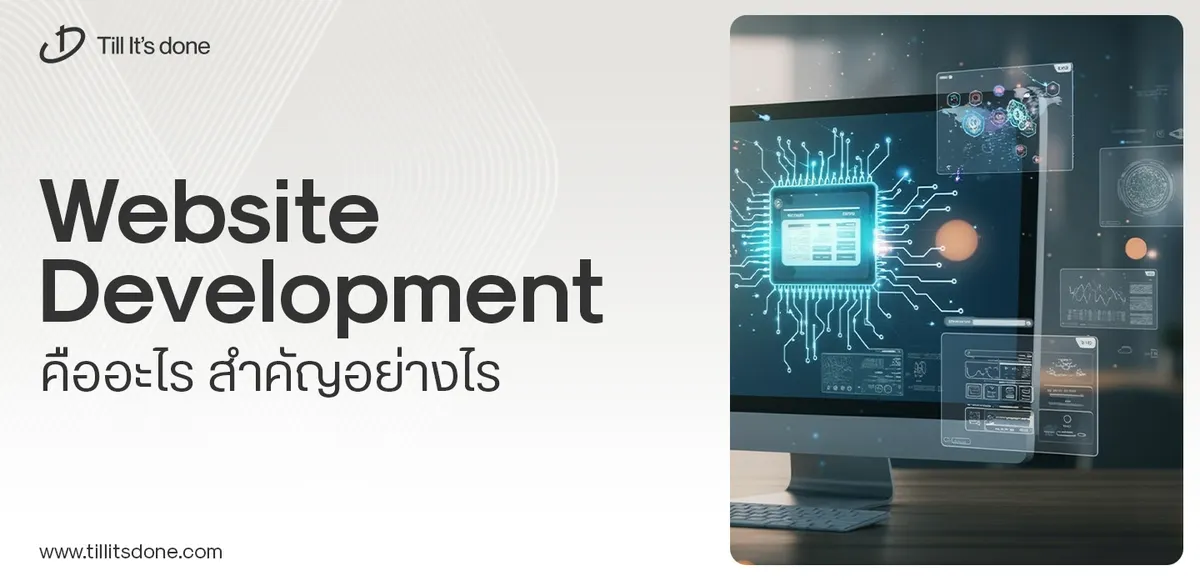
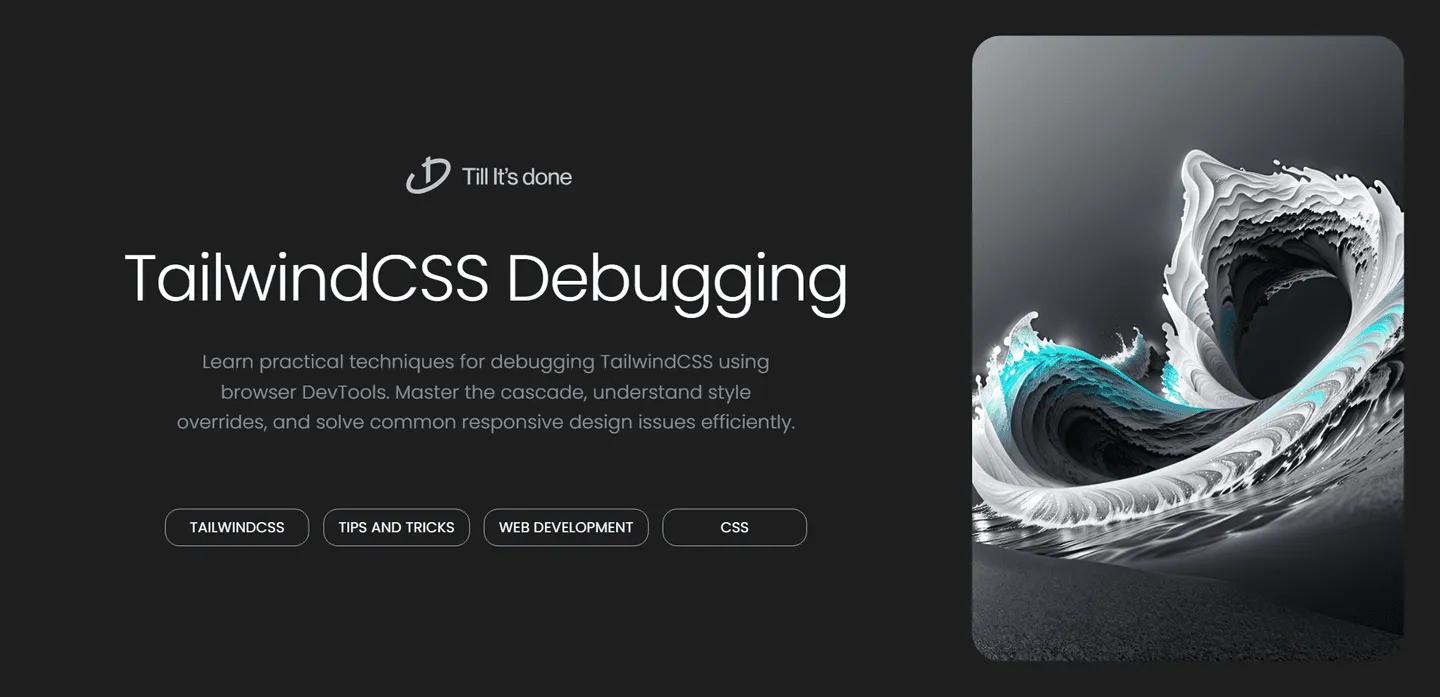
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.