- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
WebSocket Message Broadcasting in Golang Guide

Handling WebSocket Message Broadcasting in Golang
WebSocket broadcasting in Golang opens up exciting possibilities for real-time applications. In this post, I’ll guide you through implementing a robust WebSocket broadcasting system that can handle multiple client connections efficiently.
Understanding WebSocket Broadcasting
Think of WebSocket broadcasting like a radio station - one message gets transmitted to all connected listeners simultaneously. This pattern is crucial for applications like chat rooms, live dashboards, or multiplayer games.
Core Components
The heart of our broadcasting system consists of three main parts:
- A central hub managing all client connections
- Client handlers for individual WebSocket connections
- A message broadcasting mechanism
Here’s how we can implement this elegant solution:
type Hub struct { clients map[*Client]bool broadcast chan []byte register chan *Client unregister chan *Client}
type Client struct { hub *Hub conn *websocket.Conn send chan []byte}
Broadcasting Implementation
When a message arrives, our hub efficiently distributes it to all connected clients. The beauty of Golang’s concurrency model really shines here, as we can handle thousands of connections seamlessly.
Error Handling and Connection Management
One often overlooked aspect is graceful error handling. When clients disconnect or network issues occur, our system needs to clean up resources and maintain stability. I’ve found that implementing a heartbeat mechanism helps detect stale connections early.
Best Practices and Performance Tips
- Use buffered channels for better performance
- Implement connection timeouts
- Consider message queuing for high-load scenarios
- Batch messages when possible to reduce network overhead
Remember, the key to a successful WebSocket broadcasting system lies in finding the right balance between performance and reliability.
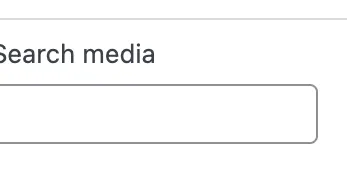





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.