- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Using Riverpod for Async Data Fetching in Flutter
Discover best practices for state management, error handling, and creating maintainable code with practical examples.

Using Riverpod for Async Data Fetching in Flutter
If you’ve been working with Flutter, you know that managing state and handling asynchronous data can be challenging. Today, I want to share my experience with Riverpod, a powerful state management solution that makes async data handling in Flutter feel like a breeze.
Why Riverpod?
Before diving into the implementation, let’s talk about why Riverpod is becoming a go-to choice for Flutter developers. Unlike traditional state management solutions, Riverpod offers a declarative approach that’s both type-safe and testable. It’s like having a smart assistant that helps you manage your app’s data flow effortlessly.
Getting Started with Riverpod
First things first, you’ll need to add Riverpod to your project. In your pubspec.yaml, include the following dependencies:
dependencies: flutter_riverpod: ^2.4.0 riverpod_annotation: ^2.0.0
dev_dependencies: riverpod_generator: ^2.0.0 build_runner: ^2.4.0
Creating Your First Provider
Let’s build something practical - a weather data fetcher. Here’s how you can create a simple async provider:
@riverpodFuture<WeatherData> weatherData(WeatherDataRef ref) async { final weatherService = WeatherService(); return await weatherService.fetchWeather();}
This code snippet might look simple, but it’s doing a lot behind the scenes. Riverpod handles all the complex state management for you, including loading and error states.
Consuming Async Data
The real magic happens when you consume this data in your widgets. Here’s how elegant it looks:
class WeatherWidget extends ConsumerWidget { @override Widget build(BuildContext context, WidgetRef ref) { final weatherAsync = ref.watch(weatherDataProvider);
return weatherAsync.when( data: (weather) => WeatherDisplay(weather), loading: () => CircularProgressIndicator(), error: (error, stack) => ErrorWidget(error.toString()), ); }}
Best Practices and Tips
- Always handle loading and error states gracefully
- Use family modifiers for parameterized data fetching
- Leverage ref.listen for side effects
- Cache your data when appropriate
Remember, Riverpod’s power comes from its simplicity and predictability. You don’t need complex architectures to build robust applications.
Conclusion
Riverpod transforms async data handling from a potential headache into a structured, maintainable process. It’s not just about writing less code; it’s about writing better, more reliable code that’s easier to test and maintain.
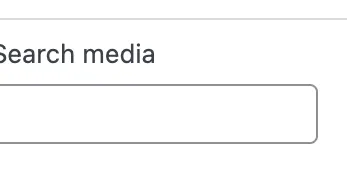





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.