- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Building a REST API with Fiber in Golang
Discover the power of Golang web development with practical examples and best practices for API design.
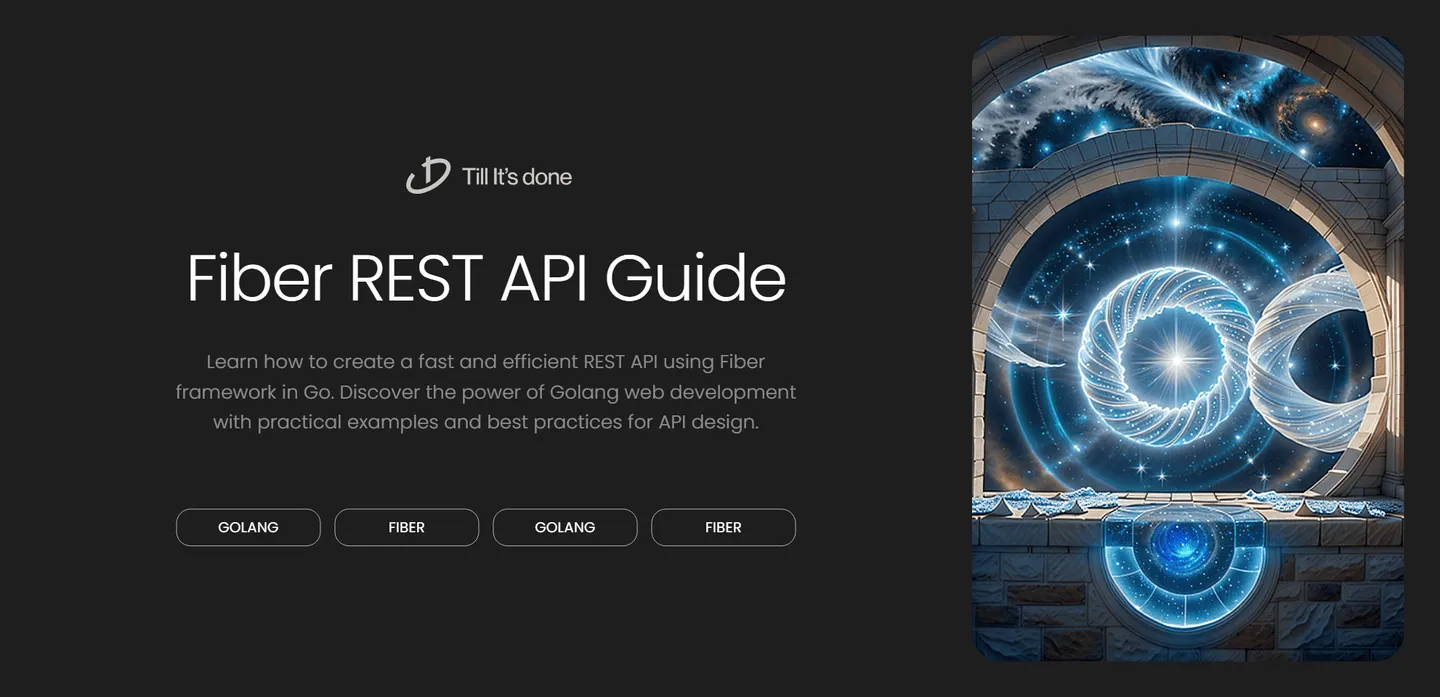
Building a REST API with Fiber in Golang
Ever since Go burst onto the scene, it’s been making waves in the backend development world. Today, I want to share my experience with one of Go’s most exciting web frameworks: Fiber. Think of Fiber as Go’s answer to Express.js – it’s fast, minimal, and gets the job done without unnecessary complexity.
Why Fiber?
Before diving into the code, you might wonder why choose Fiber among the sea of Go web frameworks. Well, Fiber stands out for a few compelling reasons. First off, it’s blazingly fast – we’re talking about sub-millisecond response times. Plus, if you’re coming from Express.js, you’ll feel right at home with Fiber’s familiar API design.
Setting Up Our Project
Getting started with Fiber is straightforward. First, you’ll need to create a new Go project and install Fiber. Here’s how I structured my project:
go mod init myapigo get github.com/gofiber/fiber/v2
Building Our First Endpoints
Let’s create a simple REST API for a book management system. We’ll implement basic CRUD operations that I use in my daily work. Here’s how we structure our main application:
package main
import ( "github.com/gofiber/fiber/v2")
type Book struct { ID string `json:"id"` Title string `json:"title"` Author string `json:"author"`}
func main() { app := fiber.New()
app.Get("/api/books", getAllBooks) app.Post("/api/books", createBook) app.Get("/api/books/:id", getBook) app.Delete("/api/books/:id", deleteBook)
app.Listen(":3000")}
Error Handling and Middleware
One thing I love about Fiber is its elegant error handling. Instead of writing try-catch blocks everywhere, Fiber provides a clean way to handle errors through middleware. Here’s a pattern I often use:
app.Use(func(c *fiber.Ctx) error { defer func() { if r := recover(); r != nil { c.Status(500).JSON(fiber.Map{ "error": "Internal Server Error", }) } }() return c.Next()})
Testing Our API
Before deploying, it’s crucial to test our endpoints. Fiber makes this surprisingly simple with its built-in testing utilities. No need for complex setups or external tools – just write your tests and run them.
The beauty of Fiber lies in its simplicity and performance. After building several production APIs with it, I can confidently say it’s one of the most developer-friendly frameworks in the Go ecosystem.
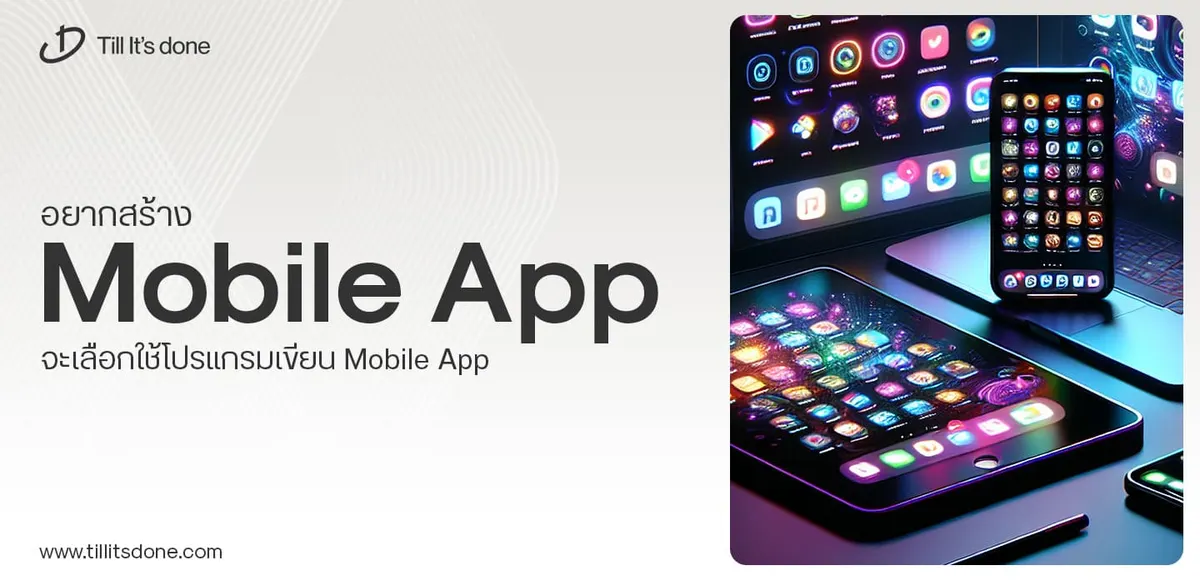
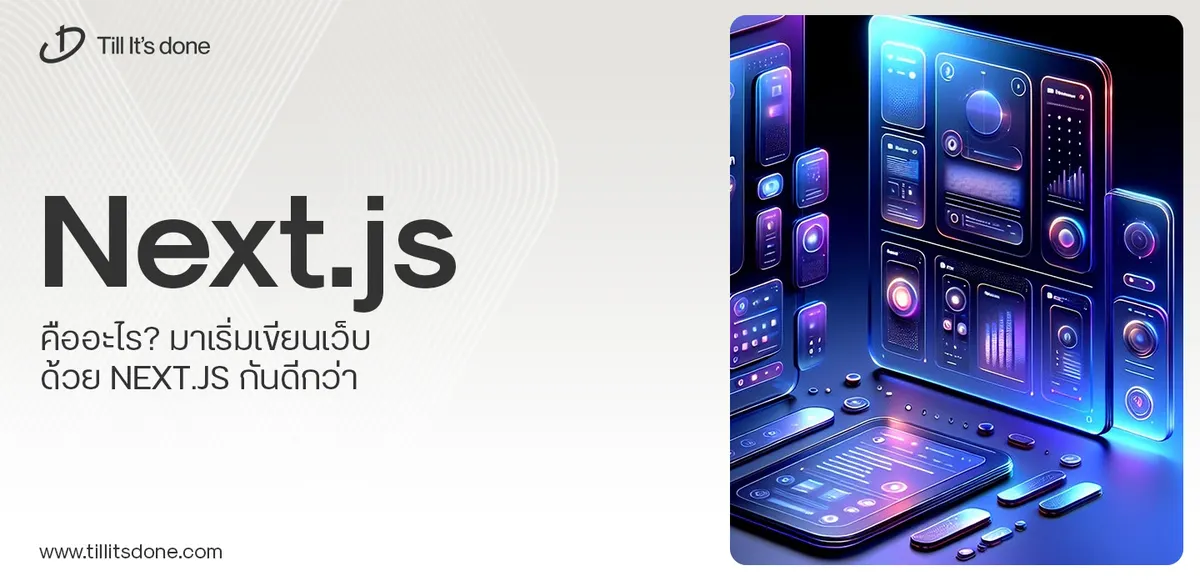
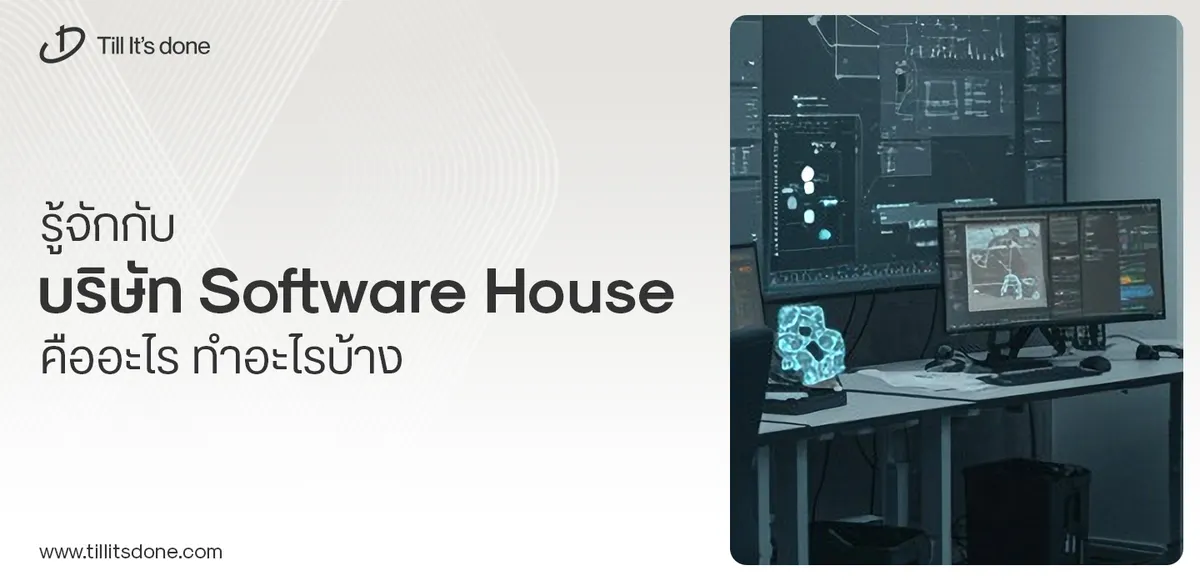
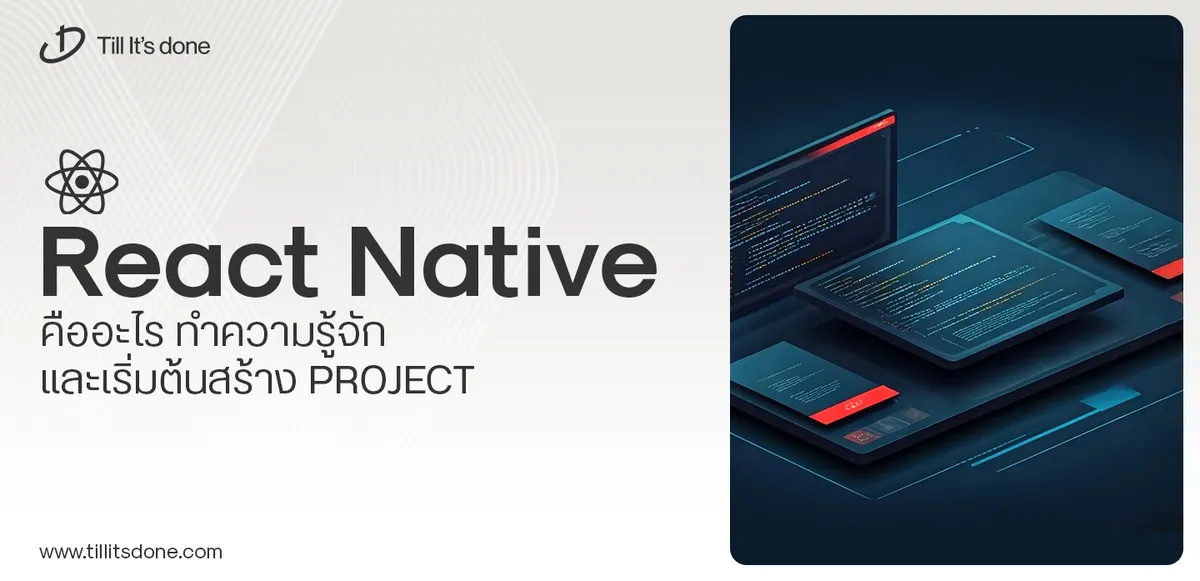
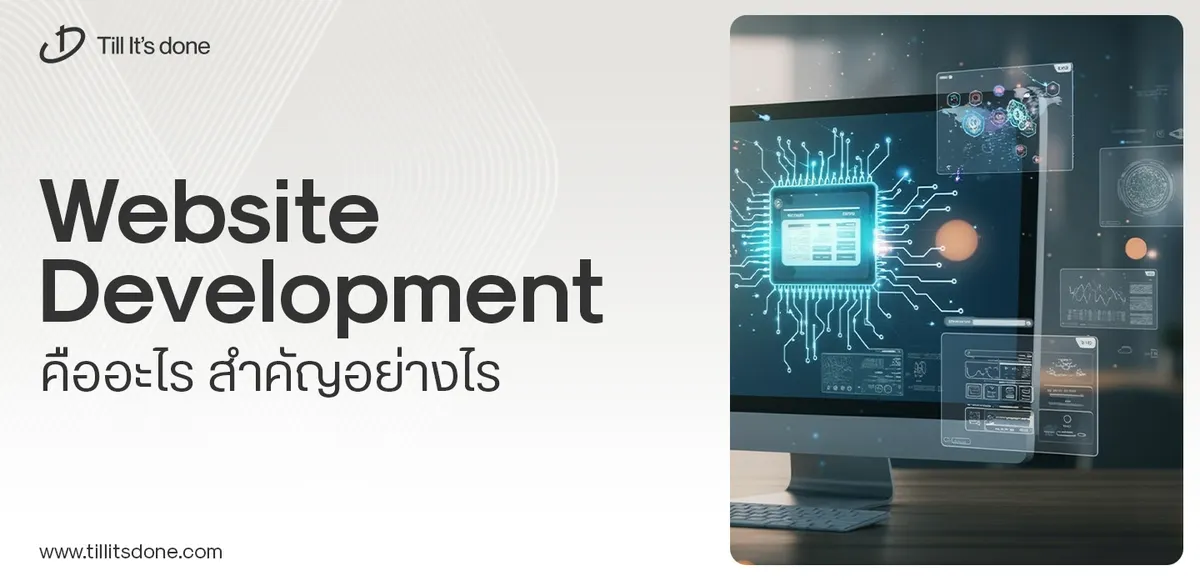
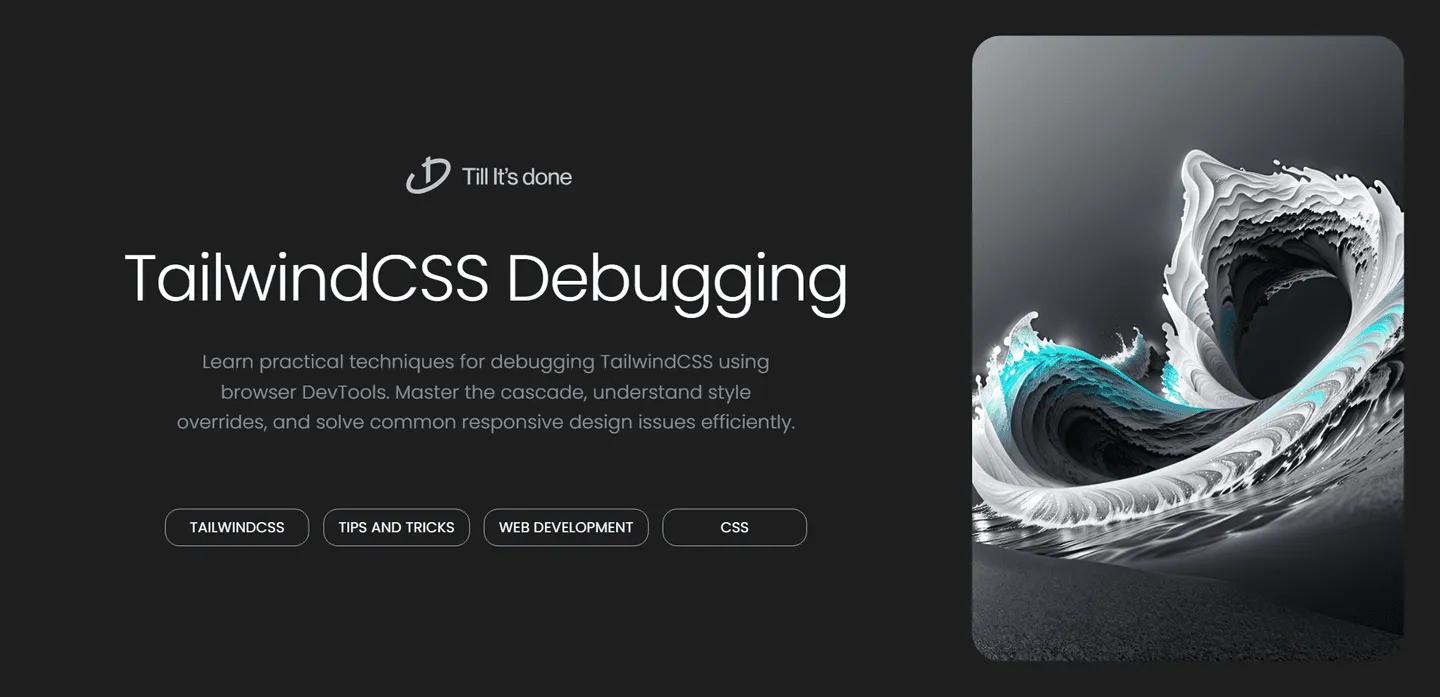
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.