- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Migrating from Redux to Zustand: Step-by-Step Guide
Discover simpler state management, reduced boilerplate, and improved developer experience.

Migrating from Redux to Zustand: Step-by-Step Guide
Are you feeling overwhelmed by Redux’s boilerplate code? You’re not alone. While Redux has been the go-to state management solution for React applications, many developers are now turning to Zustand for its simplicity and efficiency. In this guide, I’ll walk you through migrating your Redux application to Zustand, step by step.
Why Consider Zustand?
Before we dive into the migration process, let’s understand why Zustand might be the right choice for your project. Having worked with both Redux and Zustand in production environments, I can tell you that Zustand brings several advantages to the table:
- Minimal boilerplate code
- No need for context providers
- Built-in TypeScript support
- Smaller bundle size
- Simpler learning curve
Getting Started with the Migration
1. Installing Zustand
First, let’s add Zustand to your project:
npm install zustand# oryarn add zustand
2. Creating Your First Store
In Zustand, we’ll replace Redux’s store configuration with a simpler approach. Let’s convert a basic Redux store to Zustand:
// Before (Redux)const initialState = { todos: [], filter: 'all'}
// After (Zustand)import create from 'zustand'
interface TodoState { todos: Todo[] filter: string addTodo: (text: string) => void toggleTodo: (id: number) => void}
const useStore = create<TodoState>((set) => ({ todos: [], filter: 'all', addTodo: (text) => set((state) => ({ todos: [...state.todos, { id: Date.now(), text, completed: false }] })), toggleTodo: (id) => set((state) => ({ todos: state.todos.map(todo => todo.id === id ? { ...todo, completed: !todo.completed } : todo ) }))}))
3. Updating Components
Now, let’s look at how we update our components to use the new Zustand store:
// Before (Redux)const TodoList = () => { const todos = useSelector(state => state.todos) const dispatch = useDispatch()
return ( // Component JSX )}
// After (Zustand)const TodoList = () => { const todos = useStore(state => state.todos) const addTodo = useStore(state => state.addTodo)
return ( // Component JSX )}
4. Handling Middleware and Side Effects
One of Zustand’s strengths is its simplicity in handling side effects. Here’s how we can migrate Redux middleware patterns:
const useStore = create( devtools( persist( (set) => ({ // Your store logic }), { name: 'todo-storage', } ) ))
Best Practices and Tips
-
Gradual Migration: Consider migrating your stores one at a time rather than all at once. This allows for a smoother transition and easier debugging.
-
State Structure: Keep your Zustand store structure flat and simple. Unlike Redux, you don’t need to follow strict immutability patterns.
-
Selective Updates: Use Zustand’s selective updates to prevent unnecessary re-renders:
// Only subscribe to specific parts of the storeconst todos = useStore(state => state.todos)
Conclusion
Migrating from Redux to Zustand might feel daunting at first, but the benefits of reduced boilerplate and improved developer experience make it worthwhile. Remember, good state management should feel natural and straightforward – and that’s exactly what Zustand brings to the table.
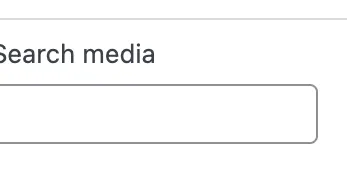





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.