- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Animate React Components with Transition Group
Learn how to implement fade effects, transitions, and create engaging user experiences.

Have you ever wondered how to make your React components smoothly fade in and out instead of abruptly appearing and disappearing? That’s where React Transition Group comes in! As a developer who’s spent countless hours perfecting UI animations, I can tell you that this library is a game-changer for creating polished user experiences.
What is React Transition Group?
React Transition Group is a powerful animation library that helps you control component transitions as they enter or leave the DOM. Think of it as a conductor, orchestrating the perfect timing for your component’s entrance and exit.
Core Components You Need to Know
1. Transition
The Transition
component is your basic building block. It manages the animation states: ‘entering’, ‘entered’, ‘exiting’, and ‘exited’. Here’s what makes it special - you get complete control over how your component looks in each state.
2. CSSTransition
This is where the magic really happens! CSSTransition
applies CSS classes automatically during transitions. It’s perfect when you want to use CSS animations or transitions. The best part? You can keep your animation logic clean and separated in your stylesheets.
3. TransitionGroup
Think of TransitionGroup
as the stage manager for your animated components. It handles multiple transitions happening at once, perfect for lists or groups of elements that need to animate together.
Why Should You Use It?
Let me share why I love using React Transition Group:
- It integrates seamlessly with React’s component lifecycle
- Provides fine-grained control over animation timing
- Works brilliantly with both CSS and JavaScript animations
- Makes complex animations manageable and maintainable
Best Practices and Tips
- Always specify clear timeout durations that match your CSS transitions
- Use classNames prop effectively to maintain clean, reusable CSS
- Consider using
onEntered
andonExited
hooks for additional logic - Keep animations subtle and meaningful - they should enhance, not distract
Performance Considerations
Remember to:
- Use
unmountOnExit
when you want to completely remove elements from the DOM - Avoid animating expensive properties like box-shadow or transform with complex calculations
- Consider using will-change CSS property for better performance
- Test animations on lower-end devices to ensure smooth performance
Conclusion
React Transition Group bridges the gap between static and dynamic UIs, making it easier than ever to create engaging user experiences. Whether you’re building a simple fade effect or complex choreographed animations, this library provides the tools you need to bring your components to life.
Remember, great animations are like seasoning in cooking - they should enhance the experience without overpowering it. Start experimenting with React Transition Group today, and watch your applications transform from static to spectacular!
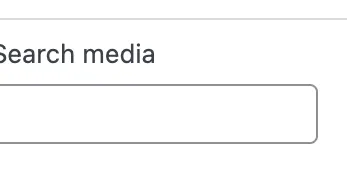





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.