- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Animating Lists in React with React Spring
Master transitions, staggered animations, and drag-and-drop interactions with performance optimization tips.

List animations can transform a static React application into a dynamic, engaging experience. In this guide, we’ll explore how to create beautiful list animations using React Spring – a spring-physics based animation library that makes complex animations feel natural and responsive.
Understanding React Spring’s Approach
React Spring stands out from traditional animation libraries by using spring physics instead of duration-based animations. This approach creates more natural-feeling movements that respond dynamically to changes in your application.
Building Your First Animated List
Let’s start with a simple example of animating items as they enter and leave a list:
import { useTransition, animated } from '@react-spring/web';
function AnimatedList({ items }) { const transitions = useTransition(items, { from: { opacity: 0, transform: 'translateY(20px)' }, enter: { opacity: 1, transform: 'translateY(0px)' }, leave: { opacity: 0, transform: 'translateY(-20px)' }, keys: item => item.id, });
return ( <div className="list-container"> {transitions((style, item) => ( <animated.div style={style}> {item.text} </animated.div> ))} </div> );}
Advanced Techniques
Staggered Animations
One of the most eye-catching effects you can create is staggered animations, where items animate in sequence:
const transitions = useTransition(items, { trail: 400, from: { opacity: 0, scale: 0.8 }, enter: { opacity: 1, scale: 1 }, leave: { opacity: 0, scale: 0.8 },});
Drag and Drop Animations
React Spring also excels at creating smooth drag-and-drop interactions:
function DraggableList({ items }) { const [springs, api] = useSprings(items.length, fn); const bind = useDrag(({ args: [index], down, movement: [x, y] }) => { api.start(i => { if (i === index) { return { x: down ? x : 0, y: down ? y : 0, immediate: down }; } }); });
return springs.map((style, i) => ( <animated.div {...bind(i)} style={style}> {items[i]} </animated.div> ));}
Performance Optimization Tips
- Always use the
keys
prop with unique identifiers - Avoid animating expensive CSS properties
- Use
config
to fine-tune animation physics - Implement virtualization for long lists
Remember that React Spring handles the heavy lifting of performance optimization, but these practices will help you create even smoother animations.
By mastering these concepts, you can create fluid, responsive animations that enhance your user experience while maintaining performance. The spring-physics approach ensures your animations feel natural and responsive, making your React applications more engaging and professional.


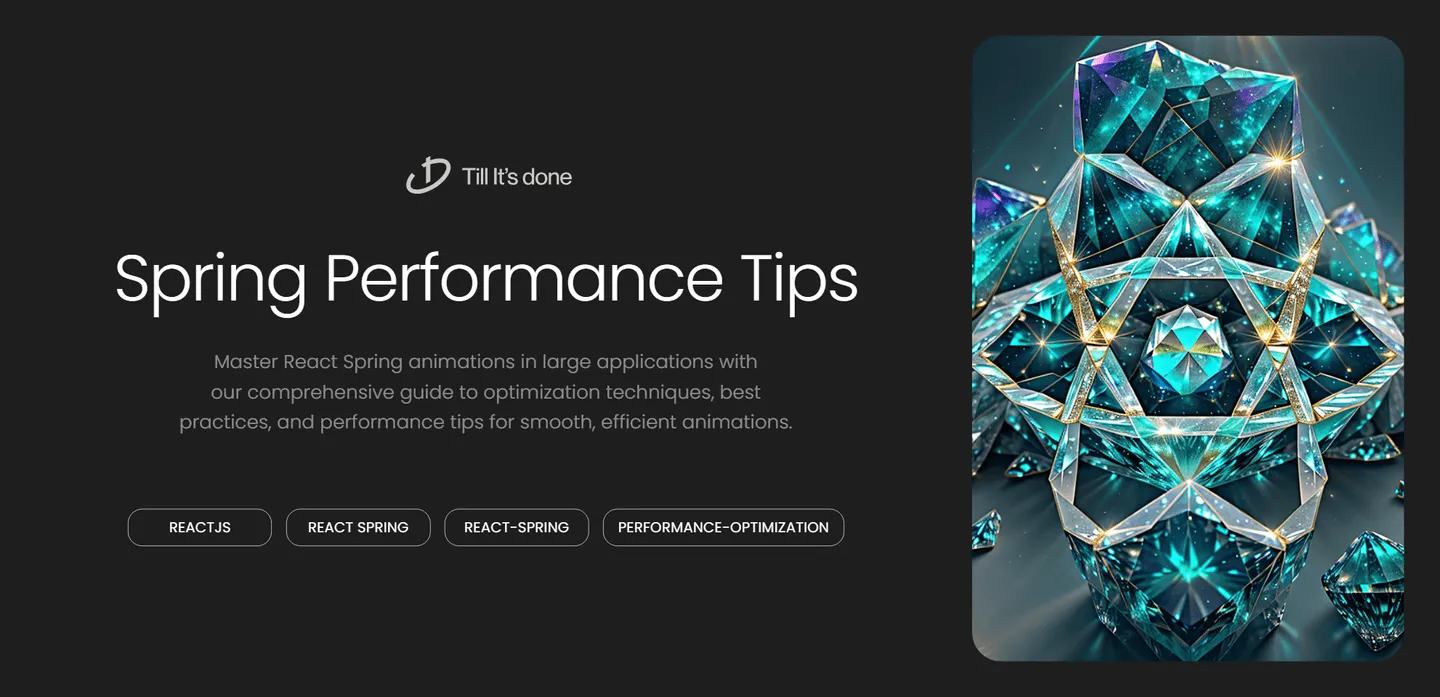

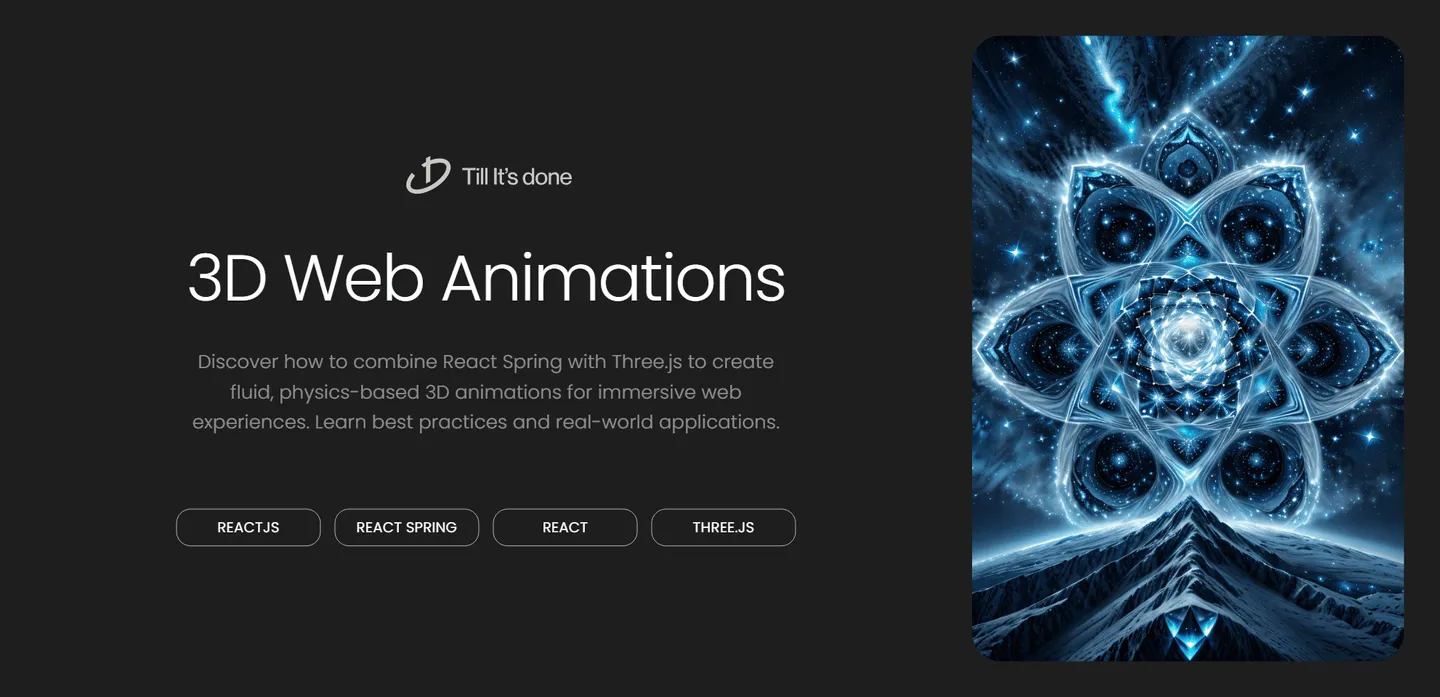

Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.