- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Performance Tips for React Semantic UI Apps
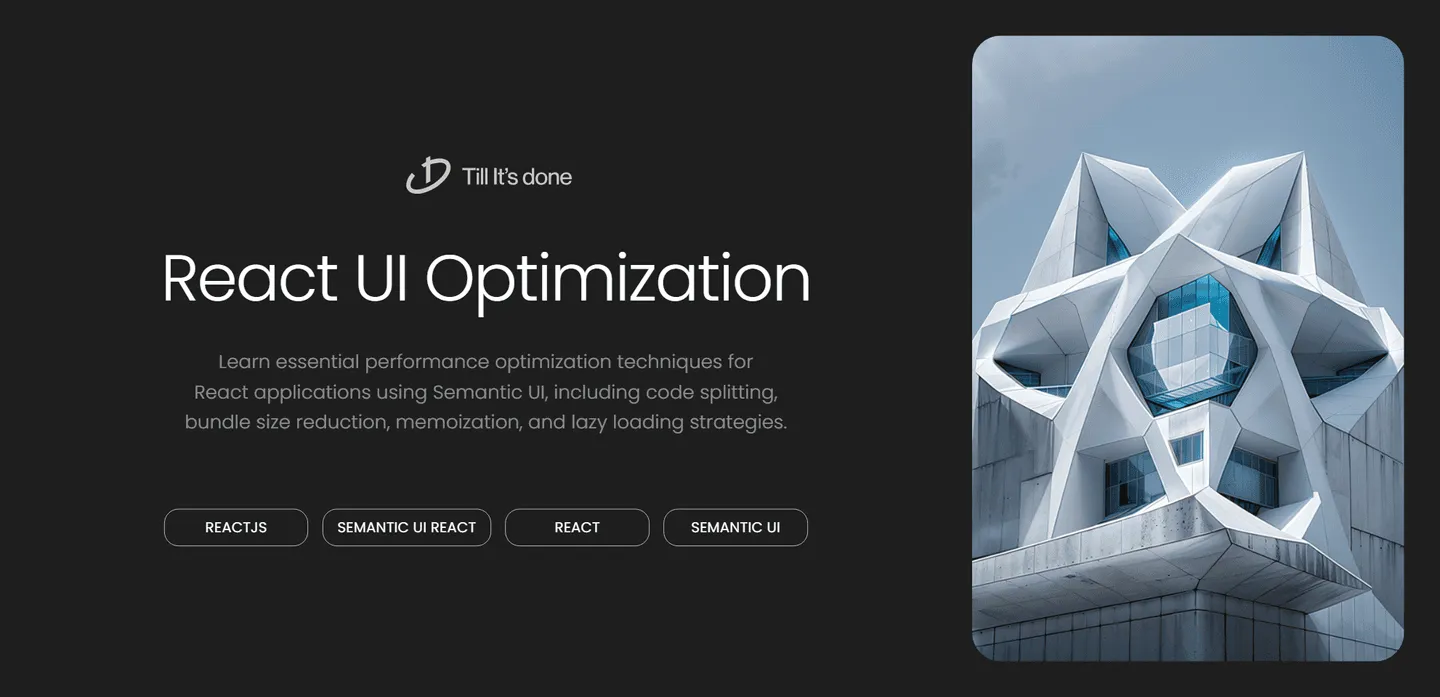
Performance Optimization Tips for React Apps Using Semantic UI
When building React applications with Semantic UI, performance optimization becomes crucial as your app grows in complexity. Today, let’s explore some practical techniques to enhance your app’s performance while maintaining the sleek look that Semantic UI provides.
Understanding the Performance Impact
While Semantic UI React offers a rich set of components that accelerate development, improper implementation can lead to performance bottlenecks. Let’s dive into some effective optimization strategies that every React developer should know.
1. Implement Code Splitting for Semantic UI Components
One of the most impactful optimizations is implementing code splitting for your Semantic UI components. Instead of importing the entire Semantic UI library, import components individually:
// Instead of thisimport { Button, Modal, Form } from 'semantic-ui-react'
// Do thisimport Button from 'semantic-ui-react/dist/commonjs/elements/Button'import Modal from 'semantic-ui-react/dist/commonjs/modules/Modal'import Form from 'semantic-ui-react/dist/commonjs/collections/Form'
2. Optimize CSS Bundle Size
Semantic UI comes with comprehensive styling, but you might not need all of it. Create a custom build including only the components you use:
@import '~semantic-ui-less/definitions/elements/button';@import '~semantic-ui-less/definitions/collections/form';@import '~semantic-ui-less/definitions/modules/modal';
3. Implement Proper Memoization
Use React.memo() wisely with Semantic UI components to prevent unnecessary re-renders:
const MemoizedDropdown = React.memo(({ options, value, onChange }) => ( <Dropdown options={options} value={value} onChange={onChange} selection />));
4. Optimize Form Handling
When working with Semantic UI forms, implement controlled components efficiently:
const [formState, setFormState] = useState({});
const handleChange = useCallback((e, { name, value }) => { setFormState(prev => ({ ...prev, [name]: value }));}, []);
5. Lazy Loading for Complex Components
Implement lazy loading for complex Semantic UI components that aren’t immediately needed:
const LazyDataTable = lazy(() => import('./components/DataTable'));
// Usage<Suspense fallback={<Loader active />}> <LazyDataTable /></Suspense>
Conclusion
By implementing these optimization techniques, you can significantly improve your React application’s performance while maintaining the aesthetic appeal of Semantic UI. Remember to measure performance impacts using React DevTools and implement these optimizations progressively based on your application’s needs.
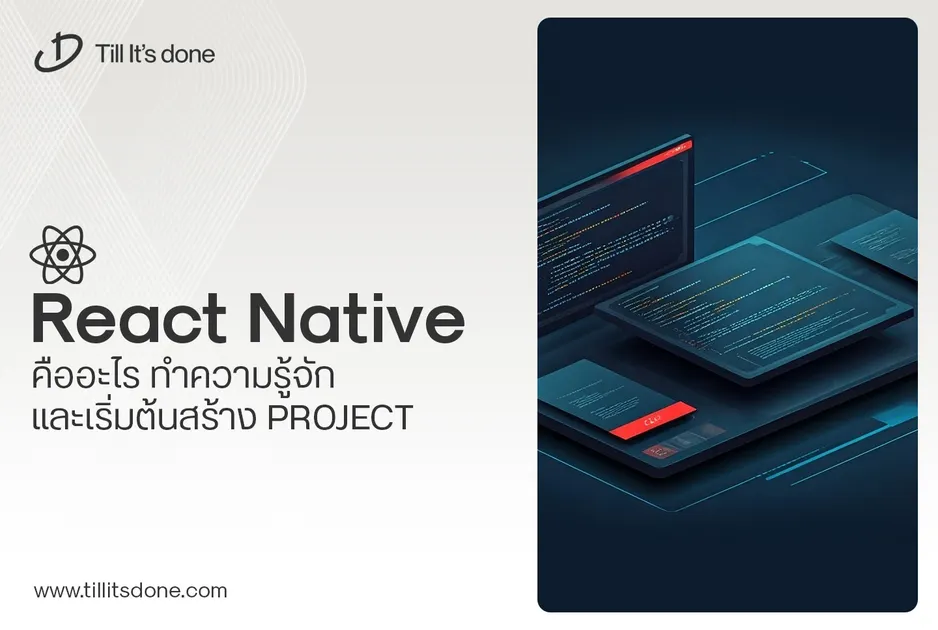
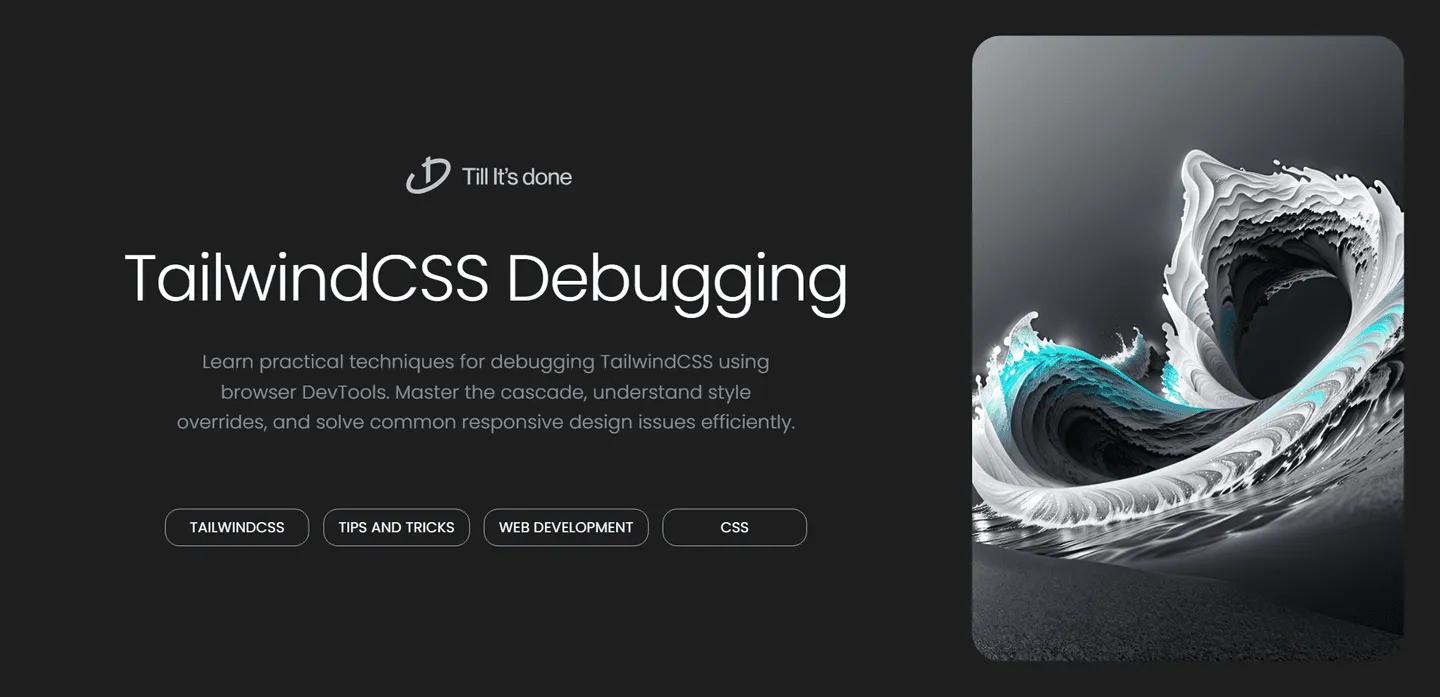
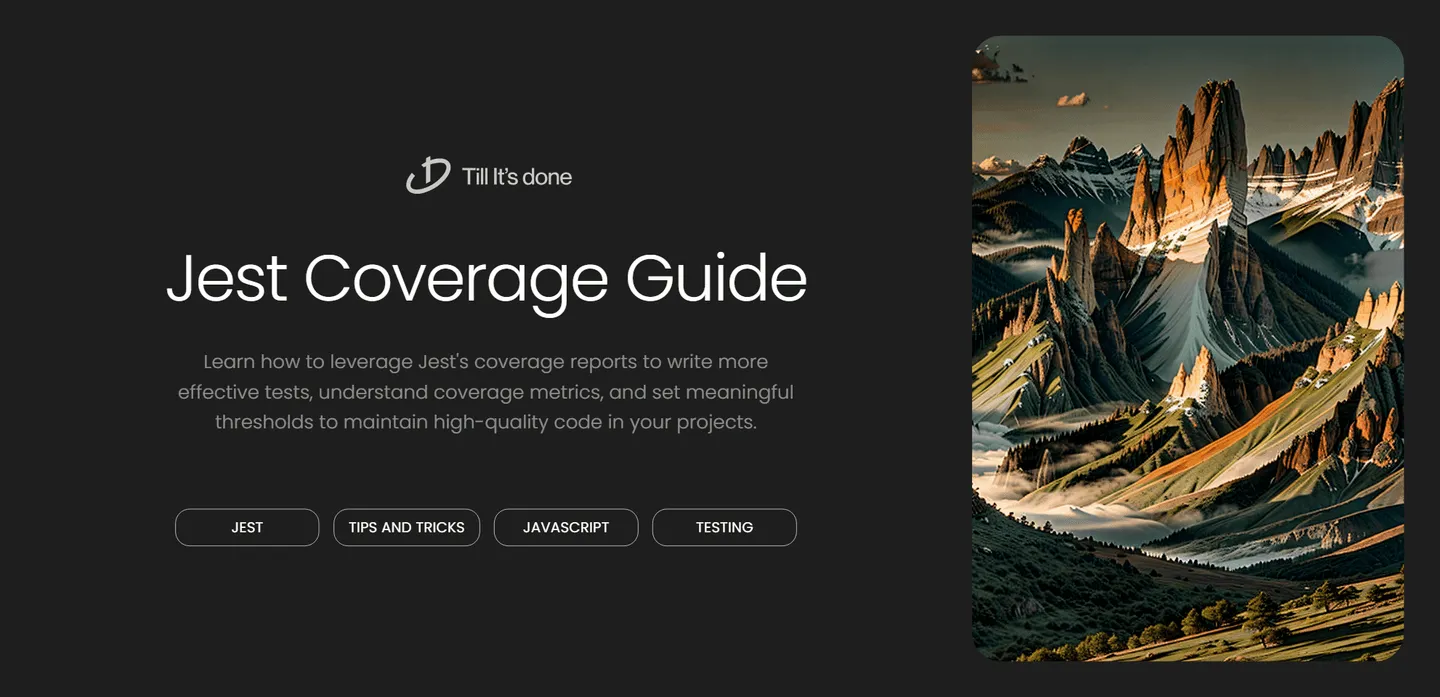
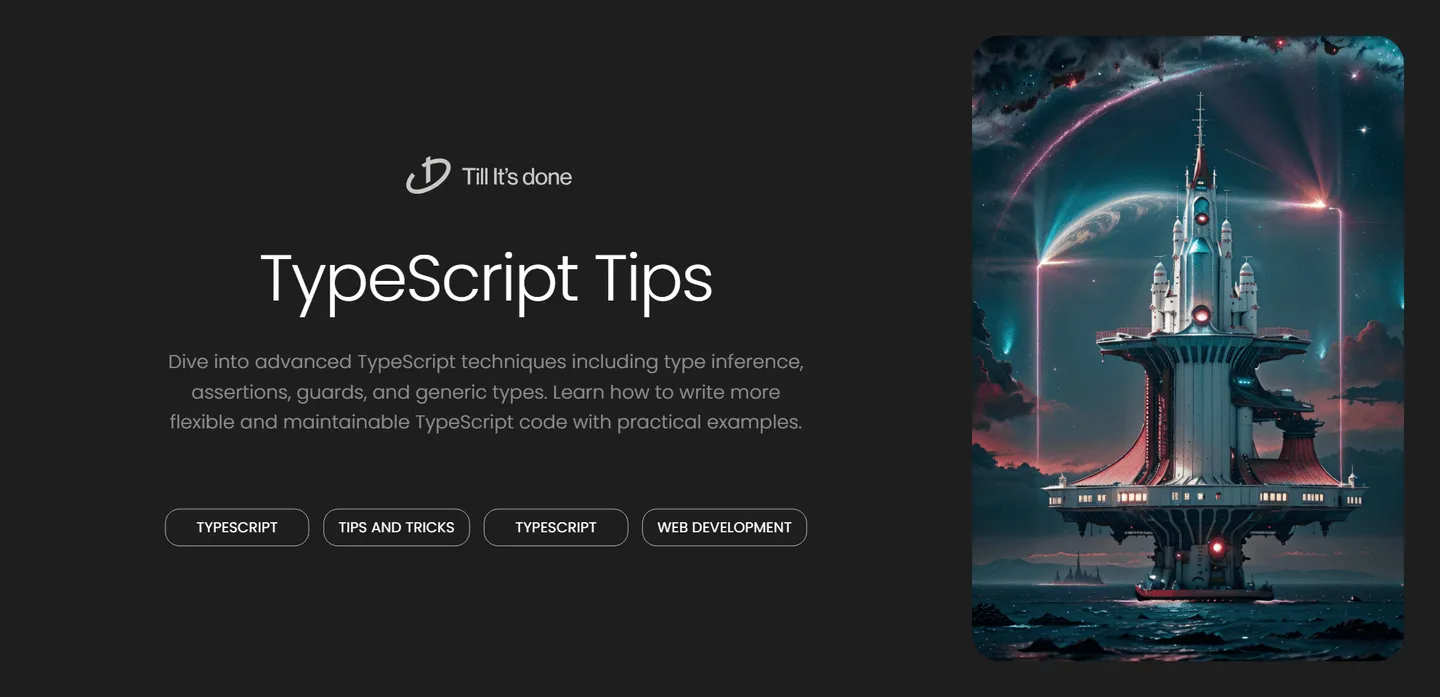
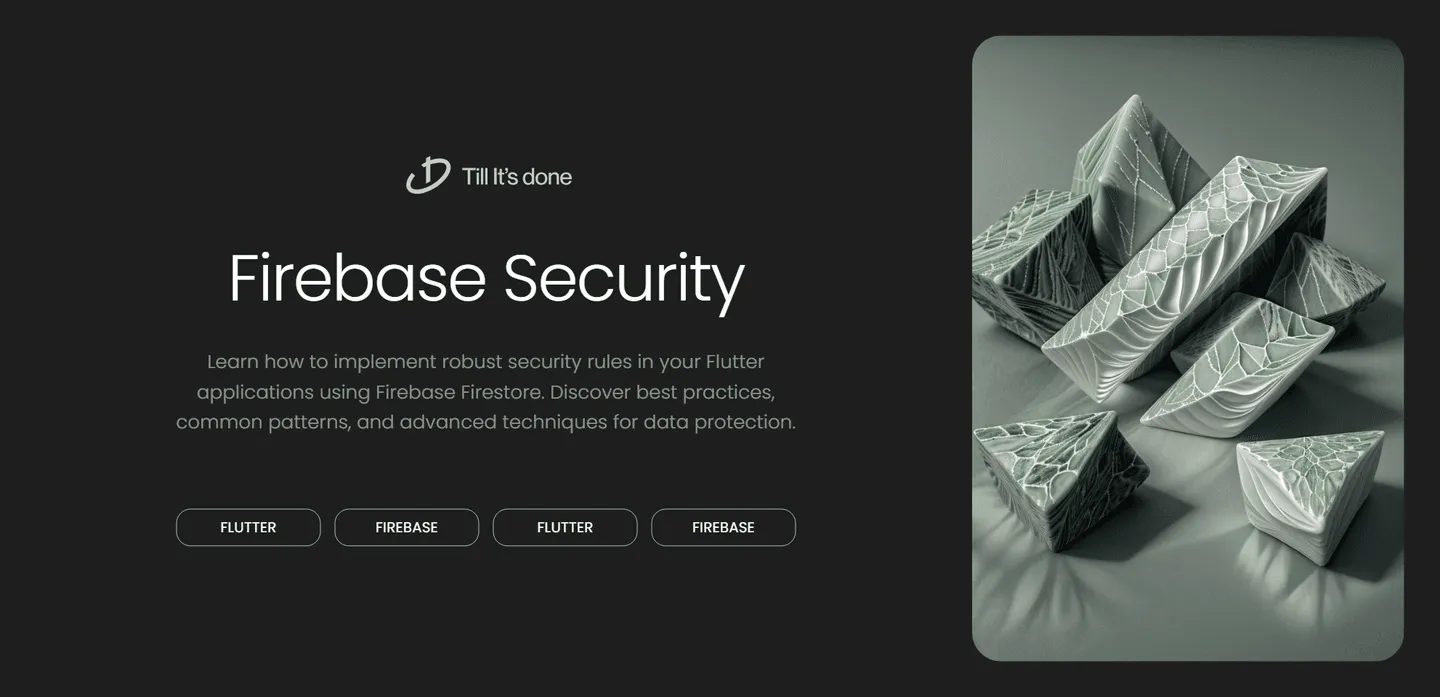
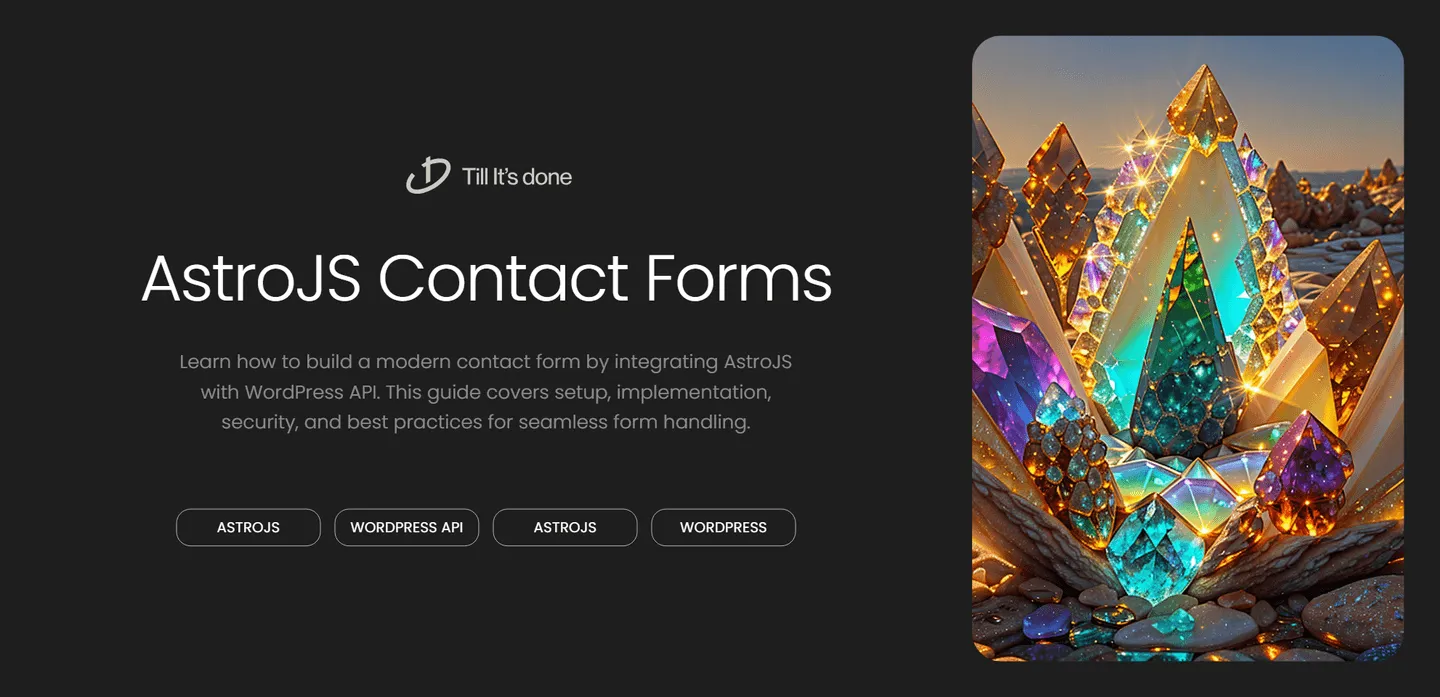
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.