- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Programmatic Navigation with React Router Guide
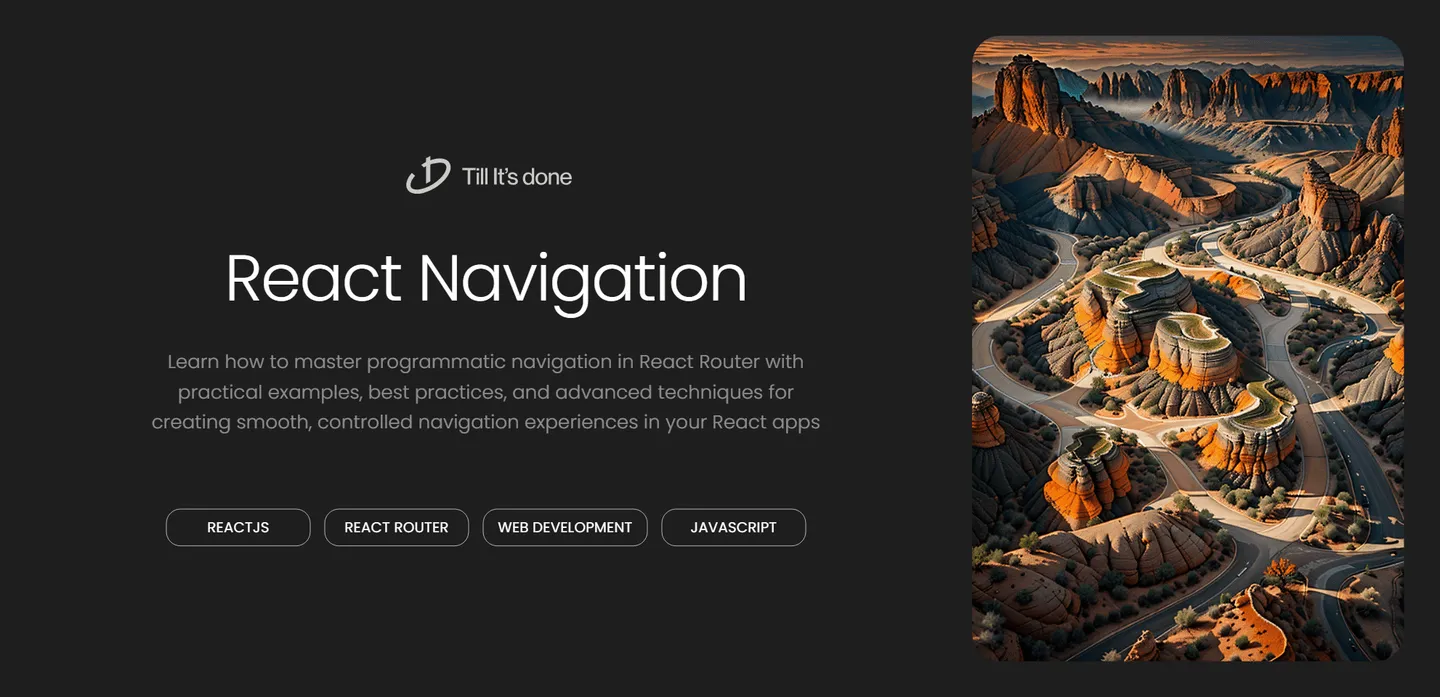
Mastering Programmatic Navigation with React Router
Have you ever needed to navigate users through your React application based on certain conditions or events? Maybe after a successful form submission, or when a timer expires? That’s where programmatic navigation comes in handy! Let’s dive into how we can leverage React Router’s powerful navigation features to create smooth, controlled user experiences.
Understanding Programmatic Navigation
Think of programmatic navigation as your app’s GPS system - instead of users clicking links to move around, your code takes the wheel and guides them to their destination. This becomes super useful when building interactive web applications that need to respond to user actions or system events.
The Navigation Hook Arsenal
React Router provides us with several hooks that make programmatic navigation a breeze. The star of the show is the useNavigate
hook. Here’s how you can use it:
import { useNavigate } from 'react-router-dom';
function LoginForm() { const navigate = useNavigate();
const handleSubmit = async (event) => { event.preventDefault(); // Perform login logic if (loginSuccessful) { navigate('/dashboard'); } };
return ( <form onSubmit={handleSubmit}> {/* form fields */} </form> );}
Advanced Navigation Techniques
Sometimes, simple navigation isn’t enough. Let’s explore some powerful patterns:
State Transfer
navigate('/user-profile', { state: { fromDashboard: true, userSettings: settings }});
Replace vs Push
// Replace current history entrynavigate('/new-page', { replace: true });
// Push new entry (default behavior)navigate('/new-page');
Best Practices
- Always handle navigation errors gracefully
- Use relative paths when possible
- Consider implementing navigation guards
- Keep your routing logic organized and maintainable
Handling Navigation Events
Remember to clean up any listeners or subscriptions when components unmount:
useEffect(() => { const unblock = history.block((tx) => { if (hasUnsavedChanges) { const confirm = window.confirm('You have unsaved changes. Are you sure?'); if (confirm) { unblock(); tx.retry(); } } });
return () => unblock();}, [hasUnsavedChanges]);
Real-World Scenarios
Programmatic navigation shines in many common scenarios:
- After form submissions
- Authentication flows
- Timeout redirects
- Wizard-style forms
- Error boundaries
Remember, with great power comes great responsibility. Use programmatic navigation thoughtfully to enhance user experience, not to create confusing navigation patterns. Happy coding!
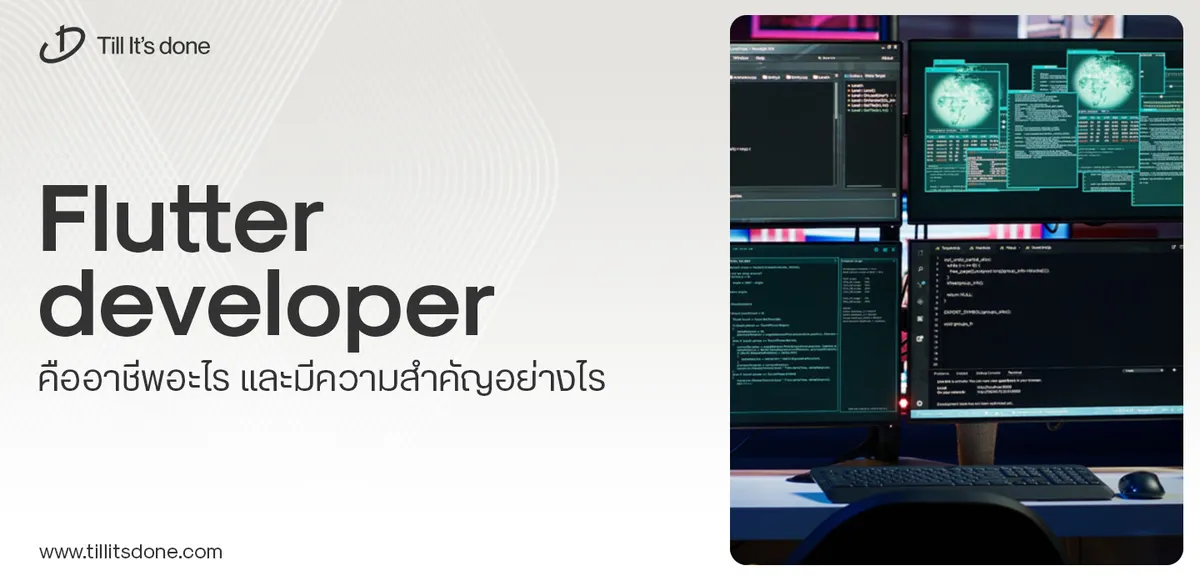
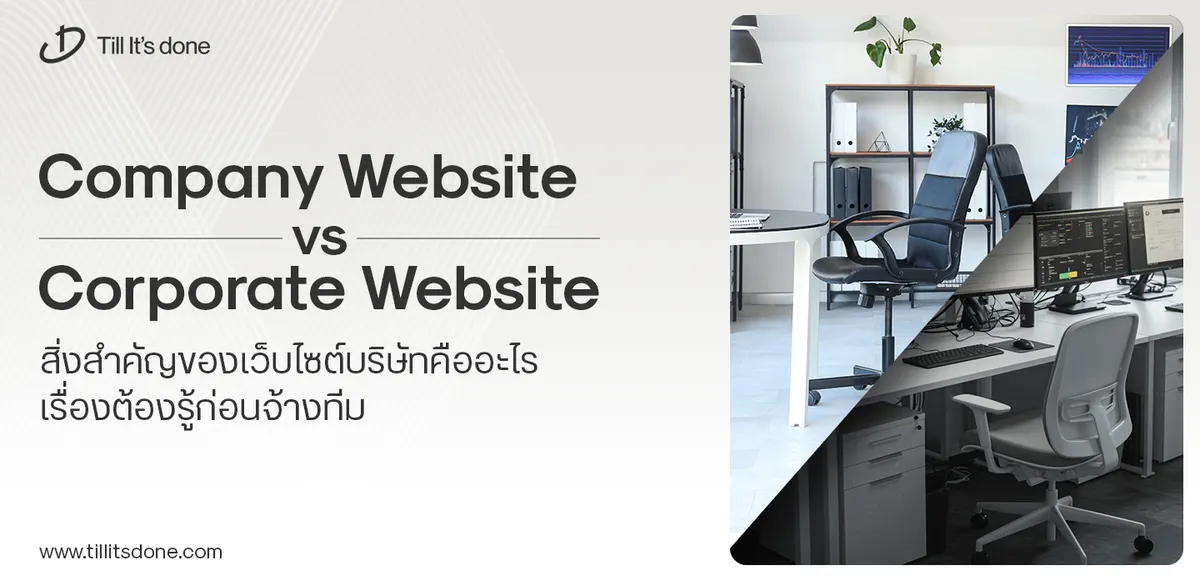
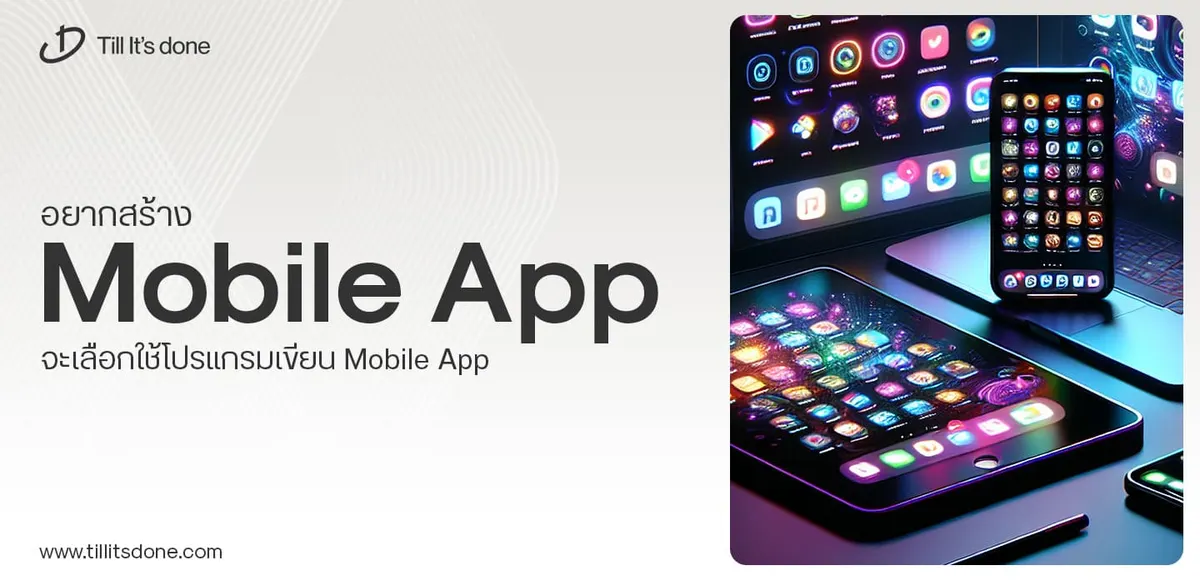
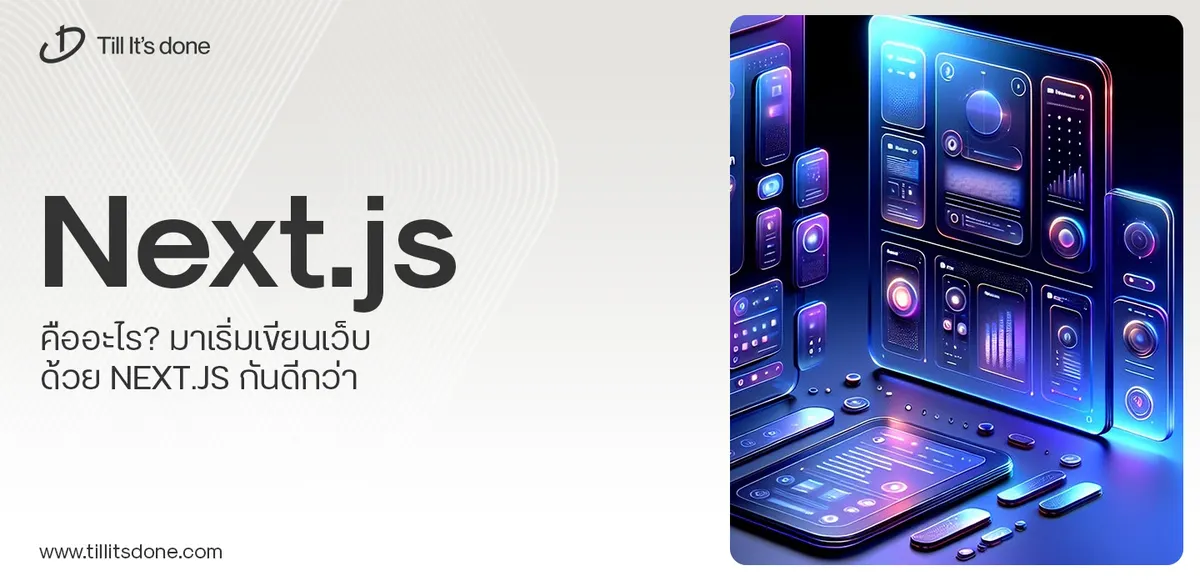
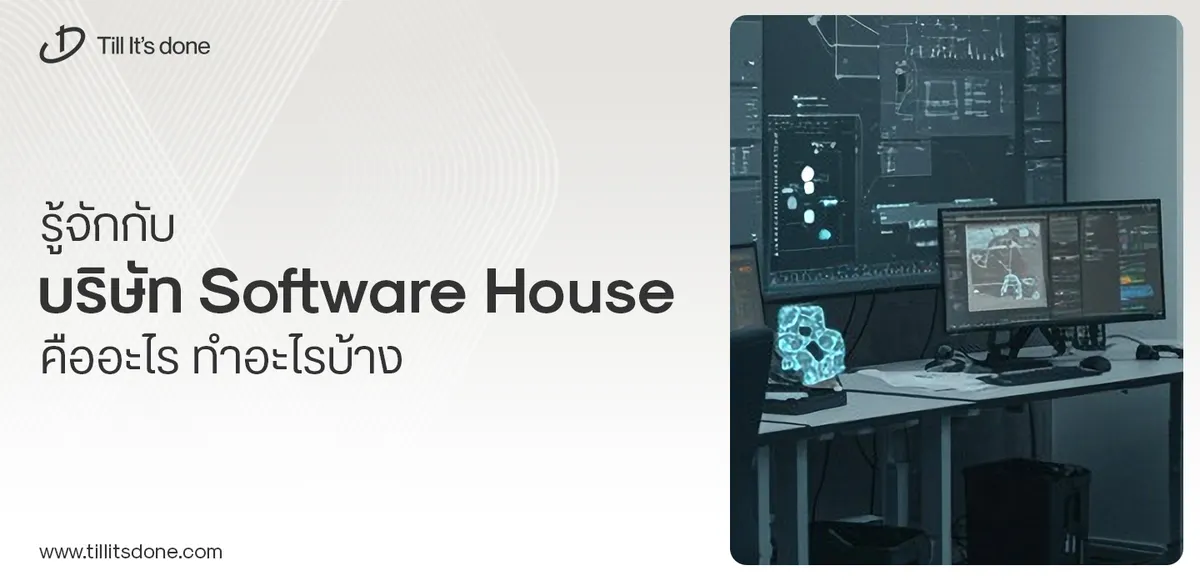
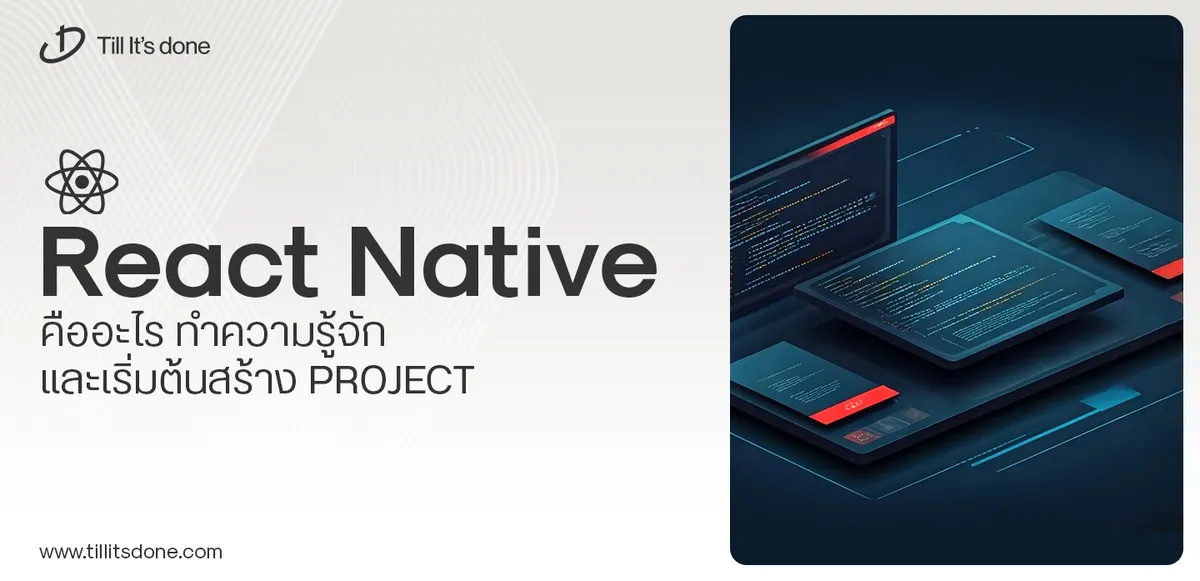
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.