- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Implement Lazy Loading with React Router Guide
Discover best practices, implementation steps, and measuring the impact of code splitting.
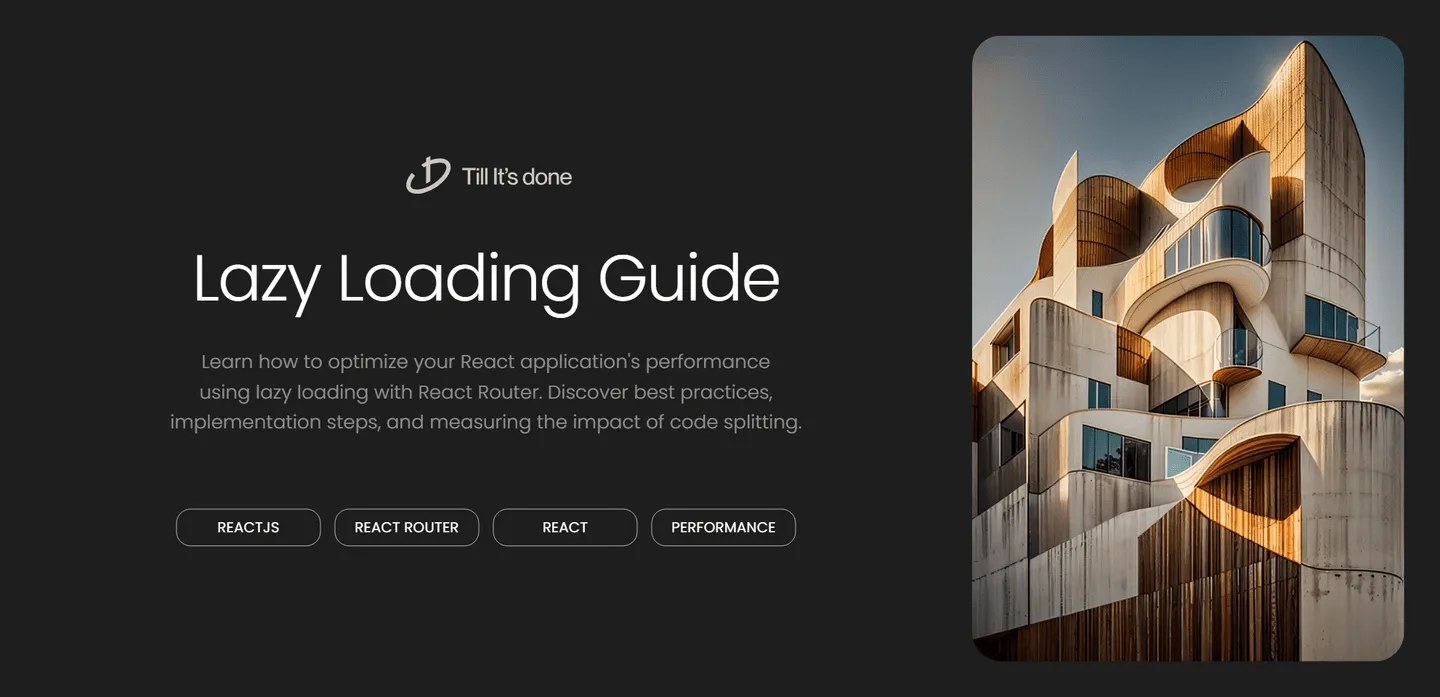
Implementing Lazy Loading with React Router: Boost Your React App’s Performance
Have you ever wondered why some React applications take forever to load? It’s probably because they’re loading everything at once! Let’s dive into how we can fix this using lazy loading with React Router - a game-changing technique that can significantly improve your app’s initial load time.
Understanding the Problem
Picture this: You’ve built an amazing React application with multiple routes, complex components, and tons of features. But as your app grows, so does your bundle size. Every time a user visits your website, their browser needs to download and parse all of this JavaScript - even if they’re only viewing the home page!
Enter Lazy Loading
Lazy loading is like having a smart delivery system for your code. Instead of sending everything at once, you only deliver what’s needed, when it’s needed. React Router makes this incredibly easy to implement.
How to Implement Lazy Loading
Here’s how you can implement lazy loading in your React application:
- First, import the necessary tools:
import { lazy, Suspense } from 'react';import { Routes, Route } from 'react-router-dom';
- Convert your regular imports to lazy imports:
// Instead of this:// import Dashboard from './pages/Dashboard';
// Do this:const Dashboard = lazy(() => import('./pages/Dashboard'));
- Wrap your routes with Suspense:
function App() { return ( <Suspense fallback={<div>Loading...</div>}> <Routes> <Route path="/dashboard" element={<Dashboard />} /> </Routes> </Suspense> );}
Best Practices for Lazy Loading
-
Choose the Right Components: Not everything needs to be lazy loaded. Focus on larger components that aren’t immediately needed.
-
Strategic Route Splitting: Group related routes together in the same chunk if they’re often accessed together.
-
Loading States: Design meaningful loading states that maintain your app’s visual consistency.
Measuring the Impact
After implementing lazy loading, you should see:
- Reduced initial bundle size
- Faster initial page load
- Better performance metrics
- Improved user experience
Remember, lazy loading isn’t just about splitting your code - it’s about creating a better experience for your users by delivering content faster and more efficiently.
Conclusion
Implementing lazy loading with React Router is a powerful optimization technique that can significantly improve your application’s performance. By loading code only when it’s needed, you’re not just making your app faster - you’re showing respect for your users’ time and resources.
Start implementing lazy loading in your React applications today, and watch your performance metrics improve dramatically!
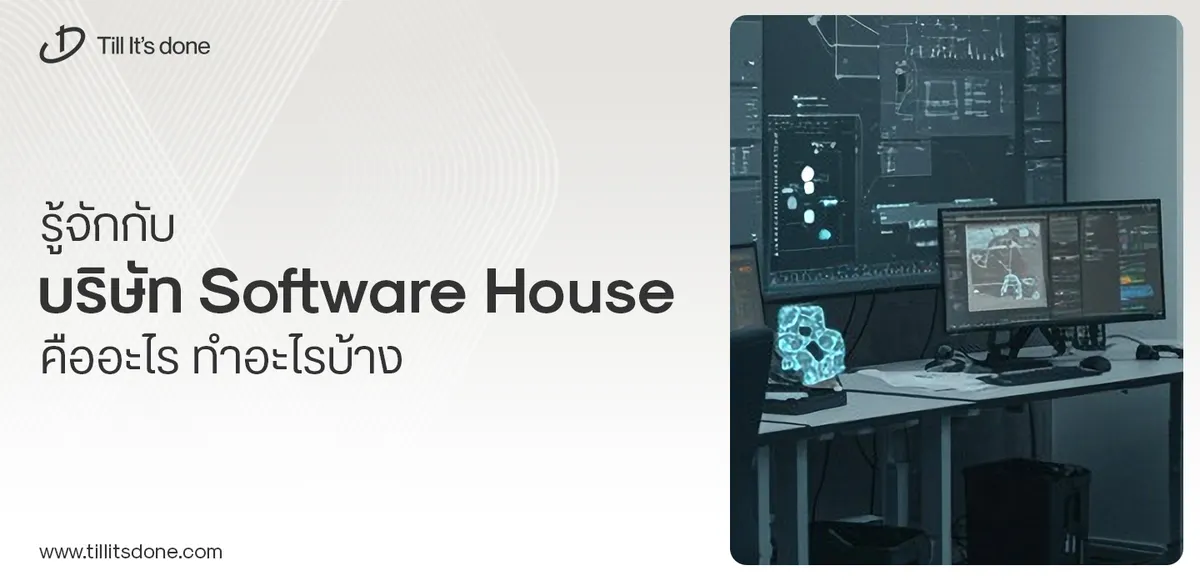
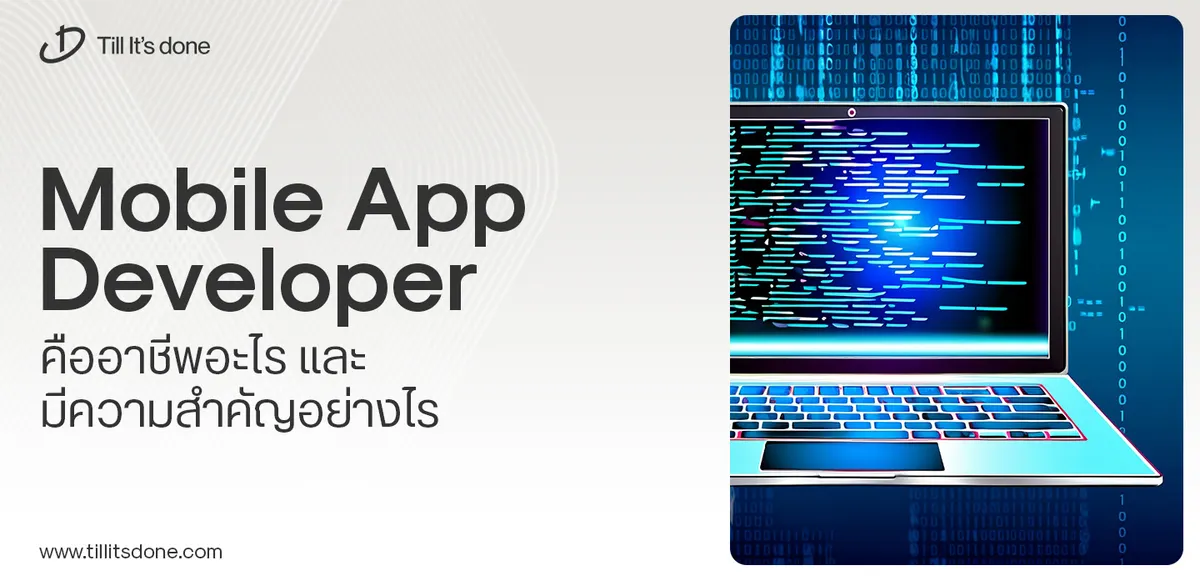
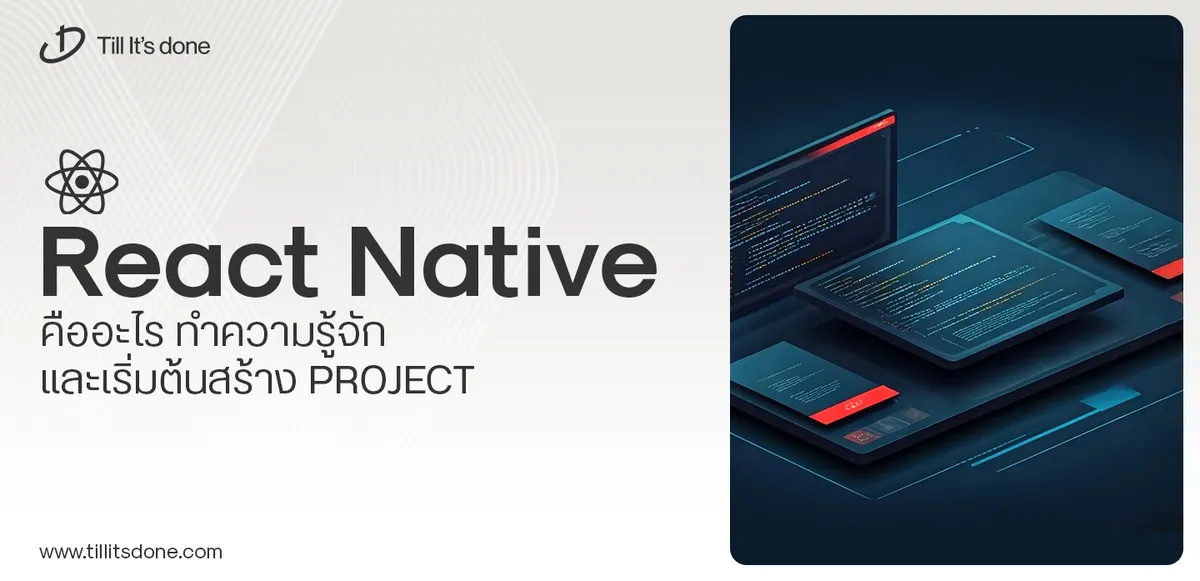
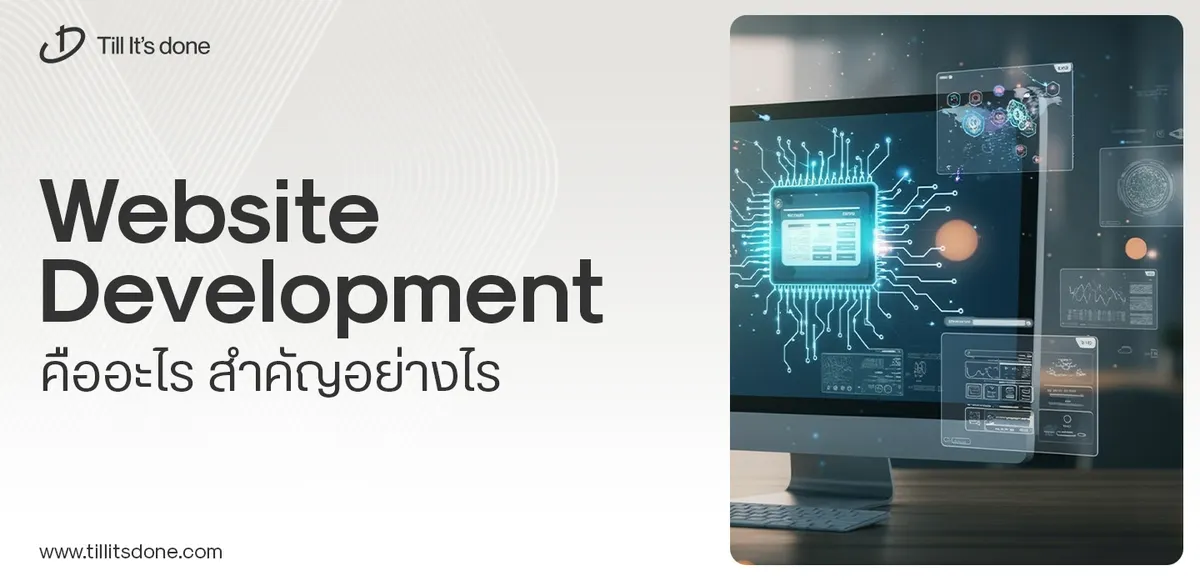
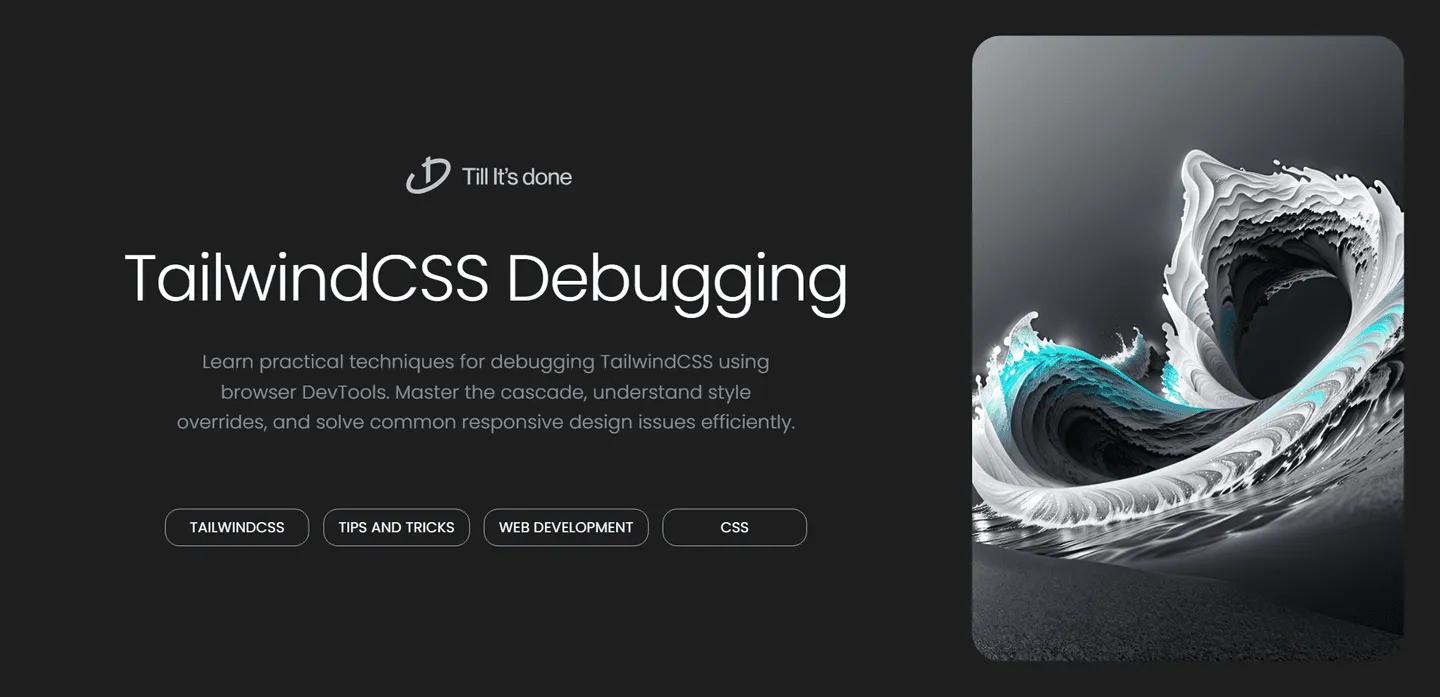
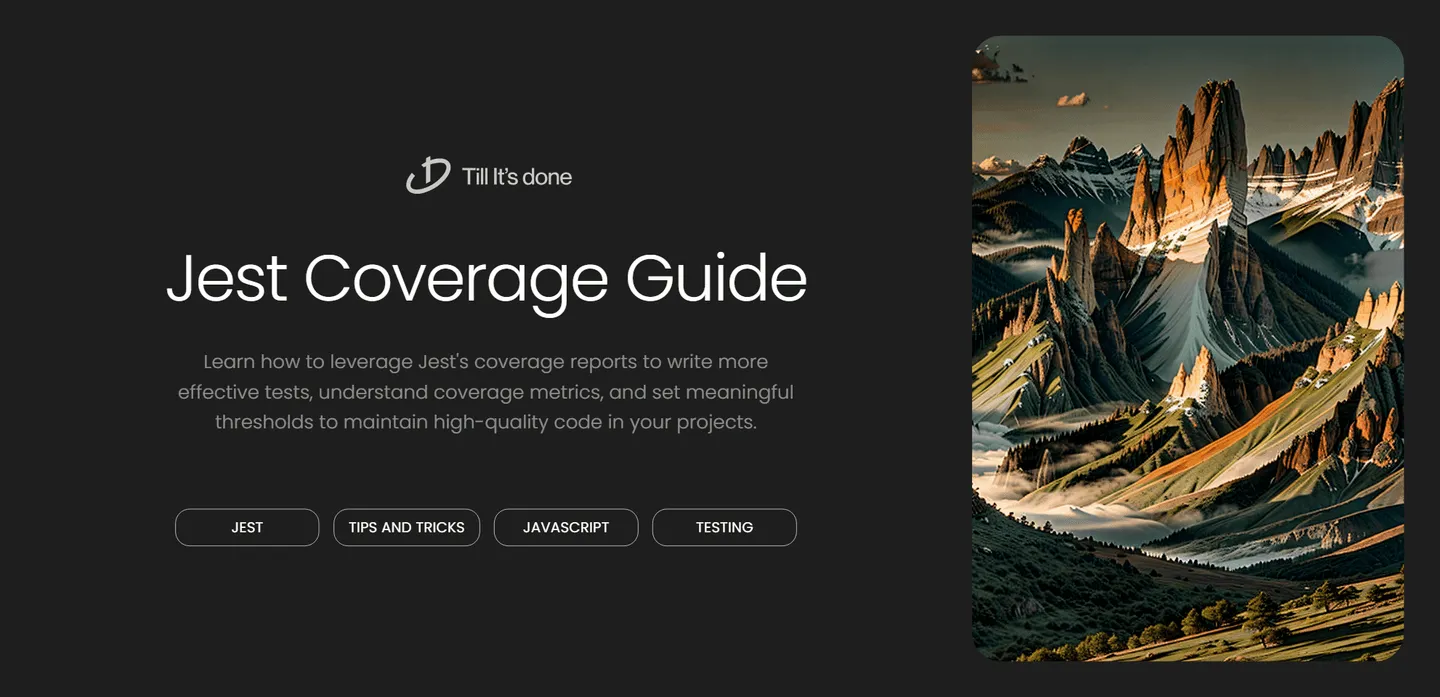
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.