- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Optimizing Component Re-renders in React
Discover how to measure and improve your app's performance.

Optimizing Component Re-renders in React: A Deep Dive
Ever watched a sluggish React application struggle to keep up with user interactions? We’ve all been there. Today, let’s explore how to make your React apps lightning-fast by mastering component re-renders.
Understanding React’s Re-rendering Behavior
Think of React components as eager performers – they want to show their latest and greatest version whenever something changes. However, this enthusiasm can sometimes lead to unnecessary performance costs.
The Common Pitfalls
-
Prop Reference Changes When passing objects or arrays as props, a new reference creates a re-render, even if the content hasn’t changed. It’s like changing the label on a box while the contents remain the same – unnecessary work!
-
State Updates in Parent Components When a parent component updates its state, all child components re-render by default. Imagine a conductor making the entire orchestra restart because one musician missed a note.
Smart Solutions for Better Performance
1. React.memo: Your First Line of Defense
React.memo is like a bouncer for your components. It checks if the new props are different from the previous ones before allowing a re-render. Here’s when to use it:
- For components that receive the same props frequently
- When render is computationally expensive
- For components that are in the middle of a deep component tree
2. useMemo and useCallback: The Dynamic Duo
These hooks are your best friends when dealing with expensive calculations or callback functions. They help maintain reference stability, crucial for optimizing child component renders.
3. State Management Best Practices
Keep your state as local as possible. It’s like organizing a city – you don’t need the entire city to know about a neighborhood block party!
Advanced Optimization Techniques
1. Virtual Lists
When dealing with long lists, consider virtualizing them. Only render what’s visible in the viewport – it’s like loading cards in a deck only when you need to see them.
2. Code Splitting
Break your application into smaller chunks. Load components only when needed, like chapters in a book – you don’t need to open the entire book to read one chapter.
3. Debouncing and Throttling
Control the frequency of updates, especially for resource-intensive operations like search inputs or window resize handlers.
Measuring Performance
Remember to measure before and after optimization. React DevTools’ Profiler is your best friend here. It’s like having a speedometer for your components – you can’t improve what you can’t measure.
Conclusion
Optimization isn’t about applying every technique everywhere. It’s about understanding your application’s needs and applying the right solutions at the right places. Start with the basics, measure the impact, and gradually implement more advanced techniques as needed.
Remember, the best optimization is often the one you don’t need to make – write clean, thoughtful code from the start, and you’ll have fewer performance issues to fix later.



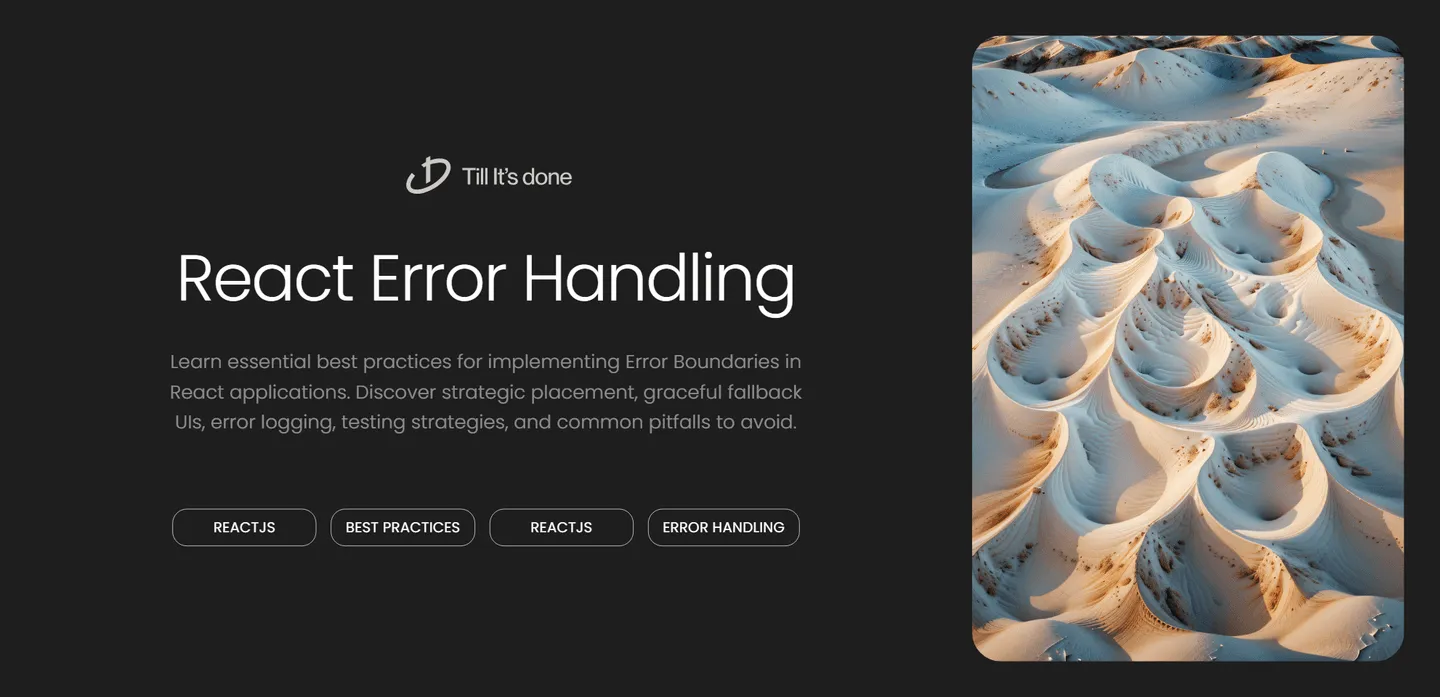


Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.