- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
React Query: Master Pagination & Infinite Scroll
Discover best practices, code examples, and pro tips for better UX.
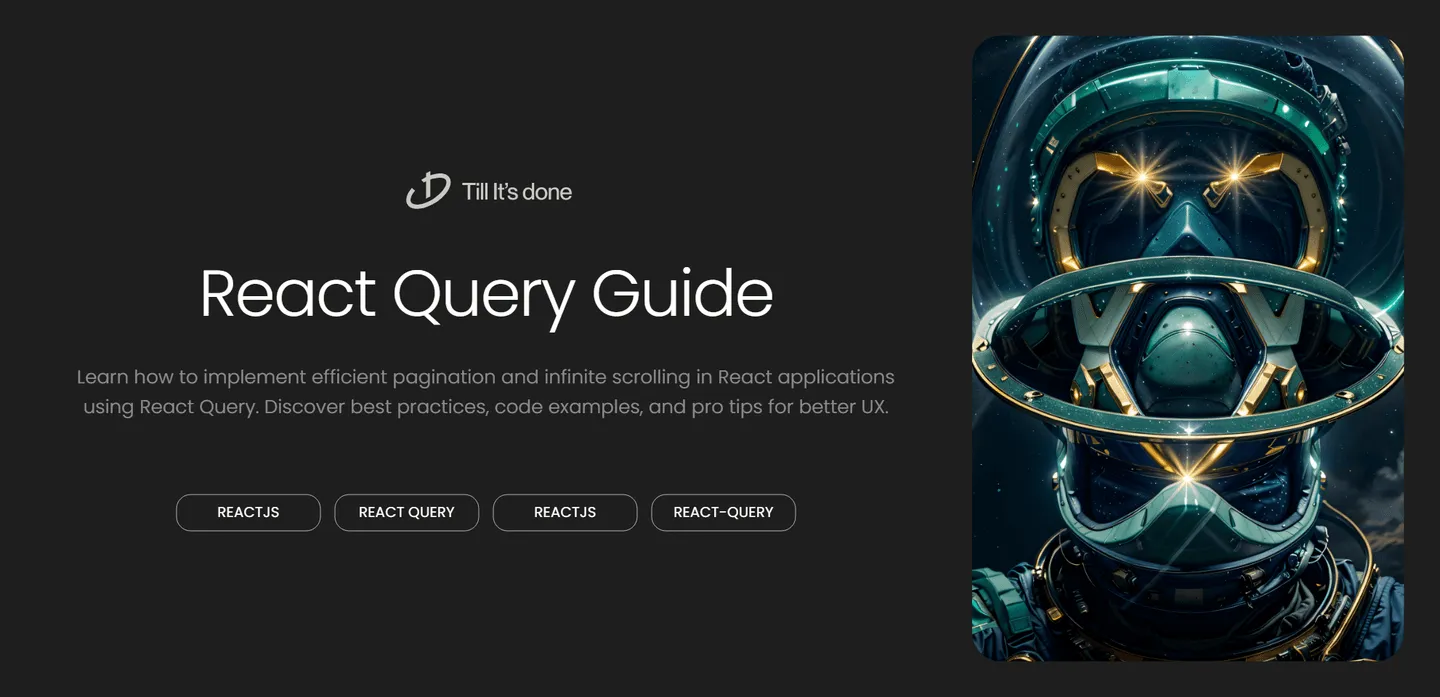
Ever found yourself wrestling with pagination in React? You’re not alone. Today, let’s dive into how React Query makes handling paginated data and infinite scrolling a breeze. Trust me, once you see how elegant this solution is, you’ll wonder why you didn’t try it sooner.
The Traditional Pagination Headache
We’ve all been there - managing page numbers, loading states, and cached data while trying to keep our UI smooth and responsive. It’s like trying to juggle while riding a unicycle. Not fun.
Enter React Query
React Query transforms this complexity into something beautifully simple. Let’s look at how we can implement both traditional pagination and infinite scrolling.
Traditional Pagination Implementation
Here’s a real-world example that you can drop into your project:
const usePaginatedPosts = (page) => { return useQuery({ queryKey: ['posts', page], queryFn: () => fetchPosts(page), keepPreviousData: true });}
function PostList() { const [page, setPage] = useState(1); const { data, isLoading, isFetching } = usePaginatedPosts(page);
if (isLoading) return <div>Loading posts...</div>;
return ( <div> {data.posts.map(post => ( <PostCard key={post.id} post={post} /> ))} <div> <button onClick={() => setPage(old => Math.max(old - 1, 1))} disabled={page === 1} > Previous Page </button> <span>Page {page}</span> <button onClick={() => setPage(old => old + 1)} disabled={!data.hasMore} > Next Page </button> </div> </div> );}
Infinite Scrolling Magic
Now, let’s look at how React Query handles infinite scrolling - it’s surprisingly straightforward:
const useInfinitePosts = () => { return useInfiniteQuery({ queryKey: ['posts'], queryFn: fetchPostPage, getNextPageParam: (lastPage) => lastPage.nextCursor, });}
function InfinitePostList() { const { data, fetchNextPage, hasNextPage, isFetchingNextPage } = useInfinitePosts();
return ( <div> {data.pages.map(page => ( page.posts.map(post => ( <PostCard key={post.id} post={post} /> )) ))}
<button onClick={() => fetchNextPage()} disabled={!hasNextPage || isFetchingNextPage} > {isFetchingNextPage ? 'Loading more...' : hasNextPage ? 'Load More' : 'Nothing more to load'} </button> </div> );}
The beauty of React Query’s solution lies in its automatic handling of cache invalidation, loading states, and error boundaries. It’s like having a smart assistant that manages all the complex state logic while you focus on building great user experiences.
Pro Tips
- Always use
keepPreviousData: true
for smoother transitions between pages - Implement proper error boundaries for production-ready code
- Consider prefetching the next page for instant loading
- Use
suspense: true
for even cleaner loading states
React Query transforms pagination from a necessary evil into a delightful implementation detail. Whether you’re building a simple blog or a complex data table, these patterns will serve you well.
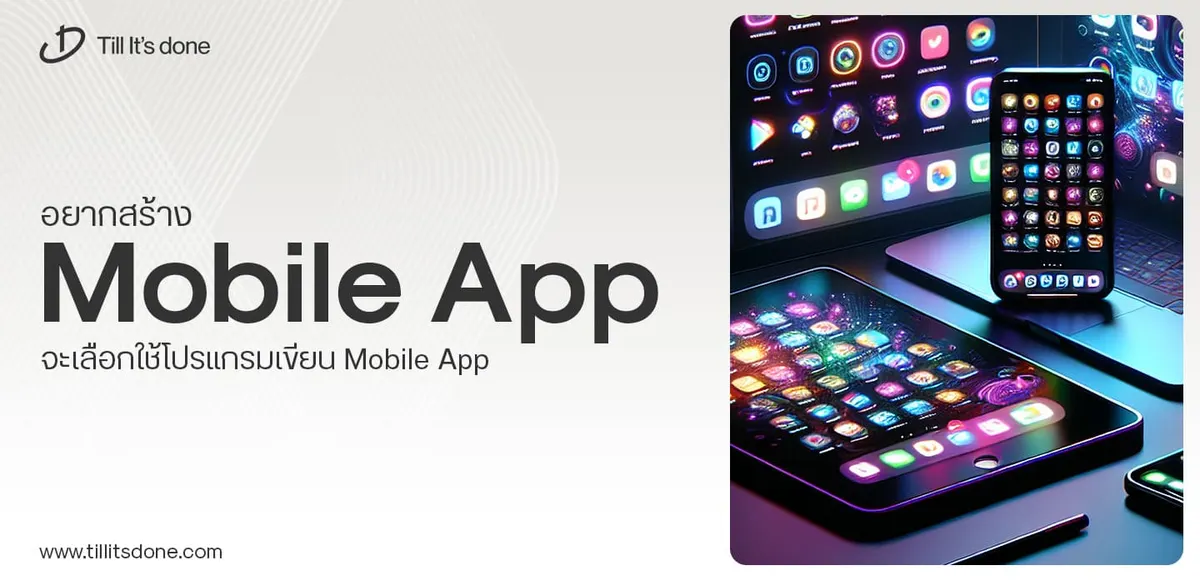
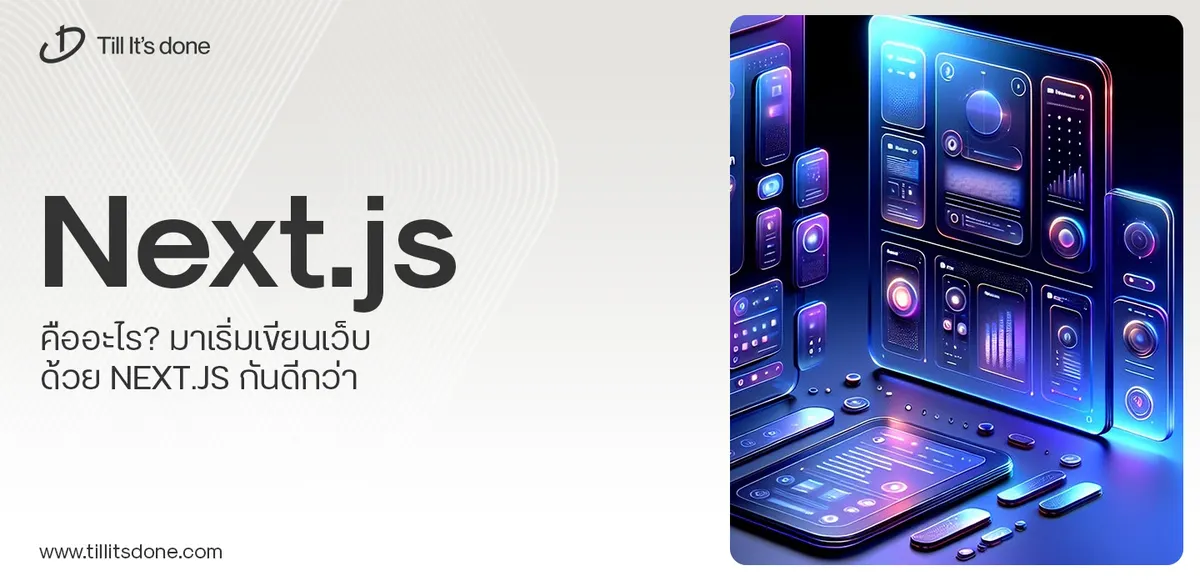
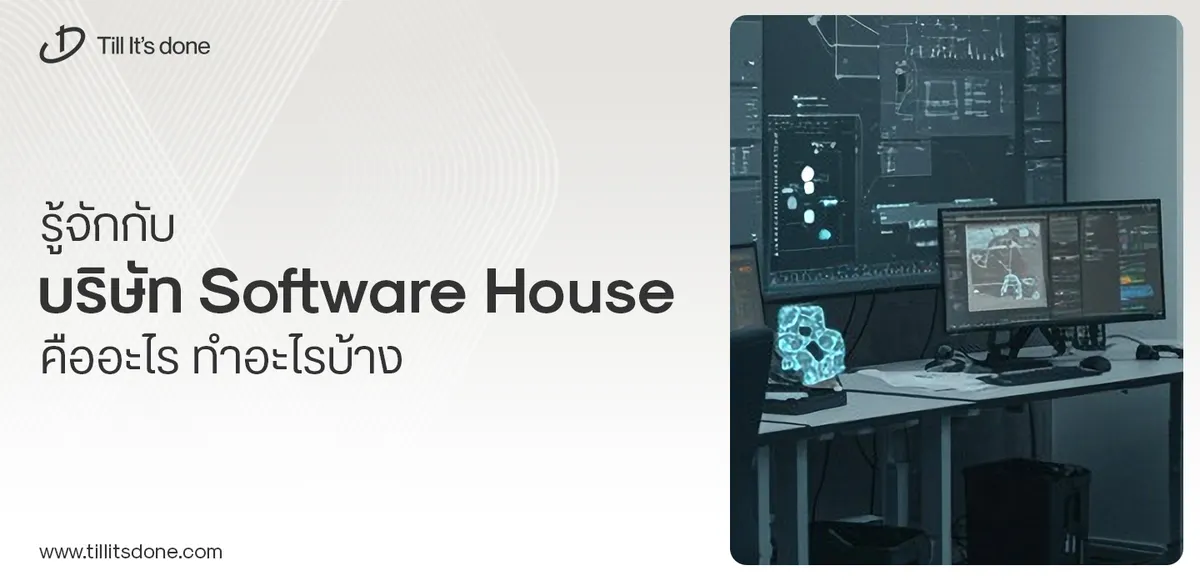
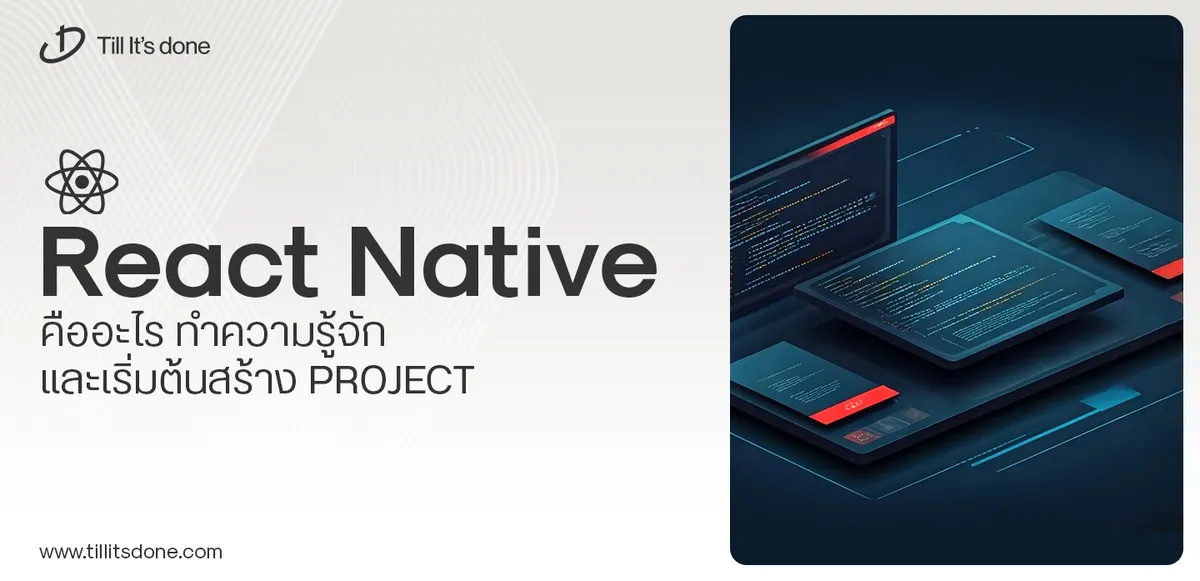
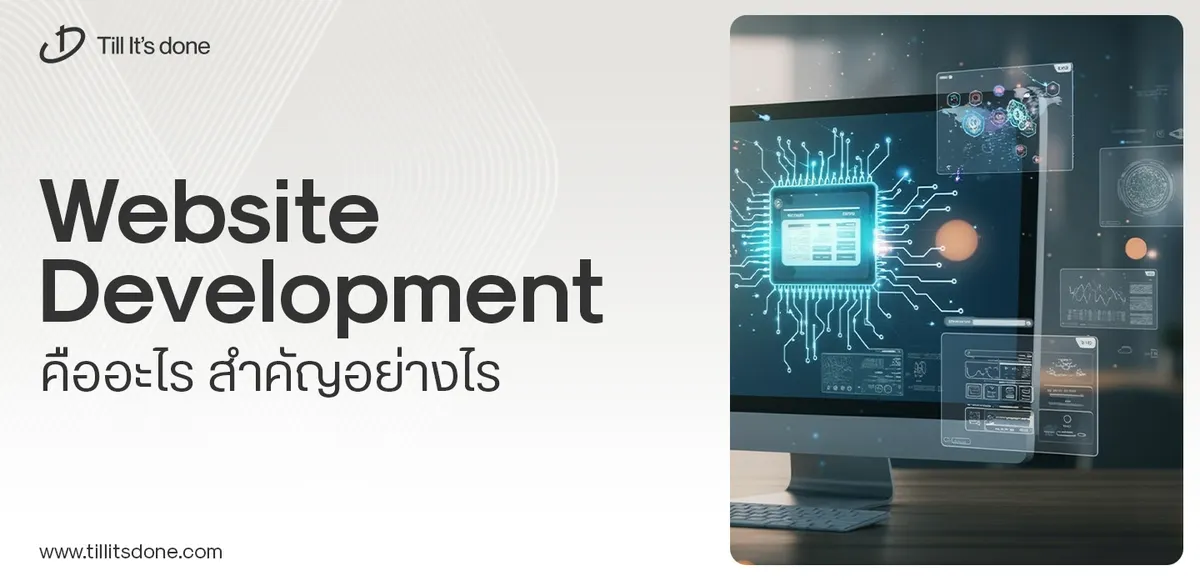
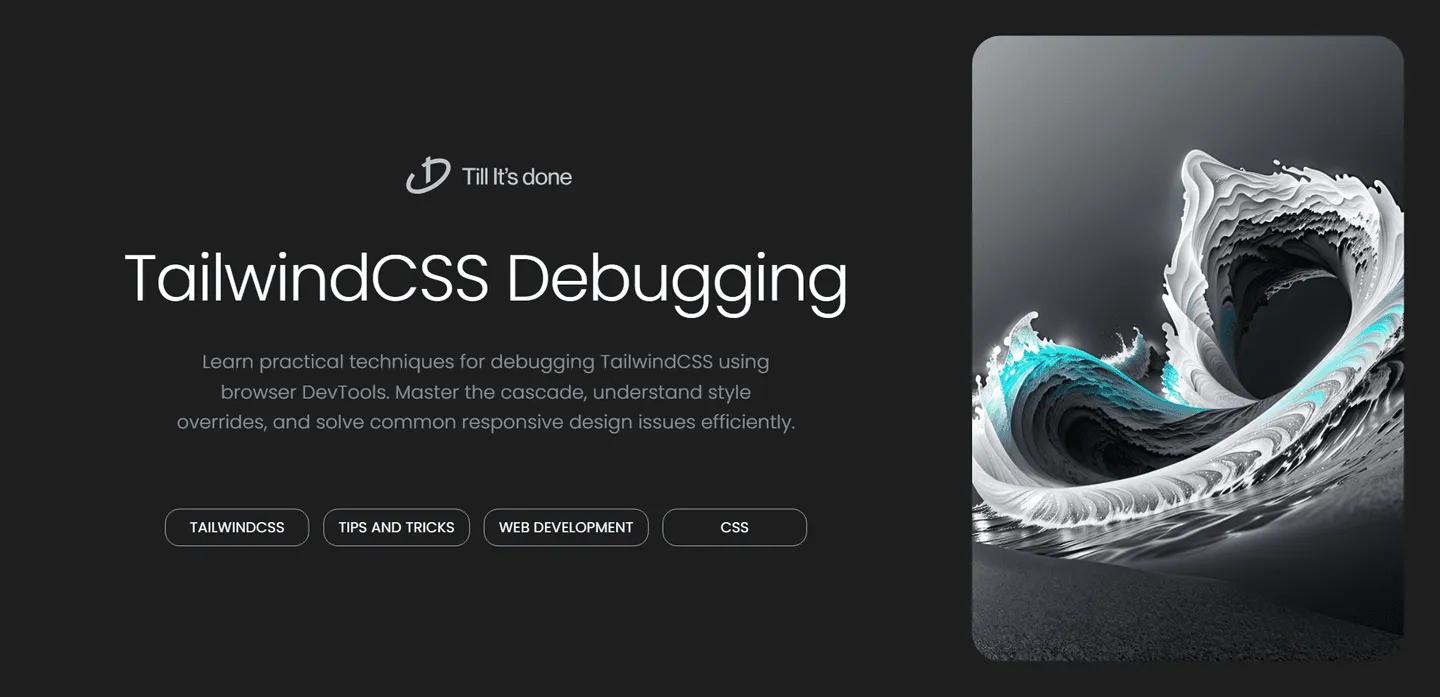
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.