- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Implement Pagination in React with Apollo Client
Master data handling with practical examples and best practices.
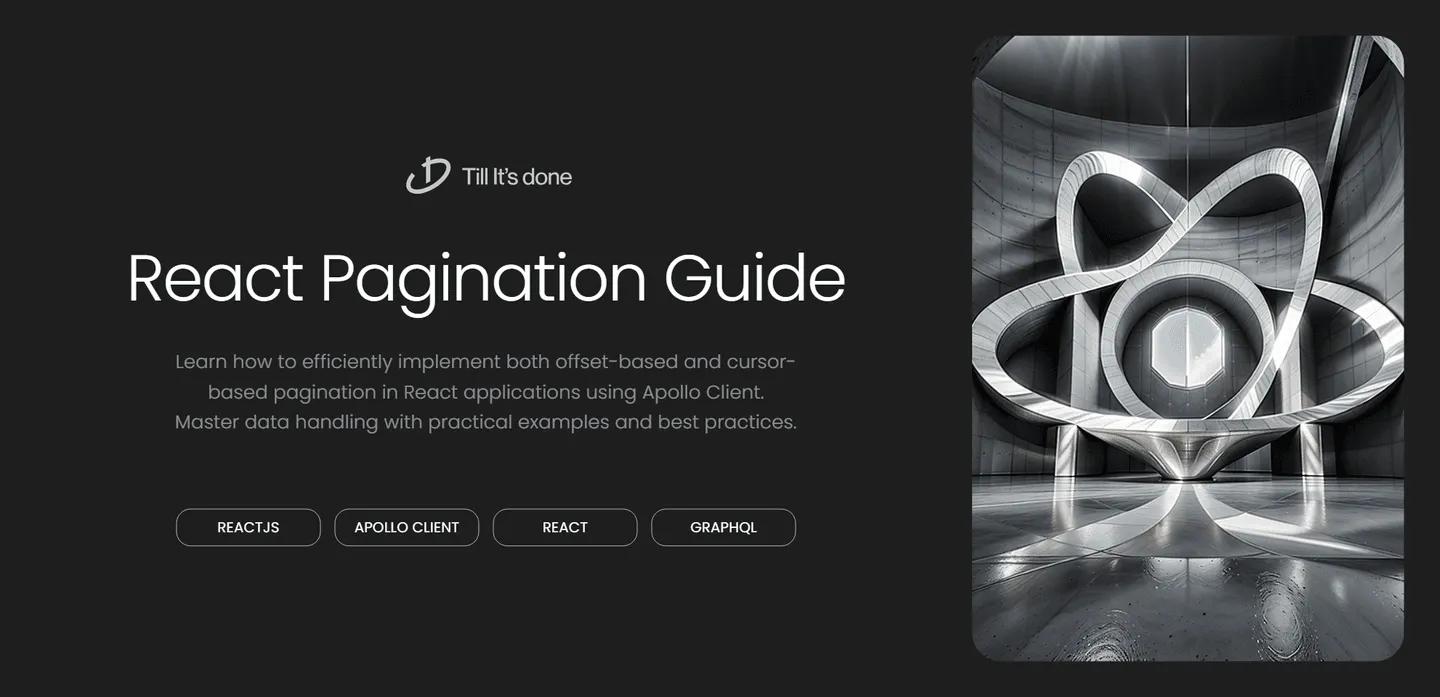
Implementing Pagination in React using Apollo Client: A Comprehensive Guide
In today’s data-driven applications, handling large datasets efficiently is crucial for optimal performance. One of the most effective ways to manage this is through pagination. Let’s dive into implementing pagination in React using Apollo Client, making our data handling both elegant and efficient.
Understanding the Basics
When working with GraphQL and Apollo Client, we have several pagination strategies at our disposal. The two most common approaches are:
- Offset-based pagination
- Cursor-based pagination
Let’s explore both methods and understand when to use each one.
Setting Up Apollo Client
Before we dive into implementation, let’s ensure our Apollo Client setup is properly configured. First, we’ll need to install the necessary dependencies and set up our Apollo Client instance. This forms the foundation of our pagination implementation.
Basic Setup Code
import { ApolloClient, InMemoryCache } from '@apollo/client';
const client = new ApolloClient({ uri: 'YOUR_GRAPHQL_ENDPOINT', cache: new InMemoryCache({ typePolicies: { Query: { fields: { posts: { // Merge function for pagination keyArgs: false, merge(existing = [], incoming) { return [...existing, ...incoming]; }, }, }, }, }, }),});
Implementing Offset-based Pagination
Offset-based pagination is straightforward and works well for static data. Here’s how we can implement it:
const GET_POSTS = gql` query GetPosts($offset: Int, $limit: Int) { posts(offset: $offset, limit: $limit) { id title content } }`;
Implementing Cursor-based Pagination
For real-time data and better performance with large datasets, cursor-based pagination is often the better choice. Here’s how we implement it:
const GET_POSTS_CURSOR = gql` query GetPosts($cursor: String, $limit: Int) { posts(after: $cursor, first: $limit) { edges { node { id title content } cursor } pageInfo { hasNextPage endCursor } } }`;
Best Practices and Optimization Tips
- Always implement error boundaries for failed queries
- Use loading states effectively
- Consider implementing infinite scroll for better UX
- Cache results properly to minimize unnecessary network requests
- Implement proper error handling and retry mechanisms
Conclusion
Implementing pagination with Apollo Client gives us a powerful way to handle large datasets in React applications. Whether you choose offset-based or cursor-based pagination depends on your specific use case, but both approaches can be implemented efficiently using Apollo Client.






Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.