- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Using React Hooks: Best Practices Guide 2024
Learn about useState lazy initialization, useCallback, useMemo, dependency arrays, and custom hooks for better React apps.

Using React Hooks: Best Practices for Performance
If you’ve been working with React for a while, you’ve probably encountered React Hooks. These powerful features have revolutionized how we write components, but like any tool, they need to be used wisely. Today, let’s dive into some battle-tested best practices that’ll help you write performant applications with React Hooks.
Understanding the Re-rendering Cycle
One of the most common performance pitfalls comes from unnecessary re-renders. Think of your component as a sensitive instrument - every state change causes it to play its tune (re-render), but we want to make sure it only plays when necessary.
1. Optimize useState with Lazy Initialization
When your initial state requires expensive calculations, use the lazy initialization pattern:
// ❌ Expensive calculation runs on every renderconst [items, setItems] = useState(calculateExpensiveInitialState());
// ✅ Expensive calculation runs only onceconst [items, setItems] = useState(() => calculateExpensiveInitialState());
2. Master useCallback and useMemo
Think of these hooks as your performance preservation tools. They’re like taking a snapshot of your functions and values, only updating them when absolutely necessary.
const memoizedCallback = useCallback(() => { doSomething(a, b);}, [a, b]); // Only changes if a or b changes
const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
3. Dependencies Array: Your Best Friend
The dependencies array is like a watchlist for your hooks. Keep it honest and complete:
// ❌ Missing dependenciesuseEffect(() => { setTotal(quantity * price);}, []); // Will not update when quantity or price changes
// ✅ Complete dependenciesuseEffect(() => { setTotal(quantity * price);}, [quantity, price]);
4. Custom Hooks: Your Secret Weapon
Extract common patterns into custom hooks. This not only makes your code more reusable but also easier to optimize:
function useWindowSize() { const [size, setSize] = useState({ width: window.innerWidth, height: window.innerHeight });
useEffect(() => { const handleResize = () => { setSize({ width: window.innerWidth, height: window.innerHeight }); };
window.addEventListener('resize', handleResize); return () => window.removeEventListener('resize', handleResize); }, []);
return size;}
5. Context Optimization
When using Context, split your context into smaller pieces to prevent unnecessary re-renders:
// ❌ One large contextconst AppContext = createContext();
// ✅ Split into focused contextsconst UserContext = createContext();const ThemeContext = createContext();const SettingsContext = createContext();
Final Thoughts
Remember, performance optimization is a journey, not a destination. Start with writing clean, readable code, and optimize when measurements show it’s needed. These best practices aren’t rules set in stone but guidelines to help you make informed decisions.
Always profile your application with React DevTools before applying optimizations. Sometimes, what seems like a performance improvement might actually add unnecessary complexity without meaningful benefits.
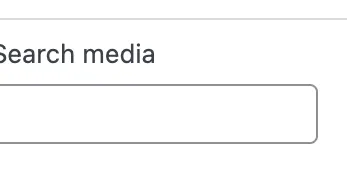





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.