- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
React Hook Form + Material-UI Integration Guide
Includes best practices and advanced tips.

Building forms in React can be challenging, especially when dealing with complex validation and state management. Today, I’m excited to share my experience combining two powerful libraries: React Hook Form and Material-UI. This integration has become my go-to solution for creating robust, beautiful forms with minimal effort.
Why Choose This Combination?
React Hook Form brings excellent form handling with minimal re-renders and great performance, while Material-UI provides polished, customizable form components. Together, they’re like peanut butter and jelly – a perfect match!
Setting Up Your Form
First, let’s create a basic login form that showcases this integration. The secret sauce is using the Controller
component from React Hook Form to wrap Material-UI components. Here’s how:
import { useForm, Controller } from 'react-hook-form';import { TextField, Button, Box } from '@mui/material';
const LoginForm = () => { const { control, handleSubmit } = useForm({ defaultValues: { email: '', password: '' } });
const onSubmit = (data) => console.log(data);
return ( <Box component="form" onSubmit={handleSubmit(onSubmit)}> <Controller name="email" control={control} rules={{ required: 'Email is required', pattern: /^\S+@\S+$/i }} render={({ field, fieldState: { error } }) => ( <TextField {...field} label="Email" error={!!error} helperText={error?.message} fullWidth margin="normal" /> )} /> </Box> );};
Advanced Validation Techniques
One of my favorite features is how seamlessly we can add complex validation. Let’s look at a real-world example:
const schema = yup.object().shape({ password: yup .string() .required('Password is required') .min(8, 'Password must be at least 8 characters') .matches(/[A-Z]/, 'Password must contain at least one uppercase letter'), confirmPassword: yup .string() .oneOf([yup.ref('password')], 'Passwords must match')});
Tips for Better Performance
Here’s something not many developers know: React Hook Form’s Controller
component is optimized for performance, but we can make it even better. I’ve found that using the shouldUnregister
option strategically can prevent unnecessary re-renders:
<Controller name="dynamicField" control={control} shouldUnregister={true} // ... rest of the props/>
Styling Best Practices
Material-UI’s theme customization is powerful, but it’s essential to maintain consistency. I prefer creating a custom theme that matches our form components:
const theme = createTheme({ components: { MuiTextField: { styleOverrides: { root: { '& .MuiOutlinedInput-root': { borderRadius: 8, } } } } }});
Conclusion
The combination of React Hook Form and Material-UI has revolutionized how I build forms. It’s not just about writing less code – it’s about creating better user experiences with robust validation and beautiful interfaces.
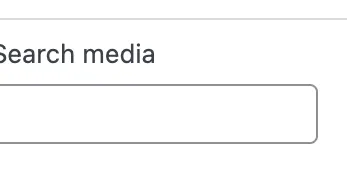





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.