- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Best Practices for Error Boundaries in React
Discover strategic placement, graceful fallback UIs, error logging, testing strategies, and common pitfalls to avoid.
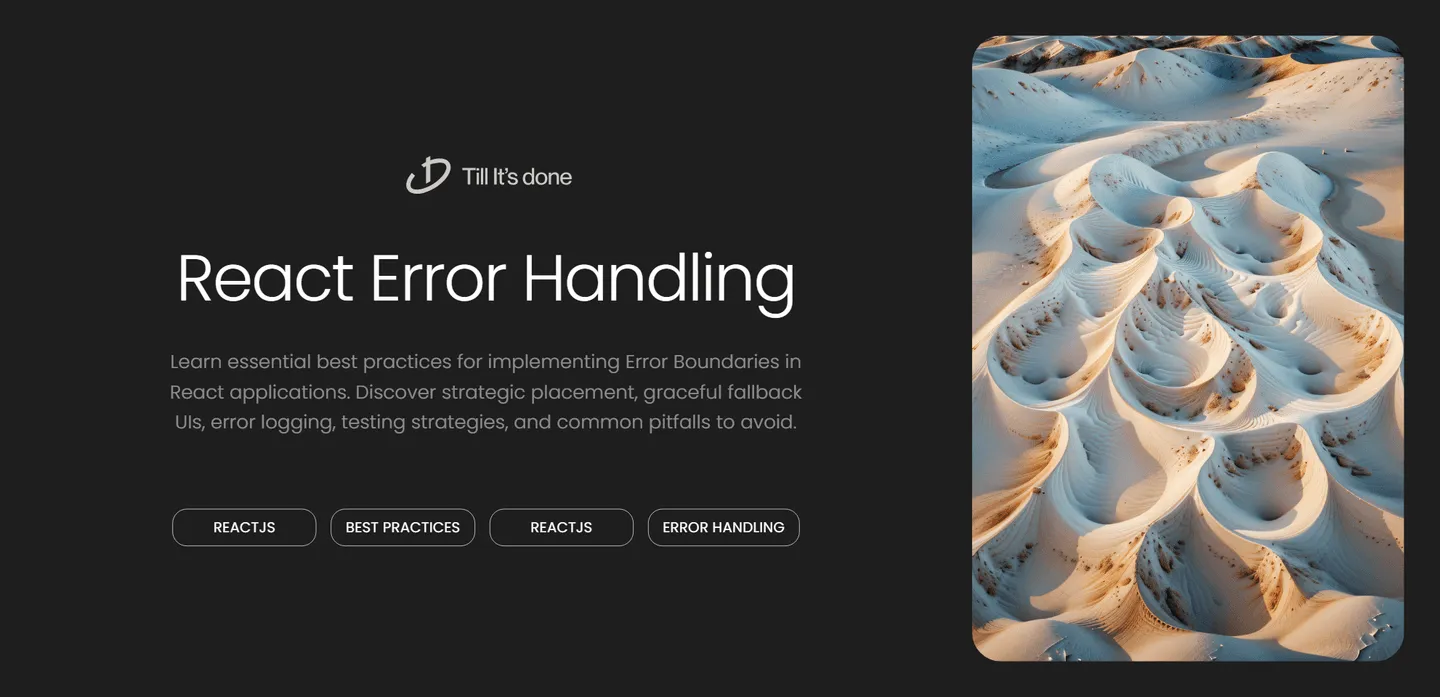
Best Practices for Error Boundaries in React
Error boundaries in React serve as the last line of defense against runtime errors that could potentially crash your entire application. Let’s dive into the best practices that will help you implement robust error handling in your React applications.
Understanding Error Boundaries
Error boundaries are React components that catch JavaScript errors anywhere in their child component tree. They’re like try-catch blocks for React components, preventing the entire app from breaking when unexpected errors occur.
Key Best Practices
1. Strategic Placement of Error Boundaries
Place error boundaries strategically around critical sections of your application. Consider wrapping:
- Route-level components
- Shared components that handle complex data
- Third-party components that might be unstable
- Features that are independent of each other
class ErrorBoundary extends React.Component { state = { hasError: false };
static getDerivedStateFromError(error) { return { hasError: true }; }
componentDidCatch(error, errorInfo) { logErrorToService(error, errorInfo); }
render() { if (this.state.hasError) { return <FallbackComponent />; } return this.props.children; }}
2. Graceful Fallback UI
Create meaningful fallback UIs that:
- Inform users about the error in plain language
- Provide options to recover (refresh, retry, go back)
- Maintain your app’s visual consistency
3. Error Logging and Monitoring
Implement comprehensive error logging:
- Capture error details and component stack traces
- Include relevant user context
- Use error monitoring services for production environments
4. Reset Error Boundaries
Allow error boundaries to reset their state when appropriate:
function ResetableErrorBoundary({ onReset, children }) { return ( <ErrorBoundary onReset={() => { // Reset error boundary state onReset(); }} > {children} </ErrorBoundary> );}
5. Testing Error Scenarios
Remember to:
- Test error boundary behavior using simulated errors
- Verify fallback UI renders correctly
- Ensure error recovery mechanisms work as expected
Common Pitfalls to Avoid
- Don’t wrap every component in an error boundary
- Avoid catching errors you can’t handle meaningfully
- Don’t ignore errors in development environment
- Remember error boundaries don’t catch all types of errors
Conclusion
Implementing error boundaries effectively requires careful planning and consideration of your application’s architecture. By following these best practices, you can create a more resilient React application that handles errors gracefully and maintains a positive user experience.
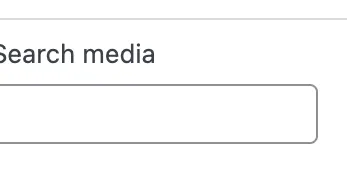





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.