- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Troubleshooting Common Issues with React Context

Troubleshooting Common Issues with React Context: A Developer’s Guide
React Context has revolutionized state management in React applications, but like any powerful tool, it comes with its own set of challenges. As someone who’s spent countless hours debugging Context-related issues, I want to share some common pitfalls and their solutions.
The Context Value Changes Too Often
One of the most frequent issues I encounter is unnecessary re-renders caused by context value changes. This typically happens when we pass a new object as the context value on every render.
Here’s what it might look like:
const MyProvider = ({ children }) => { // 🚫 Bad: Creates new object on every render return ( <MyContext.Provider value={{ user: currentUser, updateUser: handleUpdateUser }}> {children} </MyContext.Provider> );};
The solution? Memoize your context value:
const MyProvider = ({ children }) => { // ✅ Good: Memoized value only changes when dependencies change const value = useMemo(() => ({ user: currentUser, updateUser: handleUpdateUser }), [currentUser]);
return ( <MyContext.Provider value={value}> {children} </MyContext.Provider> );};
Context Consumer Not Updating
Sometimes you might find that your components aren’t re-rendering when context values change. This usually happens when you’ve accidentally nested providers incorrectly or when the context value isn’t properly propagating through the component tree.
Make sure your provider wraps the components that need access to the context:
// ✅ Good: Provider wraps all components that need the contextconst App = () => { return ( <ThemeProvider> <UserProvider> <Navigation /> <MainContent /> <Footer /> </UserProvider> </ThemeProvider> );};
Multiple Instances of Context
A subtle but frustrating issue occurs when you accidentally create multiple instances of the same context. This can happen when you define your context in a component file instead of a separate module:
// 🚫 Bad: Context created inside componentconst MyComponent = () => { const MyContext = createContext(); // New instance on every render! // ...};
// ✅ Good: Context created as module-level constantconst MyContext = createContext();const MyComponent = () => { // ...};
Default Value Confusion
The default value of createContext() only applies when a component doesn’t have a matching Provider above it in the tree. This can lead to confusion when testing or when components are used in isolation:
const UserContext = createContext(null); // Be explicit about the shape
// Better approach:const defaultValue = { user: null, updateUser: () => { console.warn('UserContext: using default value'); }};const UserContext = createContext(defaultValue);
Performance Optimization with Context
Remember that all consumers will re-render when the context value changes. To optimize performance, split your context into smaller, more focused pieces rather than one giant context store. This way, components only re-render when the specific data they need changes.
By keeping these common issues and their solutions in mind, you’ll be better equipped to handle React Context in your applications. The key is to understand how Context works under the hood and follow the best practices consistently.
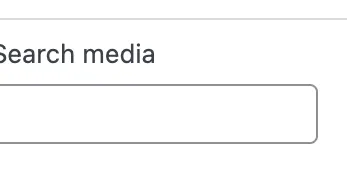





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.