- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Use React Context for Global State Management
Discover best practices, implementation patterns, and when to use Context vs other solutions.
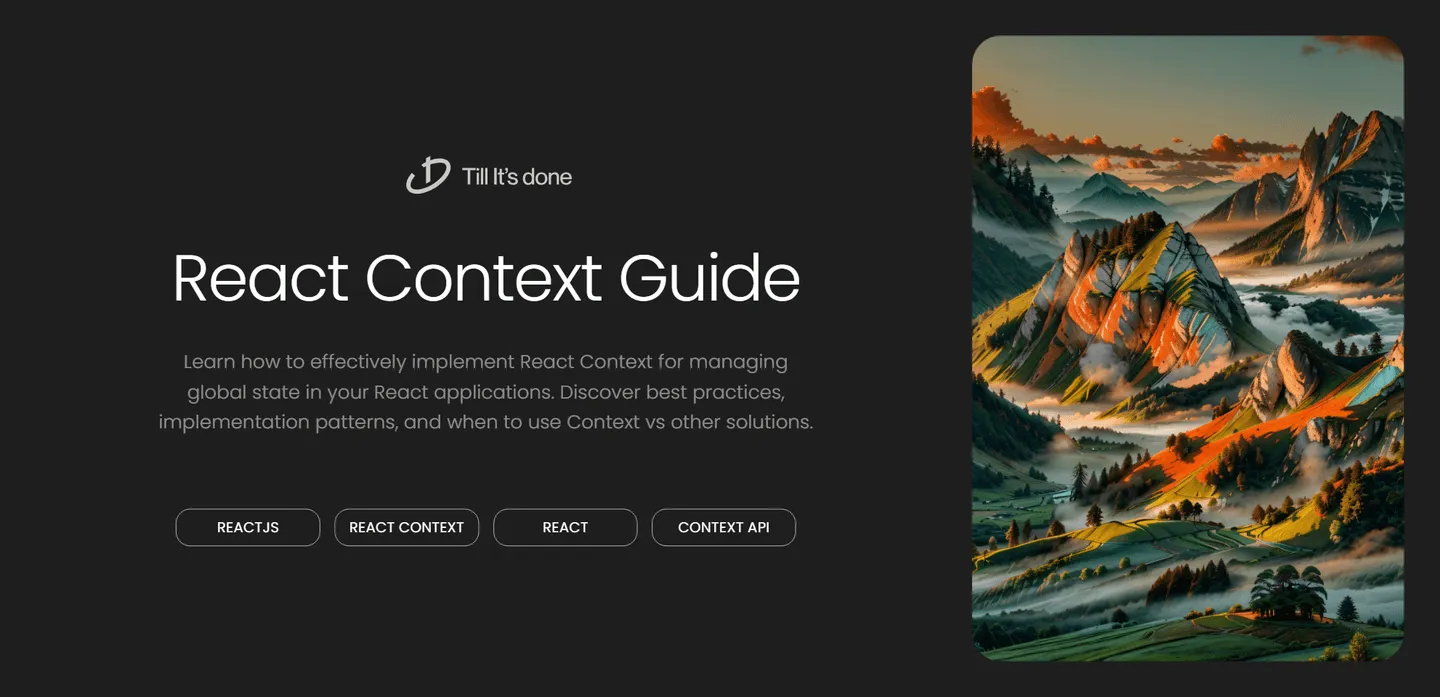
How to Use React Context for Global State Management
Managing state in React applications can become complex as your app grows. While props are great for passing data between components, they can lead to “prop drilling” when you need to pass data through multiple levels of components. This is where React Context comes in – it’s like a magical tunnel that lets you beam data directly to where it’s needed, without passing through every component in between.
Understanding React Context
Think of React Context as a broadcast system in your React app. Instead of manually passing props down through each component, Context lets you create a central data store that any component can tap into. It’s perfect for global data like user preferences, themes, or authentication status.
Setting Up Context
Creating and using Context involves three main steps:
- Create a Context using
React.createContext()
- Provide the Context value using a Provider component
- Consume the Context in child components
Here’s a practical example of implementing a theme switcher:
import { createContext, useState } from 'react';
export const ThemeContext = createContext();
export function ThemeProvider({ children }) { const [isDark, setIsDark] = useState(false);
return ( <ThemeContext.Provider value={{ isDark, setIsDark }}> {children} </ThemeContext.Provider> );}
Consuming Context
The beauty of Context is how easily components can consume it. Using the useContext
hook, any component can access the Context values:
import { useContext } from 'react';import { ThemeContext } from './ThemeContext';
function ThemedButton() { const { isDark, setIsDark } = useContext(ThemeContext);
return ( <button onClick={() => setIsDark(!isDark)}> Switch to {isDark ? 'Light' : 'Dark'} Theme </button> );}
Best Practices
-
Context Composition: Split your Context into logical domains rather than having one giant Context for everything. This makes your code more maintainable and prevents unnecessary re-renders.
-
Performance Considerations: Remember that all components consuming a Context will re-render when the Context value changes. Structure your Context values to minimize unnecessary updates.
-
Default Values: Always provide meaningful default values when creating Context. This makes your Context more reusable and helps with testing.
When to Use Context
Context is perfect for:
- Theme settings
- User authentication state
- Language preferences
- Shopping cart state
- Any global app state that many components need to access
However, it’s not always the best solution. For simple parent-child communication, props are still the way to go. And for complex state management with frequent updates, you might want to consider Redux or other state management libraries.
With React Context, you can write cleaner, more maintainable code by avoiding prop drilling and maintaining a single source of truth for your global state. Start small, experiment with simple use cases, and gradually expand your usage as you become more comfortable with the Context API.
Remember: Context is not a replacement for all state management – it’s another tool in your React toolbox. Use it wisely, and your code will thank you for it!
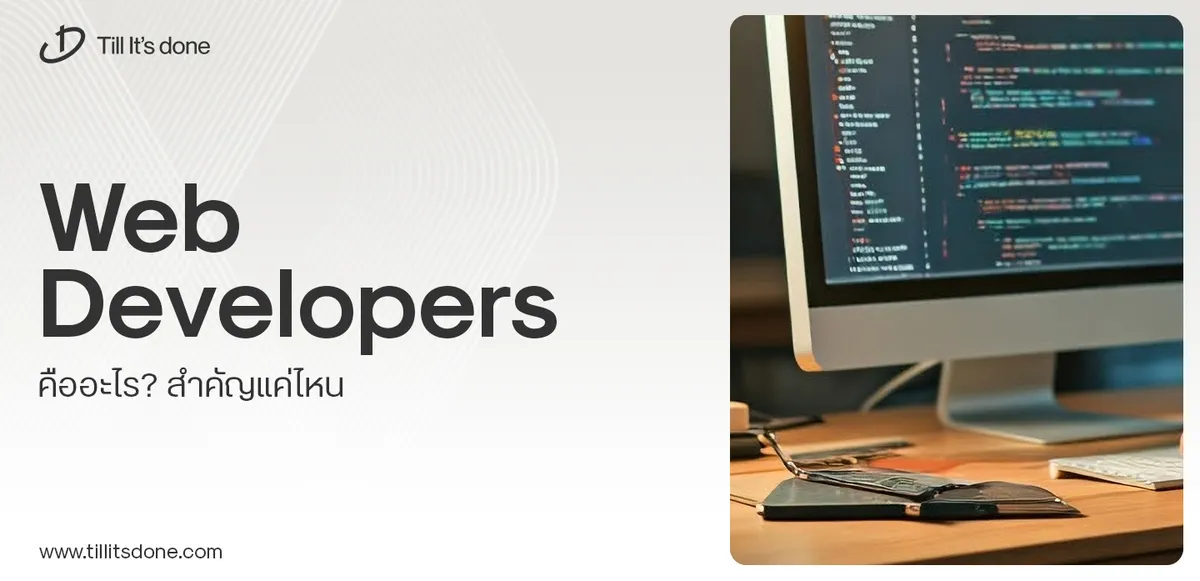
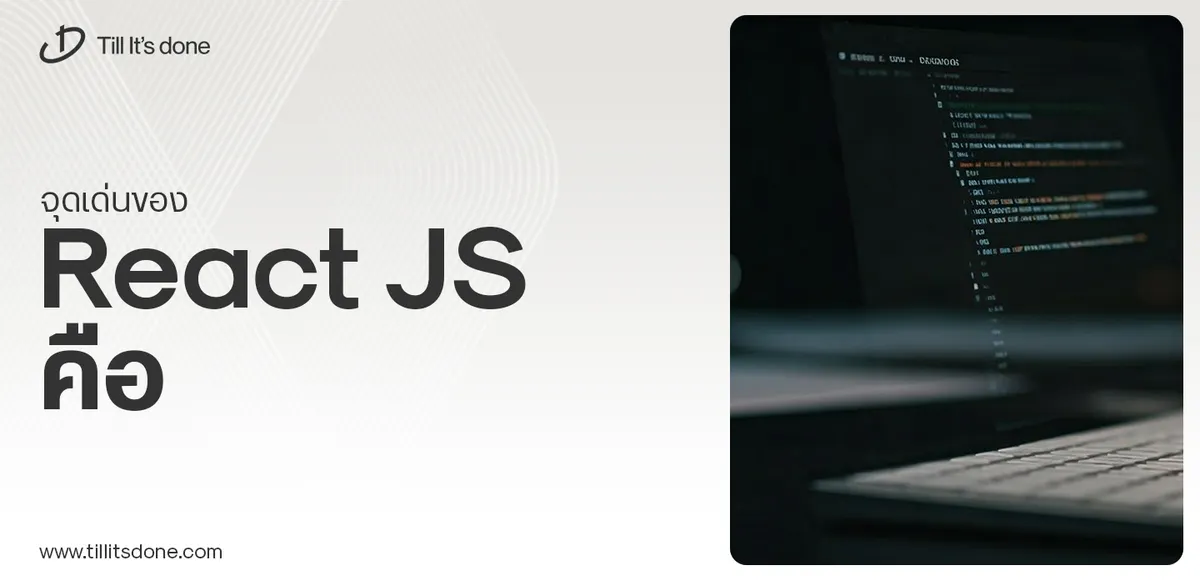
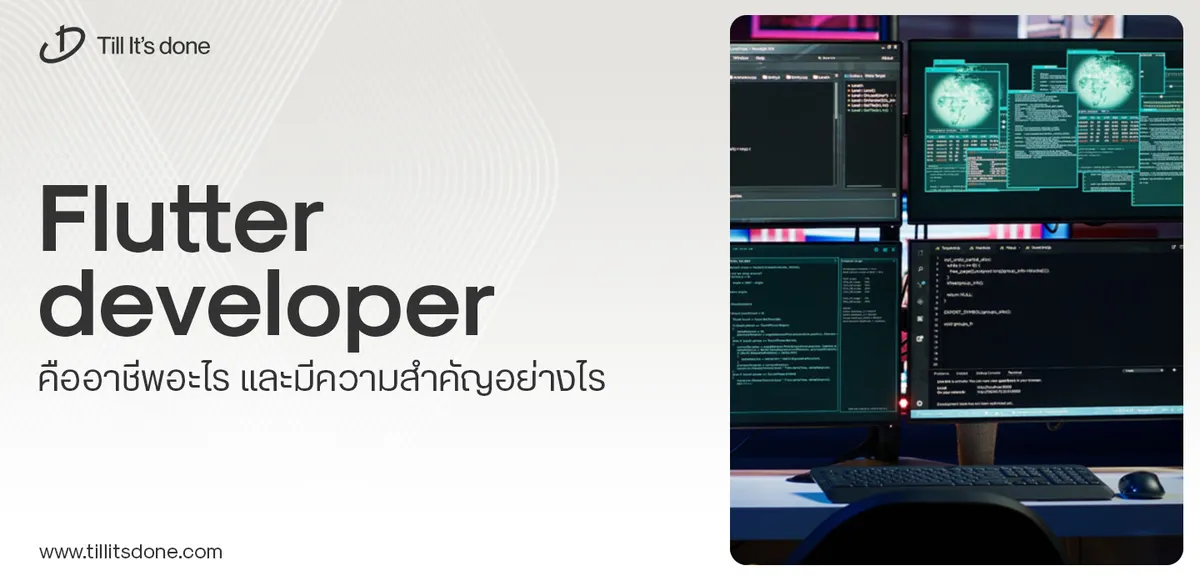
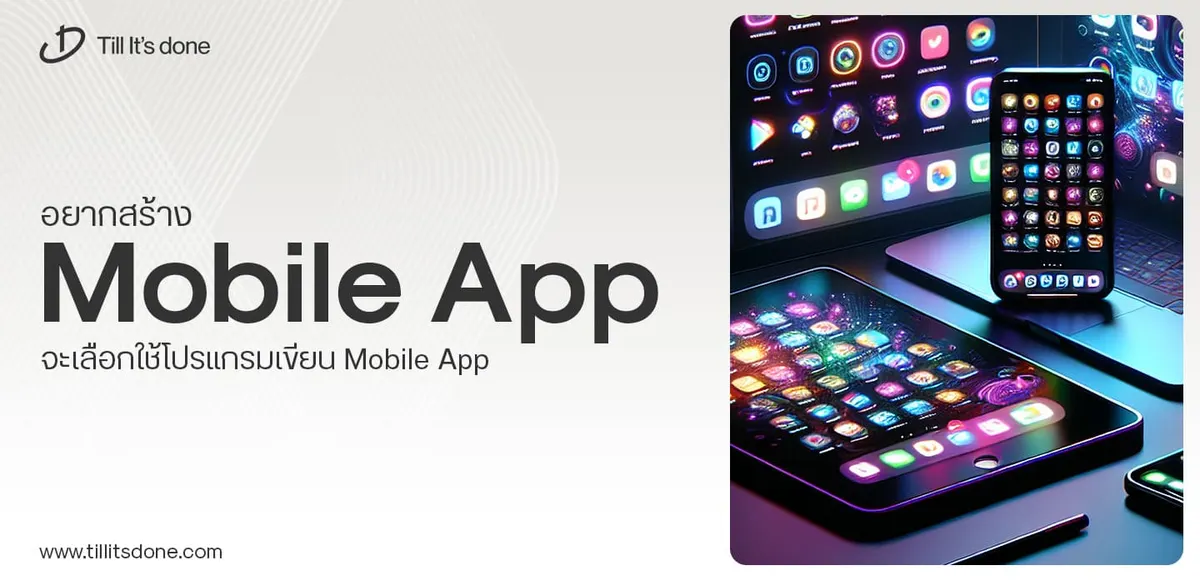
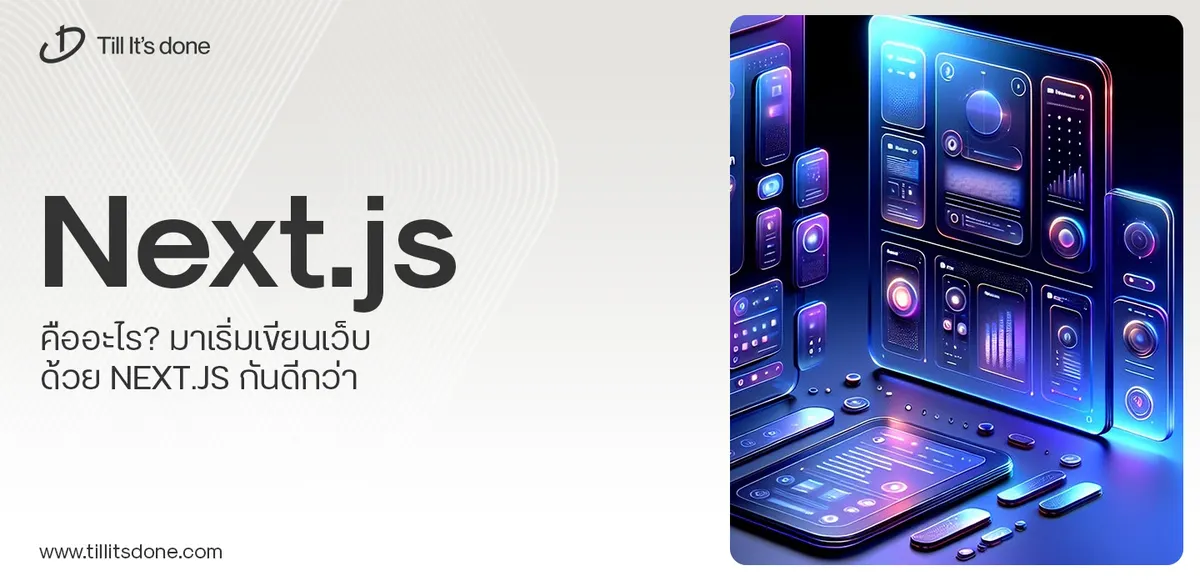
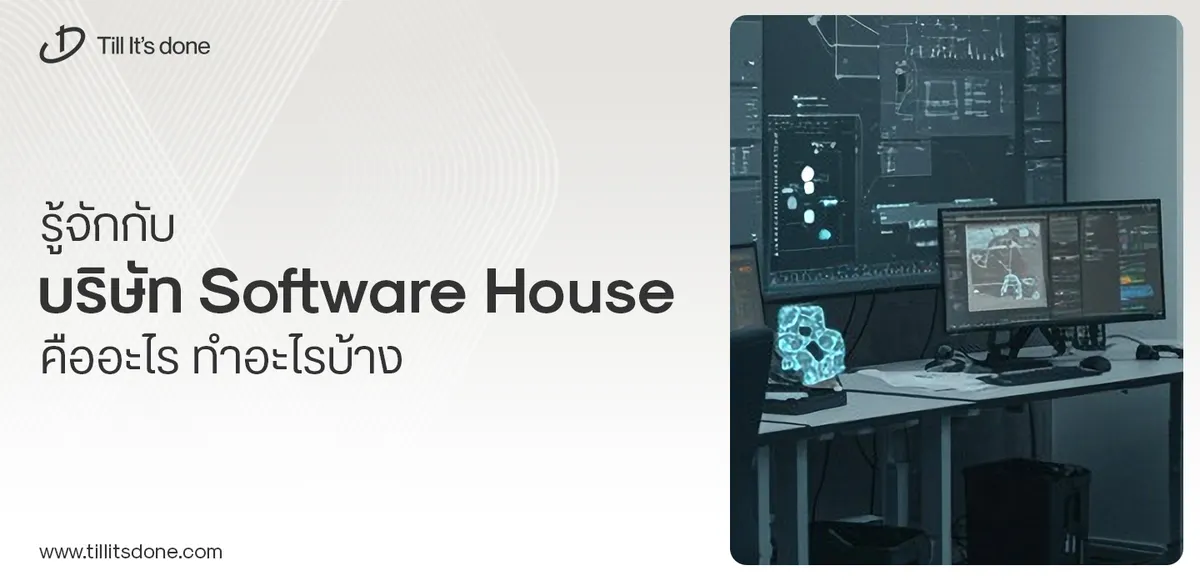
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.