- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Scaling Your React App with Context API
Learn best practices, performance optimization, and advanced patterns to scale your React applications effectively without prop drilling.
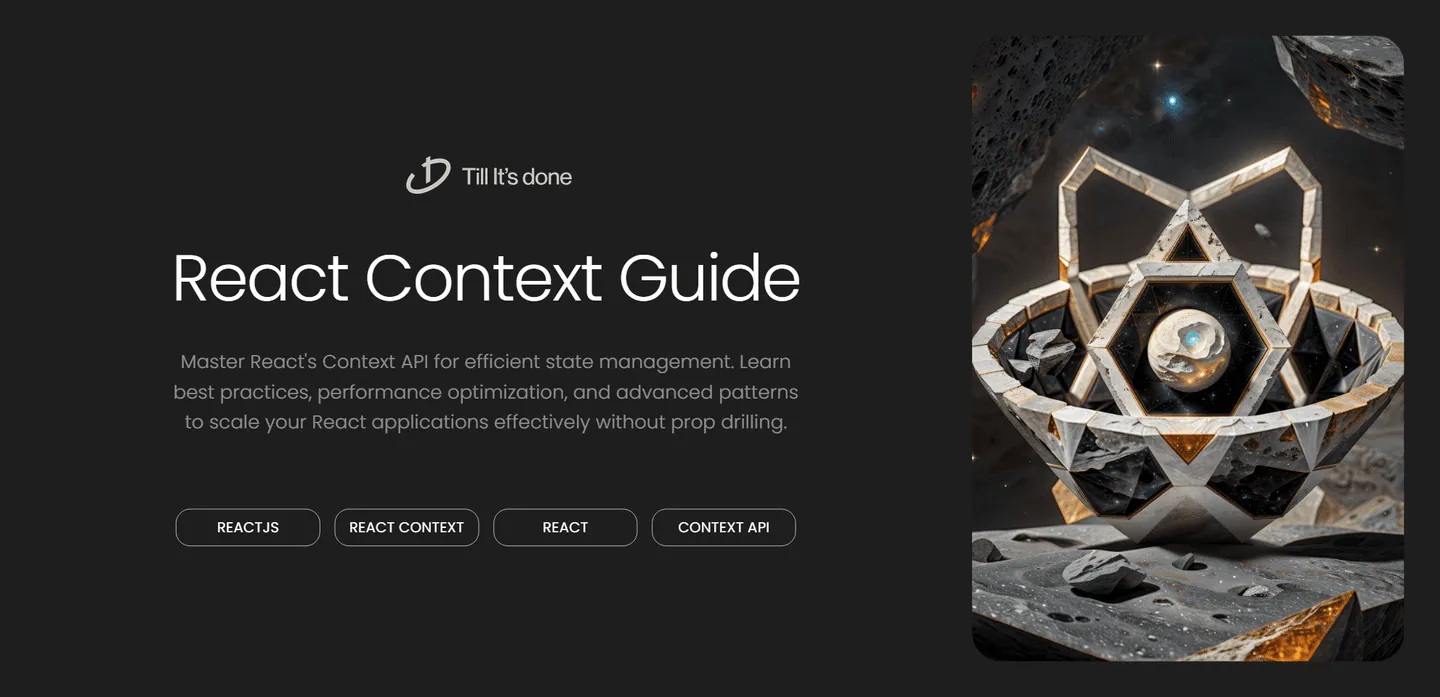
Scaling Your React App with Context API: A Modern Approach to State Management
As React applications grow larger and more complex, managing state effectively becomes increasingly crucial. While props drilling served us well in smaller applications, it can quickly become unwieldy as your component tree expands. Enter React Context API – a powerful built-in solution that elegantly solves this challenge.
Why Context API?
Think of Context API as a broadcast system for your React components. Instead of passing props through multiple layers of components (props drilling), Context API creates a direct channel between a provider (the broadcaster) and any consuming components (the receivers), regardless of their position in the component tree.
Setting Up Context API
The setup process is remarkably straightforward. Let’s break it down into digestible steps:
- Create a Context
- Set up a Provider
- Consume the Context
Here’s the interesting part – Context API isn’t just for simple state management. It’s a versatile tool that can handle complex state scenarios, including:
- User authentication state
- Theme preferences
- Language settings
- Shopping cart data
- Application configurations
Best Practices for Scaling
When scaling your application with Context API, consider these proven strategies:
1. Separate Contexts for Different Concerns
Instead of cramming everything into one massive context, create smaller, focused contexts. This approach improves maintainability and reduces unnecessary re-renders.
2. Optimize Performance
Context API is powerful, but it needs to be used thoughtfully. Every context update triggers a re-render of all consuming components. To optimize performance:
- Keep context values as small as possible
- Use multiple contexts instead of one large context
- Implement memoization where appropriate
3. Strategic Context Placement
Place your context providers as close as possible to the consuming components. This reduces the scope of context updates and improves application performance.
Advanced Patterns
As your application scales, you might want to explore some advanced patterns:
- Combining Context with useReducer for complex state logic
- Creating custom hooks that wrap context consumption
- Implementing context composition for modular state management
When to Use Context API vs Other Solutions
While Context API is powerful, it’s not always the best choice. Use Context API when:
- You need to share state across many components
- The state updates are infrequent
- You want to avoid prop drilling
- Your state management needs are relatively simple
Consider alternatives like Redux or MobX when:
- You need sophisticated state management features
- Performance is a critical concern with frequent updates
- You require robust debugging tools
- Your state logic is highly complex
Conclusion
Context API represents a powerful tool in the React ecosystem for scaling applications effectively. When used correctly, it simplifies state management while maintaining clean, maintainable code. Remember, the key to success lies in understanding both its strengths and limitations, then applying it strategically within your application architecture.
By following these best practices and patterns, you’ll be well-equipped to scale your React applications efficiently using Context API, creating more maintainable and performant applications that can grow alongside your business needs.
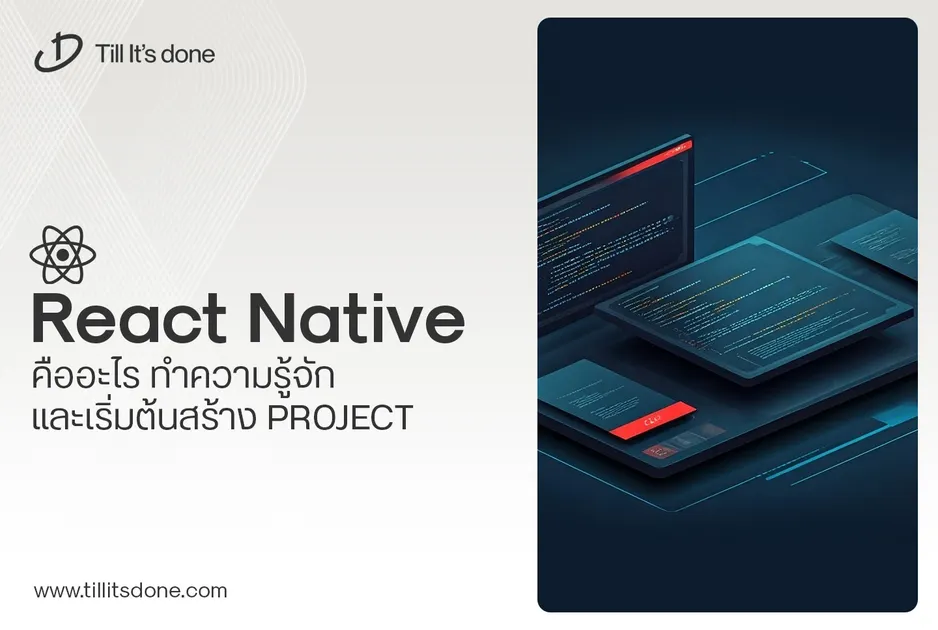
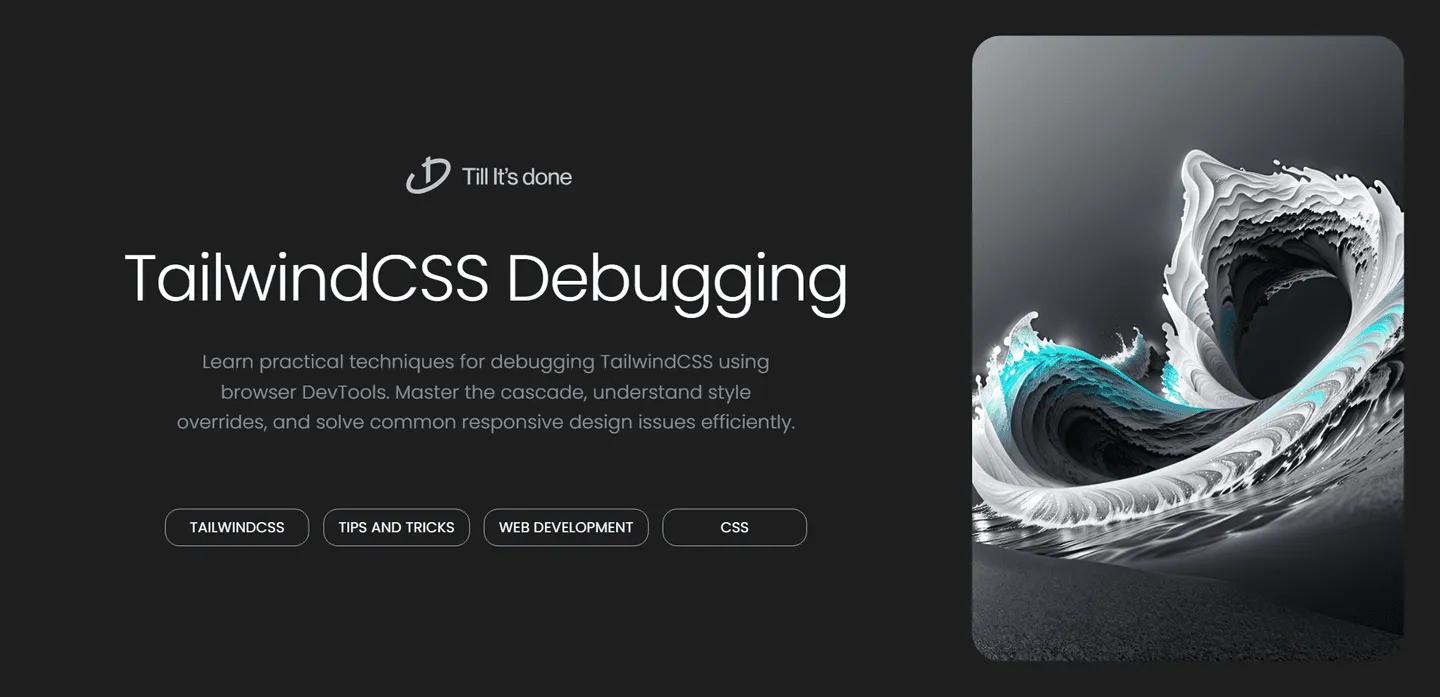
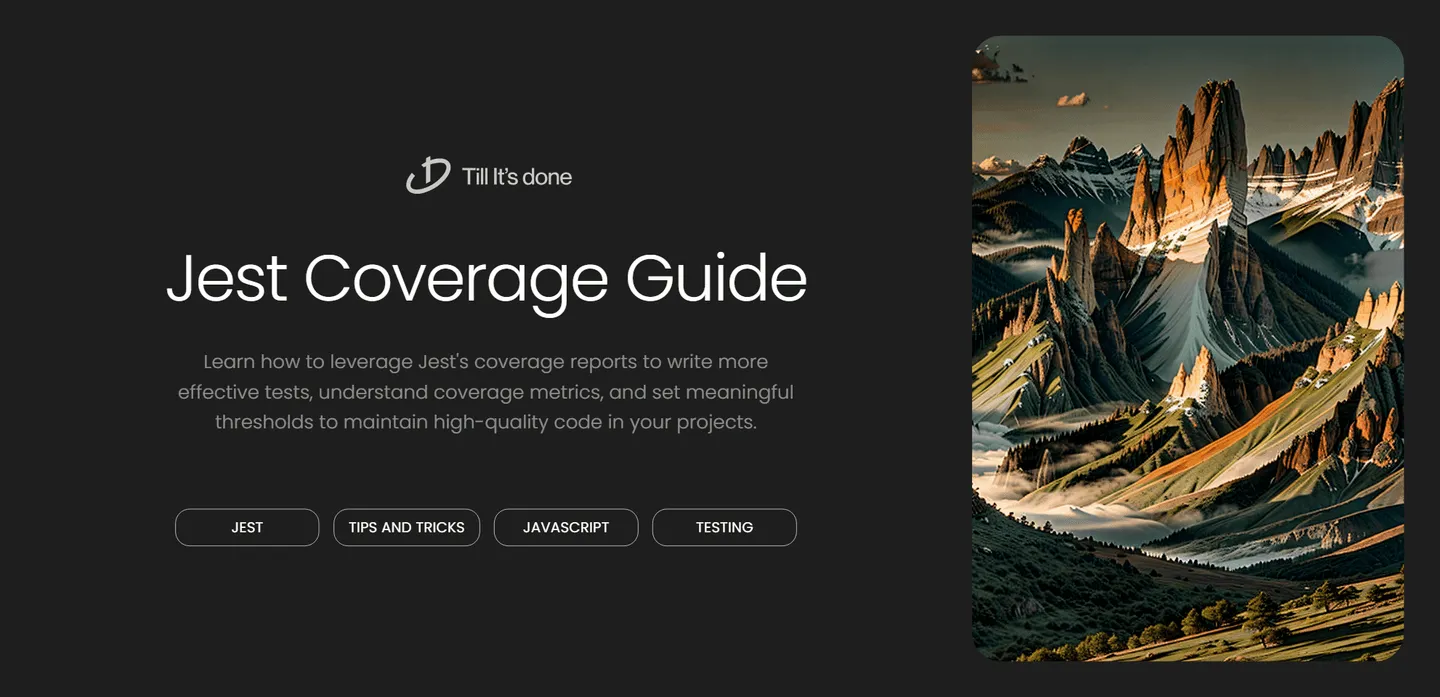
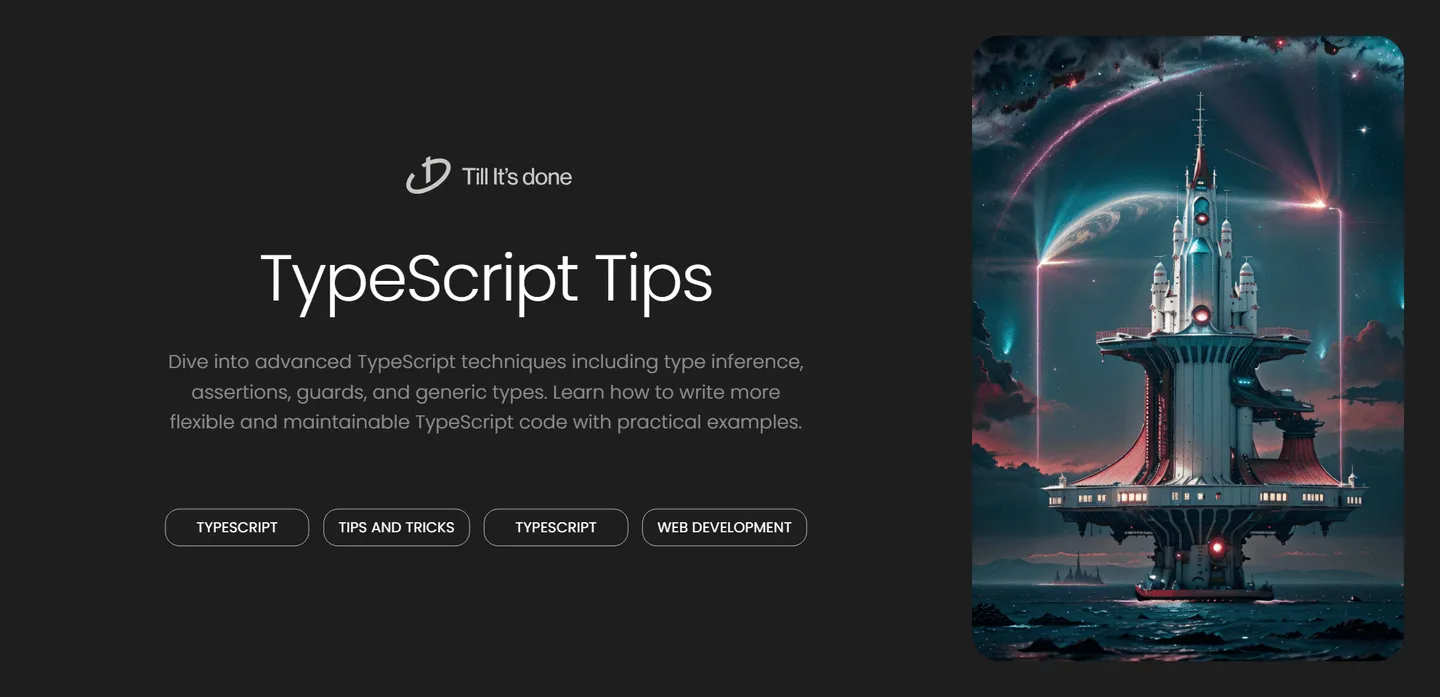
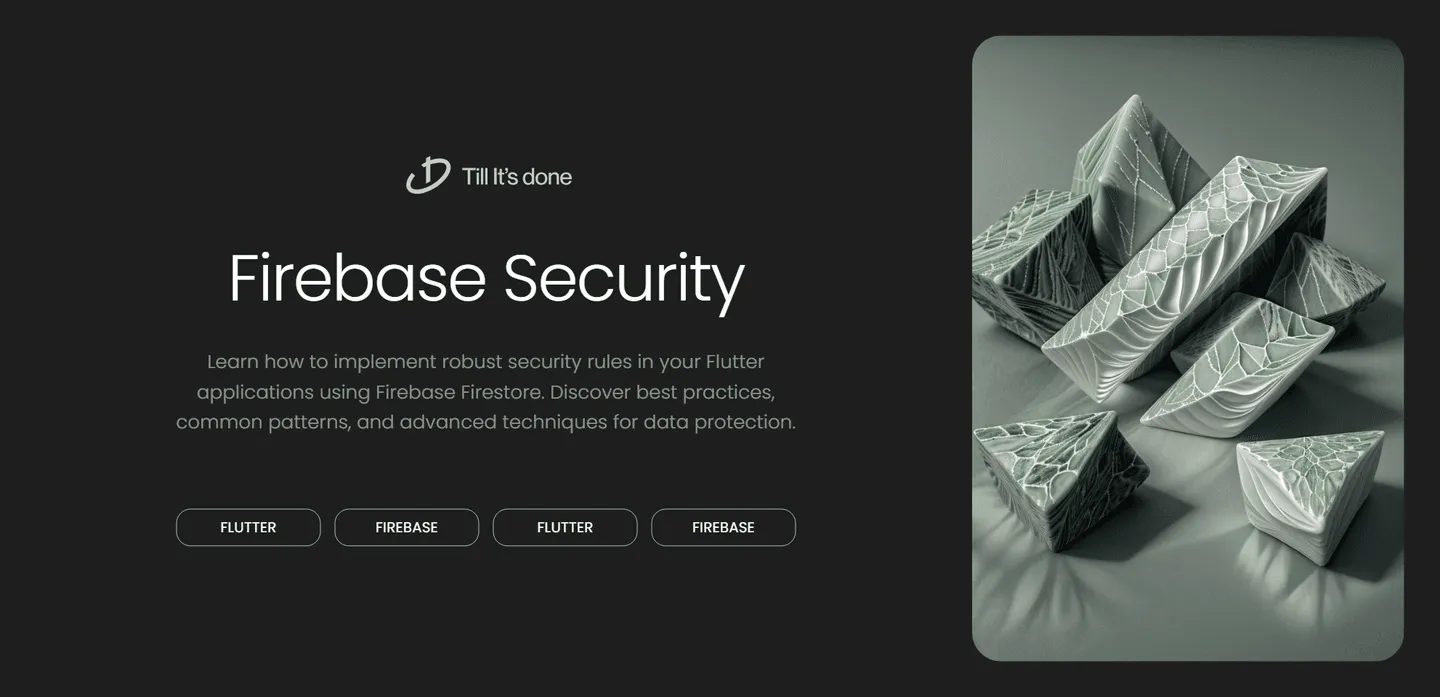
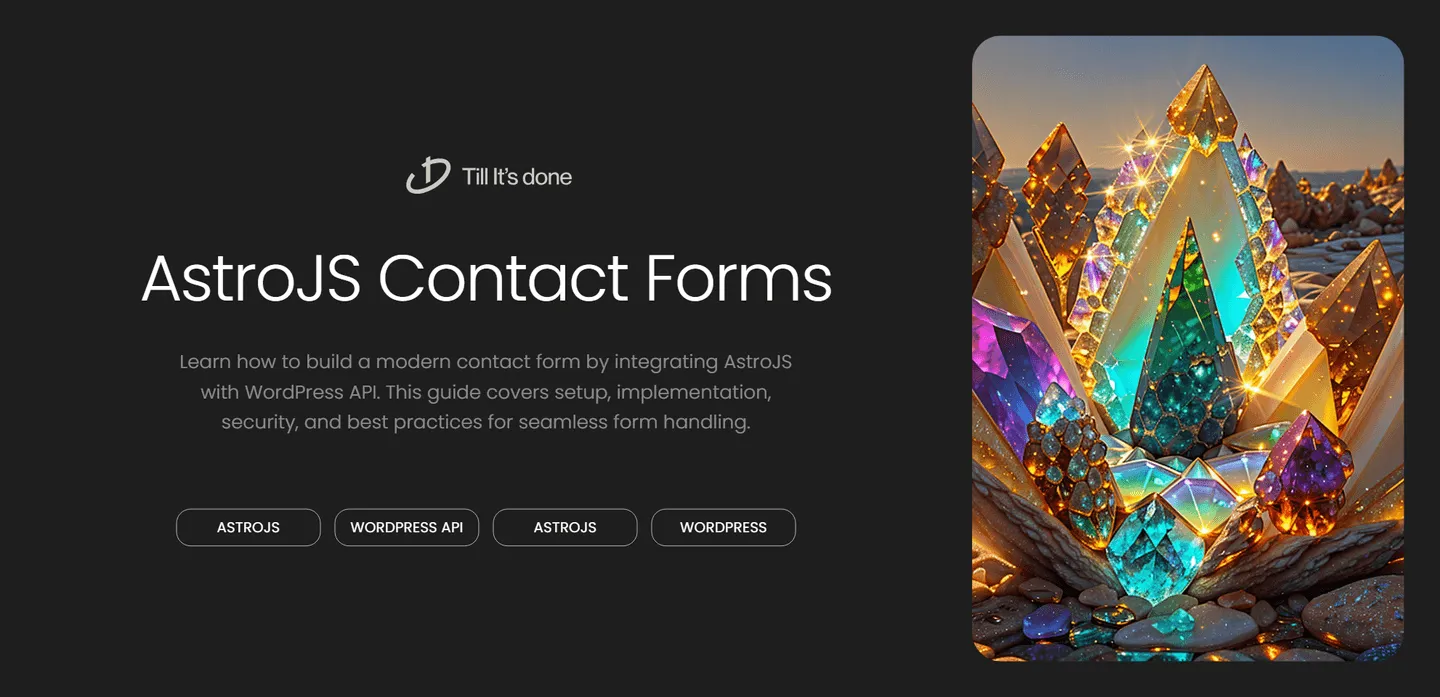
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.