- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Optimizing Performance with React Context
Discover how to build efficient, scalable React applications with proper state management.

Optimizing Performance with React Context: A Deep Dive into Efficient State Management
React Context has revolutionized how we manage state in modern React applications. While it’s a powerful tool for avoiding prop drilling, using it without proper optimization can lead to unnecessary re-renders and performance bottlenecks. Let’s explore practical strategies to make your Context implementation both efficient and maintainable.
Understanding Context Re-renders
Every time the value of a Context Provider changes, all components consuming that context will re-render. This behavior, while necessary for keeping your UI in sync, can become problematic in larger applications. Think of it like a chain reaction – one small change can trigger updates throughout your component tree.
Strategies for Performance Optimization
1. Context Value Memoization
One of the most effective ways to prevent unnecessary re-renders is by memoizing your context value. Instead of passing an object directly to your Provider, wrap it with useMemo
:
const ContextProvider = ({ children }) => { const [state, setState] = useState(initialState);
const value = useMemo(() => ({ state, setState }), [state]);
return ( <MyContext.Provider value={value}> {children} </MyContext.Provider> );};
2. Context Splitting
Rather than having one large context, split your context into smaller, more focused ones. This creates natural boundaries and ensures components only re-render when relevant data changes.
// Instead of one large contextconst AppContext = React.createContext();
// Split into focused contextsconst UserContext = React.createContext();const ThemeContext = React.createContext();const SettingsContext = React.createContext();
3. Consumer Component Optimization
Optimize your consumer components using React.memo
and careful structuring:
const UserInfo = React.memo(() => { const { user } = useContext(UserContext); return <div>{user.name}</div>;});
4. State Updates
When updating context state, be mindful of object references. Use immutable update patterns to ensure your changes are properly tracked:
const updateUser = useCallback((newData) => { setUserData(prev => ({ ...prev, ...newData }));}, []);
Best Practices for Production
- Use the Context API for truly global state
- Keep context values as small as possible
- Implement state updates with immutable patterns
- Regularly profile your application to identify re-render issues
- Consider using context alongside other state management solutions
Remember, optimization is about finding the right balance. Not every context needs to be heavily optimized – focus your efforts where they’ll have the most impact.
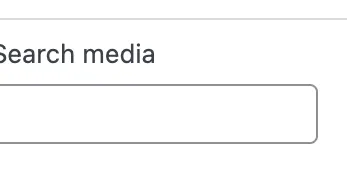





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.