- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Understanding React Context: A Beginner's Guide
Discover practical examples, best practices, and when to use Context in your React applications.
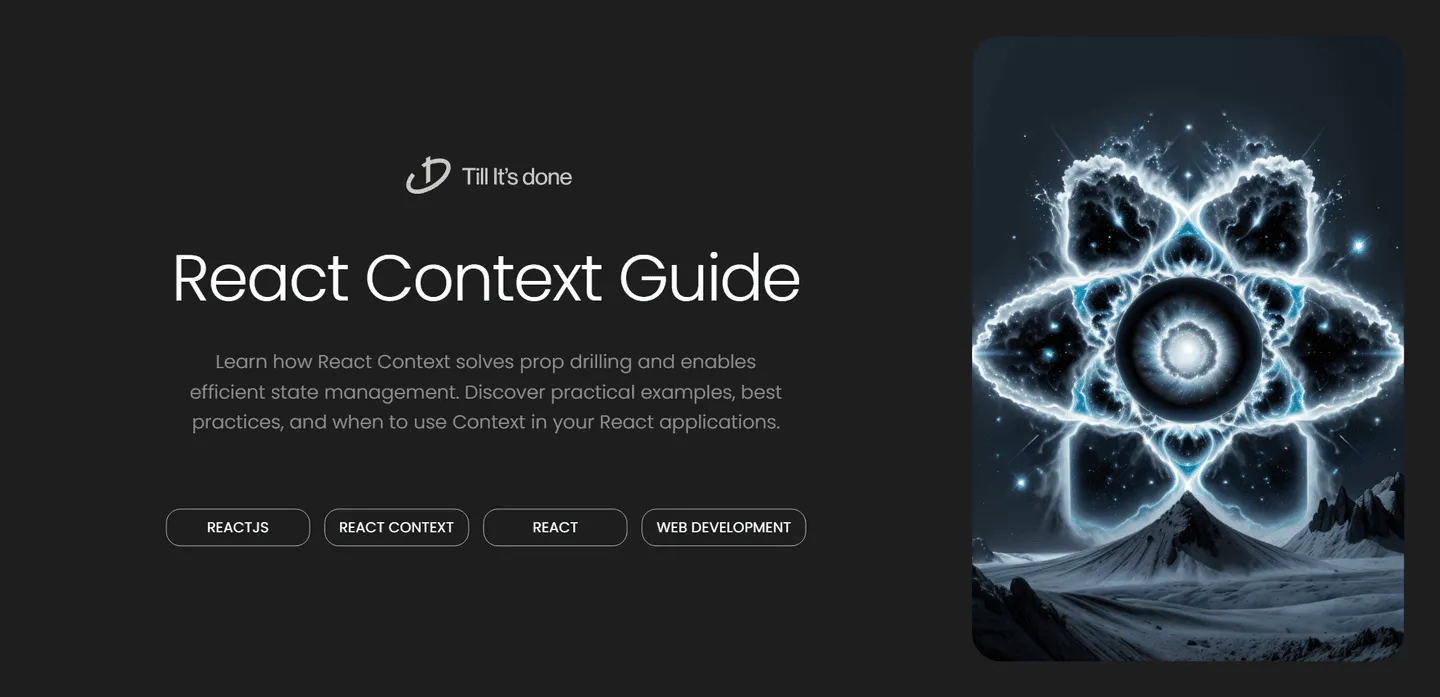
Understanding React Context: A Beginner’s Guide
Have you ever found yourself passing props through multiple components just to get some data to a deeply nested child component? If you’re nodding your head, you’re not alone! This common challenge in React development is often referred to as “prop drilling,” and it’s exactly what React Context was designed to solve.
What is React Context?
Think of React Context as a magical tunnel that can teleport data directly from a parent component to any of its children, no matter how deeply nested they are. Instead of passing props through each intermediate component, Context creates a direct line of communication.
The Problem Context Solves
Imagine you’re building a theme switcher for your application. Without Context, you’d need to pass the theme information through every single component in your component tree, even if those intermediate components don’t need the theme data themselves. It might look something like this:
// Without Contextconst App = () => { const [theme, setTheme] = useState('light'); return ( <Header theme={theme}> <Navigation theme={theme}> <UserProfile theme={theme} /> </Navigation> </Header> );};
This is where Context shines, allowing us to skip all the intermediate components and access our theme directly where it’s needed.
How to Use React Context
Using Context involves three main steps:
- Creating the Context
- Providing the Context
- Consuming the Context
Let’s break these down:
1. Creating the Context
const ThemeContext = React.createContext('light');
2. Providing the Context
const App = () => { const [theme, setTheme] = useState('light'); return ( <ThemeContext.Provider value={theme}> <Header> <Navigation> <UserProfile /> </Navigation> </Header> </ThemeContext.Provider> );};
3. Consuming the Context
You can consume the Context in any child component using the useContext hook:
const UserProfile = () => { const theme = useContext(ThemeContext); return ( <div className={`profile ${theme}`}> {/* Component content */} </div> );};
Best Practices and Tips
-
Don’t Overuse Context: While Context is powerful, it’s not meant to replace all prop drilling. Use it for truly global data like themes, user authentication, or language preferences.
-
Separate Concerns: Create different Contexts for different types of data. Don’t throw everything into a single Context object.
-
Consider Performance: React Context triggers a re-render for all components that consume it when its value changes. Structure your Context providers thoughtfully to minimize unnecessary re-renders.
When to Use Context
Context is perfect for:
- Theme switching
- User authentication state
- Language preferences
- Shopping cart state
- Any global application state that many components need to access
Remember, Context isn’t a replacement for state management libraries like Redux or MobX. It’s a tool for avoiding prop drilling and managing global state in smaller to medium-sized applications.
Wrapping Up
React Context is a powerful feature that can significantly simplify your component tree and make your code more maintainable. By understanding when and how to use it effectively, you can write cleaner, more efficient React applications that are easier to maintain and scale.
Remember, the key is to use Context judiciously. Not every prop-drilling situation calls for Context, but when you do need it, it’s an invaluable tool in your React development toolkit.
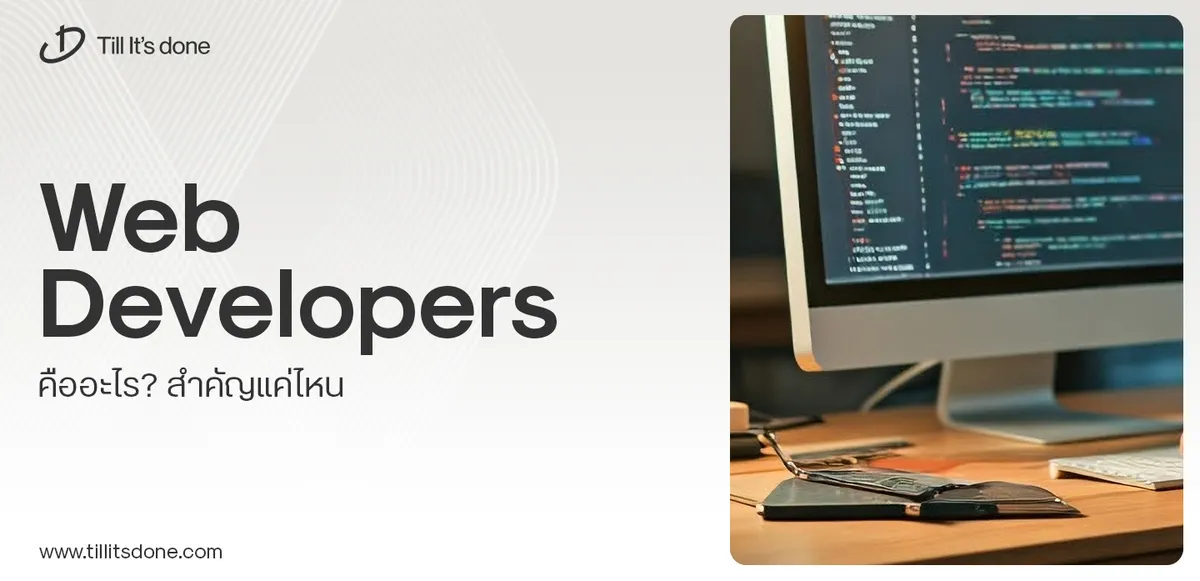
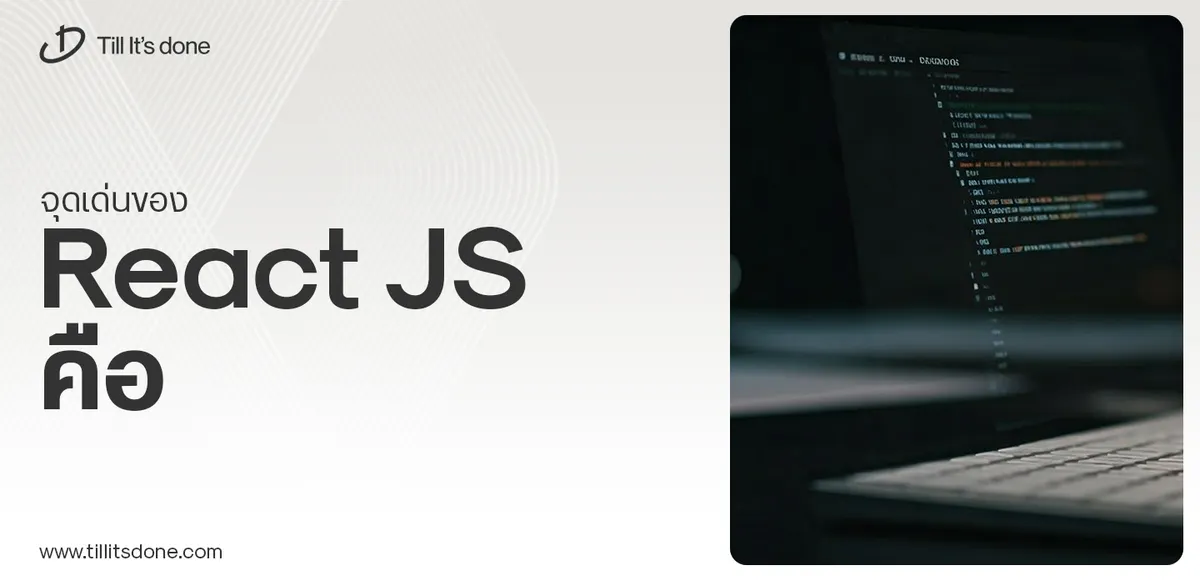
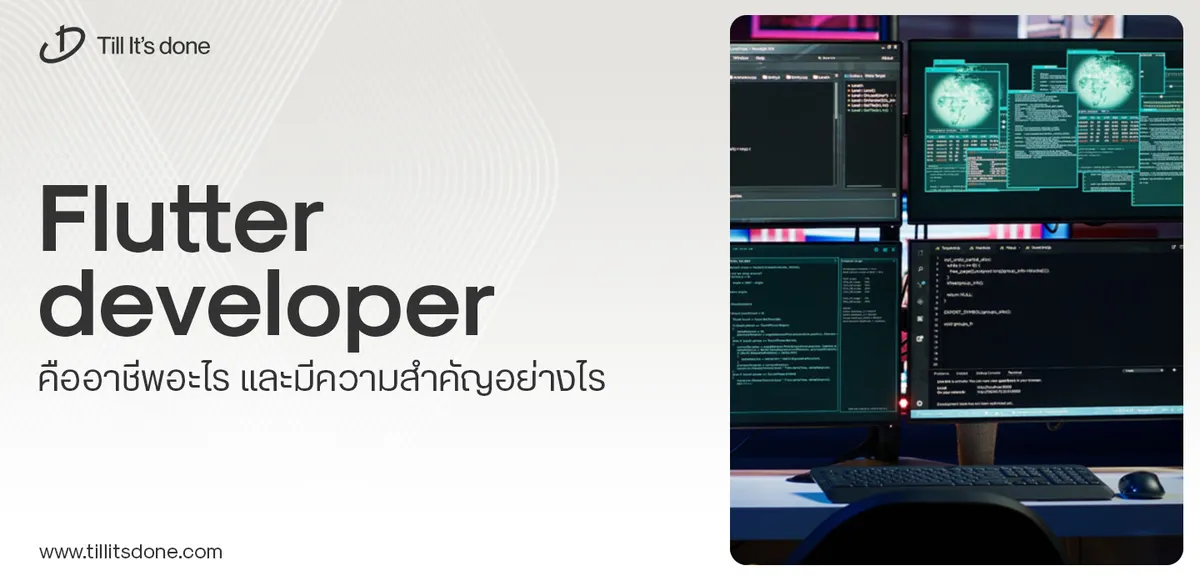
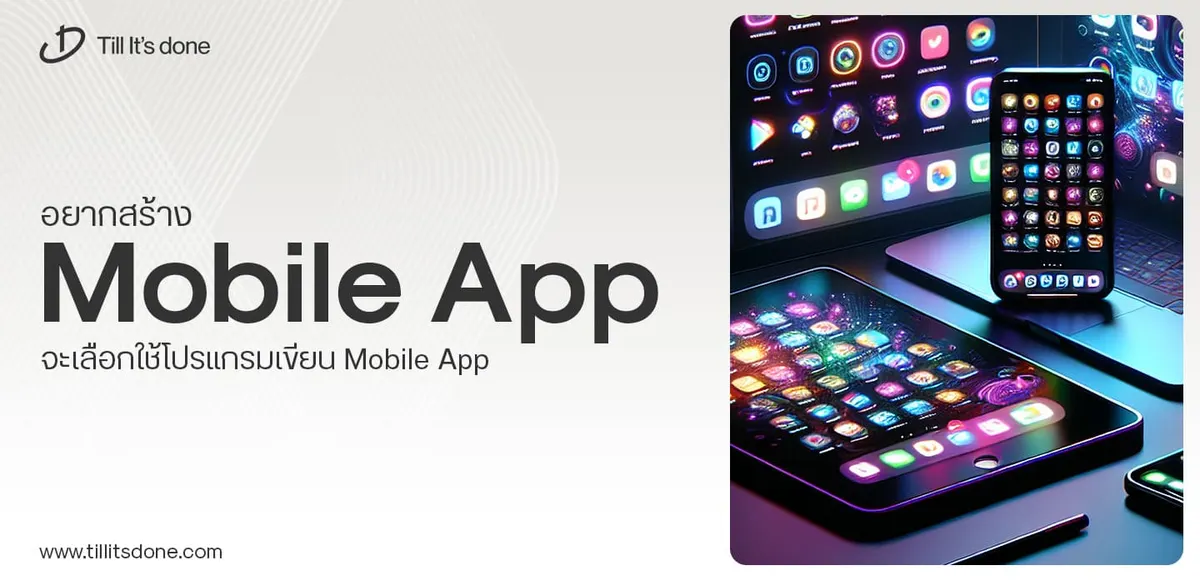
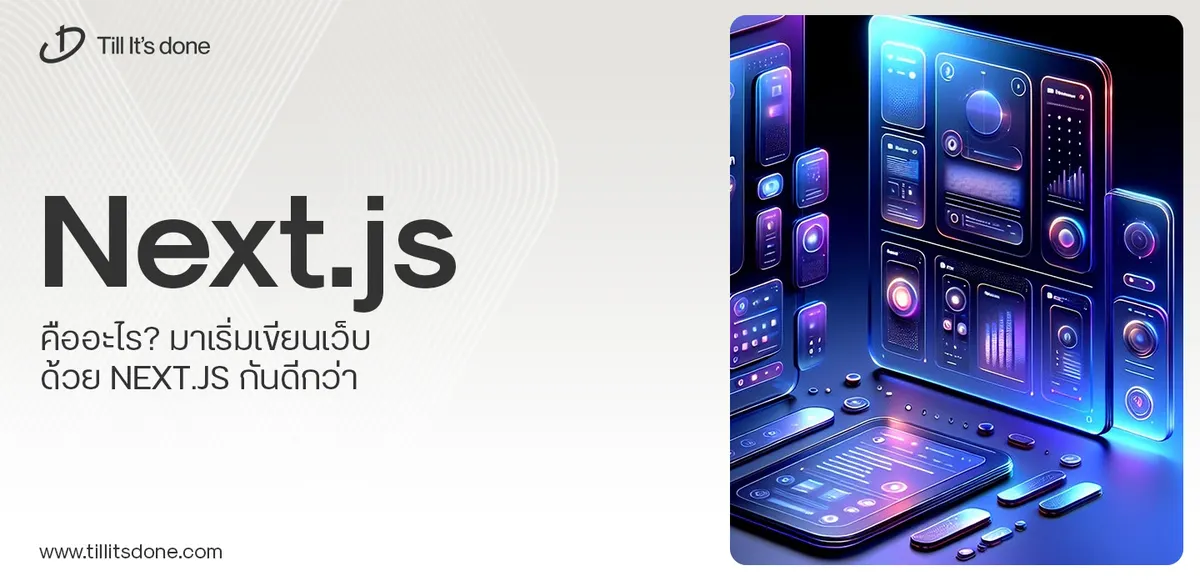
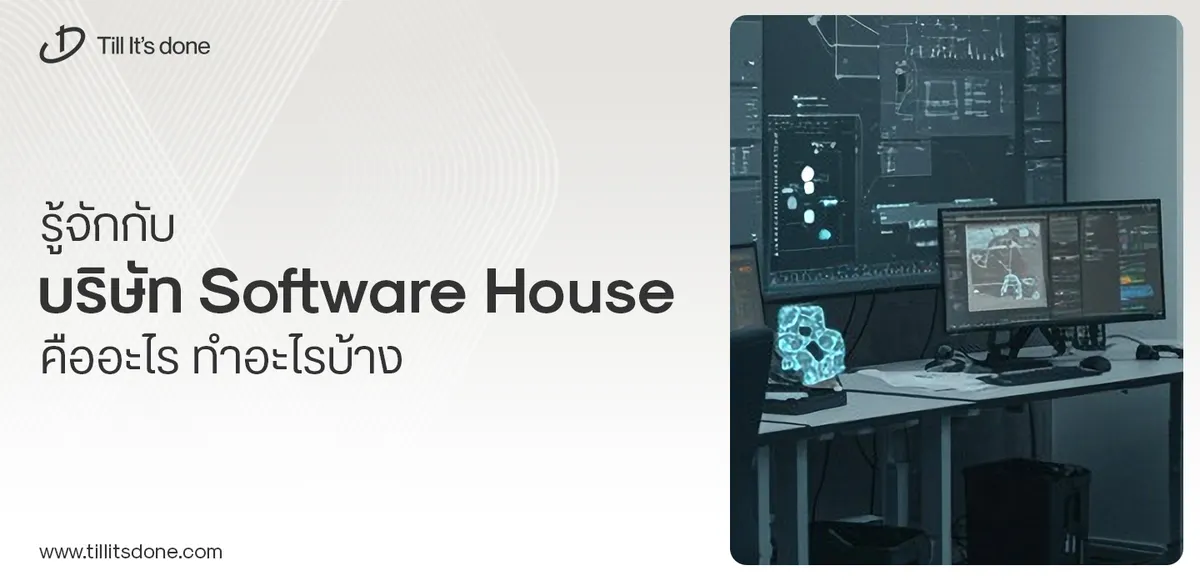
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.