- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Components and Props in ReactJS Guide
Learn how to create reusable UI elements and manage data flow in your React applications effectively.
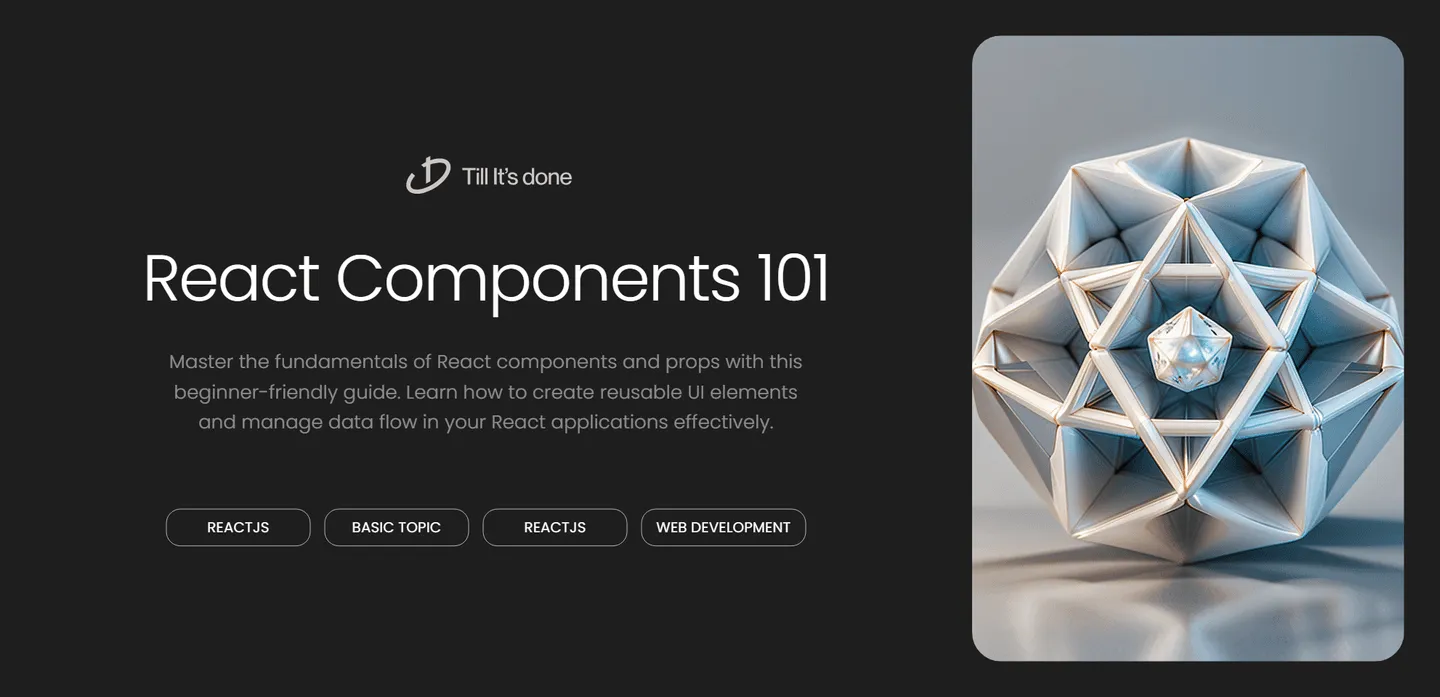
Components and Props in ReactJS: A Beginner’s Guide
Are you starting your journey with ReactJS? One of the first and most fundamental concepts you’ll encounter is Components and Props. Let’s break these down in a way that’s easy to understand, just like explaining it to a friend over coffee.
What are Components?
Think of components as LEGO blocks. Just like how you can use different LEGO pieces to build a castle or a spaceship, you use components to build your web applications. Components are reusable pieces of code that represent a part of your user interface.
Two Types of Components:
- Function Components (Modern Approach)
function Welcome(props) { return <h1>Hello, {props.name}!</h1>;}
- Class Components (Traditional Approach)
class Welcome extends React.Component { render() { return <h1>Hello, {this.props.name}!</h1>; }}
Understanding Props
Props (short for properties) are like passing arguments to a function. They’re how components talk to each other, sharing data from parent to child.
Think of props as a special delivery service. When you order food (parent component), you can specify instructions (props) that get passed to the restaurant (child component).
How Props Work:
- Passing Props
<Welcome name="John" age={25} />
- Receiving Props
function Welcome(props) { return ( <div> <h1>Hello, {props.name}!</h1> <p>You are {props.age} years old.</p> </div> );}
Best Practices
- Keep Components Small: Each component should do one thing well
- Props are Read-Only: Never modify props inside a component
- Use Meaningful Names: Choose descriptive names for both components and props
- Destructure Props: Make your code cleaner by destructuring props
function Profile({ name, age, occupation }) { return ( <div> <h2>{name}</h2> <p>Age: {age}</p> <p>Job: {occupation}</p> </div> );}
Putting It All Together
Components and props work together to create a modular and maintainable application structure. Think of it as building with LEGO blocks where each block (component) can have different specifications (props) that determine its appearance and behavior.
Remember: Props flow down, events flow up. This one-way data flow makes your application predictable and easier to debug.
Conclusion
Components and props are the building blocks of React applications. By understanding these concepts, you’re well on your way to creating amazing web applications. Keep practicing, and soon you’ll be combining components like a master LEGO builder!
Remember: The best way to learn is by doing. Start with simple components, gradually add props, and watch your applications come to life.
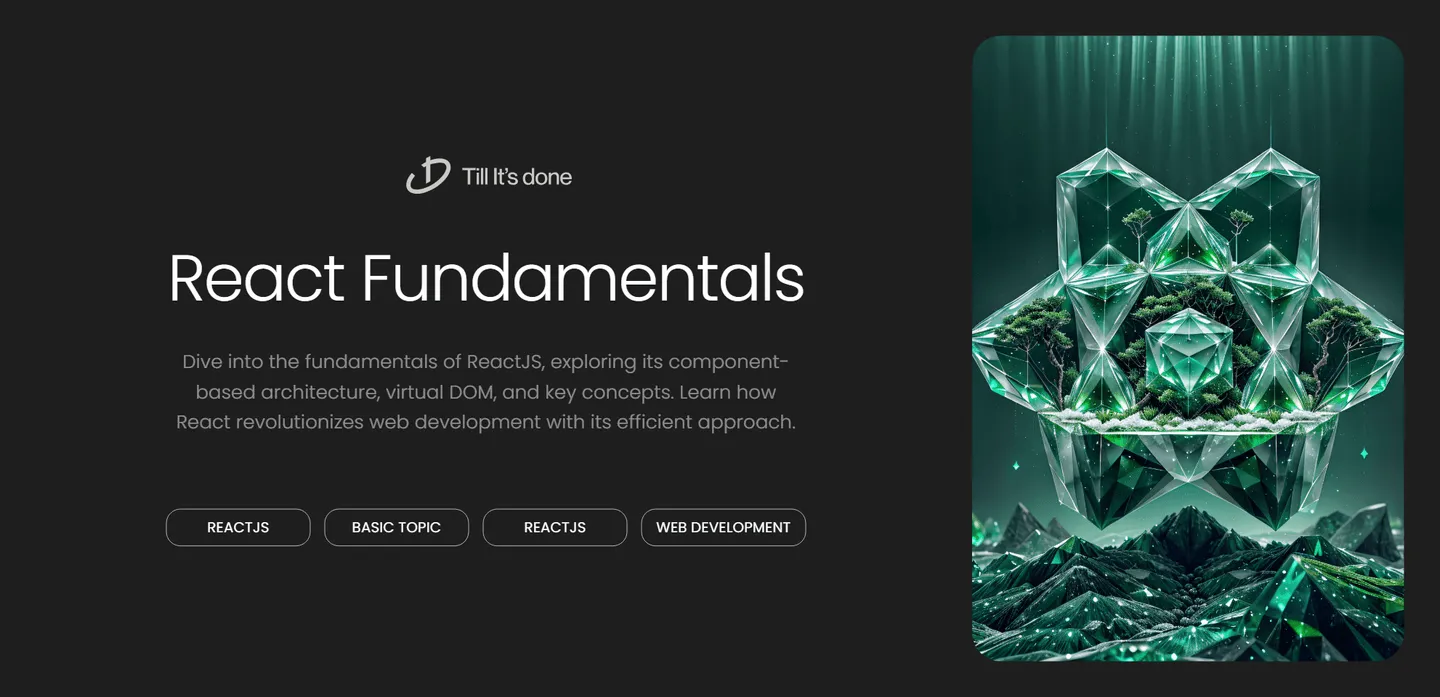
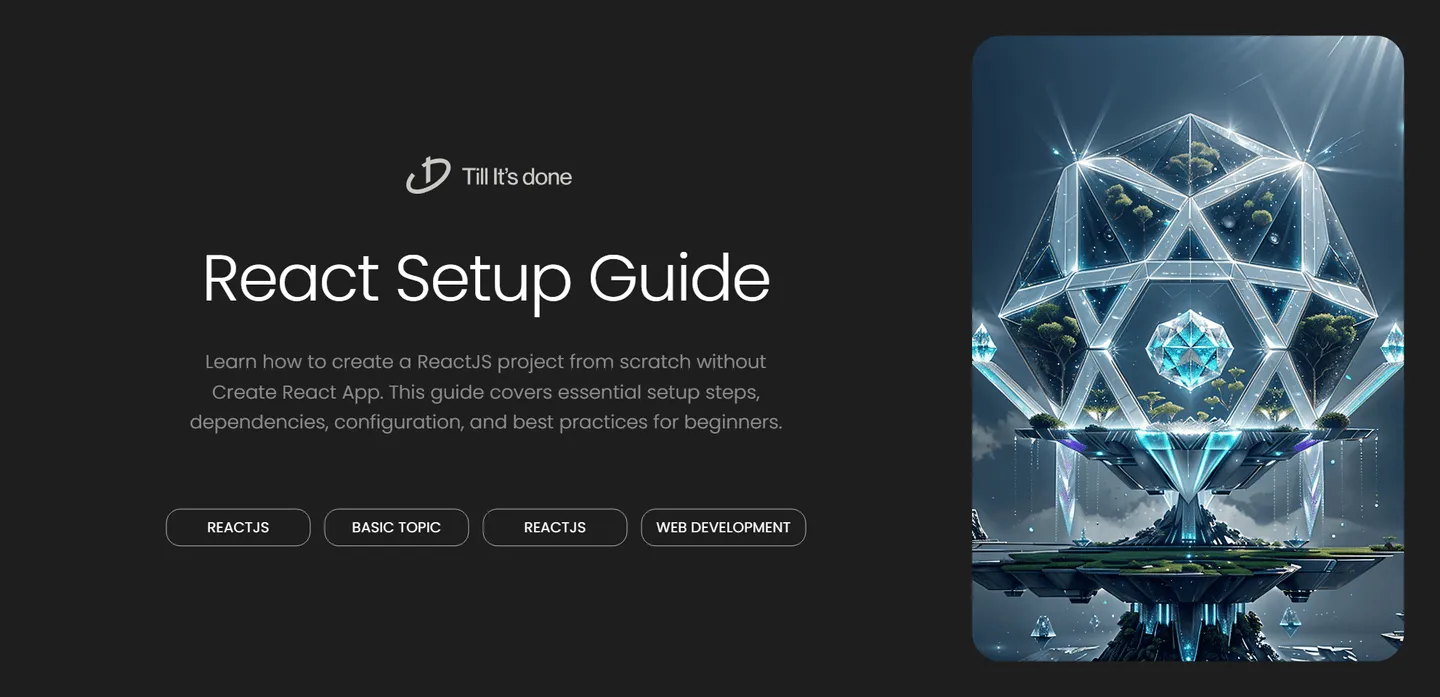
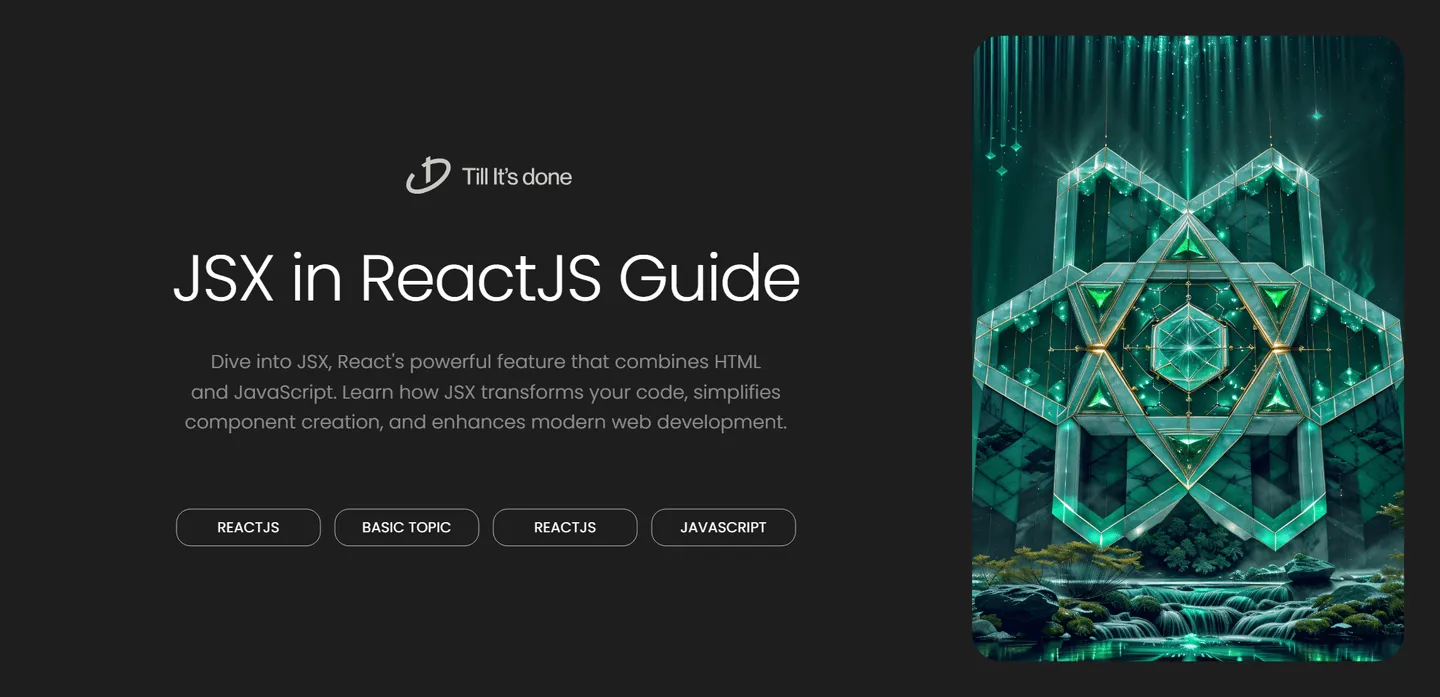
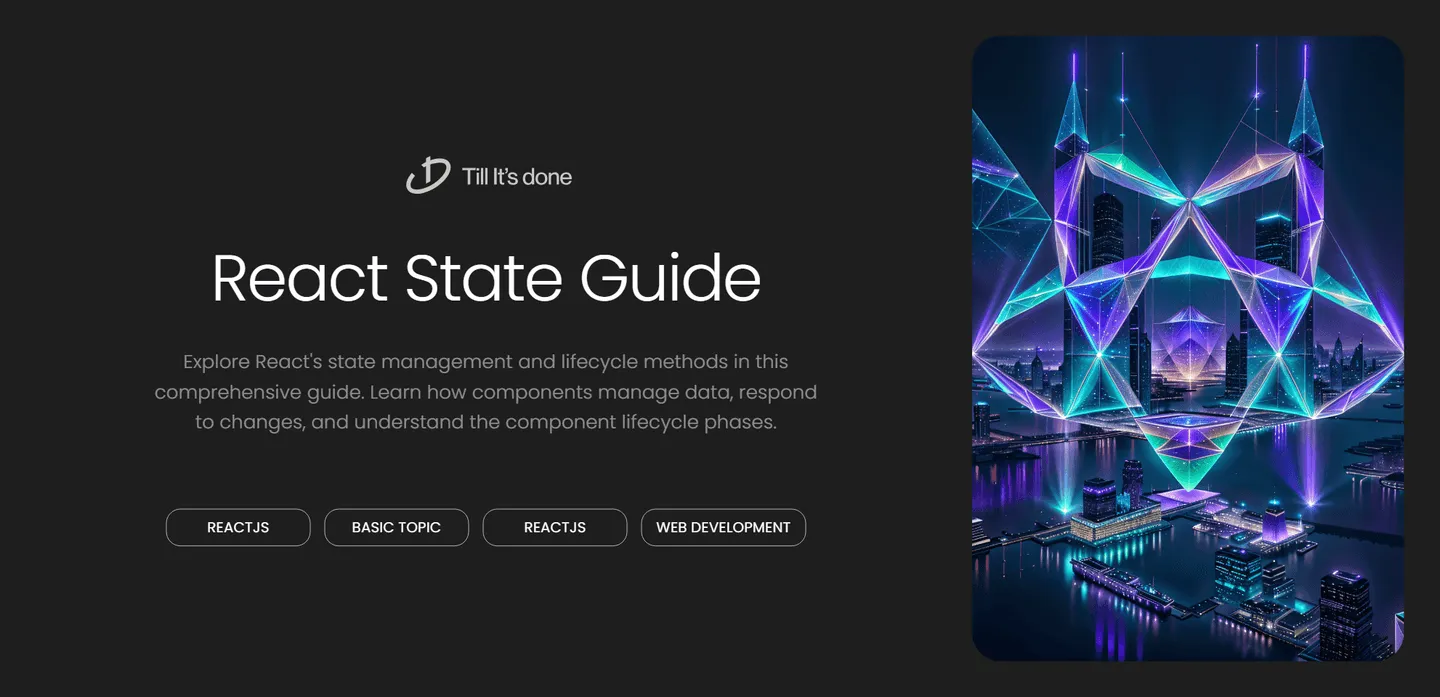
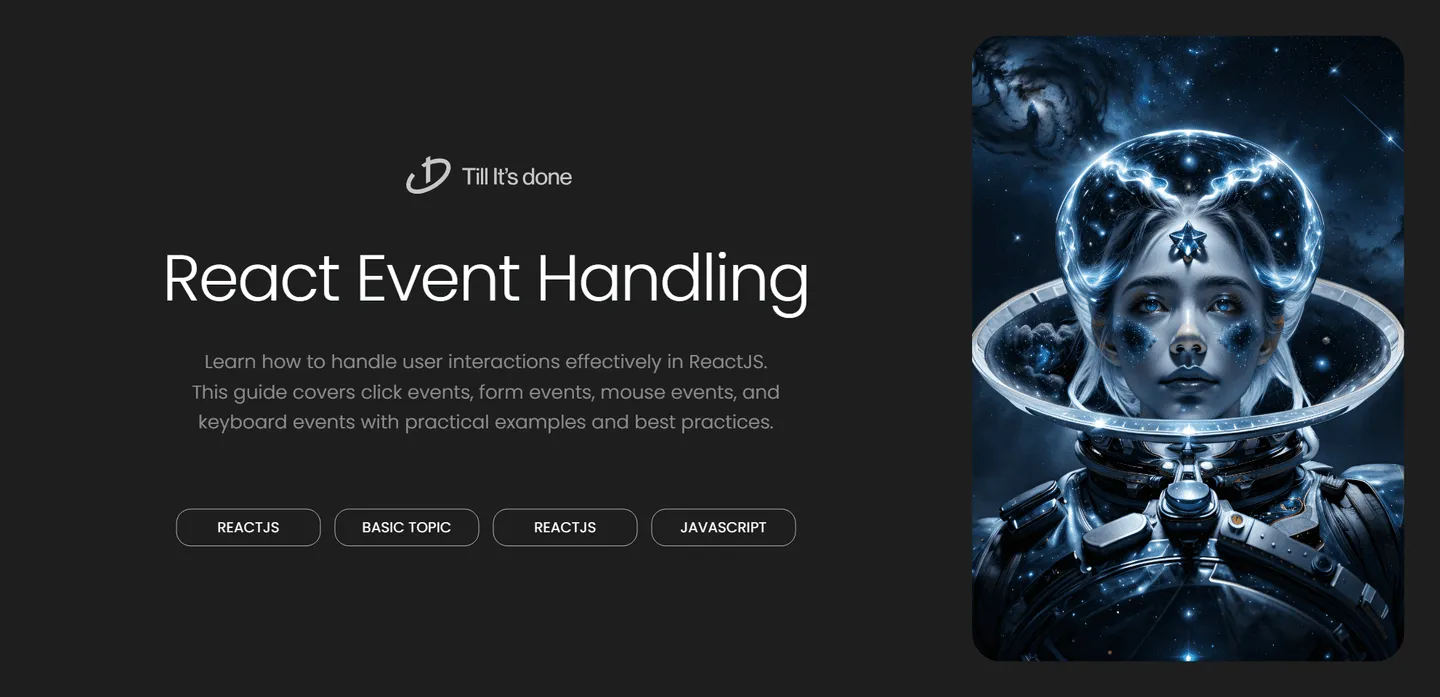
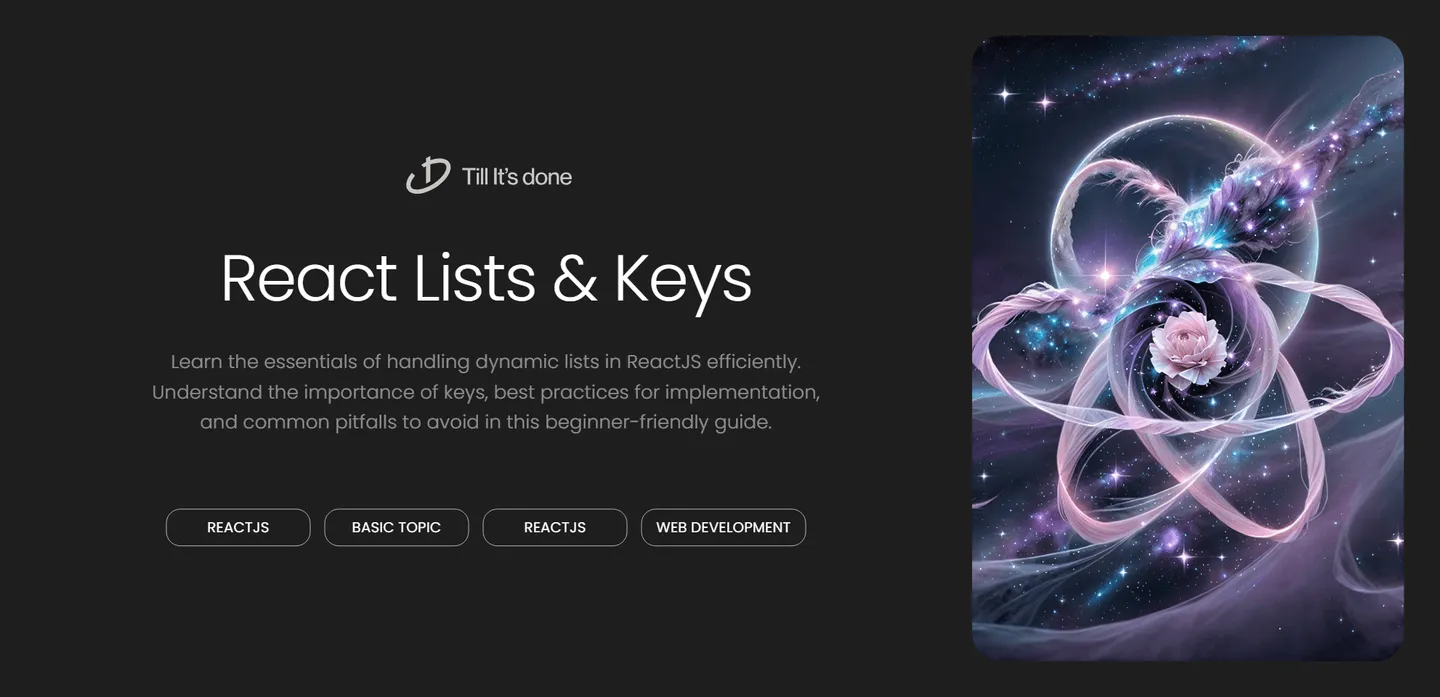
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.