- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Getting Started with Puppeteer in Node.js
This guide covers installation, basic usage, web scraping, form automation, and best practices for browser automation.
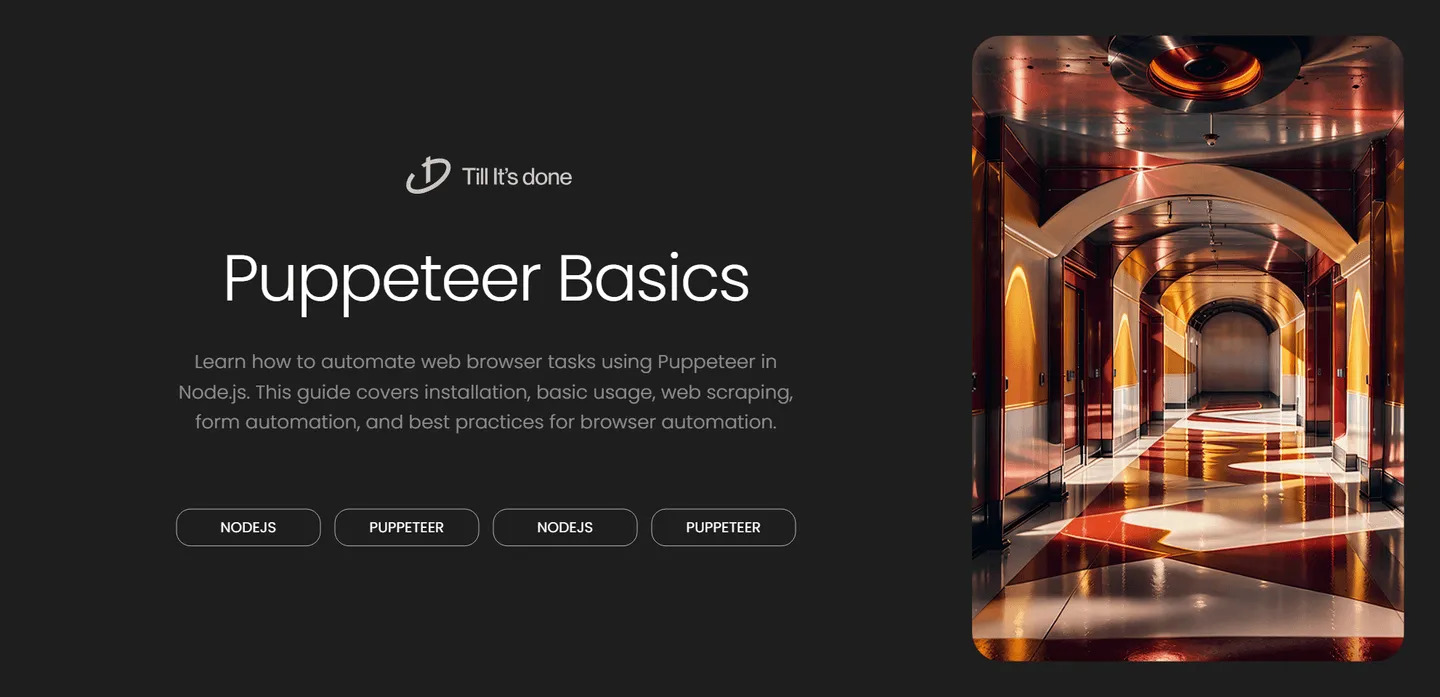
Getting Started with Puppeteer in Node.js
Web automation has become an essential part of modern web development, and Puppeteer stands out as one of the most powerful tools in this domain. In this guide, we’ll explore how to get started with Puppeteer in Node.js and unlock its potential for web scraping, testing, and automation.
What is Puppeteer?
Puppeteer is a Node.js library that provides a high-level API to control Chrome or Chromium programmatically. Think of it as a puppet master controlling a browser – you can tell it exactly what to do, where to click, and what to look for, all through code.
Setting Up Your First Puppeteer Project
First, let’s create a new Node.js project and install Puppeteer. Open your terminal and run:
mkdir puppeteer-democd puppeteer-demonpm init -ynpm install puppeteer
Now, let’s create a simple script that takes a screenshot of a website:
const puppeteer = require('puppeteer');
async function captureScreenshot() { // Launch the browser const browser = await puppeteer.launch();
// Create a new page const page = await browser.newPage();
// Navigate to a website await page.goto('https://example.com');
// Take a screenshot await page.screenshot({ path: 'screenshot.png' });
// Close the browser await browser.close();}
captureScreenshot();
Advanced Features and Common Use Cases
Puppeteer isn’t just about taking screenshots. Here are some powerful things you can do:
Web Scraping
You can extract data from websites using Puppeteer’s powerful selectors:
const data = await page.evaluate(() => { const title = document.querySelector('h1').innerText; const paragraphs = Array.from(document.querySelectorAll('p')) .map(p => p.innerText); return { title, paragraphs };});
Form Automation
Puppeteer excels at automating form submissions:
await page.type('#username', 'myuser');await page.type('#password', 'mypassword');await page.click('#submit');
PDF Generation
You can generate PDFs from web pages:
await page.pdf({ path: 'page.pdf', format: 'A4' });
Best Practices and Tips
- Always close your browser instances to prevent memory leaks
- Use headless mode in production but headed mode for debugging
- Implement proper error handling for network issues
- Set reasonable timeouts for operations
- Use page.waitForSelector() instead of arbitrary delays
Conclusion
Puppeteer opens up a world of possibilities for web automation in Node.js. Whether you’re building a web scraper, generating PDFs, or automating tests, Puppeteer provides the tools you need to get the job done efficiently.
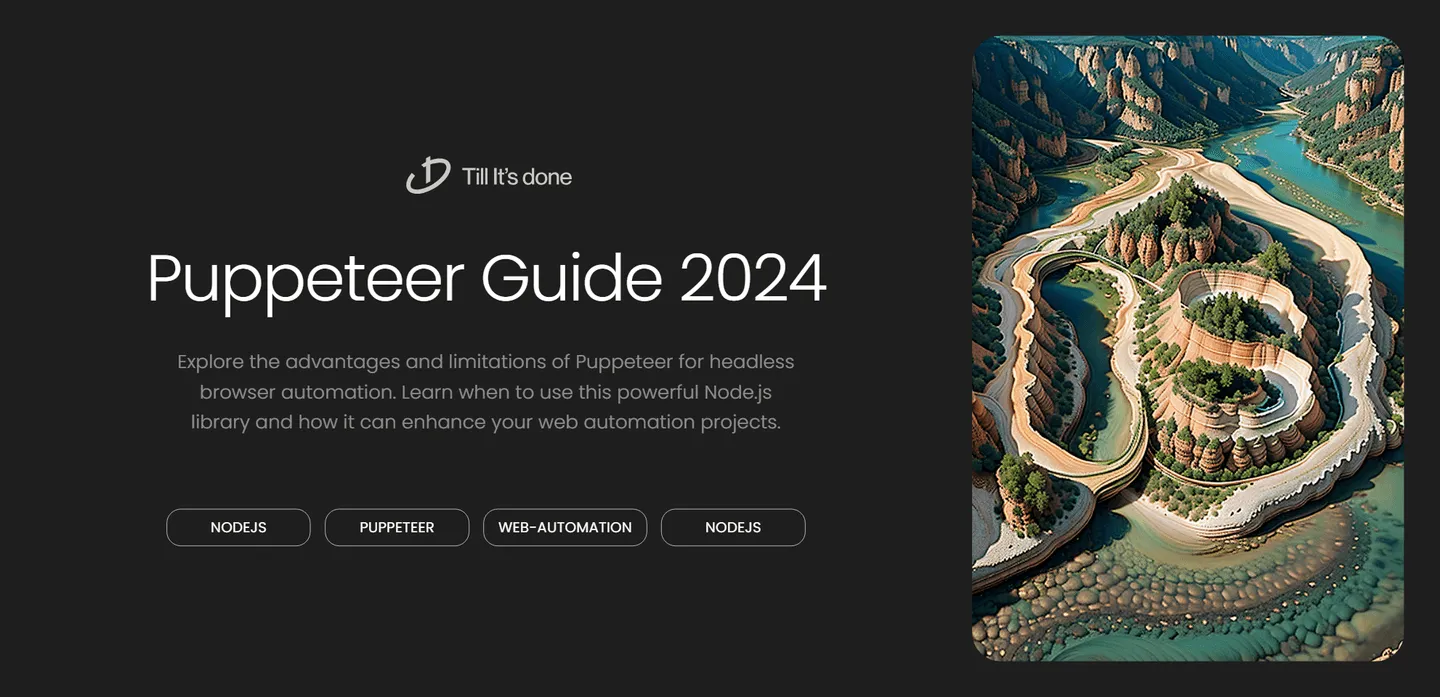
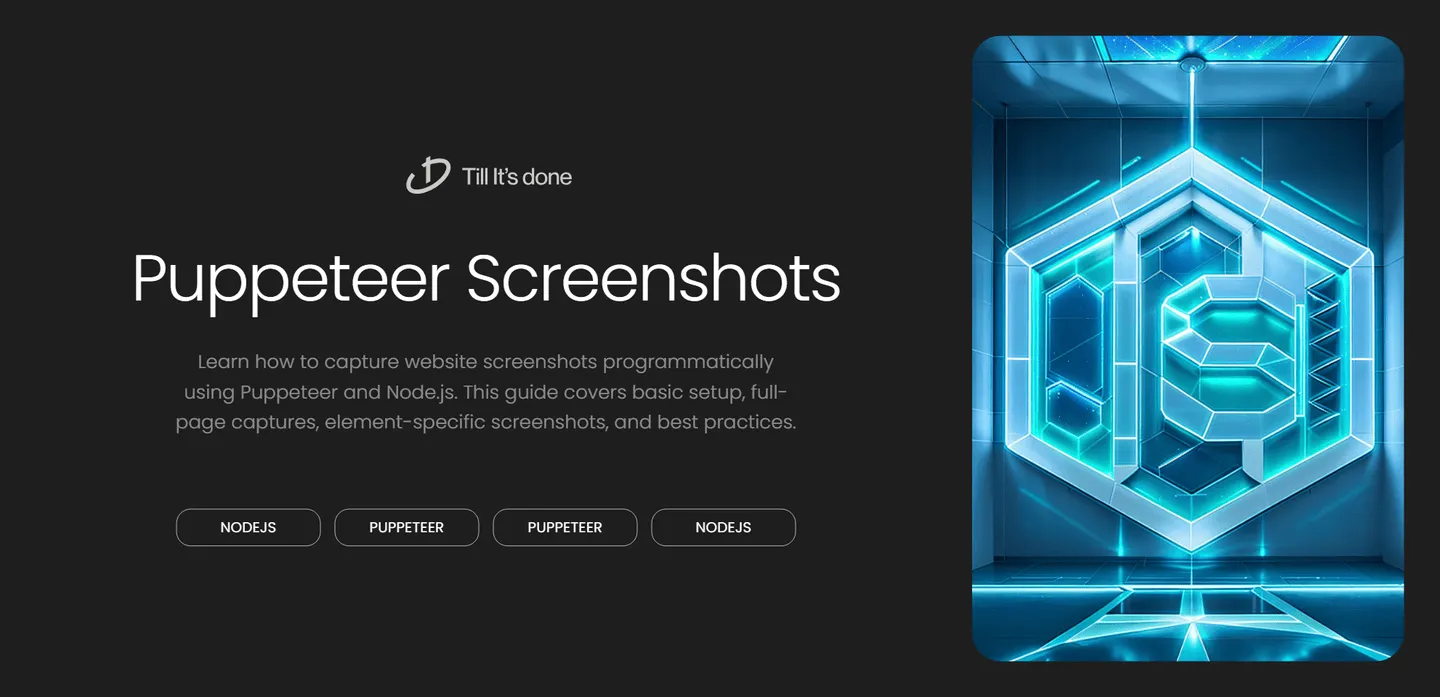
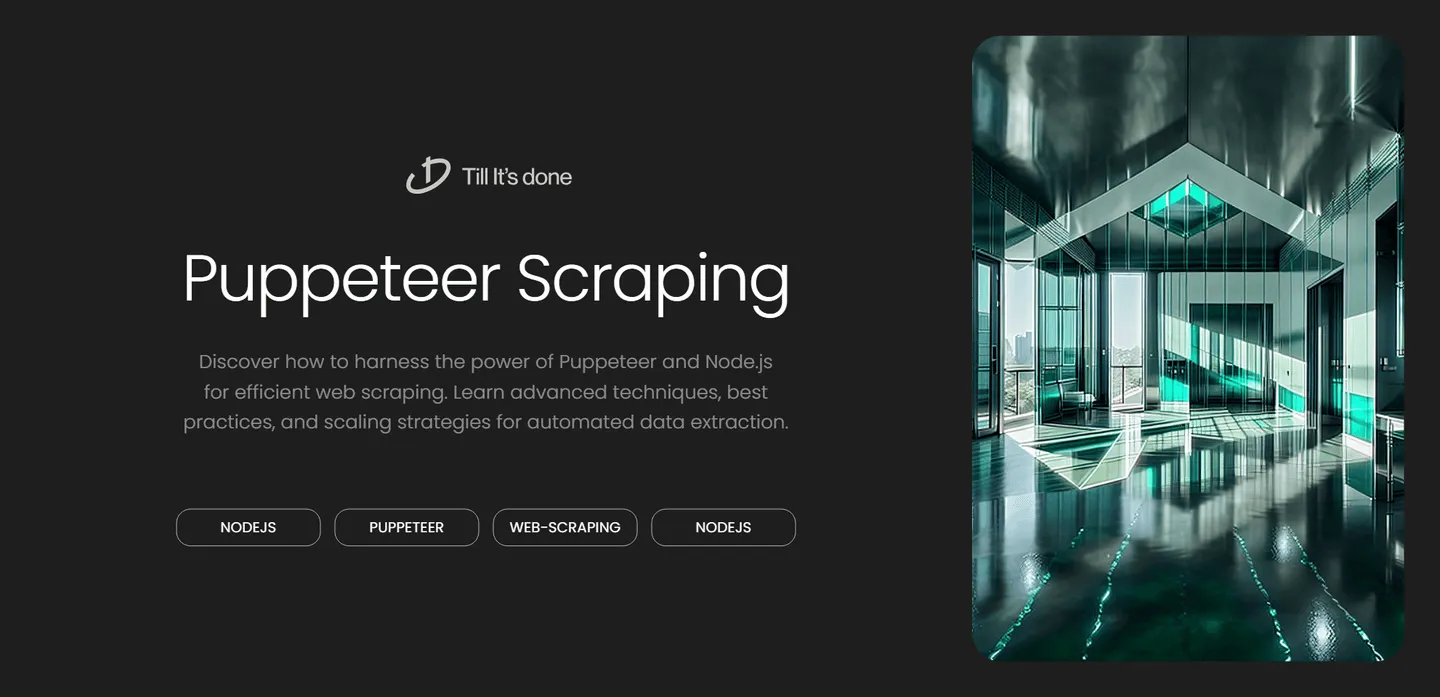
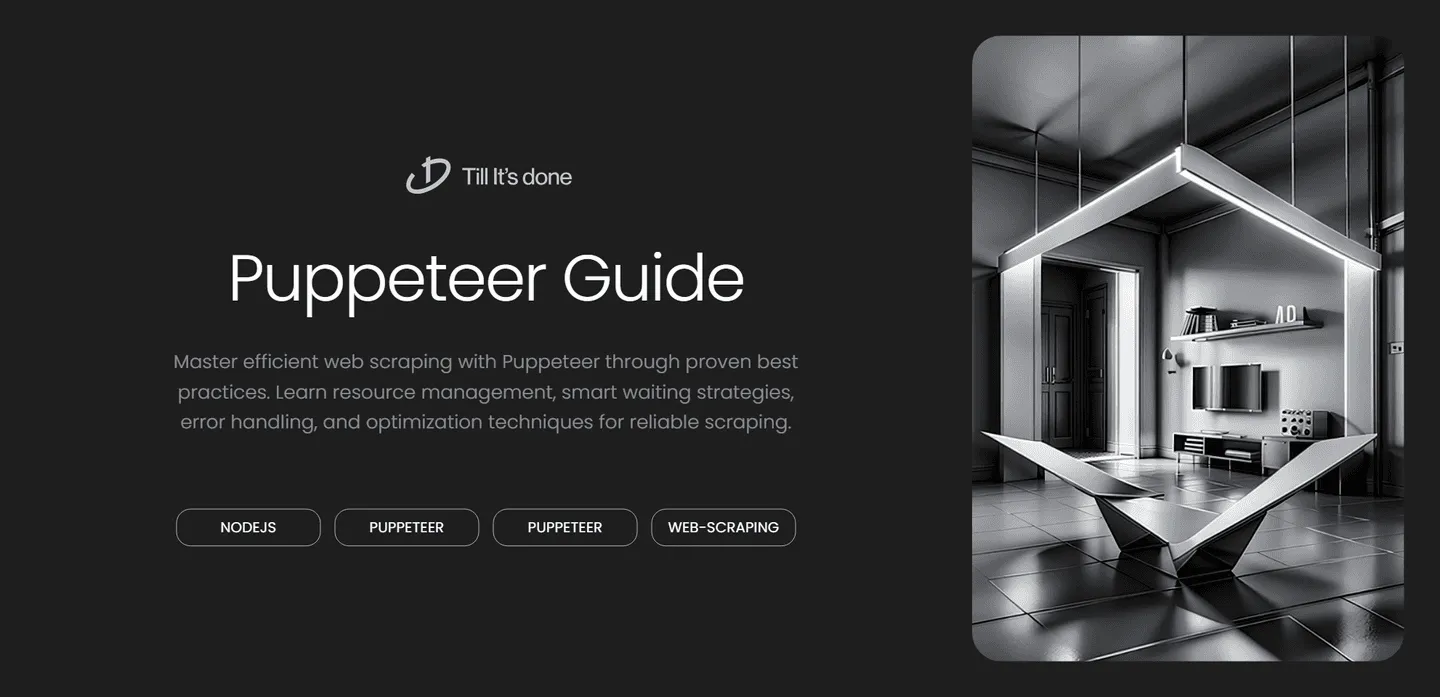
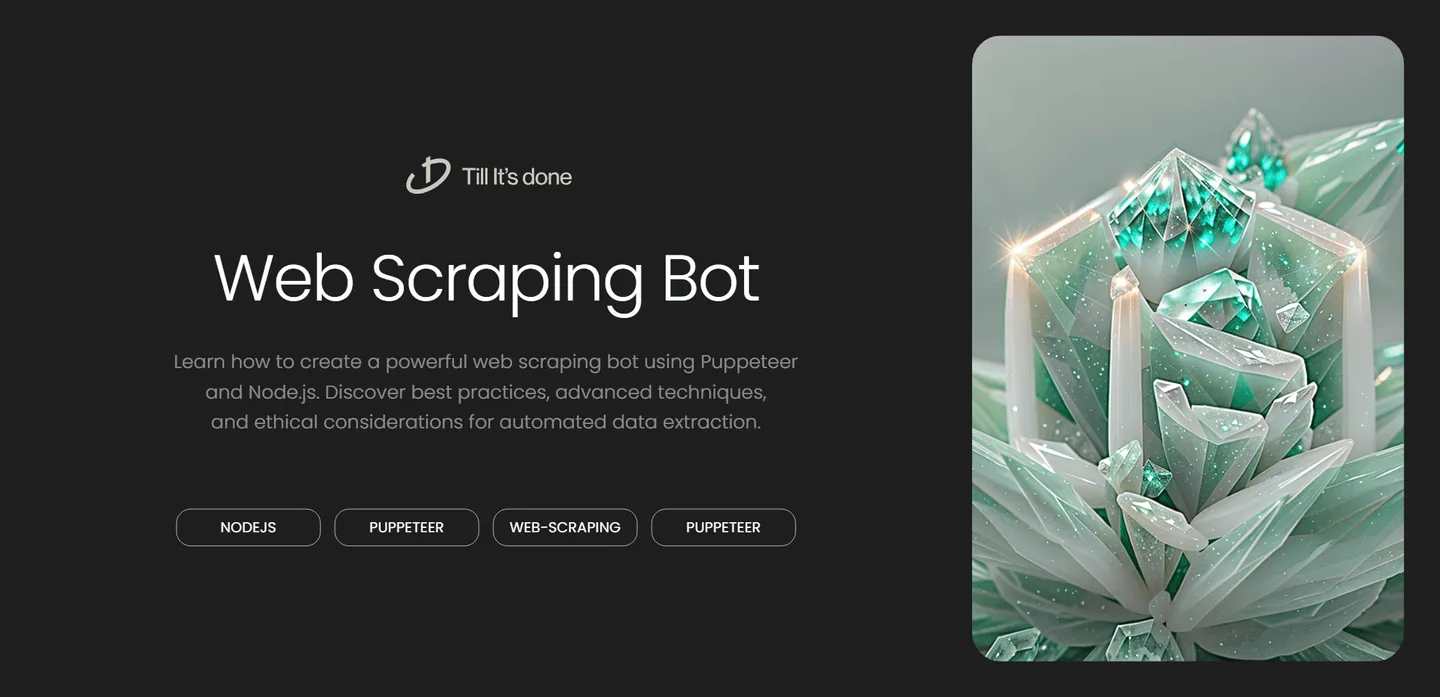
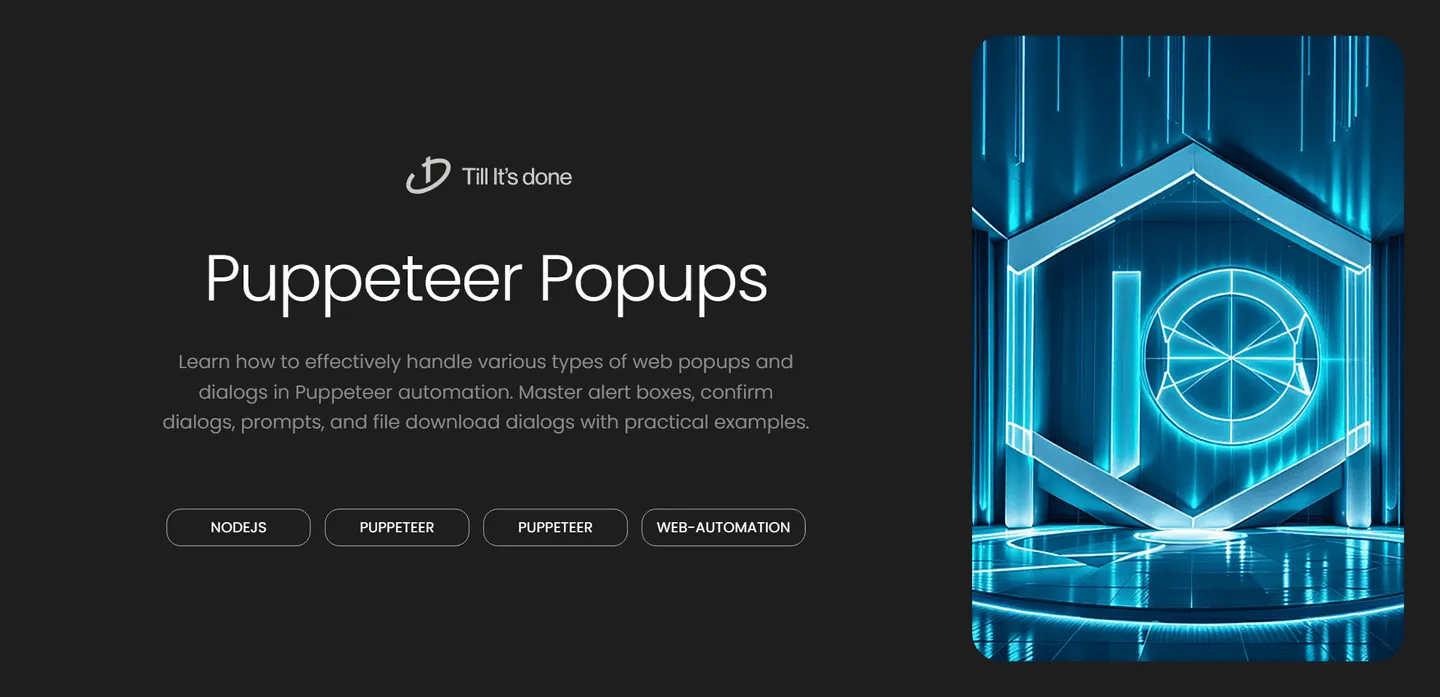
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.