- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Understanding Worker Threads in Node.js Guide
Discover best practices, use cases, and advanced concepts for CPU-intensive tasks and performance optimization.

Understanding and Implementing Worker Threads in Node.js
Node.js is known for its single-threaded nature, but what if we could harness the power of multiple CPU cores? Enter Worker Threads - a powerful feature that enables true parallel processing in Node.js applications.
What are Worker Threads?
Worker Threads allow you to run JavaScript in parallel using threads, sharing memory through SharedArrayBuffer and transferring data through MessageChannel. Unlike the cluster module, Worker Threads can share memory, making them perfect for CPU-intensive tasks.
Why Use Worker Threads?
Traditional Node.js applications run on a single thread, which can become a bottleneck for:
- Complex calculations
- Image/video processing
- Big data operations
- Machine learning tasks
Basic Implementation
Here’s a simple example of implementing Worker Threads:
const { Worker } = require('worker_threads');
function runWorker(workerData) { return new Promise((resolve, reject) => { const worker = new Worker('./worker.js', { workerData }); worker.on('message', resolve); worker.on('error', reject); worker.on('exit', (code) => { if (code !== 0) { reject(new Error(`Worker stopped with exit code ${code}`)); } }); });}
const { parentPort, workerData } = require('worker_threads');
// Perform heavy computationconst result = heavyComputation(workerData);
parentPort.postMessage(result);
Best Practices
-
Thread Pool Management
- Create a pool of workers
- Reuse workers instead of creating new ones
- Implement proper error handling
-
Data Transfer Optimization
- Use transferable objects when possible
- Minimize data copying between threads
- Leverage SharedArrayBuffer for large datasets
-
Resource Management
- Implement proper thread termination
- Monitor memory usage
- Handle thread crashes gracefully
Advanced Concepts
SharedArrayBuffer
const sharedBuffer = new SharedArrayBuffer(1024);const int32Array = new Int32Array(sharedBuffer);
Message Channel
const { MessageChannel } = require('worker_threads');const { port1, port2 } = new MessageChannel();
Common Use Cases
- Image processing pipelines
- Real-time data analysis
- Scientific computations
- Blockchain operations
- Game server calculations
Remember that Worker Threads aren’t always the best solution. Use them wisely when the computational benefits outweigh the overhead of thread management.


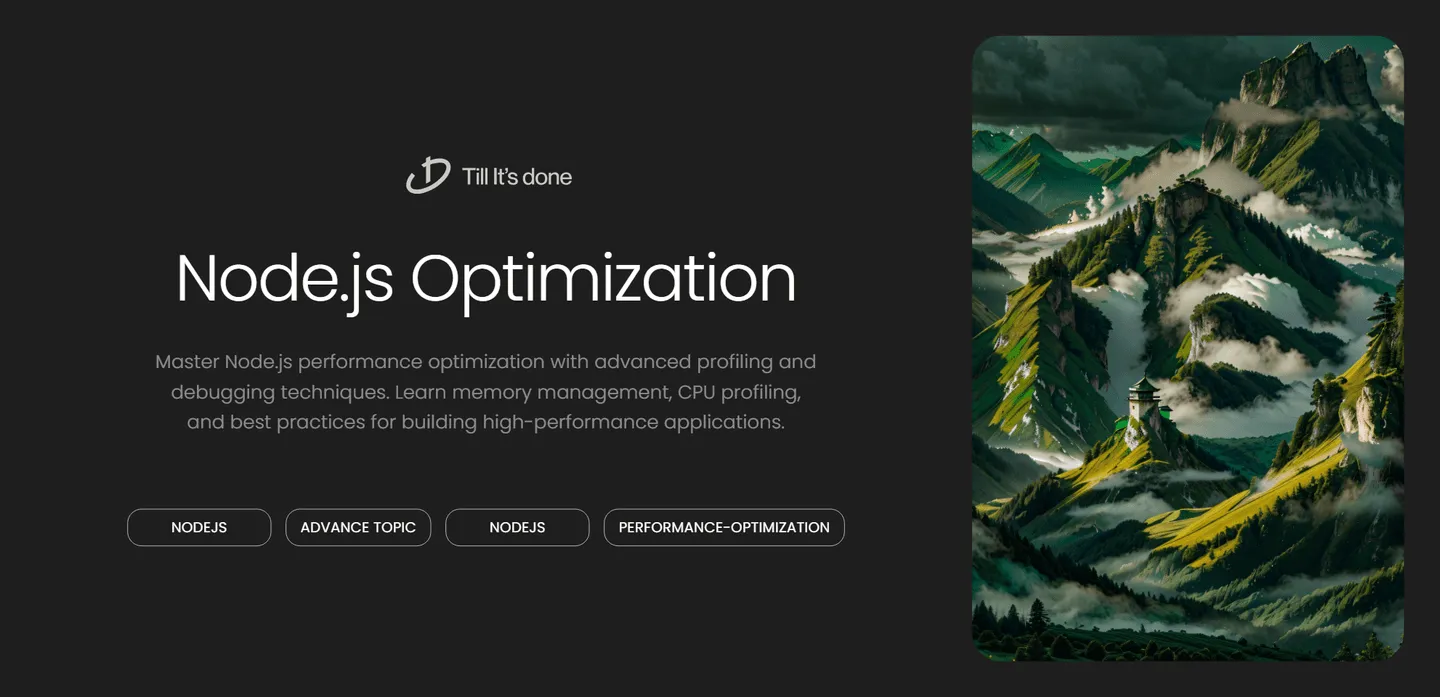

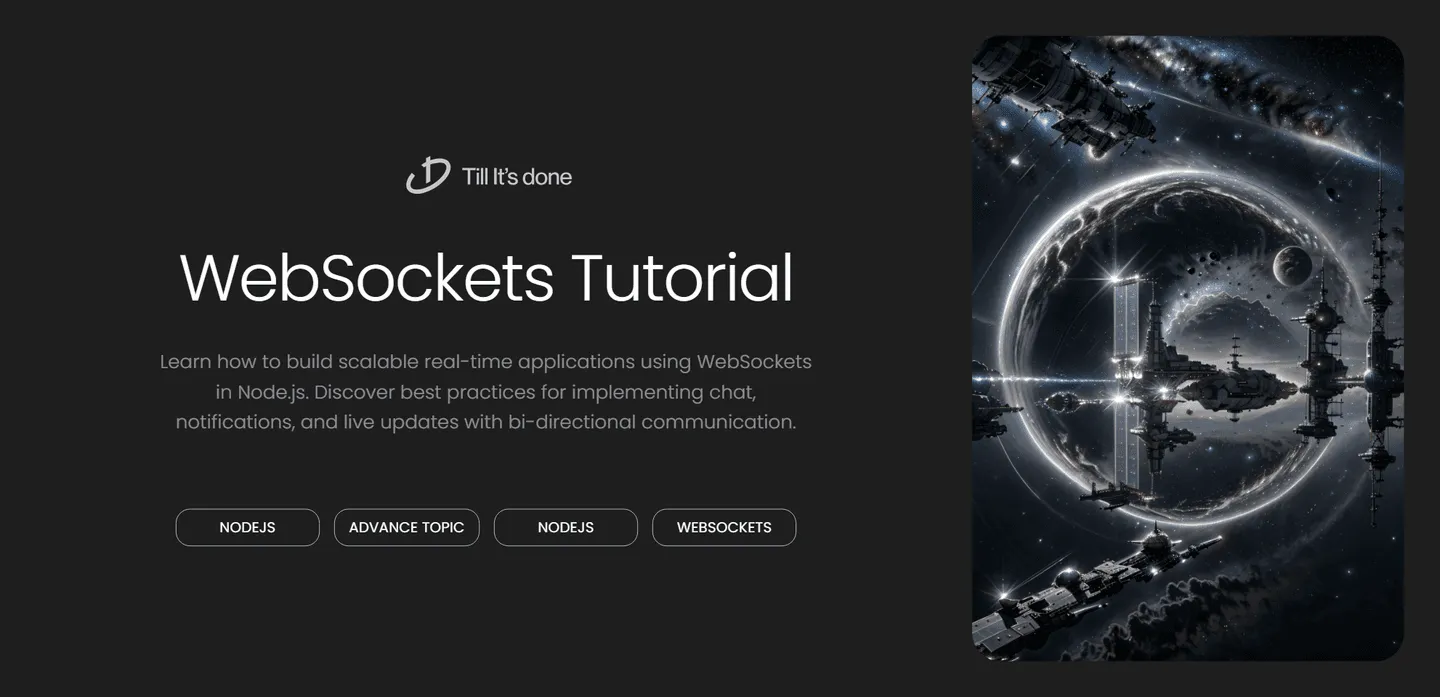
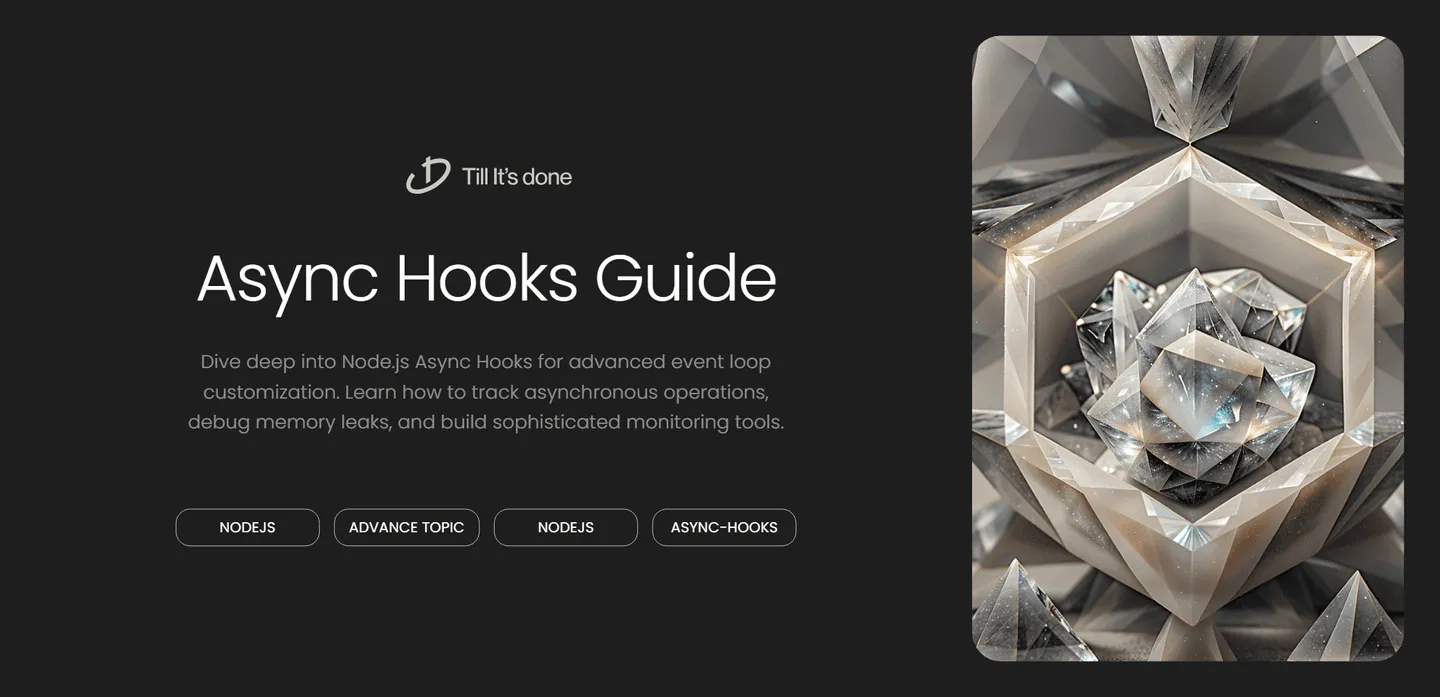
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.