- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Unit Testing and Code Coverage in Node.js
Discover Jest testing framework, code coverage metrics, and best practices for reliable testing.
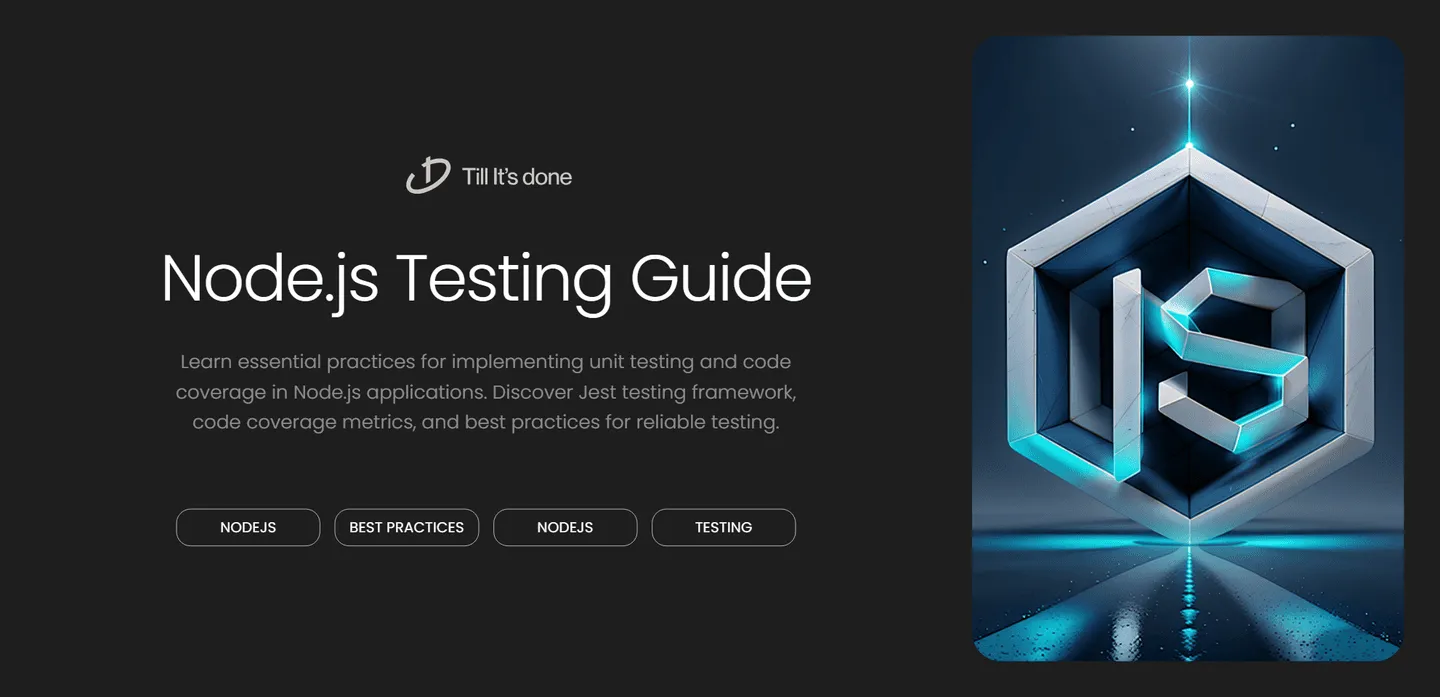
Unit Testing and Code Coverage in Node.js: A Developer’s Guide
In the ever-evolving landscape of Node.js development, unit testing and code coverage aren’t just buzzwords – they’re essential practices that can make or break your application’s reliability. Let’s dive into how you can implement these crucial practices effectively.
Why Unit Testing Matters
Testing isn’t just about finding bugs; it’s about building confidence in your code. When you’re working on a Node.js application, especially in a team environment, unit tests serve as your first line of defense against potential issues.
Getting Started with Jest
Jest has emerged as the go-to testing framework for Node.js applications. Here’s how to set up your testing environment:
- Install Jest as a dev dependency:
npm install --save-dev jest
- Update your package.json:
{ "scripts": { "test": "jest", "test:coverage": "jest --coverage" }}
Writing Your First Test
Let’s look at a practical example. Suppose we have a simple utility function:
function add(a, b) { return a + b;}module.exports = { add };
Here’s how we’d test it:
const { add } = require('../calculator');
describe('Calculator', () => { test('adds two numbers correctly', () => { expect(add(2, 3)).toBe(5); });});
Understanding Code Coverage
Code coverage helps you understand how much of your code is being tested. Jest provides comprehensive coverage reports that include:
- Statement coverage
- Branch coverage
- Function coverage
- Line coverage
Best Practices
- Test in Isolation: Mock external dependencies to ensure true unit testing.
- Follow the AAA Pattern: Arrange, Act, Assert.
- Keep Tests Simple: One test should verify one specific behavior.
- Maintain Test Quality: Tests should be as well-maintained as production code.
Setting Coverage Thresholds
Add coverage thresholds to ensure maintaining high testing standards:
module.exports = { coverageThreshold: { global: { branches: 80, functions: 80, lines: 80, statements: 80 } }};
Continuous Integration
Integrate testing into your CI/CD pipeline to automate quality checks. Popular tools like GitHub Actions make this straightforward:
name: Testson: [push, pull_request]jobs: test: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - run: npm install - run: npm test
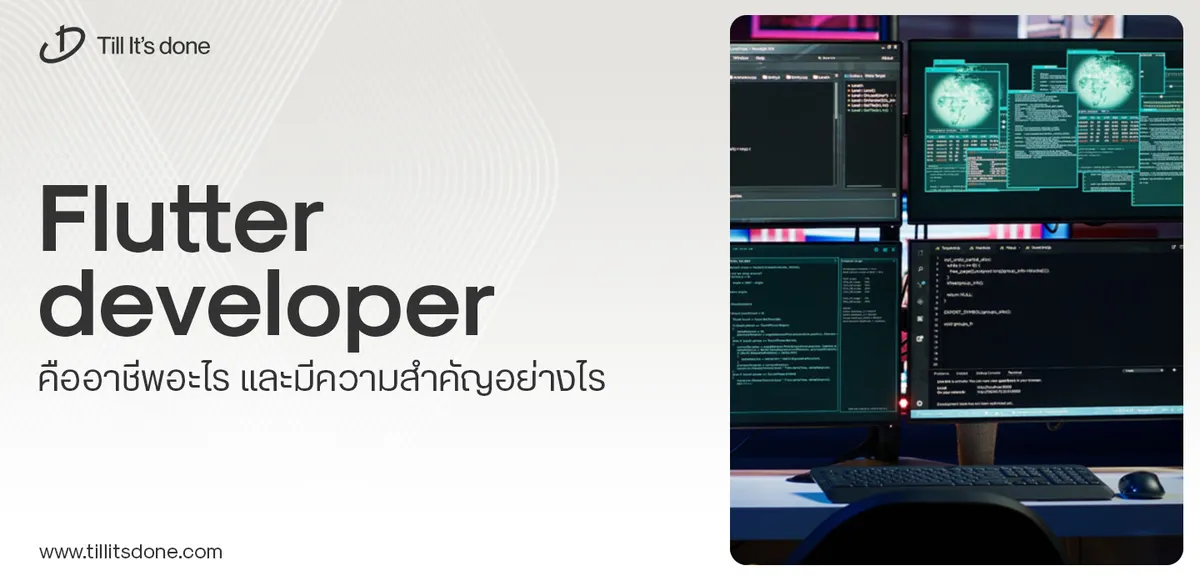
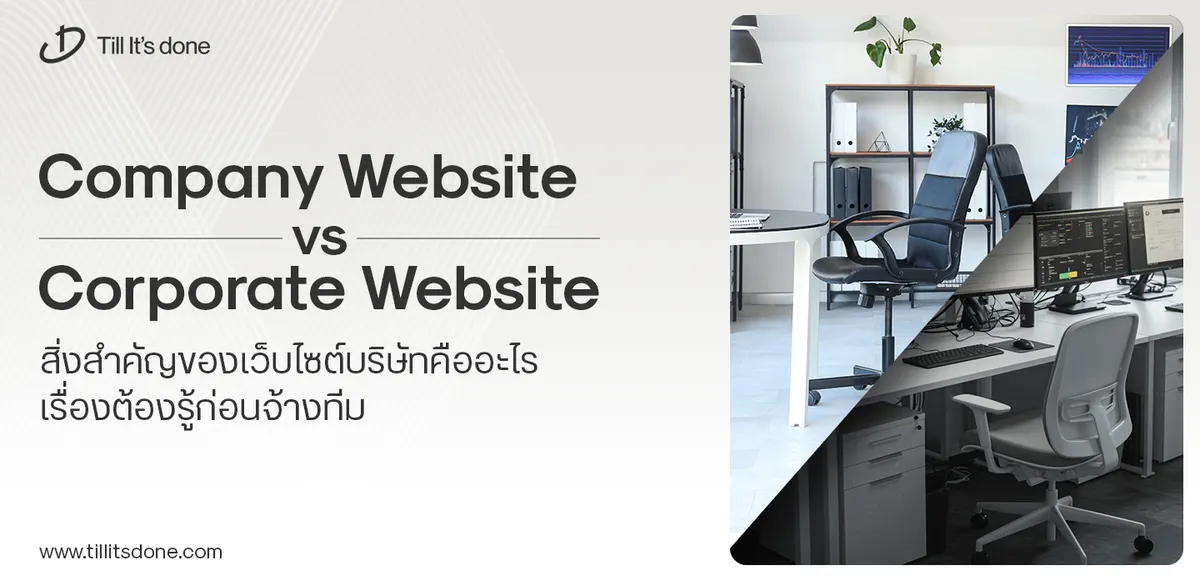
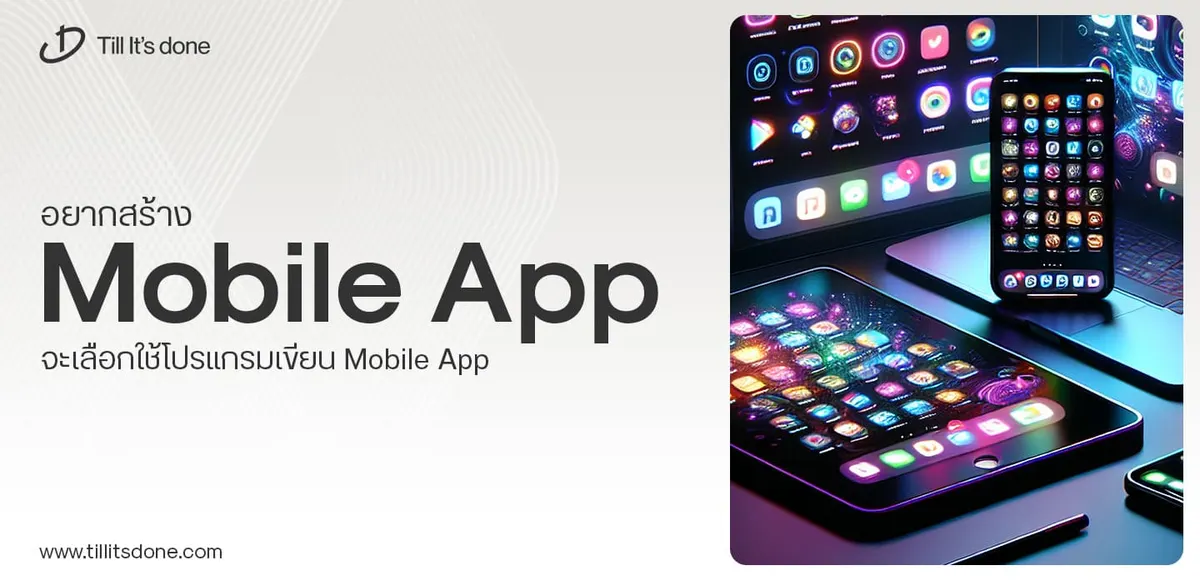
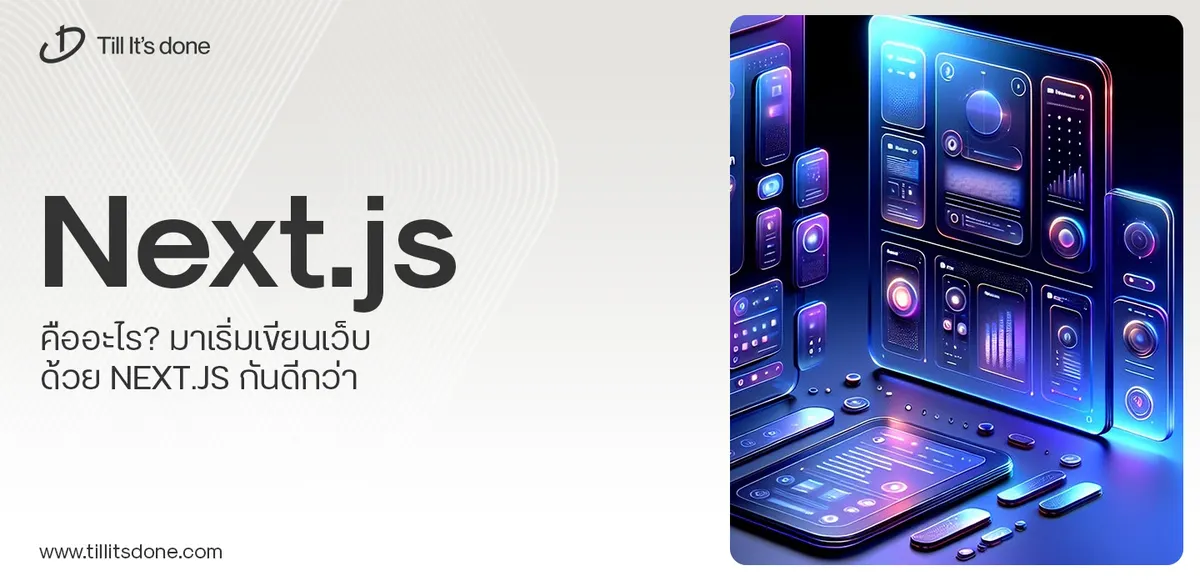
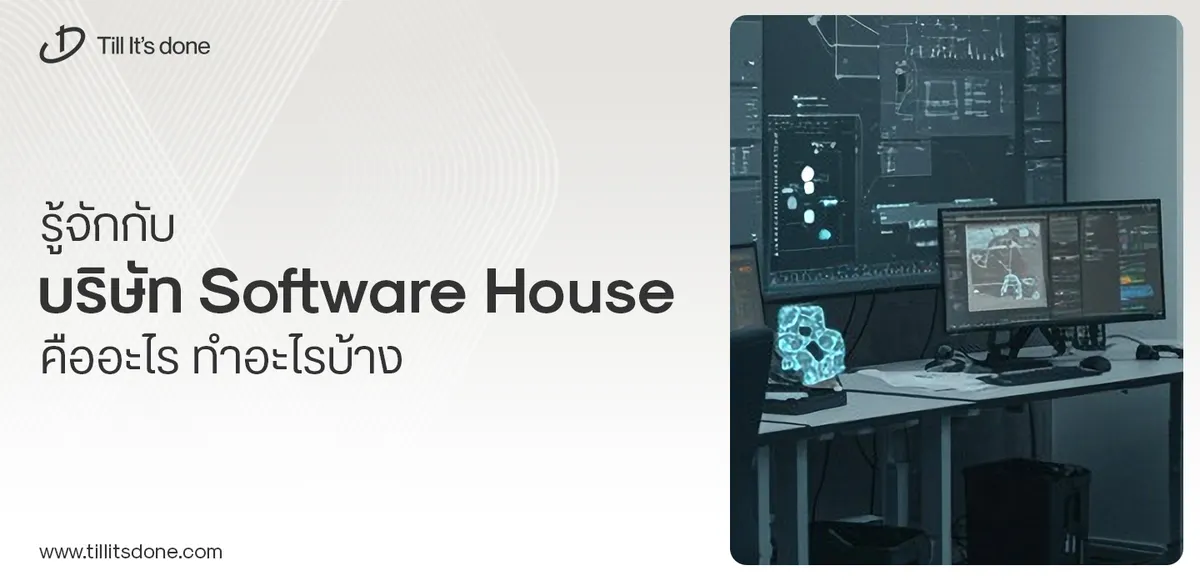
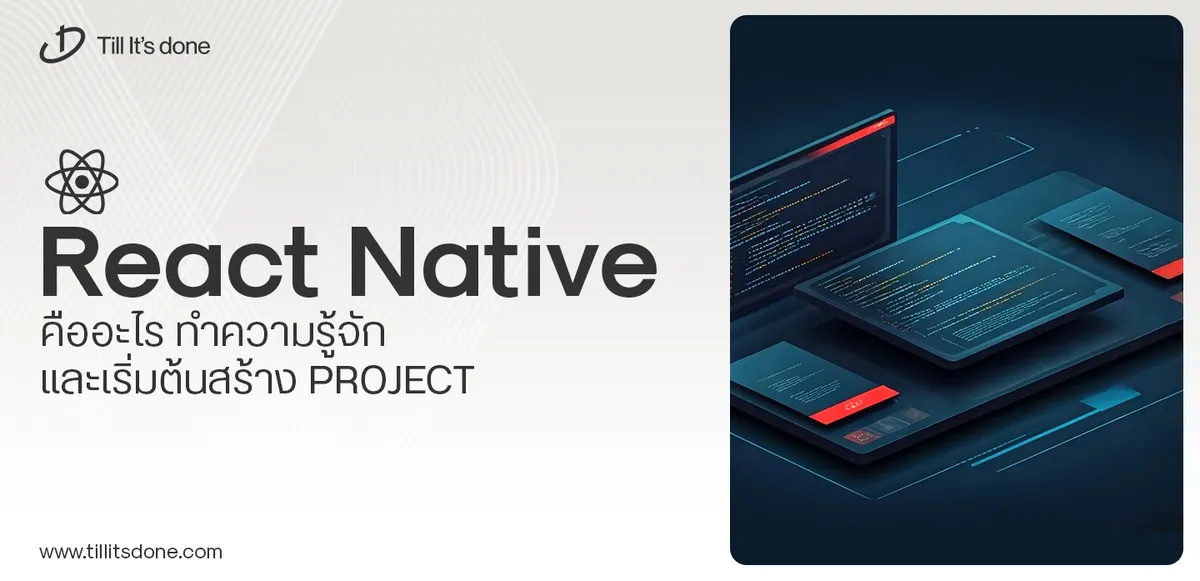
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.