- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Mastering Async Hooks in Node.js Event Loop
Learn how to track asynchronous operations, debug memory leaks, and build sophisticated monitoring tools.
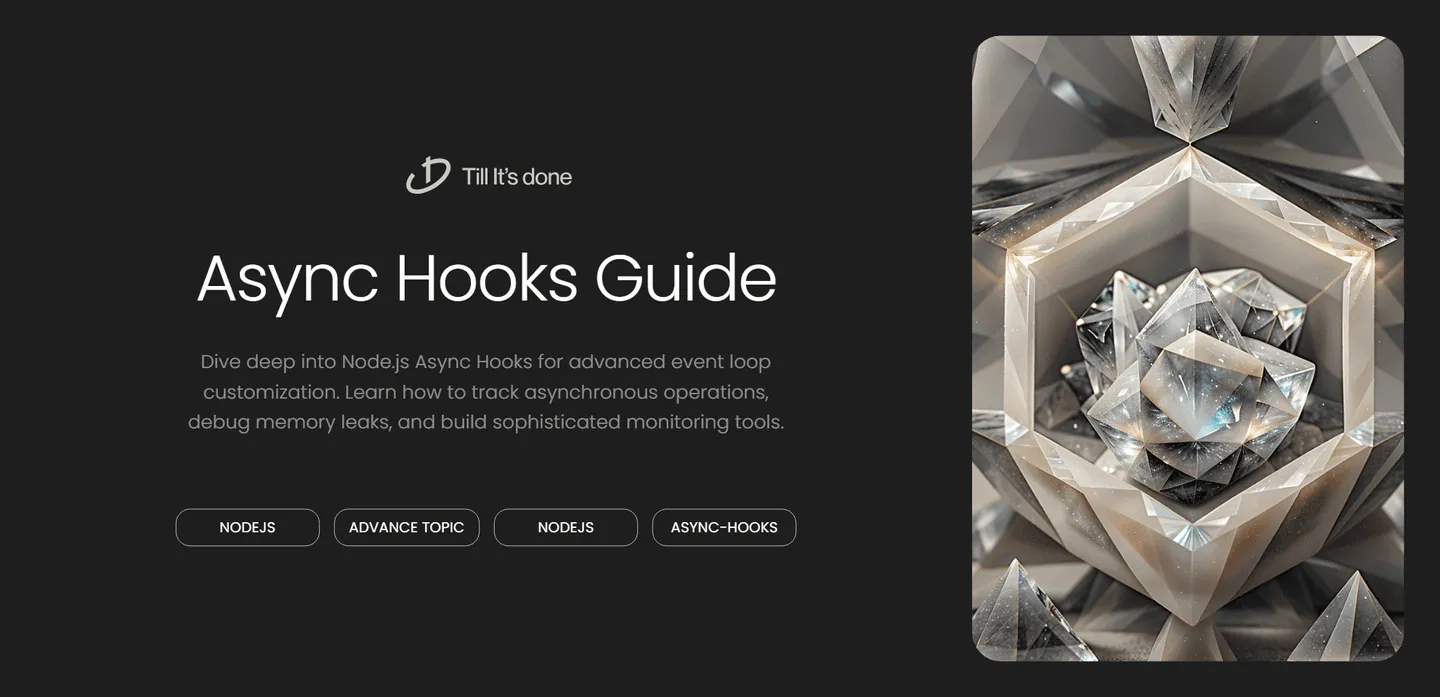
Customizing the Event Loop: Async Hooks in Node.js
Node.js’s event loop is like the heartbeat of your application, orchestrating asynchronous operations behind the scenes. But what if you could peek under the hood and track these operations? That’s where Async Hooks come in - they’re like attaching sensors to your application’s nervous system.
Understanding Async Hooks
Think of Async Hooks as special observers that let you track the lifecycle of your asynchronous resources. Whether it’s a timeout, a database query, or a file operation, Async Hooks give you X-ray vision into what’s happening.
Here’s a simple example of creating an async hook:
const async_hooks = require('async_hooks');const hook = async_hooks.createHook({ init(asyncId, type, triggerAsyncId) { console.log(`Async resource created: ${type}`); }, destroy(asyncId) { console.log(`Async resource destroyed`); }});
Practical Applications
One of the most powerful uses of Async Hooks is debugging memory leaks. By tracking which async resources aren’t being properly cleaned up, you can identify bottlenecks in your application.
let resources = new Map();
const hook = async_hooks.createHook({ init(asyncId, type) { resources.set(asyncId, type); }, destroy(asyncId) { resources.delete(asyncId); }});
Advanced Tracking Techniques
You can also use Async Hooks to build sophisticated monitoring tools. For instance, tracking the execution chain of async operations:
const executionChain = new Map();
hook.enable();
function trackAsyncOperation(operation) { const triggerAsyncId = async_hooks.executionAsyncId(); executionChain.set(triggerAsyncId, operation);}
Performance Considerations
While Async Hooks are powerful, they do come with a performance overhead. Use them judiciously in production environments. Consider enabling them only when needed for debugging or monitoring specific issues.
Remember that the event loop is sacred in Node.js - any performance impact on it can cascade through your entire application. Always profile your application with and without Async Hooks to understand the impact.
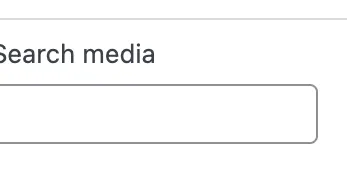





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.