- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Node.js API Rate Limiting for High Traffic
Explore fixed window, sliding window, and token bucket implementations with practical examples.

Node.js API Rate Limiting Techniques for High Traffic Applications
In today’s digital landscape, building scalable APIs that can handle high traffic volumes while maintaining performance and security is crucial. One of the most effective ways to protect your Node.js API from abuse and ensure fair resource usage is through rate limiting. Let’s dive into various rate-limiting techniques and implementation strategies.
Understanding Rate Limiting
Rate limiting is like having a bouncer at a popular club – it controls how many requests a user can make within a specific timeframe. This prevents any single client from overwhelming your server or monopolizing resources.
Popular Rate Limiting Strategies
Fixed Window Rate Limiting
This is the simplest form of rate limiting. For example, allowing 100 requests per hour. Think of it as a bucket that empties completely at the start of each hour. While simple to implement, it can lead to traffic spikes at window boundaries.
const rateLimit = require('express-rate-limit');
const limiter = rateLimit({ windowMs: 60 * 60 * 1000, // 1 hour max: 100 // limit each IP to 100 requests per windowMs});
app.use(limiter);
Sliding Window Rate Limiting
This technique offers more granular control by tracking requests over a rolling time window. It’s like having a queue that continuously moves forward, dropping off old requests and adding new ones.
Using Redis with the sliding window algorithm provides excellent scalability:
const Redis = require('ioredis');const redis = new Redis();
async function slidingWindowRateLimiter(userId, windowSize, maxRequests) { const now = Date.now(); const windowKey = `ratelimit:${userId}`;
await redis.zremrangebyscore(windowKey, 0, now - windowSize); const requestCount = await redis.zcard(windowKey);
if (requestCount >= maxRequests) { return false; }
await redis.zadd(windowKey, now, now); await redis.expire(windowKey, windowSize / 1000); return true;}
Token Bucket Algorithm
This approach models rate limiting as a bucket that continuously fills with tokens at a fixed rate. Each request consumes one token, and once the bucket is empty, requests are rejected until new tokens arrive.
class TokenBucket { constructor(capacity, fillPerSecond) { this.capacity = capacity; this.fillPerSecond = fillPerSecond; this.tokens = capacity; this.lastFill = Date.now(); }
consume() { this.refill(); if (this.tokens > 0) { this.tokens -= 1; return true; } return false; }
refill() { const now = Date.now(); const deltaSeconds = (now - this.lastFill) / 1000; this.tokens = Math.min( this.capacity, this.tokens + deltaSeconds * this.fillPerSecond ); this.lastFill = now; }}
Best Practices for Implementation
- Use distributed rate limiting with Redis for scalability
- Implement proper error responses (429 Too Many Requests)
- Include rate limit information in response headers
- Consider different limits for different API endpoints
- Monitor and adjust limits based on usage patterns
Advanced Considerations
- Implement retry-after headers
- Use dynamic rate limits based on user tiers
- Consider rate limiting by IP and by user account
- Implement backup strategies for when Redis is down
- Monitor rate limiting metrics for system health
Remember to balance security with user experience. Too strict limits can frustrate legitimate users, while too lenient ones might not protect your system effectively.

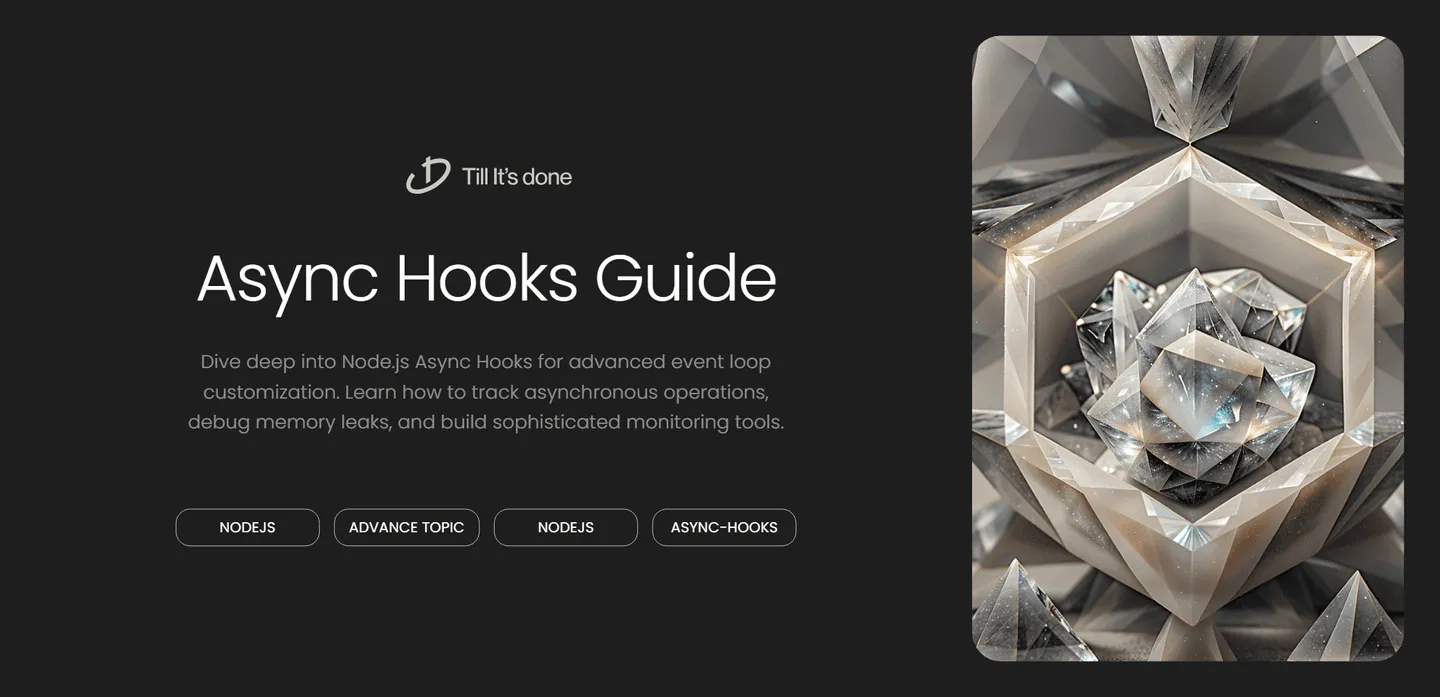


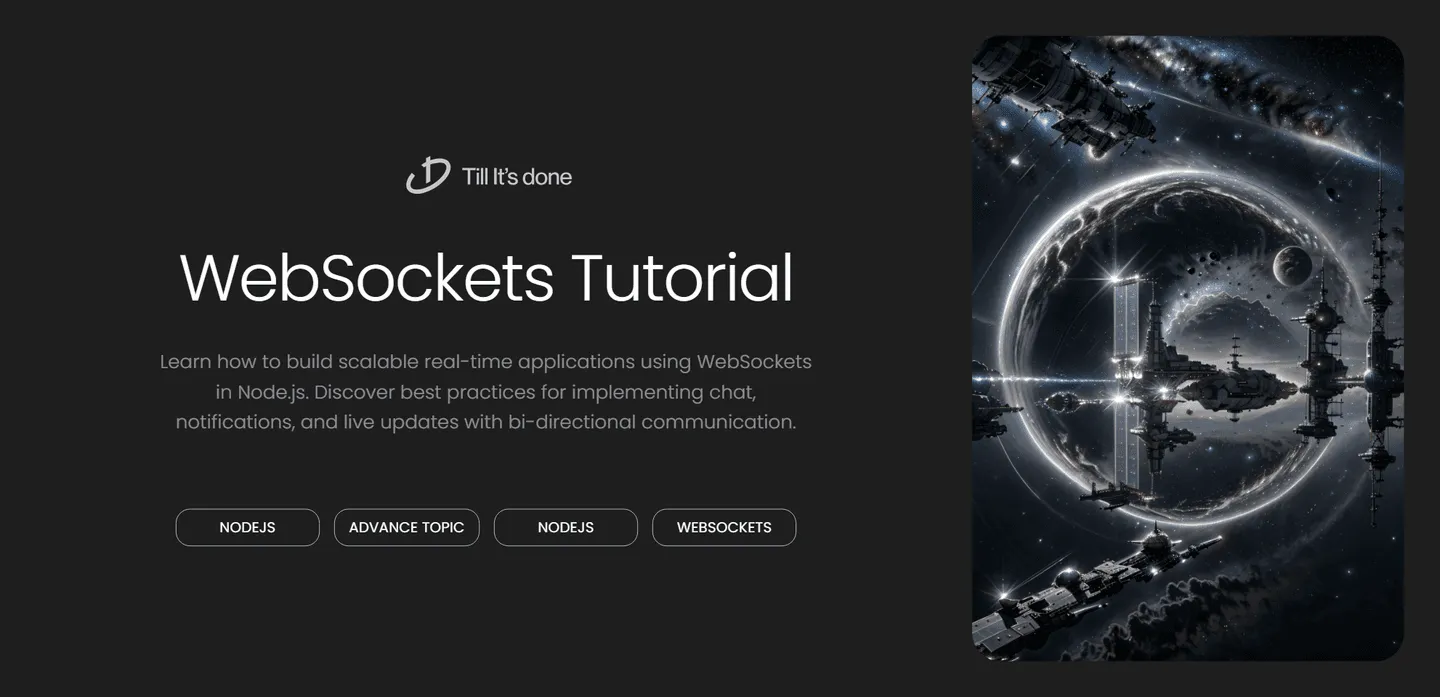

Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.