- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Advanced Patterns for State Management in Next.js
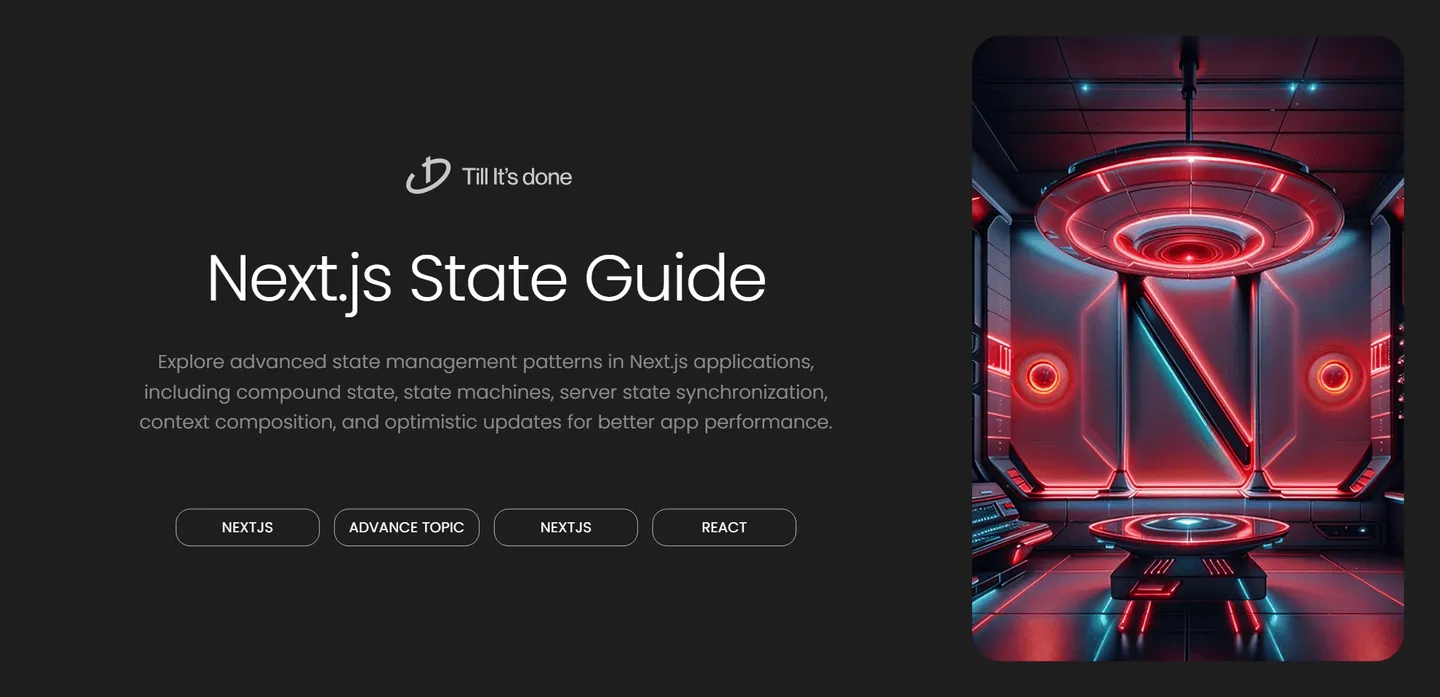
Advanced Patterns for State Management in Next.js Apps
Managing state in modern Next.js applications can be challenging as your application grows in complexity. Let’s explore some advanced patterns that can help you maintain clean, scalable, and performant state management in your Next.js projects.
Understanding Client and Server State
One of the most crucial aspects of Next.js state management is distinguishing between client and server state. With the introduction of React Server Components, we need to be more mindful about where and how we manage our state.
Advanced State Management Patterns
1. Compound State Pattern
Instead of storing multiple related pieces of state separately, we can combine them into a single, cohesive state object. This approach reduces the number of state updates and makes our code more maintainable:
// Instead of thisconst [isLoading, setIsLoading] = useState(false);const [error, setError] = useState(null);const [data, setData] = useState(null);
// Use thisconst [state, setState] = useState({ status: 'idle', // idle | loading | success | error error: null, data: null});
2. State Machines for Complex UI
When dealing with complex UI states, implementing a state machine can make your code more predictable and easier to debug. Libraries like XState work great with Next.js:
const todoMachine = createMachine({ initial: 'idle', states: { idle: { on: { FETCH: 'loading' } }, loading: { on: { SUCCESS: 'success', ERROR: 'error' } }, success: { on: { RETRY: 'loading' } }, error: { on: { RETRY: 'loading' } } }});
3. Server State Synchronization
With Next.js 13+ and React Server Components, we can implement efficient server state synchronization:
async function PostsList() { const posts = await fetchPosts(); return <PostsClient initialData={posts} />;}
4. Context Composition Pattern
Instead of having one giant context provider, break down your state into smaller, focused contexts:
const AuthProvider = ({ children }) => { // Auth-specific state};
const ThemeProvider = ({ children }) => { // Theme-specific state};
const AppProvider = ({ children }) => ( <AuthProvider> <ThemeProvider>{children}</ThemeProvider> </AuthProvider>);
5. Optimistic Updates Pattern
Implement optimistic updates to make your app feel more responsive:
const updateTodo = async (id, newData) => { // Update local state immediately setTodos(current => current.map(todo => todo.id === id ? { ...todo, ...newData } : todo ) );
try { // Perform actual API update await api.updateTodo(id, newData); } catch (error) { // Revert on error setTodos(previousTodos); showError(error); }};
Best Practices and Tips
- Use React Query or SWR for server state management
- Implement proper error boundaries for state-related errors
- Keep state as close as possible to where it’s needed
- Use TypeScript for better state type safety
- Consider using Zustand for global state management
- Implement proper loading and error states
Remember that the best state management pattern depends on your specific use case. Don’t overcomplicate things if a simpler solution works well for your needs.
Conclusion
Advanced state management in Next.js requires careful consideration of where and how state is managed. By implementing these patterns appropriately, you can build more maintainable and scalable applications while providing a better user experience.
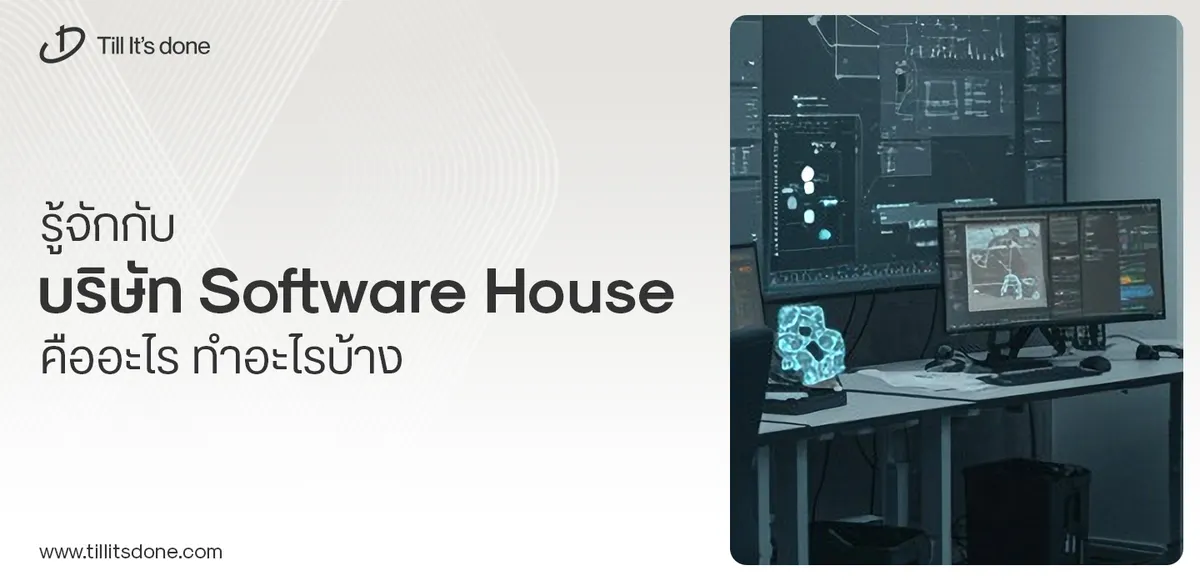
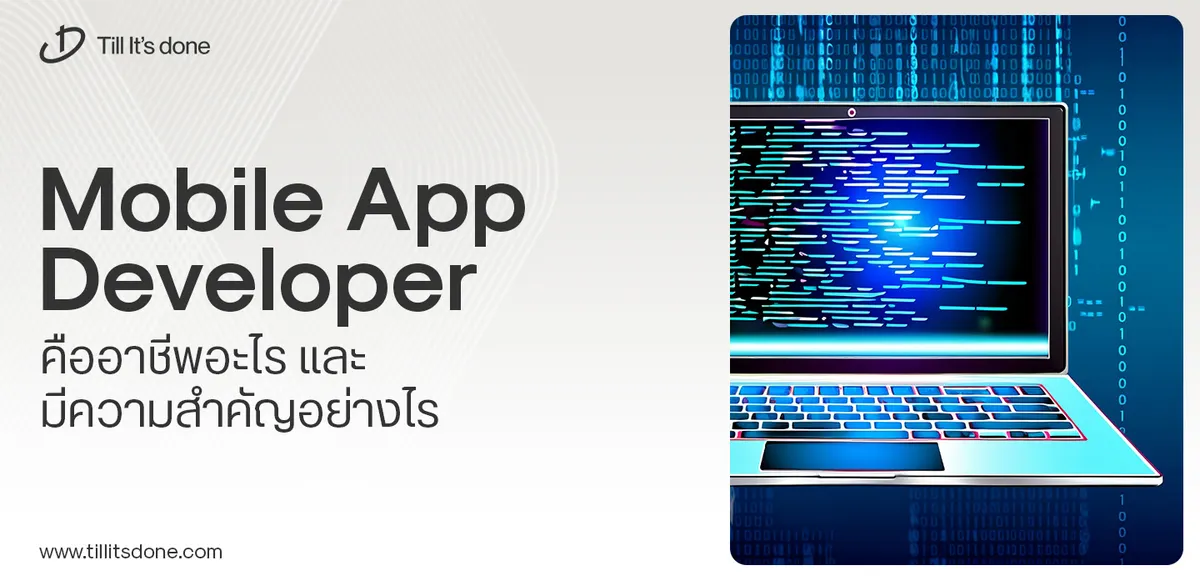
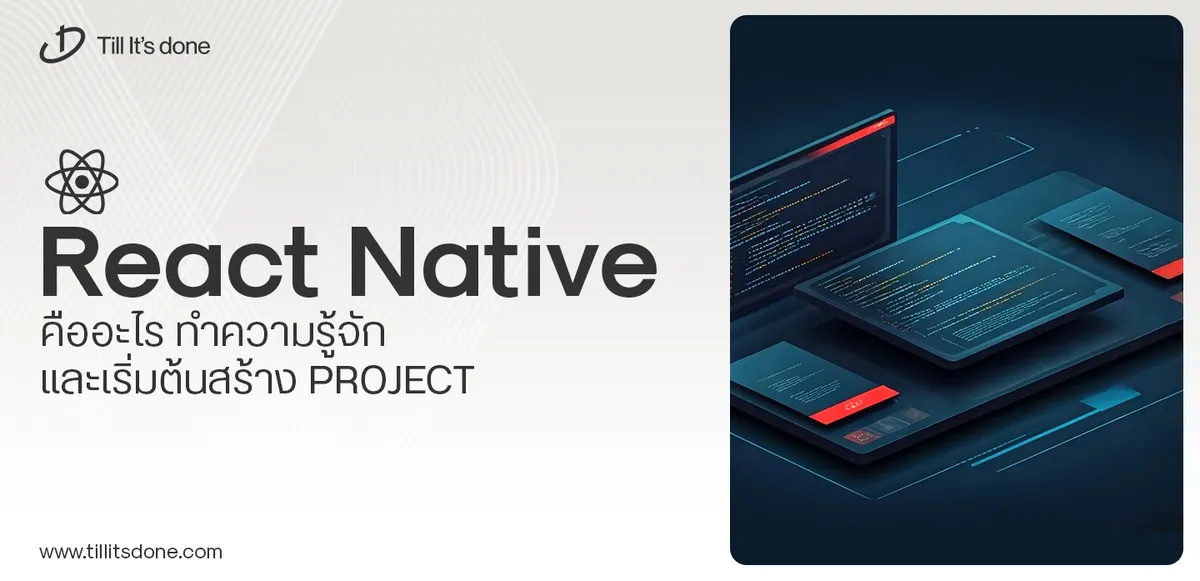
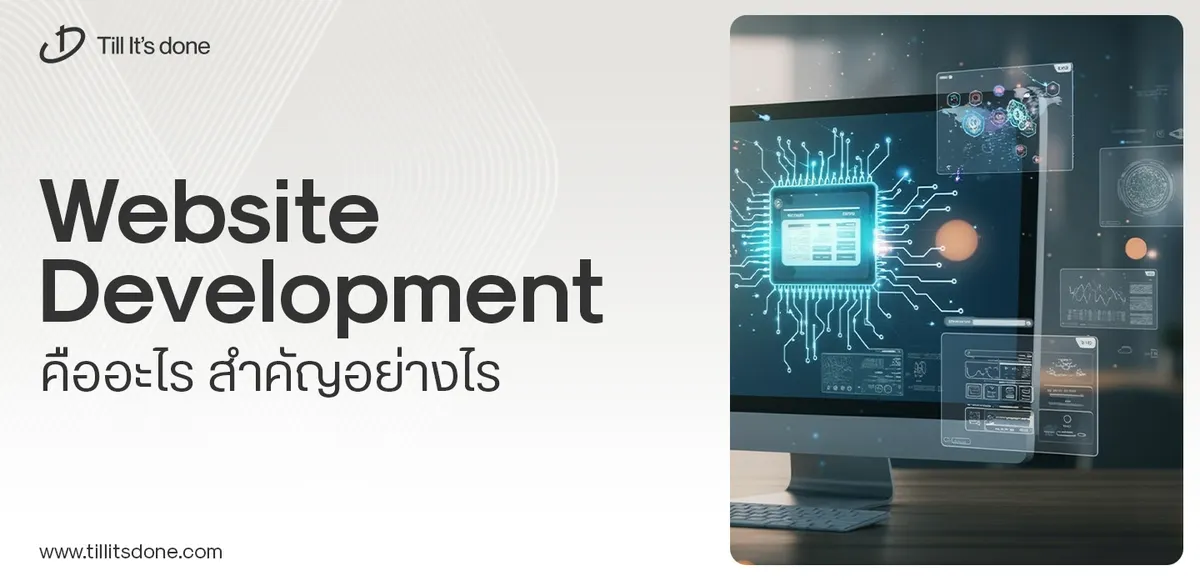
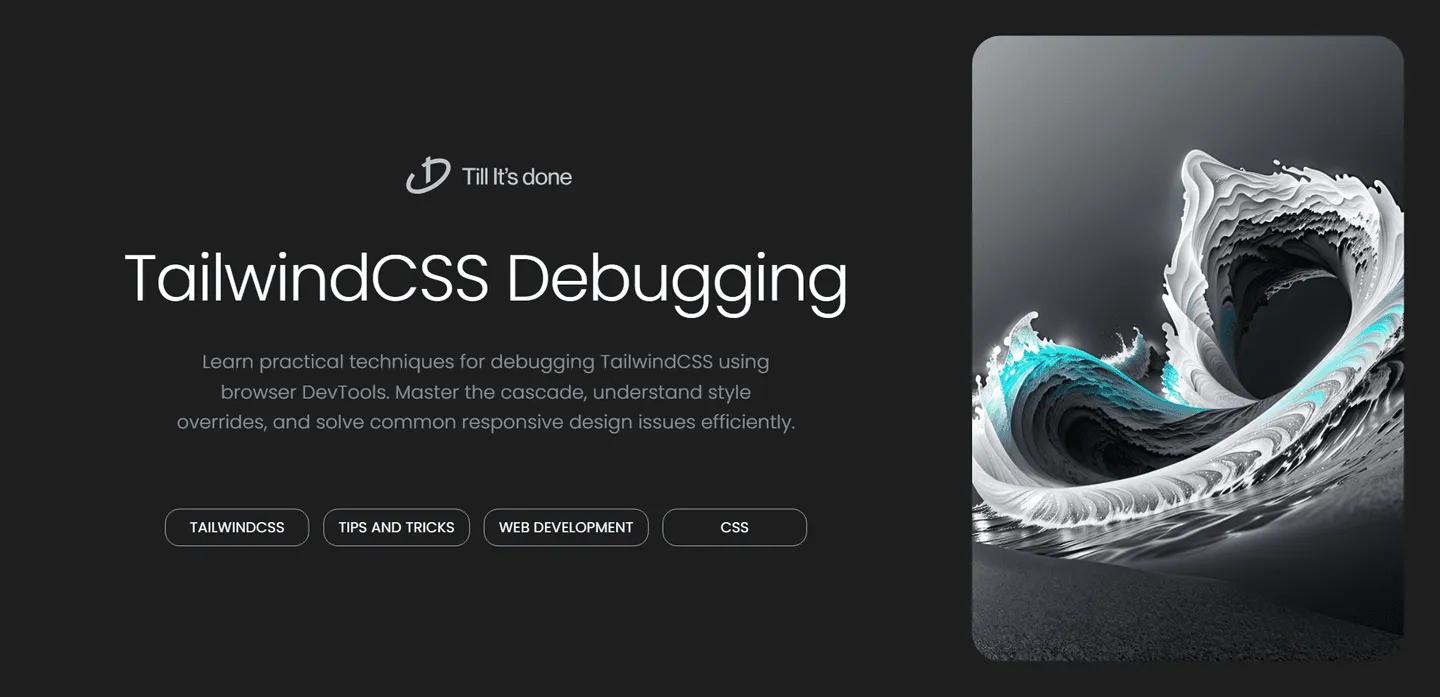
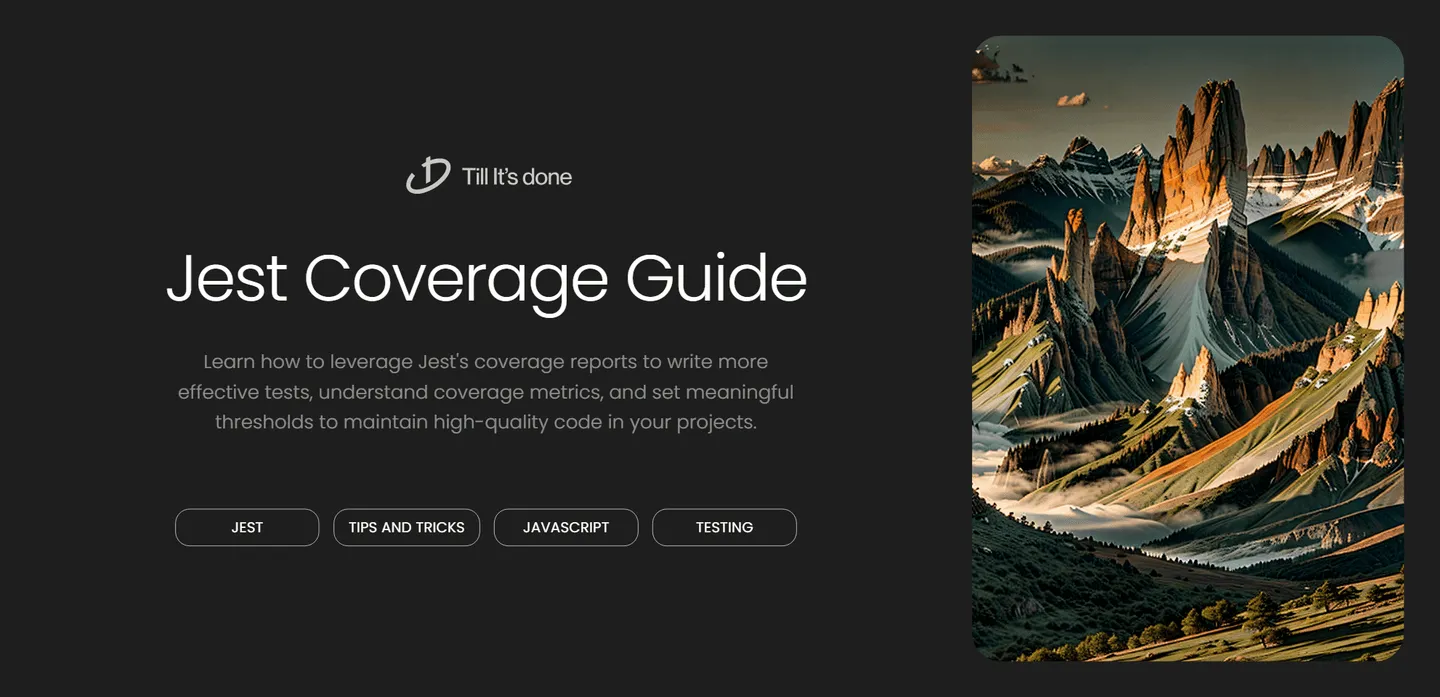
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.