- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Error Handling and Debugging in Next.js
Learn to build more resilient applications.

Error Handling and Debugging in Next.js: A Developer’s Guide to Smooth Problem Solving
As developers, we’ve all been there – staring at a blank screen or a cryptic error message, wondering where things went wrong in our Next.js application. Let’s dive into practical approaches for handling errors and debugging effectively in Next.js, making your development journey smoother and more productive.
Understanding Next.js Error Types
Next.js applications can encounter various types of errors. Let’s break them down into manageable categories:
Build-time Errors
These occur during the compilation process. They’re actually your first line of defense, catching issues before they reach production. Build errors typically relate to:
- Invalid import statements
- Syntax errors
- Configuration mistakes
- Missing dependencies
Runtime Errors
These are the trickier ones that pop up while your application is running. Common culprits include:
- Data fetching failures
- Undefined variables
- Component rendering issues
- API route problems
Implementing Error Boundaries
Error boundaries are your safety net in React and Next.js applications. They catch JavaScript errors anywhere in their child component tree and log these errors while displaying a fallback UI.
class ErrorBoundary extends React.Component { constructor(props) { super(props) this.state = { hasError: false } }
static getDerivedStateFromError(error) { return { hasError: true } }
componentDidCatch(error, errorInfo) { console.error('Error caught by boundary:', error, errorInfo) }
render() { if (this.state.hasError) { return <h1>Something went wrong. Please try again later.</h1> }
return this.props.children }}
Advanced Debugging Techniques
Custom Error Pages
Next.js allows you to create custom error pages that match your application’s design. Create pages/404.js
or pages/500.js
to handle specific error scenarios gracefully.
Server-Side Error Handling
When working with API routes or server-side code, proper error handling becomes crucial:
export async function getServerSideProps() { try { const data = await fetchData() return { props: { data } } } catch (error) { console.error('Server side error:', error) return { props: { error: { message: 'Failed to load data', status: error.status || 500 } } } }}
Debugging Tools and Tips
-
Chrome DevTools
- Use the Network tab to monitor API calls
- Leverage the Console for logging
- Set breakpoints in the Sources panel
-
Environment Variables
- Double-check your
.env
files - Verify environment variable naming (NEXT_PUBLIC_*)
- Use different environments for development and production
- Double-check your
-
Logging Strategies
- Implement structured logging
- Use debug statements strategically
- Consider logging services for production
Best Practices for Error Prevention
-
Type Checking
- Implement TypeScript for better type safety
- Use PropTypes for component props validation
- Add input validation for forms and API endpoints
-
Testing
- Write unit tests for critical components
- Implement integration tests for key user flows
- Use end-to-end testing for complex scenarios
-
Code Organization
- Keep components small and focused
- Implement proper error handling at all levels
- Use try-catch blocks judiciously
Remember, good error handling isn’t just about catching errors – it’s about providing a smooth user experience even when things go wrong. By implementing these strategies, you’ll create more resilient Next.js applications that can handle unexpected situations gracefully.




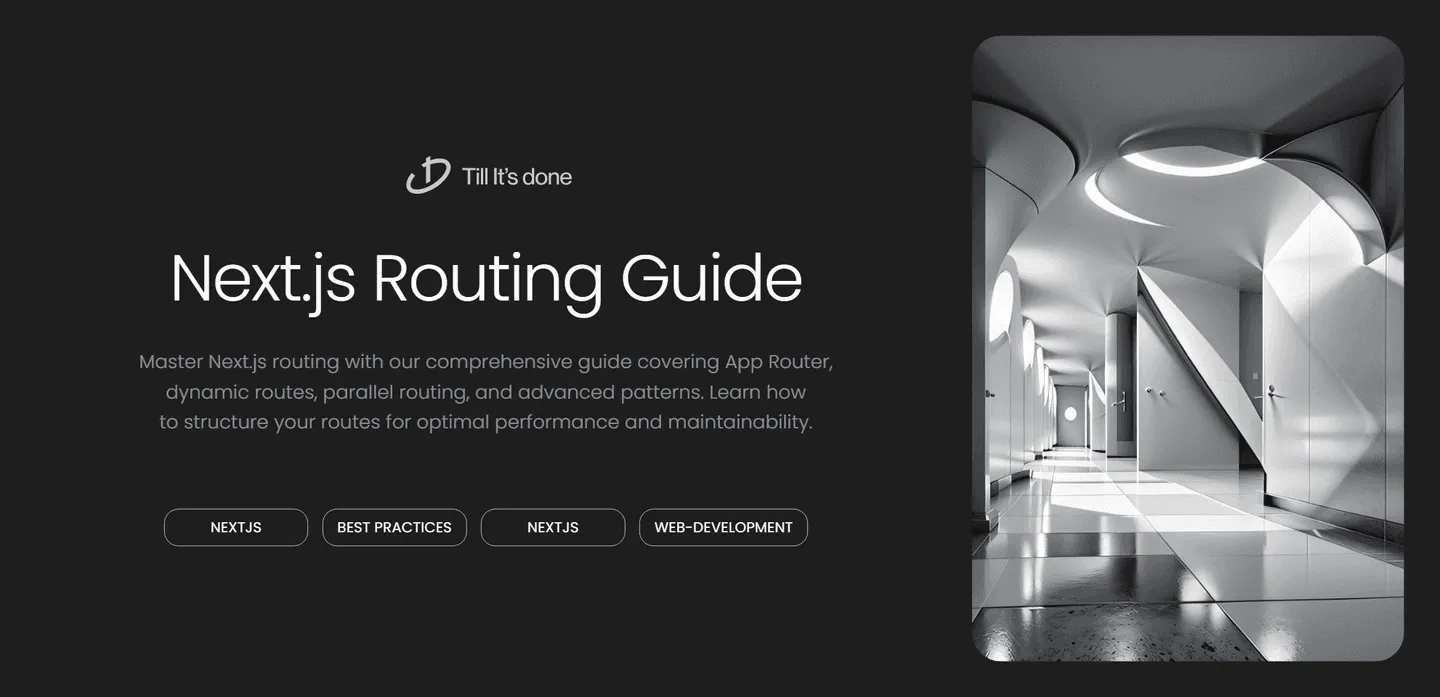

Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.