- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Top 10 Next.js Development Tips for Beginners
Perfect for developers starting their Next.js journey.
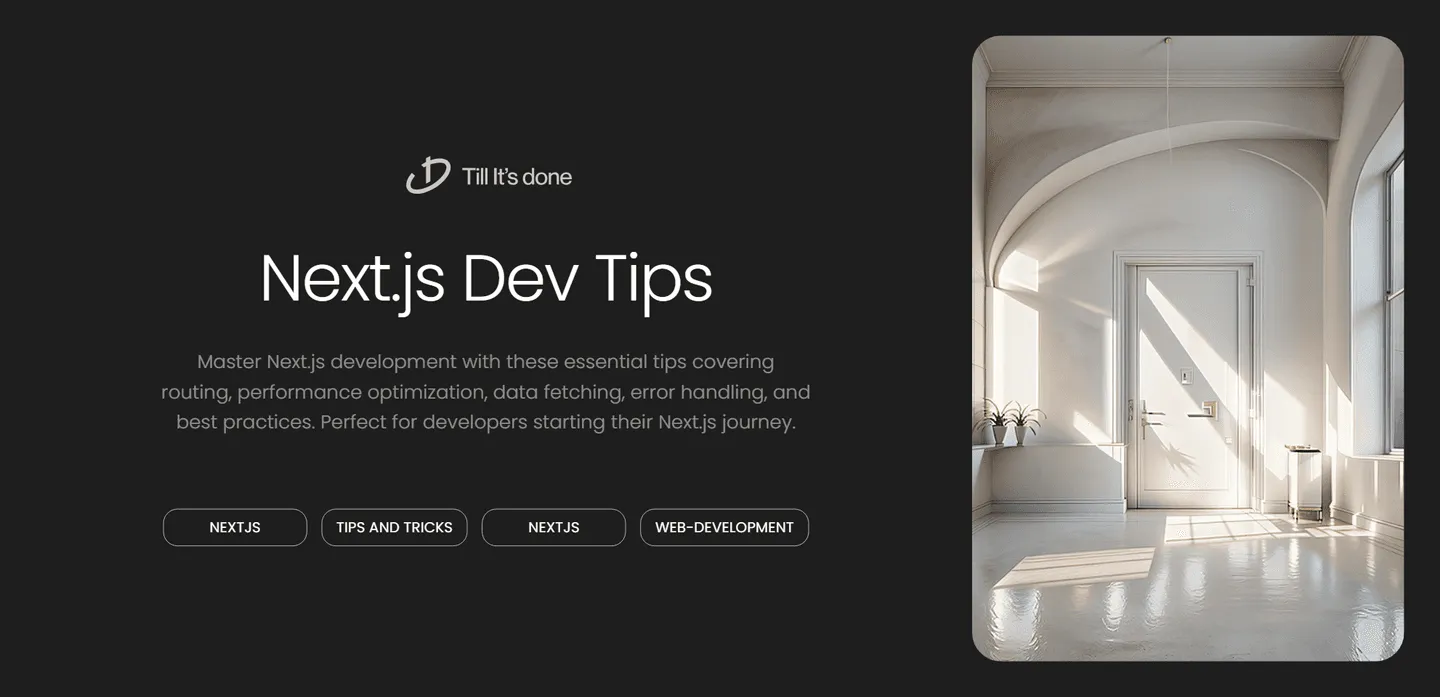
Getting started with Next.js can feel overwhelming, but don’t worry! I’ve compiled my top 10 beginner-friendly tips that will help you build better Next.js applications right from the start. These insights come from my personal experience and common pitfalls I’ve seen developers encounter.
1. Master the File-System Based Routing
One of Next.js’s most powerful features is its intuitive routing system. Instead of configuring routes manually, simply create files in your pages
directory, and Next.js automatically sets up the corresponding routes. For example, creating pages/about.js
instantly gives you an /about
route.
Pro tip: Keep your file names lowercase and use hyphens for multi-word routes (like blog-post.js
instead of blogPost.js
) to maintain clean, SEO-friendly URLs.
2. Embrace Static Site Generation (SSG)
Next.js shines when it comes to performance optimization. Whenever possible, use getStaticProps
to pre-render pages at build time. This approach significantly improves loading speeds and SEO performance. Your users will thank you for the lightning-fast page loads!
3. Implement Smart Image Optimization
Always use the built-in Image
component from next/image
instead of regular HTML <img>
tags. This component automatically handles image optimization, lazy loading, and responsive sizing. Your images will load faster and look crisp on all devices.
4. Utilize the App Directory Structure
The new app directory structure is a game-changer for organizing your code. It promotes better component organization and enables powerful features like server components. Structure your components logically within the app directory to maintain a clean and scalable codebase.
5. Leverage API Routes Effectively
Next.js API routes are perfect for building backend functionality right within your frontend application. Create API endpoints by adding files to the pages/api
directory. Remember to keep your API routes focused and modular.
6. Master Data Fetching Methods
Understanding when to use getStaticProps
, getServerSideProps
, or client-side fetching is crucial. Generally:
- Use
getStaticProps
for content that rarely changes - Use
getServerSideProps
when you need real-time data - Use client-side fetching for user-specific or frequently changing data
7. Implement Proper Error Handling
Create custom error pages by adding 404.js
and 500.js
files in your pages directory. This gives users a better experience when things go wrong. Also, implement try-catch blocks in your data fetching functions to handle errors gracefully.
8. Optimize Performance with Code Splitting
Next.js automatically code-splits your application, but you can enhance this further by using dynamic imports. Use next/dynamic
for components that aren’t immediately needed on page load, especially for heavy components like charts or complex forms.
9. Use Environment Variables Wisely
Keep your sensitive data secure by utilizing environment variables. Create a .env.local
file for local development and use proper naming conventions:
NEXT_PUBLIC_
prefix for client-side variables- Regular names for server-side variables
10. Implement Progressive Enhancement
Build your applications with progressive enhancement in mind. Start with a solid, functional base that works without JavaScript, then enhance the experience with interactive features. This ensures your app remains accessible and functional for all users.
These tips should give you a solid foundation for building robust Next.js applications. Remember, the best way to learn is by doing – start implementing these practices in your projects, and you’ll see immediate improvements in your development workflow and application quality.
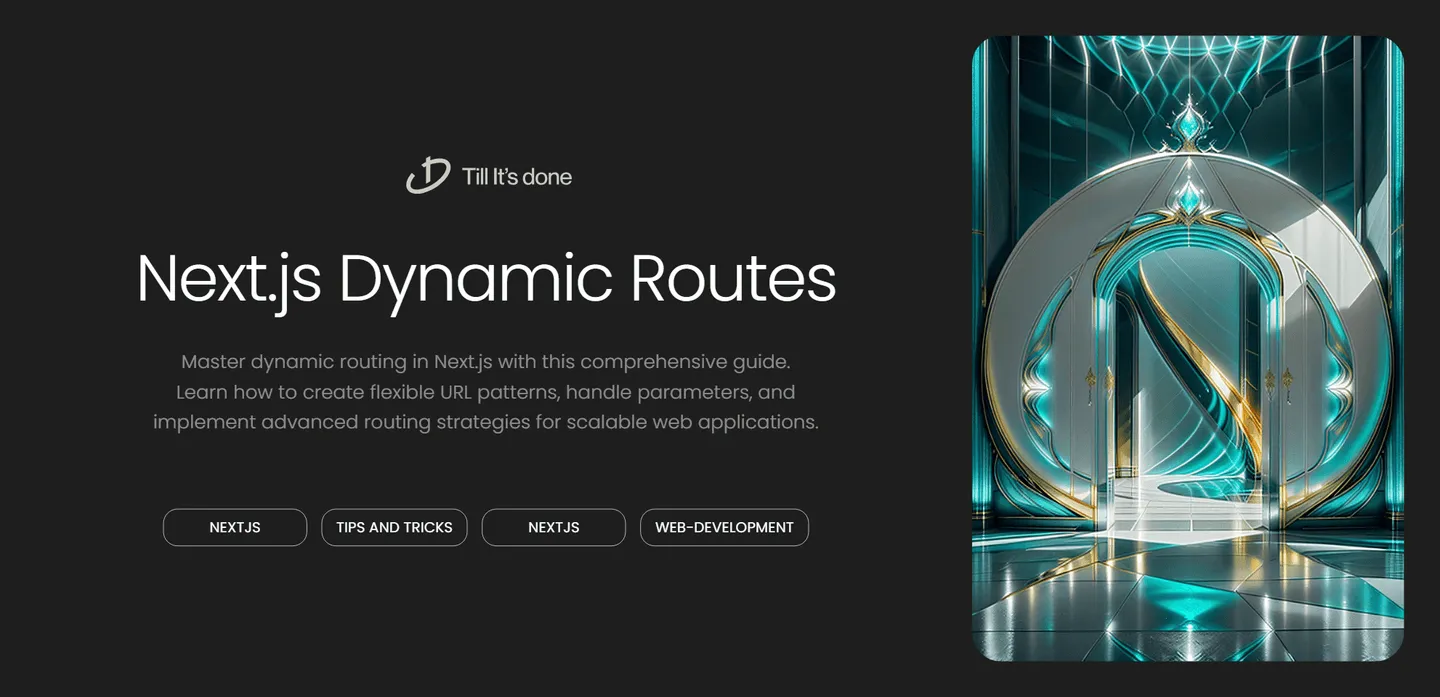




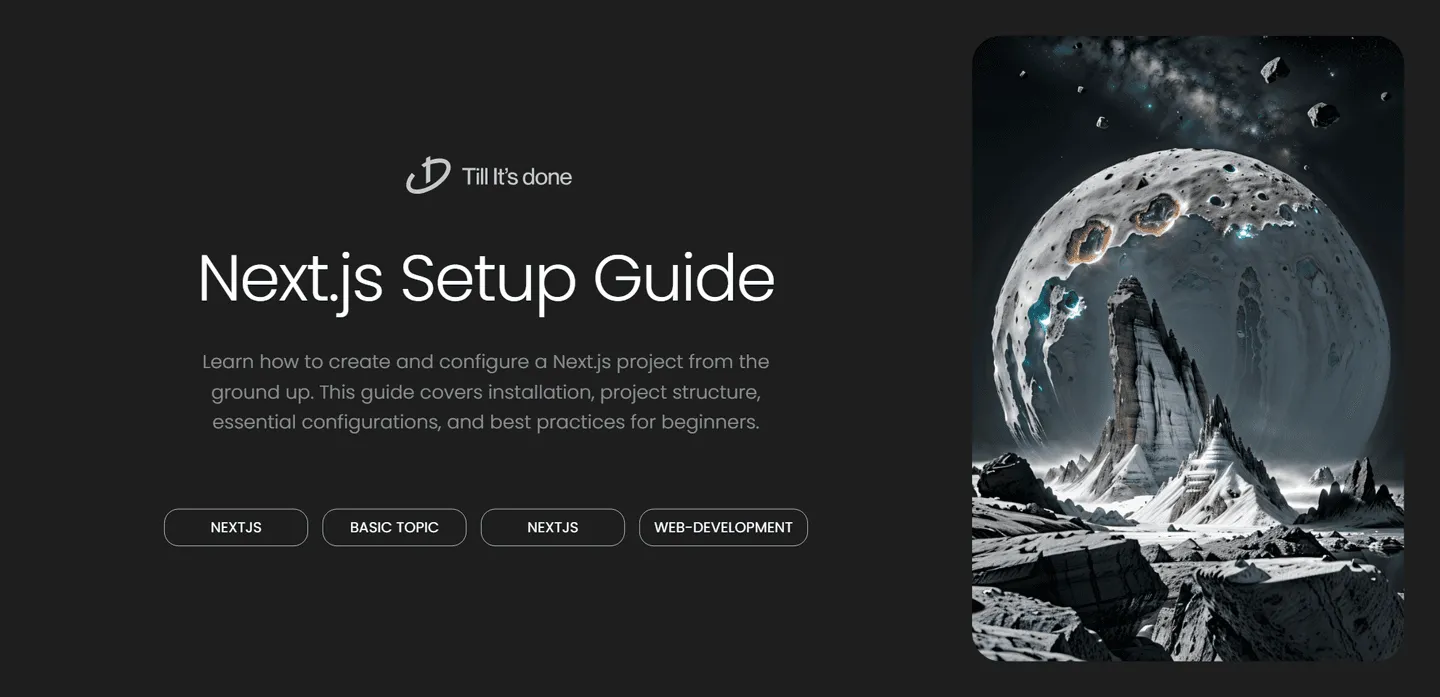
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.