- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Implementing Authentication in Next.js Guide
Learn about NextAuth.js, middleware protection, and industry best practices for user authentication.

Implementing Authentication in Next.js: A Comprehensive Guide
Authentication is the cornerstone of modern web applications, serving as the gateway between your users and their personalized experiences. In this guide, we’ll dive deep into implementing robust authentication in Next.js applications using industry best practices.
Understanding Authentication in Next.js
The web has evolved significantly, and with it, our approaches to authentication. Next.js provides several powerful features that make implementing secure authentication more straightforward than ever. Before we dive into the implementation details, let’s understand the key concepts that make Next.js authentication unique.
Authentication Approaches in Next.js
1. Built-in Authentication Solutions
Next.js works seamlessly with various authentication providers. The most popular approaches include:
- NextAuth.js: A complete authentication solution
- Custom JWT implementation
- OAuth providers integration
- Magic links authentication
2. Server-side vs. Client-side Authentication
Next.js’s hybrid nature allows for both server-side and client-side authentication strategies. Here’s how to choose between them:
Server-side authentication benefits:
- Enhanced security
- Better performance
- SEO-friendly
- Reduced client-side JavaScript
Client-side authentication benefits:
- Rich user experience
- Real-time feedback
- Flexible state management
- Easier integration with SPAs
Implementing Authentication Step by Step
Let’s walk through implementing authentication using NextAuth.js, one of the most popular solutions:
- First, install the required dependencies:
npm install next-auth
- Create an API route for authentication:
import NextAuth from 'next-auth'import Providers from 'next-auth/providers'
export default NextAuth({ providers: [ Providers.GitHub({ clientId: process.env.GITHUB_ID, clientSecret: process.env.GITHUB_SECRET, }), // Add more providers as needed ], session: { jwt: true, }, callbacks: { async session(session, user) { return session }, async jwt(token, user, account) { return token }, },})
- Wrap your application with the authentication provider:
import { SessionProvider } from 'next-auth/react'
function MyApp({ Component, pageProps: { session, ...pageProps } }) { return ( <SessionProvider session={session}> <Component {...pageProps} /> </SessionProvider> )}
export default MyApp
Best Practices and Security Considerations
- Environment Variables: Always store sensitive information in environment variables:
NEXTAUTH_URL=http://localhost:3000NEXTAUTH_SECRET=your-secret-key
- Protected Routes: Implement middleware to protect sensitive routes:
export async function middleware(req) { const session = await getSession({ req }) if (!session) { return NextResponse.redirect('/auth/signin') } return NextResponse.next()}
- Error Handling: Implement proper error handling and user feedback:
try { await signIn('credentials', { redirect: false, email, password, })} catch (error) { console.error('Authentication error:', error) // Handle error appropriately}
Advanced Authentication Features
- Implement password recovery flows
- Add multi-factor authentication
- Set up role-based access control
- Configure session management
- Implement OAuth 2.0 flows
Testing Authentication
Always test your authentication implementation thoroughly:
describe('Authentication Flow', () => { it('should successfully log in a user', async () => { // Test implementation })
it('should handle invalid credentials', async () => { // Test implementation })})
Conclusion
Implementing authentication in Next.js doesn’t have to be complicated. By following these best practices and utilizing the right tools, you can create a secure and user-friendly authentication system that scales with your application.
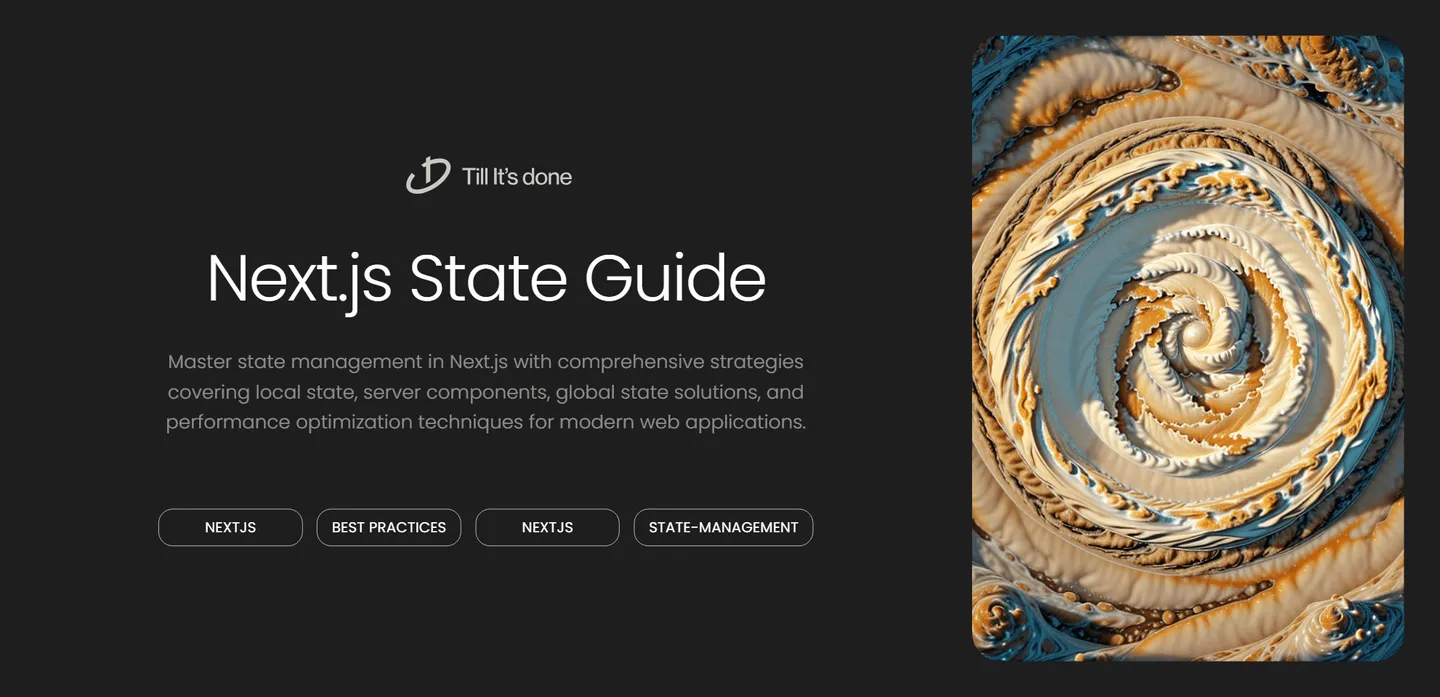
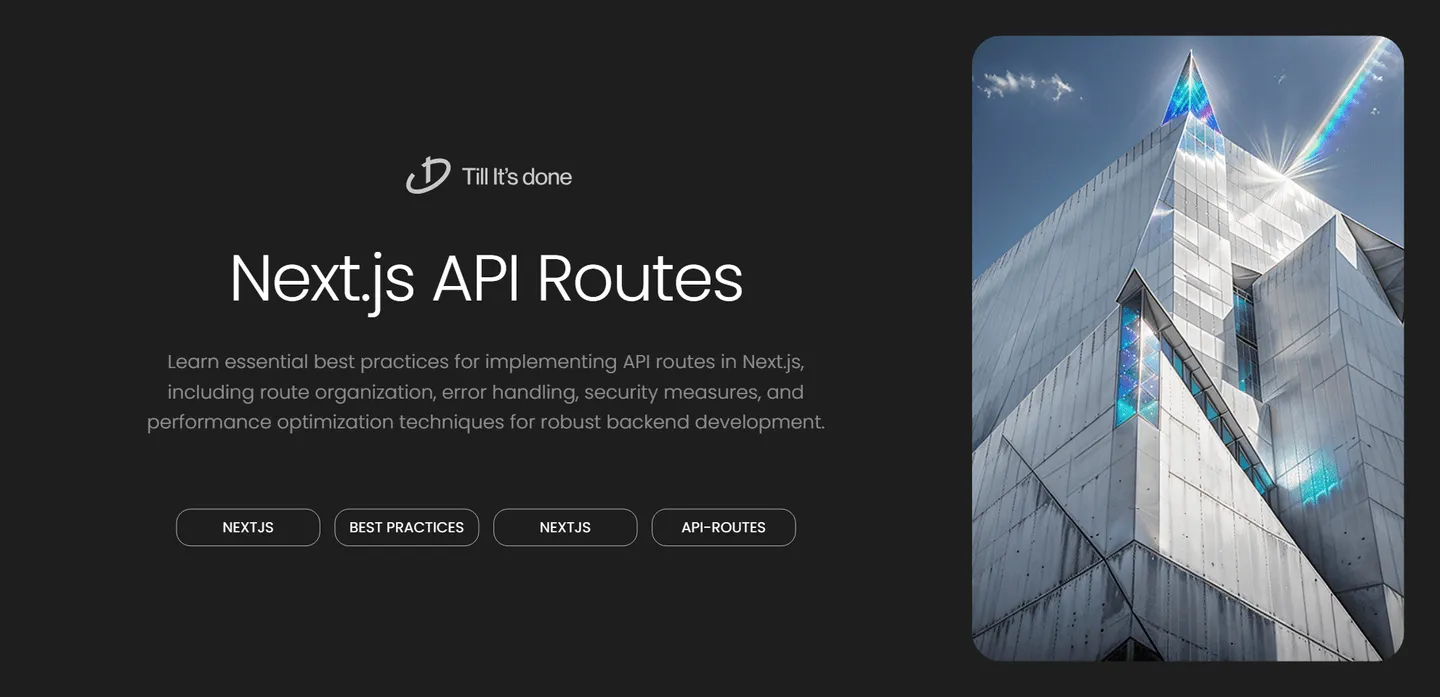

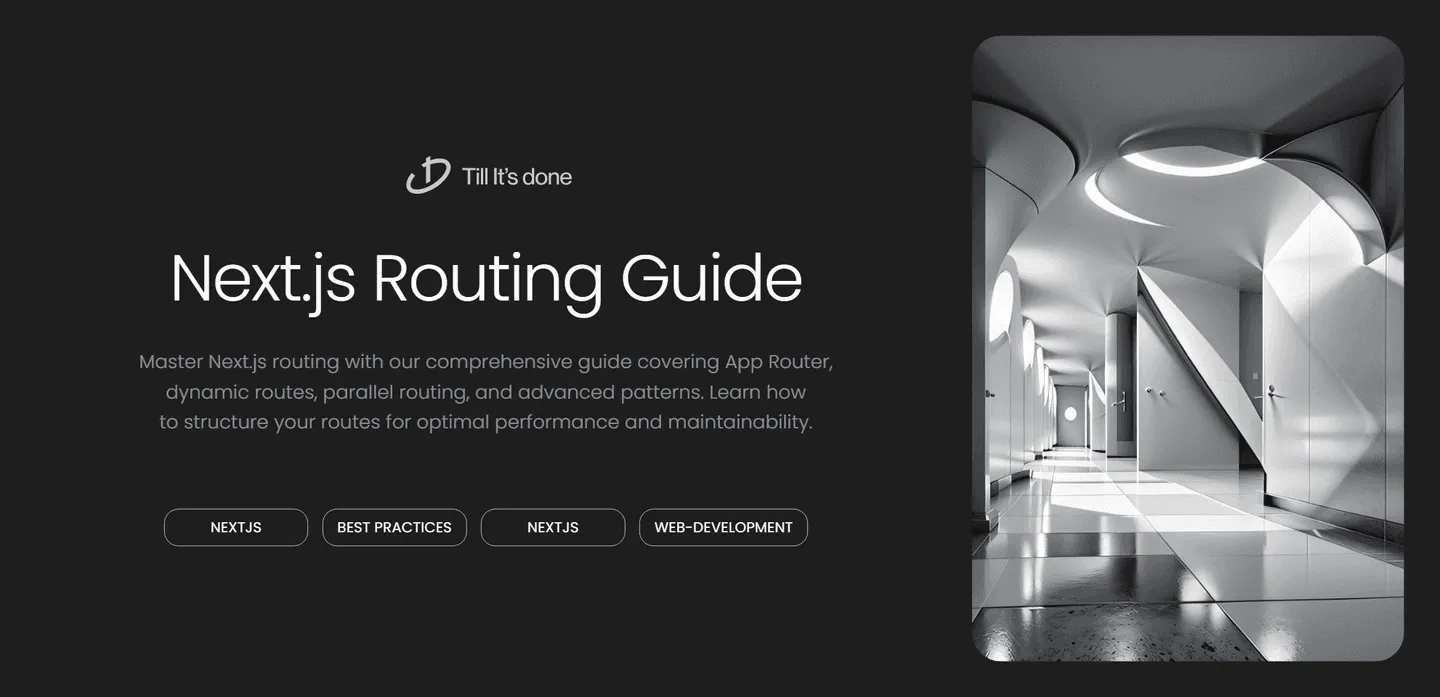


Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.