- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Master Time in Node.js with Moment.js Guide
Learn date manipulation, formatting, and best practices for modern web development in this comprehensive guide.
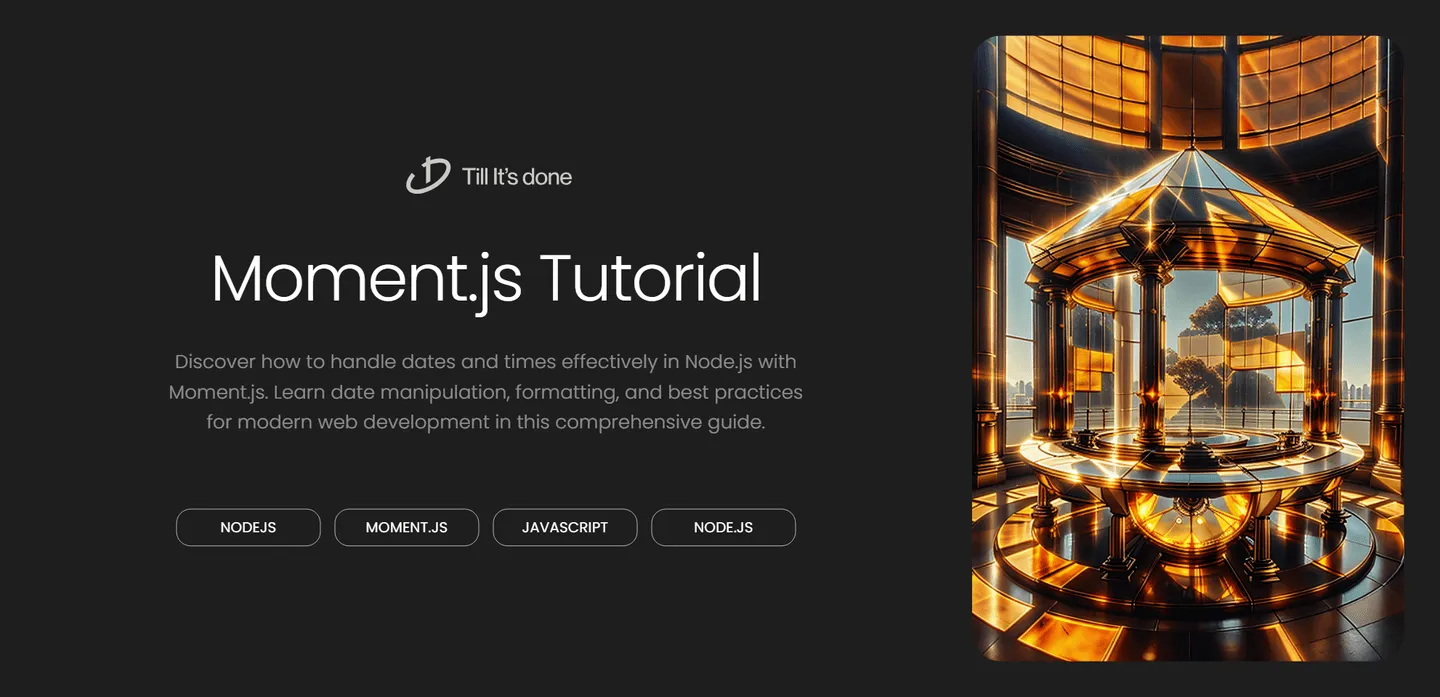
Introduction to Moment.js: A Comprehensive Guide for Node.js Developers
Working with dates and times in JavaScript can be challenging. Enter Moment.js – a powerful library that makes date manipulation and formatting a breeze for Node.js developers. This guide will walk you through everything you need to know to master time handling in your applications.
Getting Started with Moment.js
First things first, let’s set up Moment.js in your Node.js project. Install it using npm:
npm install moment
Then import it into your project:
const moment = require('moment');
Core Concepts and Basic Usage
Moment.js works by creating a wrapper around the date object, providing a cleaner interface for manipulation. Here’s how you can create a moment object:
// Current date and timeconst now = moment();
// Create from stringconst date = moment("2024-03-23");
// Create from formatconst customDate = moment("23-03-2024", "DD-MM-YYYY");
Date Formatting and Display
One of Moment.js’s strongest features is its formatting capabilities:
const date = moment();
console.log(date.format('MMMM Do YYYY')); // March 23rd 2024console.log(date.format('DD/MM/YYYY')); // 23/03/2024console.log(date.fromNow()); // a few seconds ago
Date Manipulation and Calculations
Moment.js shines when it comes to date manipulation:
const future = moment().add(7, 'days');const past = moment().subtract(1, 'month');
// Check if a date is before or after anotherconsole.log(future.isAfter(past)); // true
// Calculate durationconst duration = moment.duration(future.diff(past));console.log(duration.asDays()); // Number of days between dates
Best Practices and Performance Tips
- Chain methods for cleaner code:
const nextWeek = moment().add(1, 'week').format('DD/MM/YYYY');
- Use specific imports for better performance:
const moment = require('moment/moment');
- Consider timezone handling:
moment().tz('America/New_York').format();
Common Pitfalls to Avoid
Remember that Moment.js objects are mutable. To avoid unexpected behaviors:
// Clone moments when you need a separate instanceconst original = moment();const copy = original.clone();
Conclusion
Moment.js continues to be a reliable choice for date and time manipulation in Node.js applications. While newer alternatives exist, its mature ecosystem and extensive documentation make it a solid option for developers who need robust date handling capabilities.
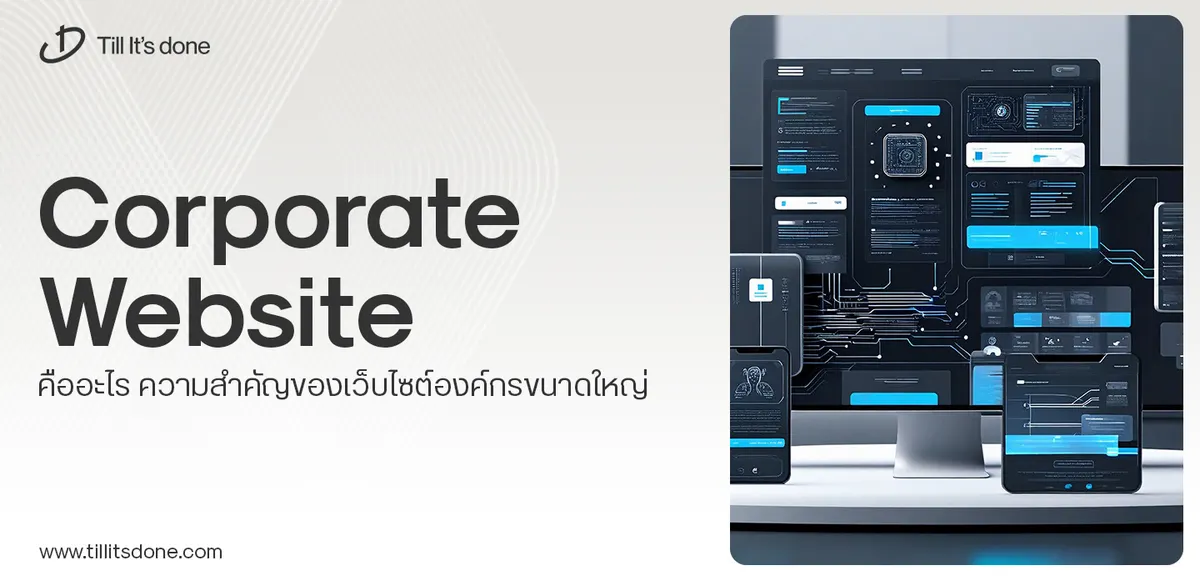
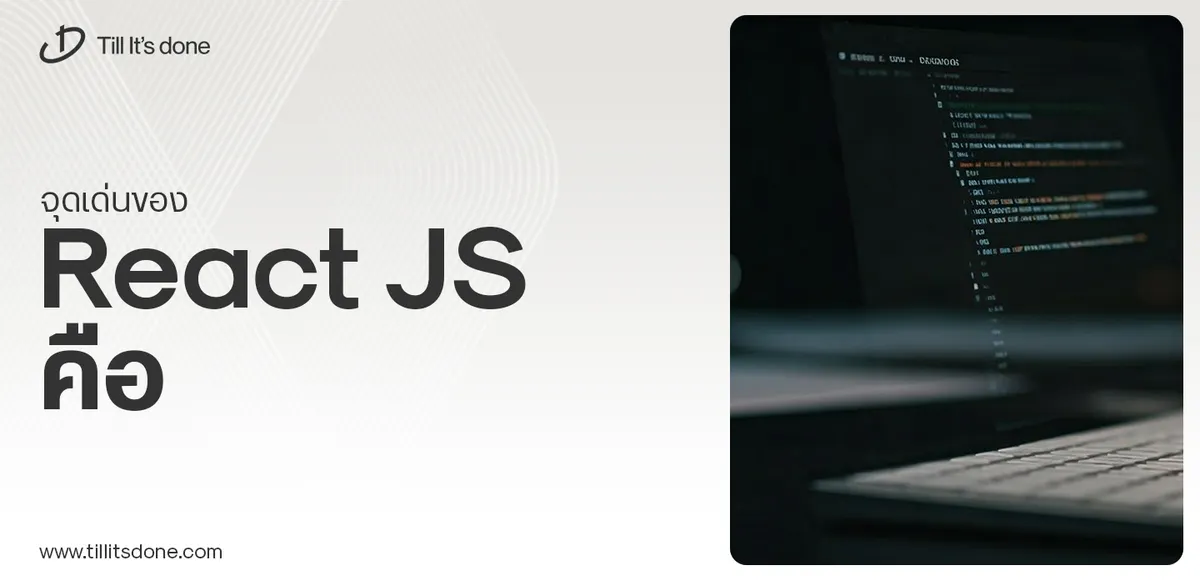
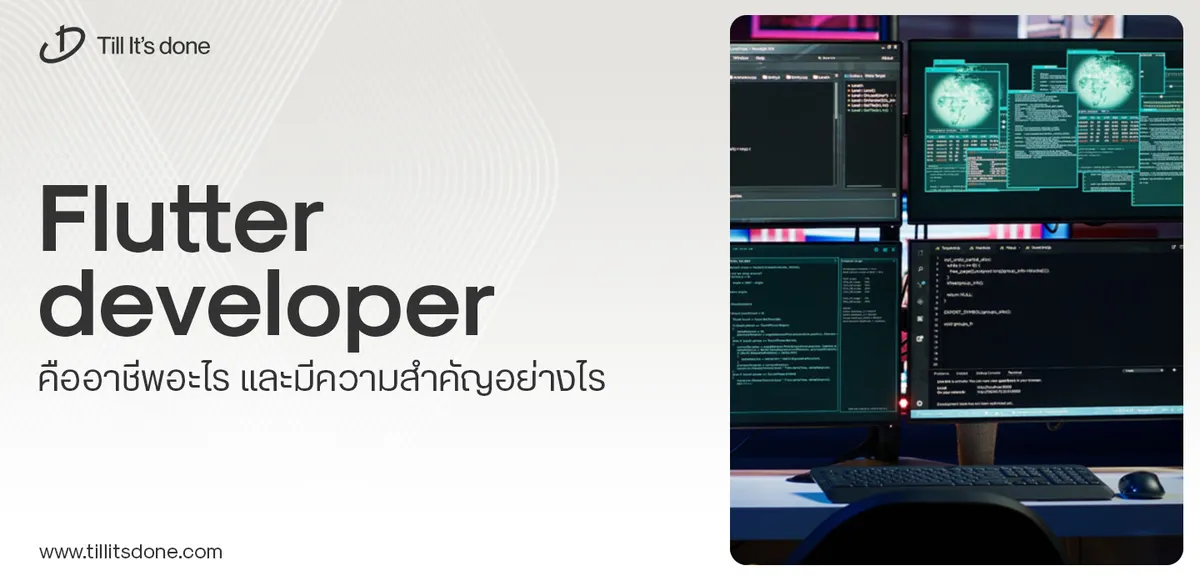
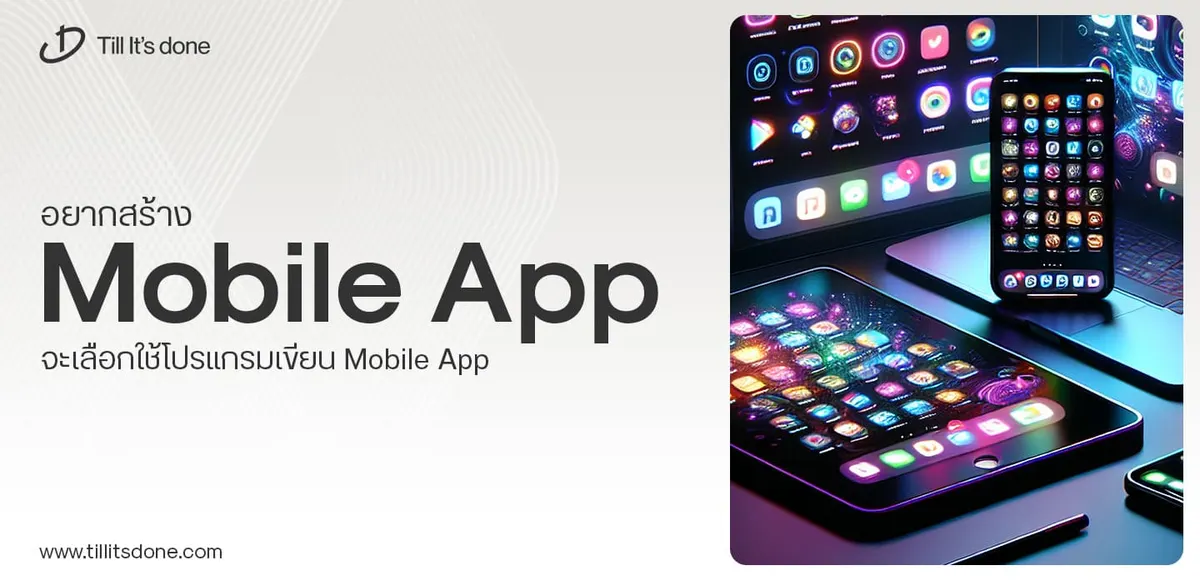
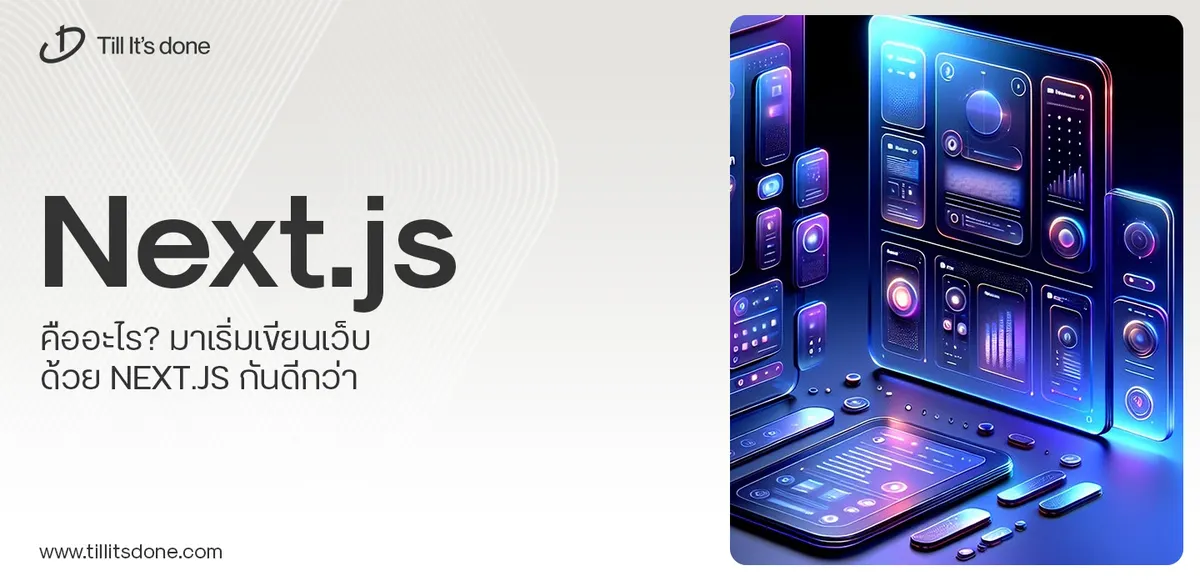
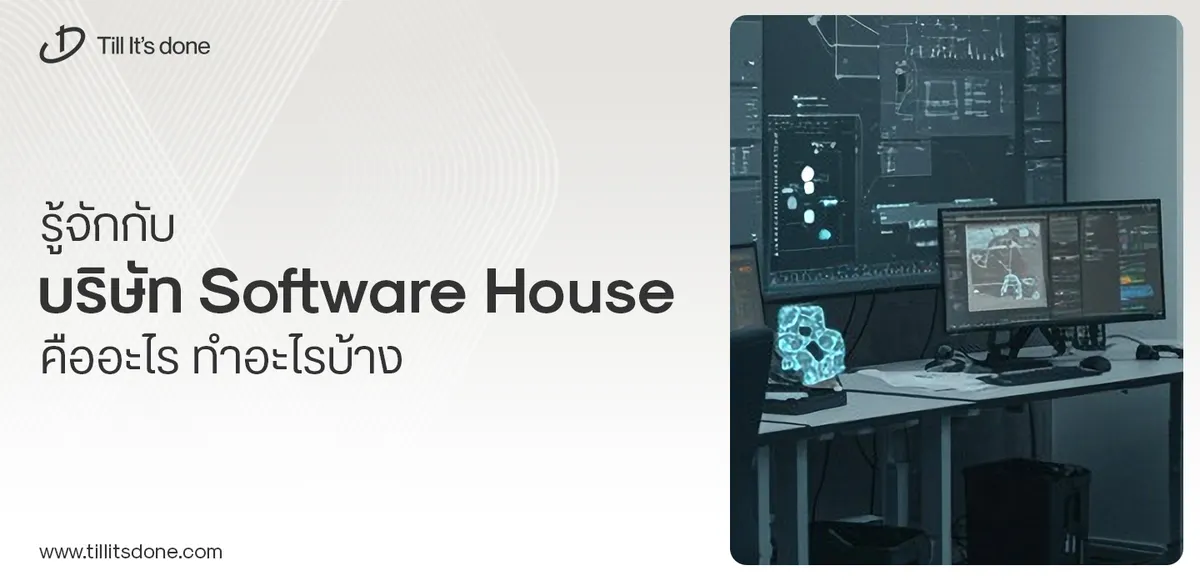
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.