- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Mock Dio Requests for Flutter Unit Testing
Discover best practices, code examples, and practical implementation techniques for testing network calls.

Testing Network Requests in Flutter: A Guide to Mocking Dio
In the world of Flutter development, handling and testing network requests is a crucial skill. While Dio is an excellent HTTP client for making API calls, testing these network interactions can be challenging. Today, let’s explore how to effectively mock Dio requests for unit testing in Flutter.
Understanding the Importance of Mocking
When writing unit tests, we want them to be fast, reliable, and independent of external services. Making actual network calls during tests can lead to flaky tests and slower execution times. This is where mocking comes in handy.
Setting Up Your Testing Environment
First, let’s set up our testing environment with the necessary dependencies. Add these to your pubspec.yaml
:
dev_dependencies: mockito: ^5.4.0 http_mock_adapter: ^0.4.4
Creating Mock Responses
Let’s look at a practical example of how to mock Dio requests. We’ll create a simple user service and test it:
class UserService { final Dio dio;
UserService(this.dio);
Future<User> getUser(int id) async { final response = await dio.get('/users/$id'); return User.fromJson(response.data); }}
Here’s how we can test this service:
void main() { late Dio dio; late UserService userService; late DioAdapter dioAdapter;
setUp(() { dio = Dio(); dioAdapter = DioAdapter(dio: dio); dio.httpClientAdapter = dioAdapter; userService = UserService(dio); });
test('should return user when API call is successful', () async { final mockData = { 'id': 1, 'name': 'John Doe', 'email': 'john@example.com' };
dioAdapter.onGet( '/users/1', (server) => server.reply(200, mockData), );
final user = await userService.getUser(1);
expect(user.name, equals('John Doe')); expect(user.email, equals('john@example.com')); });}
Best Practices for Mocking Dio
- Always mock error scenarios
- Test different HTTP methods
- Verify request headers and parameters
- Test timeout scenarios
- Mock interceptors when needed
Here’s an example of testing an error scenario:
test('should throw exception when API call fails', () async { dioAdapter.onGet( '/users/1', (server) => server.throws( 404, DioError( requestOptions: RequestOptions(path: '/users/1'), response: Response( statusCode: 404, requestOptions: RequestOptions(path: '/users/1'), ), ), ), );
expect( () => userService.getUser(1), throwsA(isA<DioError>()), );});
Conclusion
Mocking Dio requests is essential for writing reliable unit tests in Flutter. By following these patterns and best practices, you can ensure your network-related code is thoroughly tested and maintainable.
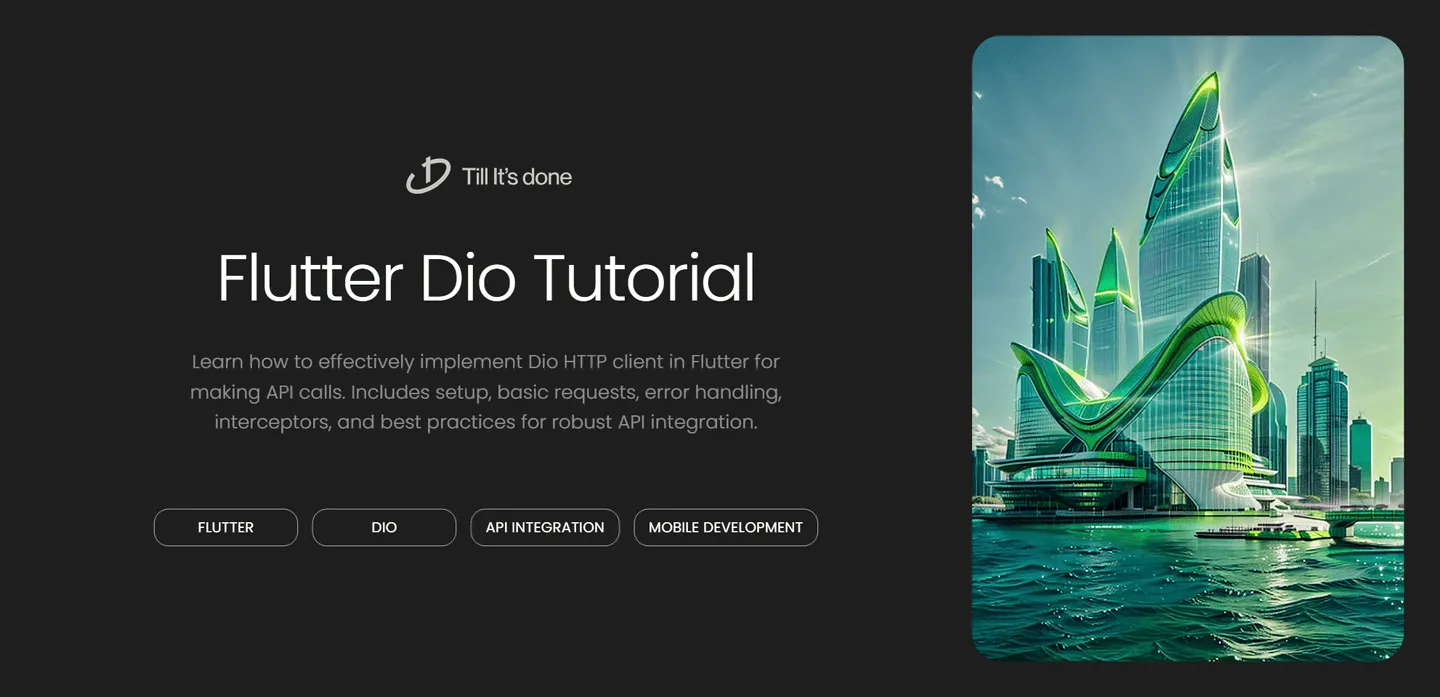




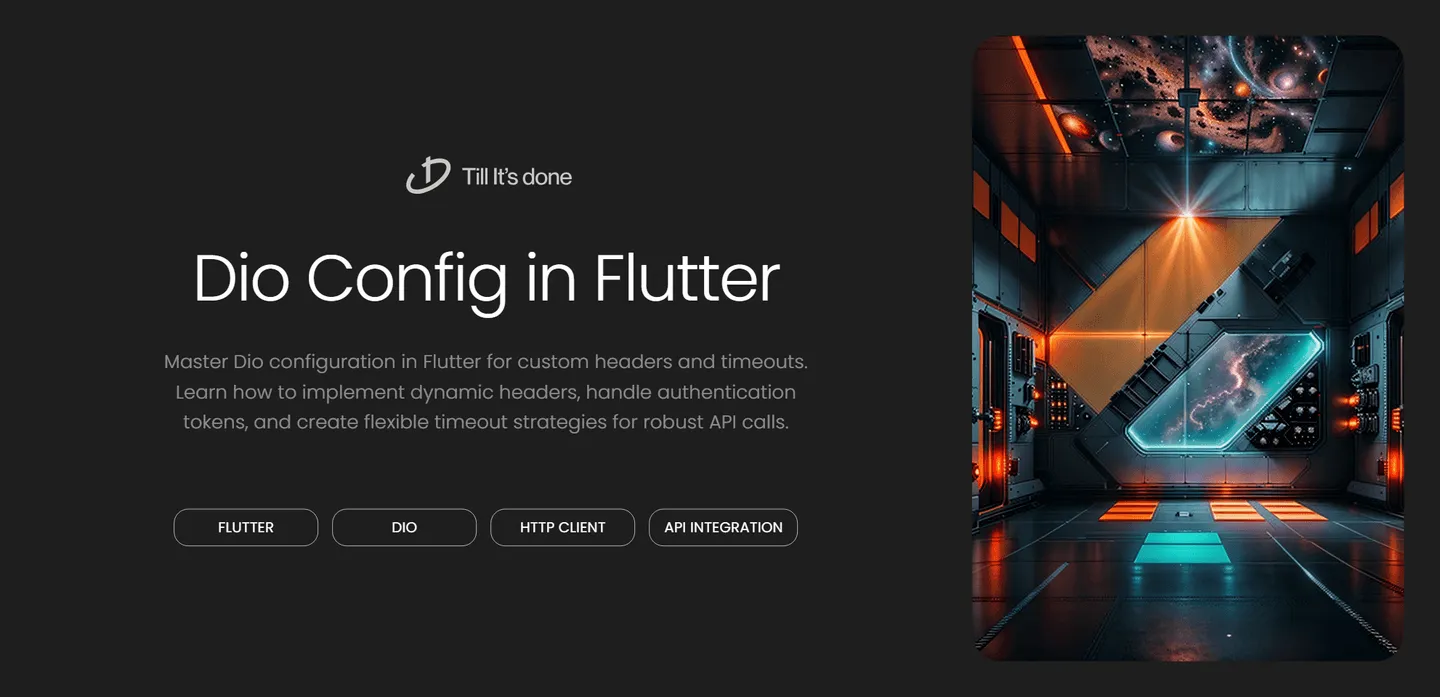
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.