- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
How to Integrate Dio for API Calls in Flutter
Includes setup, basic requests, error handling, interceptors, and best practices for robust API integration.
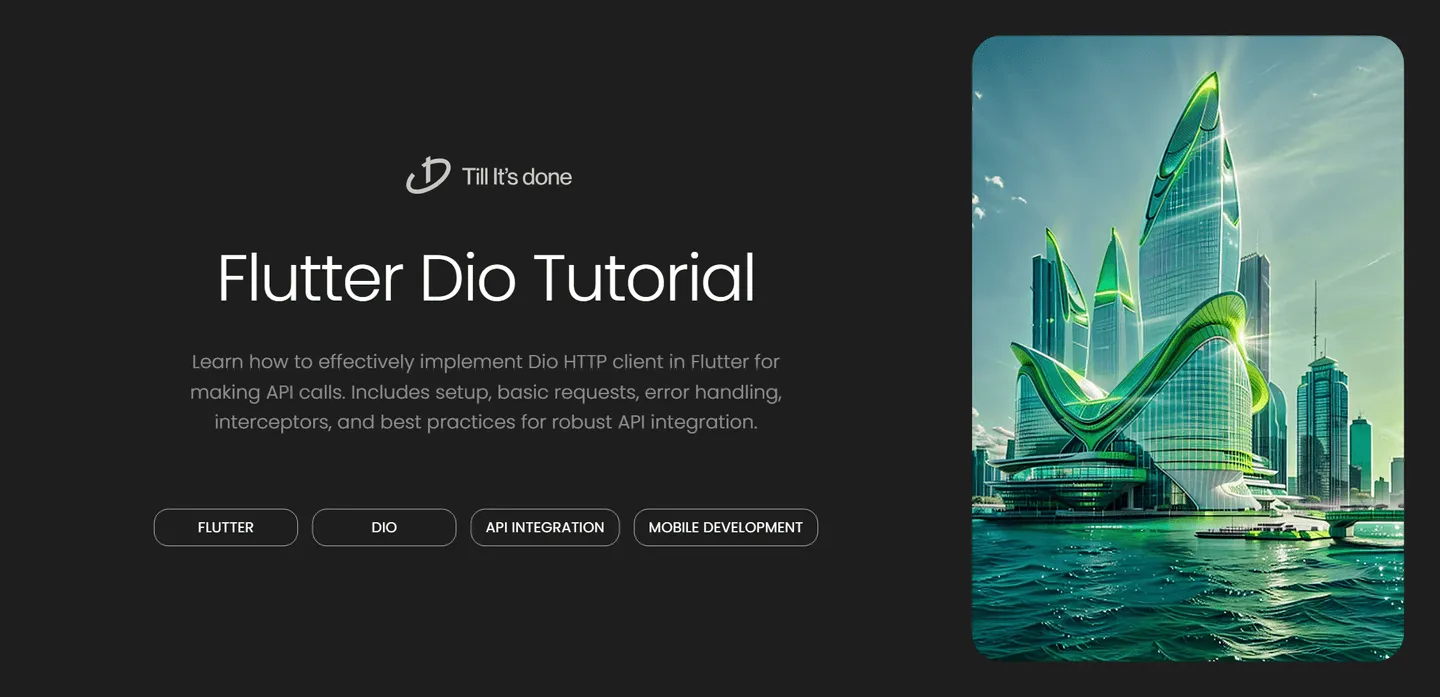
How to integrate Dio for API calls in Flutter
In today’s interconnected world, almost every mobile application needs to communicate with web servers. When building Flutter applications, Dio stands out as a powerful and flexible HTTP client that makes API integration a breeze. Let’s dive into how we can effectively implement Dio in our Flutter projects.
Getting Started with Dio
First things first, we need to add Dio to our project. Add the following dependency to your pubspec.yaml
file:
dependencies: dio: ^5.3.2
After adding the dependency, run:
flutter pub get
Setting Up Dio Client
Creating a well-structured Dio client is crucial for maintaining clean and maintainable code. Here’s how we can set up a basic Dio instance:
import 'package:dio/dio.dart';
class DioClient { final Dio _dio = Dio();
DioClient() { _dio.options.baseUrl = 'https://api.example.com'; _dio.options.connectTimeout = const Duration(milliseconds: 5000); _dio.options.receiveTimeout = const Duration(milliseconds: 3000);
// Add interceptors for logging _dio.interceptors.add(LogInterceptor( requestBody: true, responseBody: true, )); }}
Making API Calls
Now that we have our Dio client set up, let’s look at how to make different types of API calls:
GET Request
Future<dynamic> getUsers() async { try { final response = await _dio.get('/users'); return response.data; } catch (error) { throw handleError(error); }}
POST Request
Future<dynamic> createUser(Map<String, dynamic> userData) async { try { final response = await _dio.post('/users', data: userData); return response.data; } catch (error) { throw handleError(error); }}
Error Handling
Proper error handling is crucial for a robust application. Here’s a simple error handler:
dynamic handleError(DioError error) { switch (error.type) { case DioErrorType.connectionTimeout: return 'Connection timed out'; case DioErrorType.receiveTimeout: return 'Receive timeout in connection'; case DioErrorType.badResponse: return _handleErrorResponse(error.response?.statusCode); default: return 'Something went wrong'; }}
String _handleErrorResponse(int? statusCode) { switch (statusCode) { case 400: return 'Bad request'; case 401: return 'Unauthorized'; case 403: return 'Forbidden'; case 404: return 'Not found'; case 500: return 'Internal server error'; default: return 'Something went wrong'; }}
Using Interceptors
Interceptors are powerful tools in Dio that allow you to monitor and transform requests and responses:
class AuthInterceptor extends Interceptor { @override void onRequest(RequestOptions options, RequestInterceptorHandler handler) { options.headers['Authorization'] = 'Bearer your-token-here'; handler.next(options); }}
// Add to Dio instance_dio.interceptors.add(AuthInterceptor());
Best Practices
- Always implement proper error handling
- Use interceptors for common operations like adding headers
- Set appropriate timeouts
- Implement retry mechanisms for failed requests
- Use response type adapters for automatic JSON parsing
Remember to handle the disposal of Dio instances properly when they’re no longer needed to prevent memory leaks.
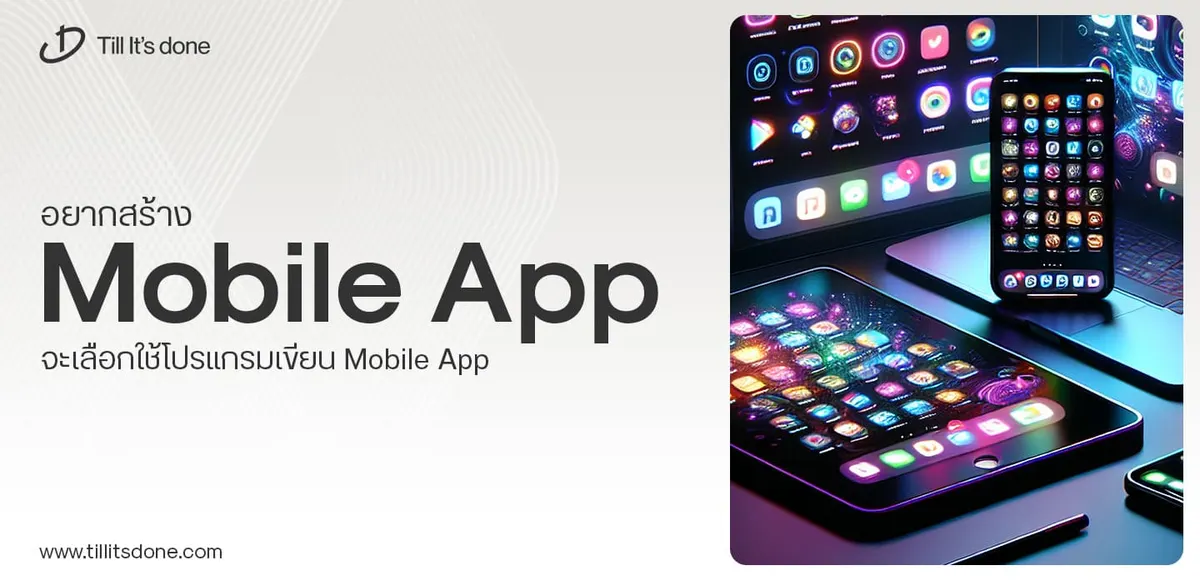
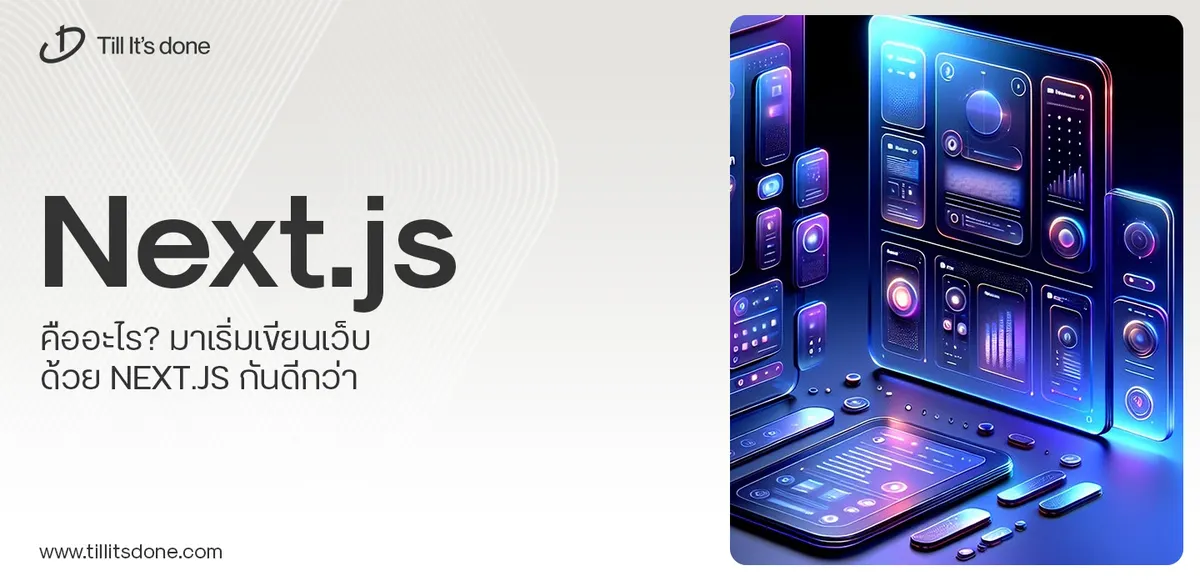
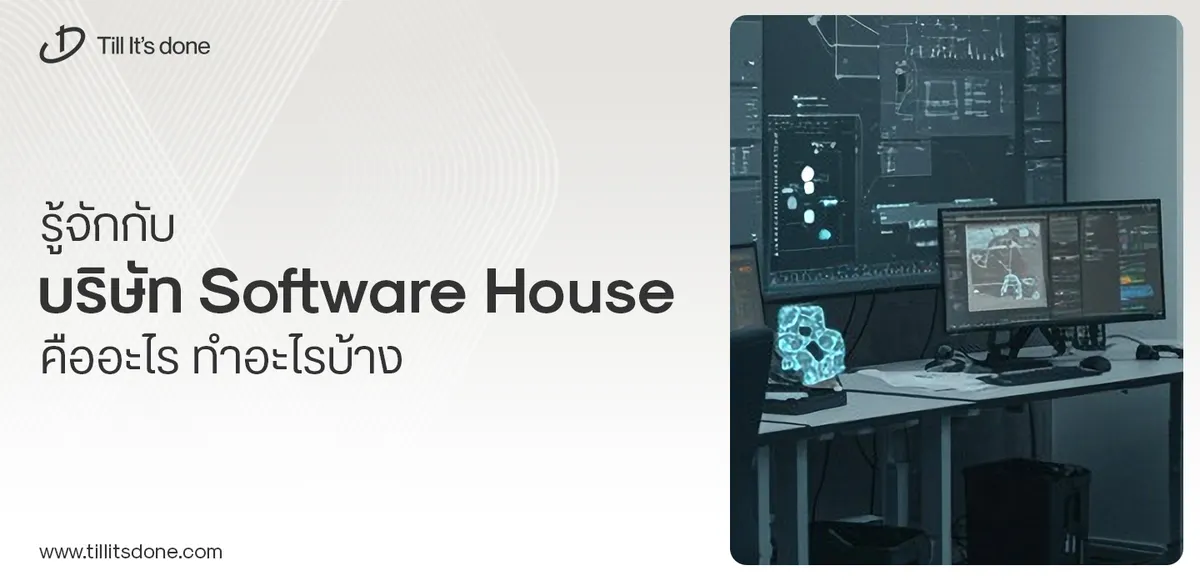
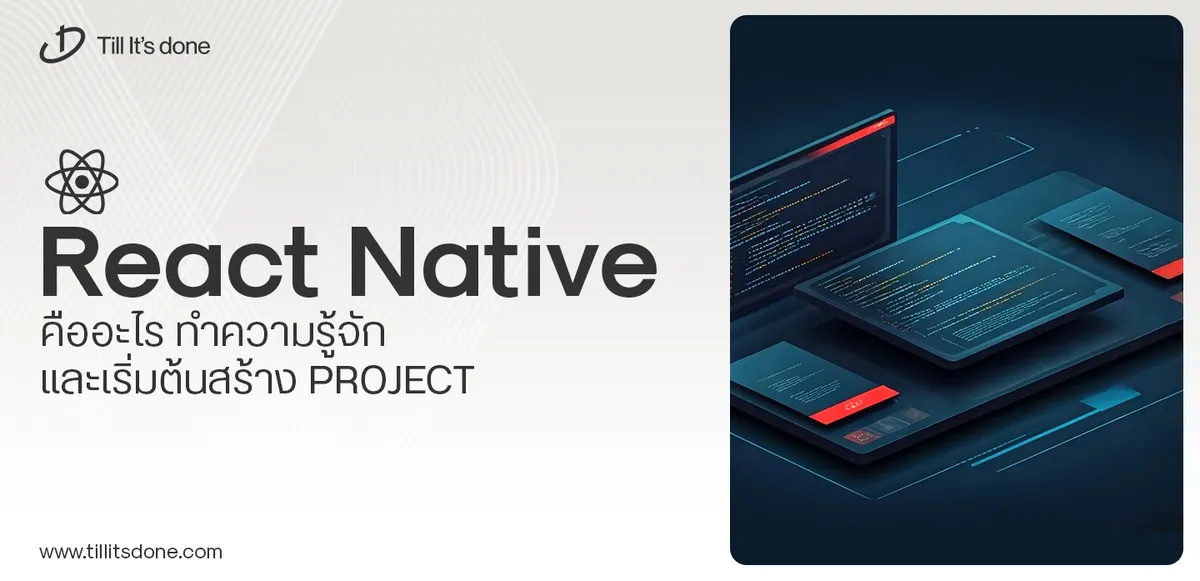
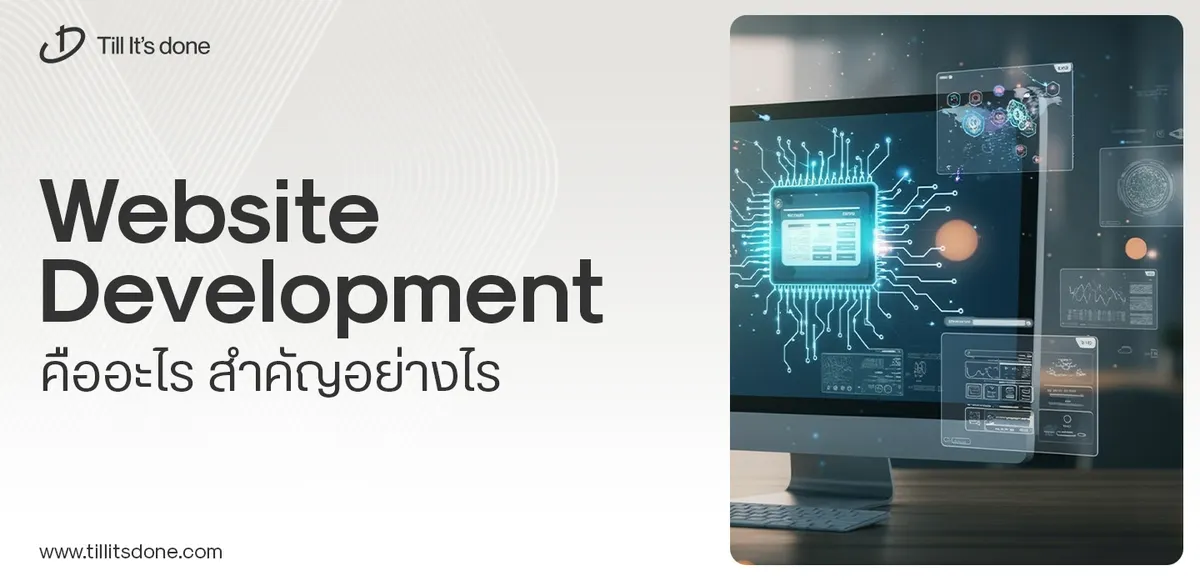
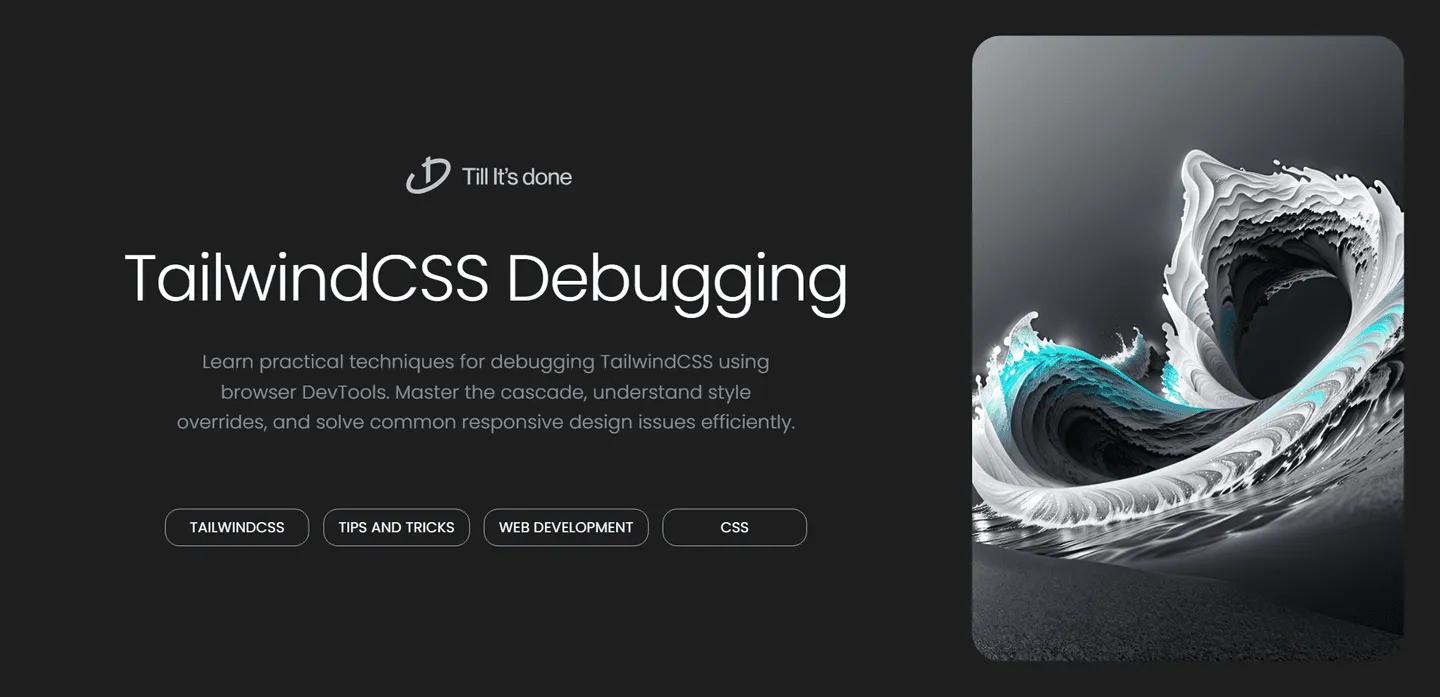
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.