- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Introduction to MobX with ReactJS
Learn about observables, actions, and reactions while mastering efficient state management patterns.

Introduction to MobX with ReactJS
State management in React applications can sometimes feel overwhelming, especially as your application grows. Enter MobX – a battle-tested library that makes state management simple and scalable. Let’s dive into how MobX can transform the way you handle state in your React applications.
What is MobX?
Think of MobX as your personal assistant that keeps all your application’s data in sync. Unlike other state management solutions that require a lot of boilerplate code, MobX takes a more straightforward approach. It uses observable patterns – a tried-and-true programming concept that ensures your UI always reflects your application’s current state.
Core Concepts of MobX
1. Observables
Observables are the heart of MobX. They’re like special containers that can hold any type of data – from simple numbers to complex objects. When these containers change, MobX automatically updates any part of your UI that depends on them.
import { makeObservable, observable } from 'mobx';
class TodoStore { todos = [];
constructor() { makeObservable(this, { todos: observable }); }}
2. Actions
Actions are how we modify our observables. Think of them as the only entrance to change our application’s state. This makes our code more predictable and easier to debug.
class TodoStore { todos = [];
constructor() { makeObservable(this, { todos: observable, addTodo: action }); }
addTodo(task) { this.todos.push({ task, completed: false }); }}
3. Reactions
Reactions are the magic that happens when our observables change. They automatically update our UI without us having to manually manage subscriptions or updates.
Integrating MobX with React
The integration between MobX and React is seamless. Here’s a simple example:
import { observer } from 'mobx-react-lite';import { makeObservable, observable, action } from 'mobx';
class CounterStore { count = 0;
constructor() { makeObservable(this, { count: observable, increment: action }); }
increment() { this.count += 1; }}
const Counter = observer(({ store }) => ( <div> <h1>Count: {store.count}</h1> <button onClick={() => store.increment()}>Increment</button> </div>));
Best Practices
- Keep your stores focused and small
- Use actions for all state modifications
- Leverage computed values for derived data
- Use the
observer
HOC only on components that directly use observable data
When to Choose MobX?
MobX shines when you:
- Need a simpler alternative to Redux
- Want less boilerplate code
- Prefer a more flexible state management solution
- Have a team familiar with object-oriented programming
The beauty of MobX lies in its simplicity and flexibility. You can start small and scale as your application grows, without being forced into a specific architecture or pattern.
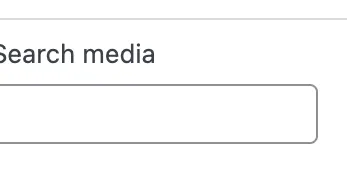





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.