- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
MobX Best Practices for React Developers
Discover tips for store organization, performance optimization, and advanced patterns to build scalable React apps.
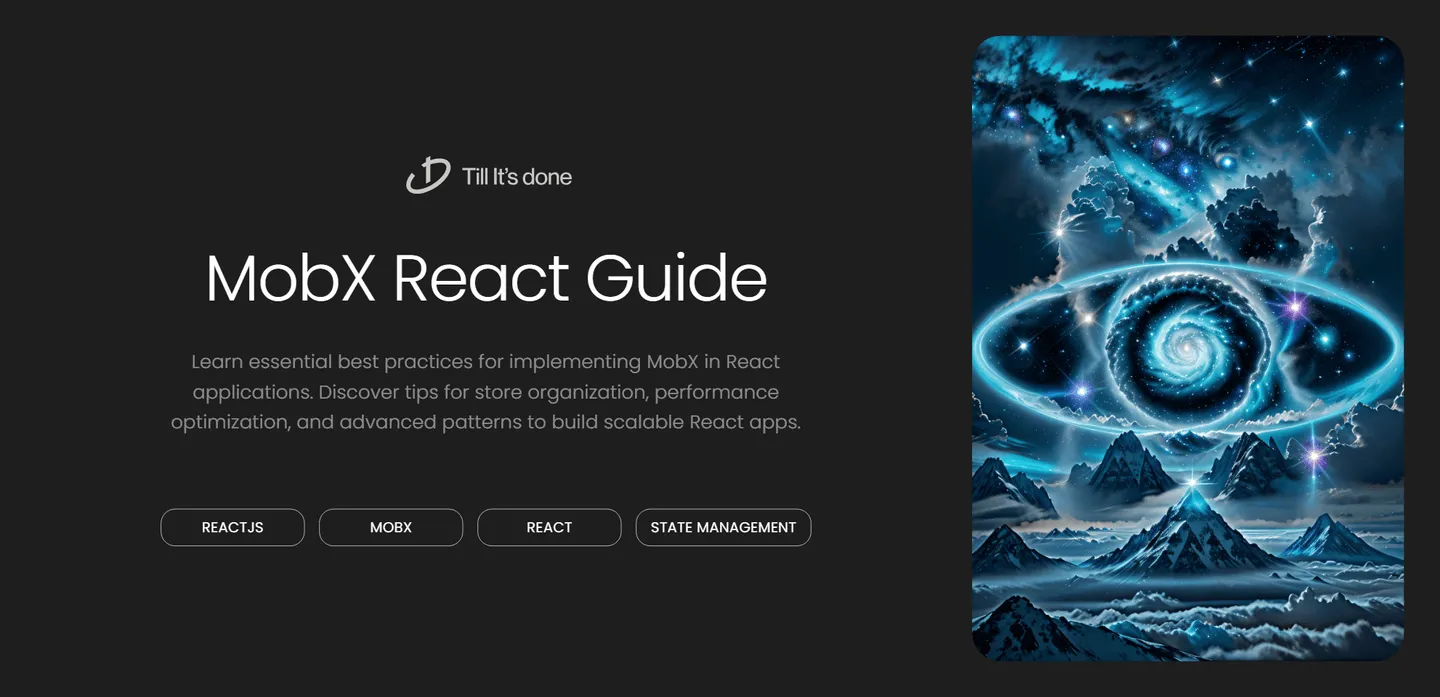
MobX Best Practices for React Developers
Modern React applications demand efficient state management, and MobX stands out as a powerful solution. Let’s explore best practices that will help you write cleaner, more maintainable React applications with MobX.
Understanding MobX Core Concepts
Before diving into best practices, it’s crucial to grasp MobX’s fundamental principles. MobX revolves around three main concepts: observable state, actions, and reactions. Think of your application state as a spreadsheet where changes automatically propagate through your app.
Best Practices for Store Organization
1. Keep Stores Focused and Modular
Instead of creating one massive store, break down your application state into domain-specific stores. For example:
class UserStore { @observable users = []; @observable currentUser = null;
@action setCurrentUser(user) { this.currentUser = user; }}
// productStore.jsclass ProductStore { @observable products = []; @observable selectedProduct = null;}
2. Use Computed Values Effectively
Leverage computed values for derived state. They’re automatically cached and only recalculate when their dependencies change:
class OrderStore { @observable orders = [];
@computed get totalRevenue() { return this.orders.reduce((sum, order) => sum + order.amount, 0); }}
3. Implement Actions for State Modifications
Always use actions to modify state. This ensures proper tracking and helps with debugging:
class TodoStore { @observable todos = [];
@action addTodo(text) { this.todos.push({ id: Date.now(), text, completed: false }); }
@action toggleTodo(id) { const todo = this.todos.find(t => t.id === id); if (todo) { todo.completed = !todo.completed; } }}
Performance Optimization Tips
- Use
observer
Only When Necessary - Implement
reaction
for Side Effects - Utilize
runInAction
for Async Operations - Structure Components to Minimize Re-renders
// Good Example@observerclass TodoList extends React.Component { render() { return ( <div> {this.props.todoStore.filteredTodos.map(todo => ( <TodoItem key={todo.id} todo={todo} /> ))} </div> ); }}
Advanced Patterns
- Use Root Store Pattern for Complex Applications
- Implement Dependency Injection
- Leverage MobX-Utils for Common Patterns
- Handle Async Operations Properly
Remember to keep your stores clean, actions explicit, and components focused. This leads to maintainable and scalable applications.
By following these best practices, you’ll create more robust and maintainable React applications with MobX. Happy coding!
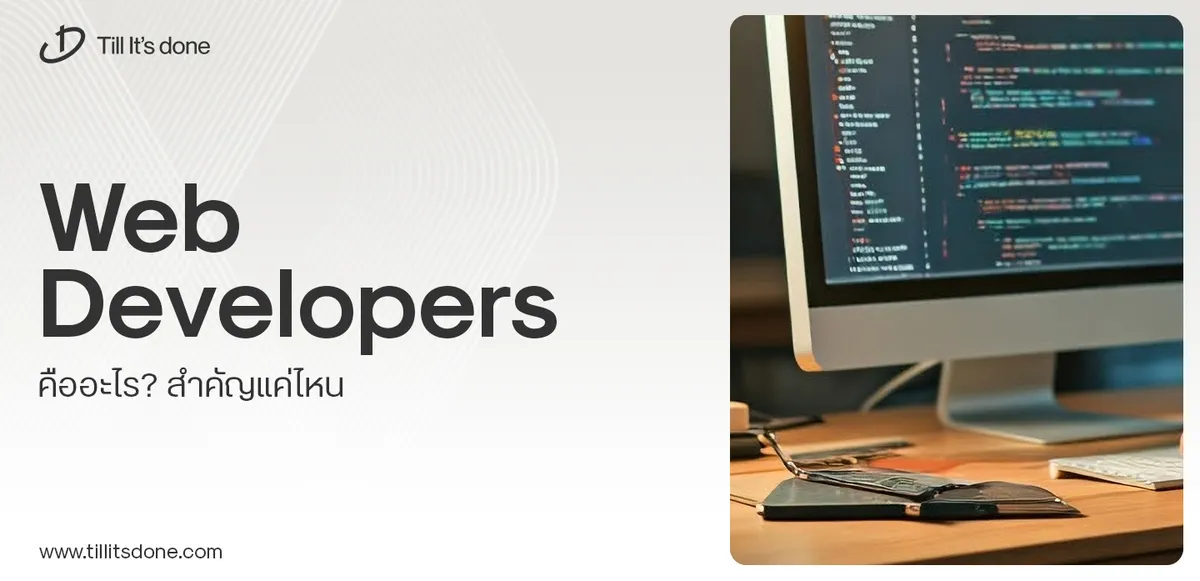
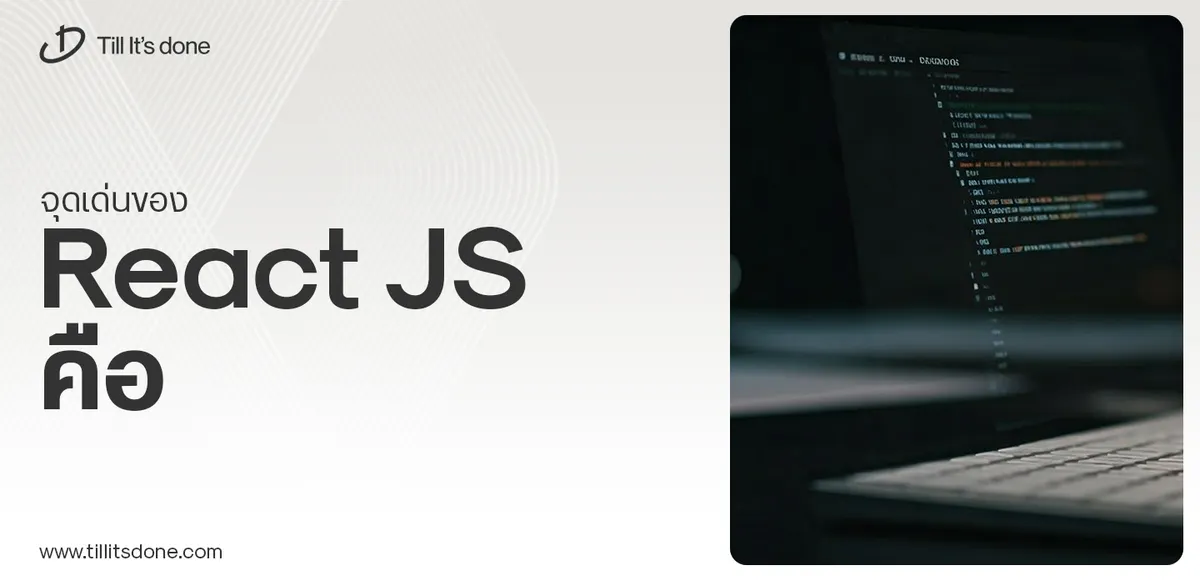
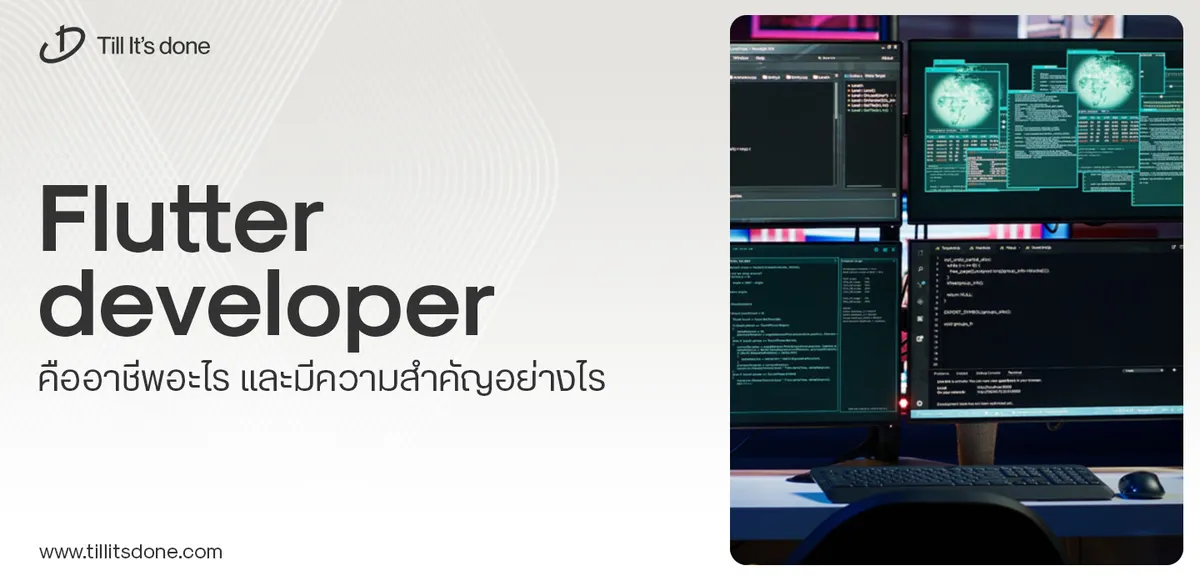
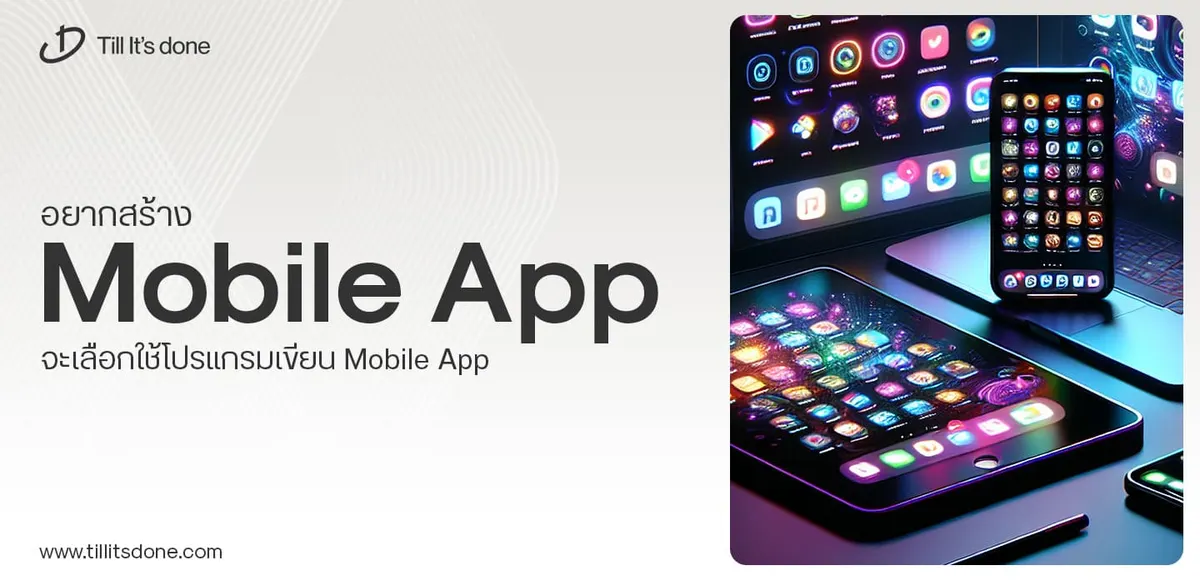
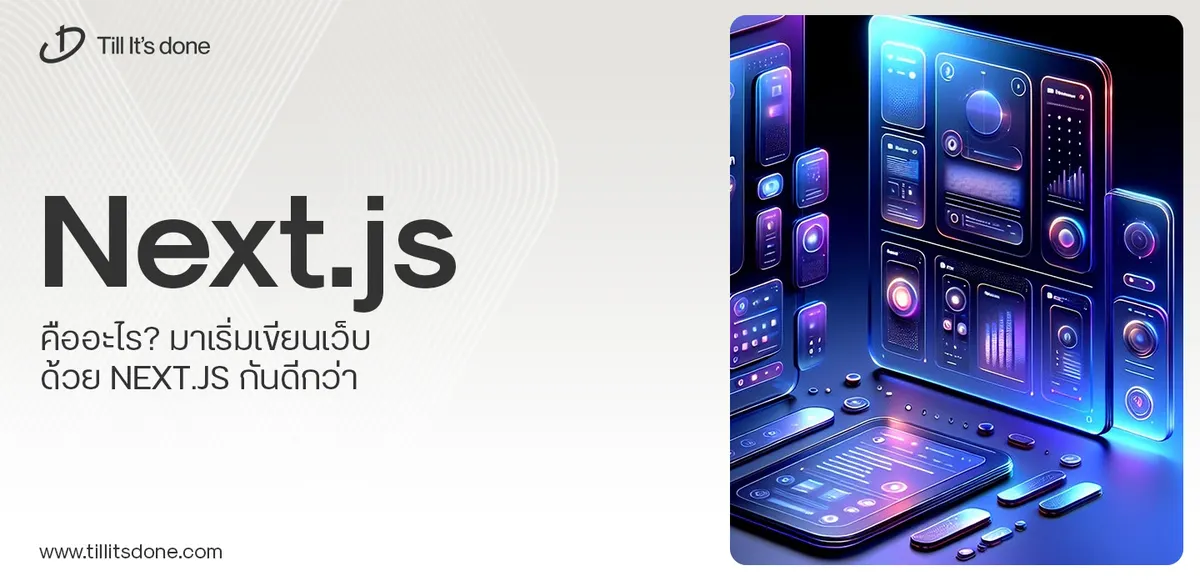
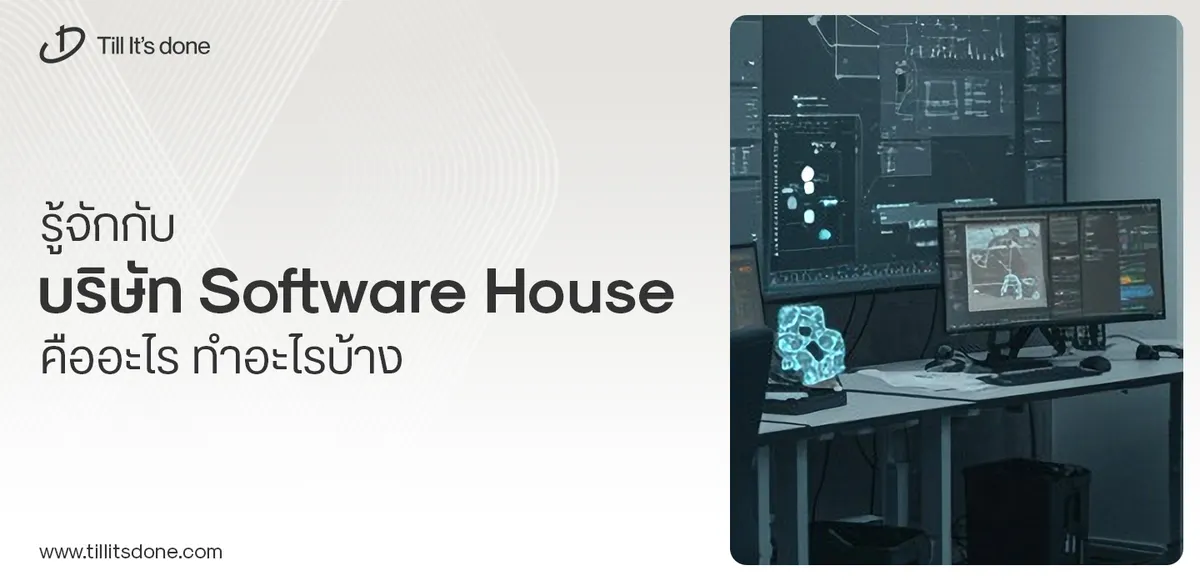
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.