- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
EN
TH
rick@tillitsdone.com
+66824564755
Mastering Async Operations in Koa.js
Learn how to handle asynchronous operations effectively in Koa.js applications.
Explore middleware patterns, error handling, parallel operations, and real-world examples for building robust Node.js applications.
Explore middleware patterns, error handling, parallel operations, and real-world examples for building robust Node.js applications.
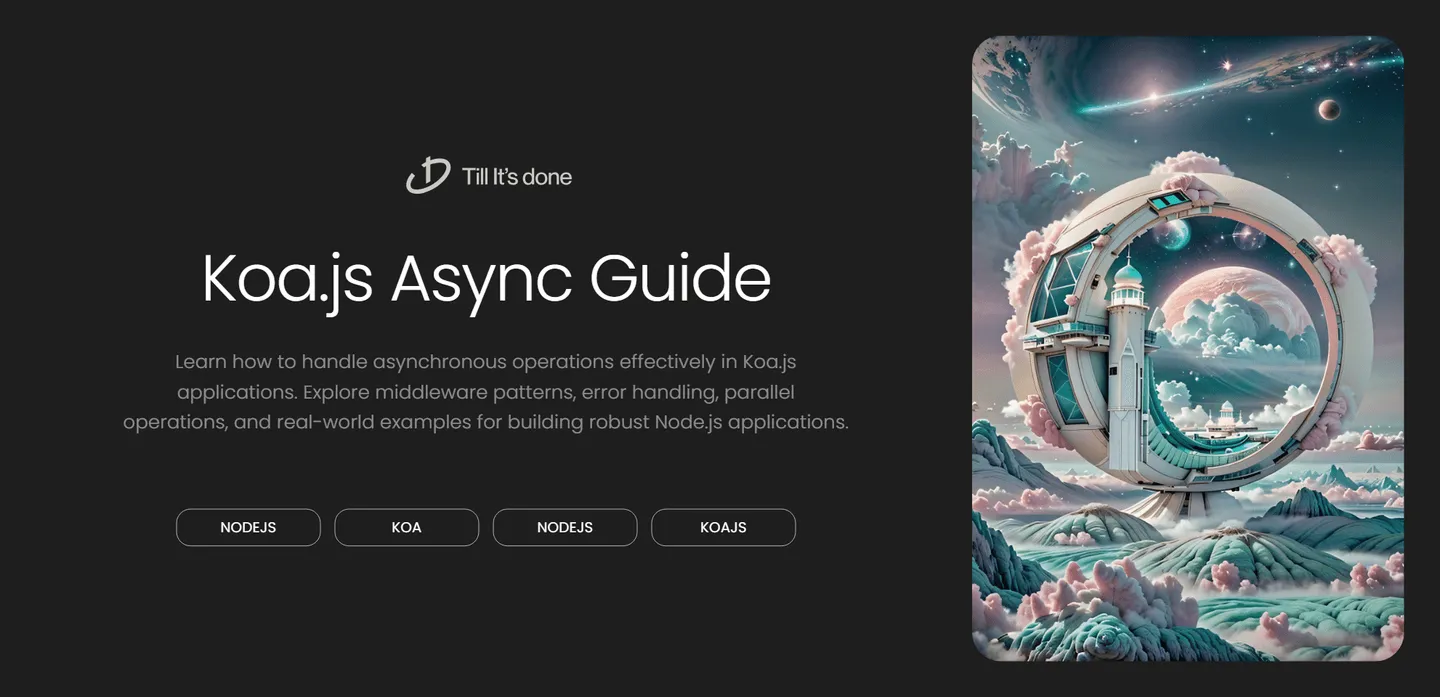
Handling Asynchronous Operations in Koa.js
Asynchronous operations are at the heart of Node.js applications, and Koa.js makes handling them incredibly elegant. Let’s dive into how we can master async operations in Koa to build more efficient and maintainable web applications.
Understanding Async Operations in Koa
Koa’s middleware architecture is built around async/await, making it a perfect framework for handling complex asynchronous operations. Unlike traditional callback-based approaches, Koa’s middleware stack flows downstream and then upstream, creating a “waterfall” pattern that’s both powerful and intuitive.
Key Patterns for Async Operations
1. Basic Async Middleware
app.use(async (ctx, next) => { const start = Date.now(); await next(); const ms = Date.now() - start; ctx.set('X-Response-Time', `${ms}ms`);});
2. Error Handling
One of Koa’s strengths is its elegant error handling approach:
app.use(async (ctx, next) => { try { await next(); } catch (err) { ctx.status = err.status || 500; ctx.body = { error: err.message }; ctx.app.emit('error', err, ctx); }});
3. Parallel Operations
Sometimes you need to run multiple async operations simultaneously:
app.use(async (ctx) => { const [users, posts] = await Promise.all([ User.find({}), Post.find({}) ]); ctx.body = { users, posts };});
Best Practices
- Always use try-catch blocks for error handling
- Leverage Promise.all() for concurrent operations
- Keep middleware functions focused and composable
- Use async/await consistently throughout your application
Real-world Example
Here’s a practical example of handling multiple async operations in a Koa route:
router.get('/dashboard', async (ctx) => { try { const userPromise = User.findById(ctx.user.id); const analyticsPromise = Analytics.getRecentData(); const notificationsPromise = Notification.getUserNotifications(ctx.user.id);
const [user, analytics, notifications] = await Promise.all([ userPromise, analyticsPromise, notificationsPromise ]);
ctx.body = { user, analytics, notifications }; } catch (error) { ctx.throw(500, error.message); }});
Discover our top articles, selected to support the growth of your business.
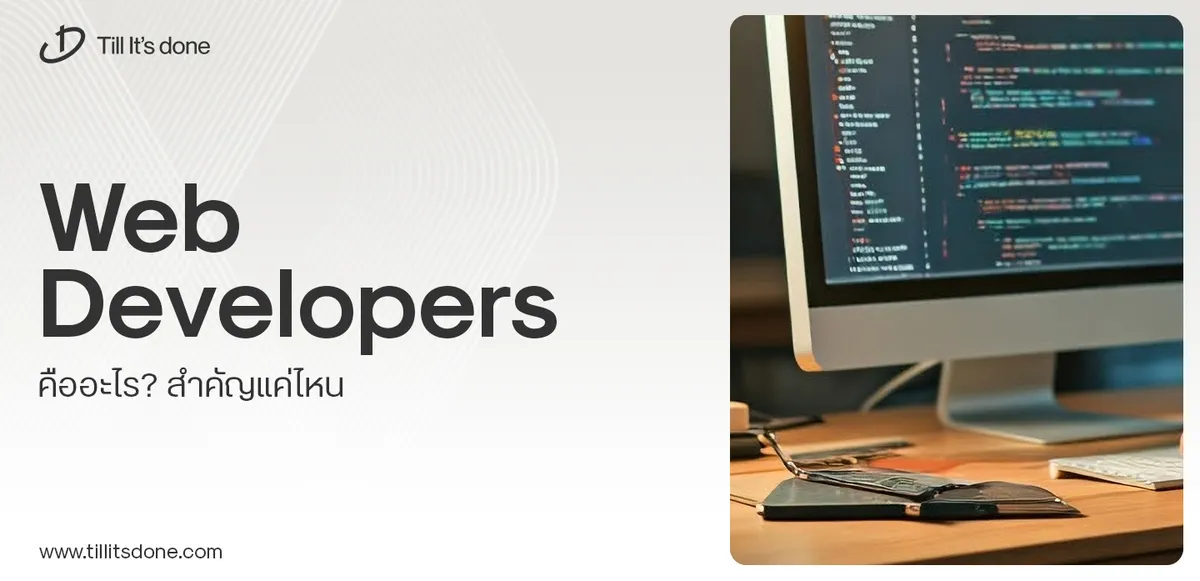
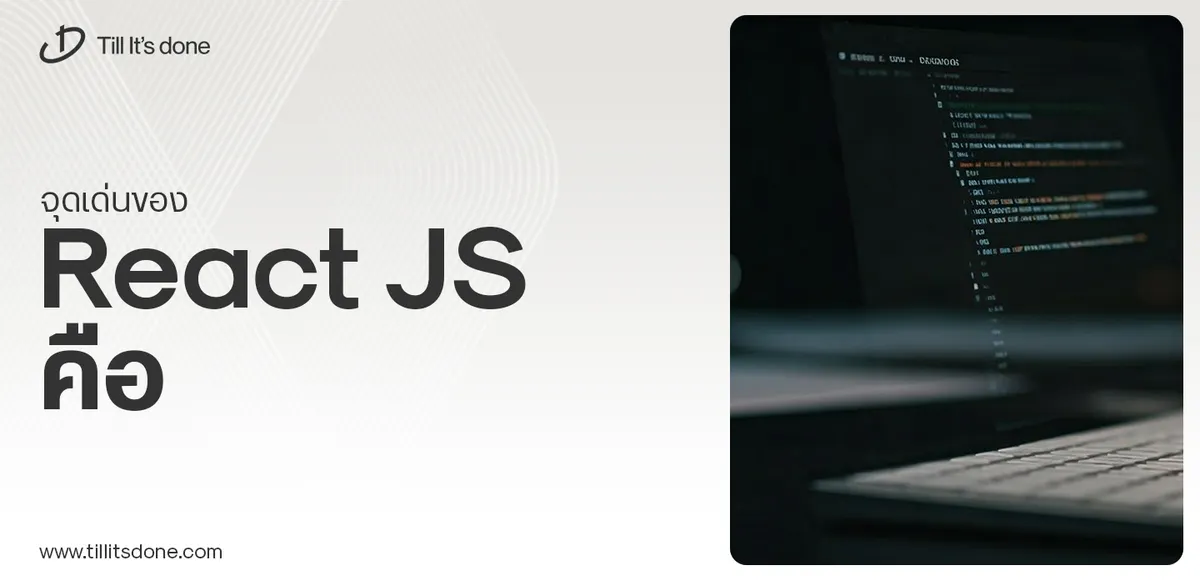
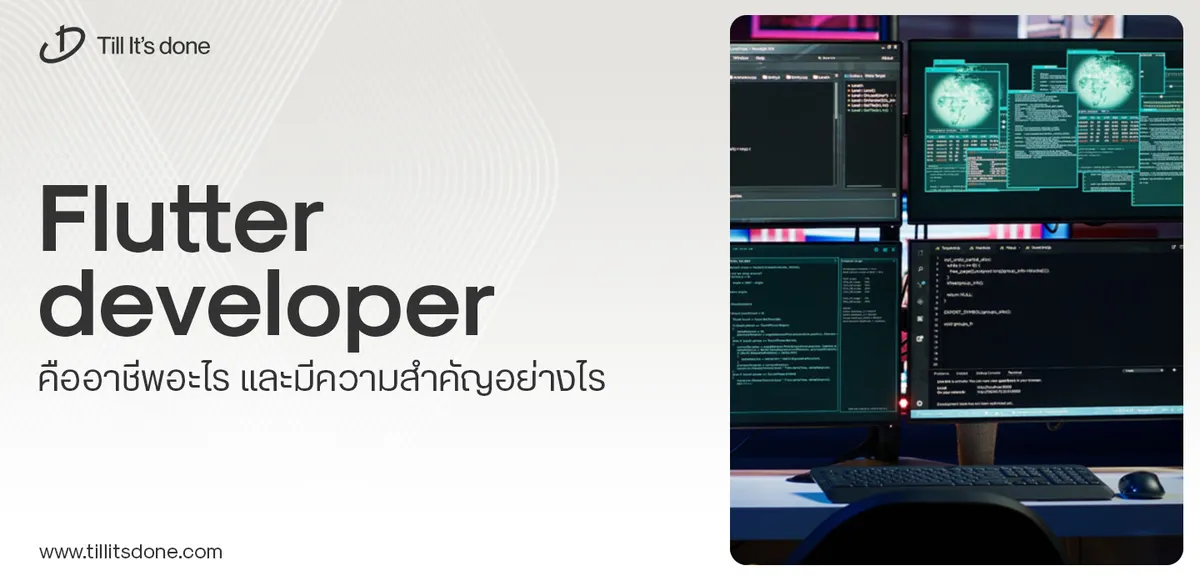
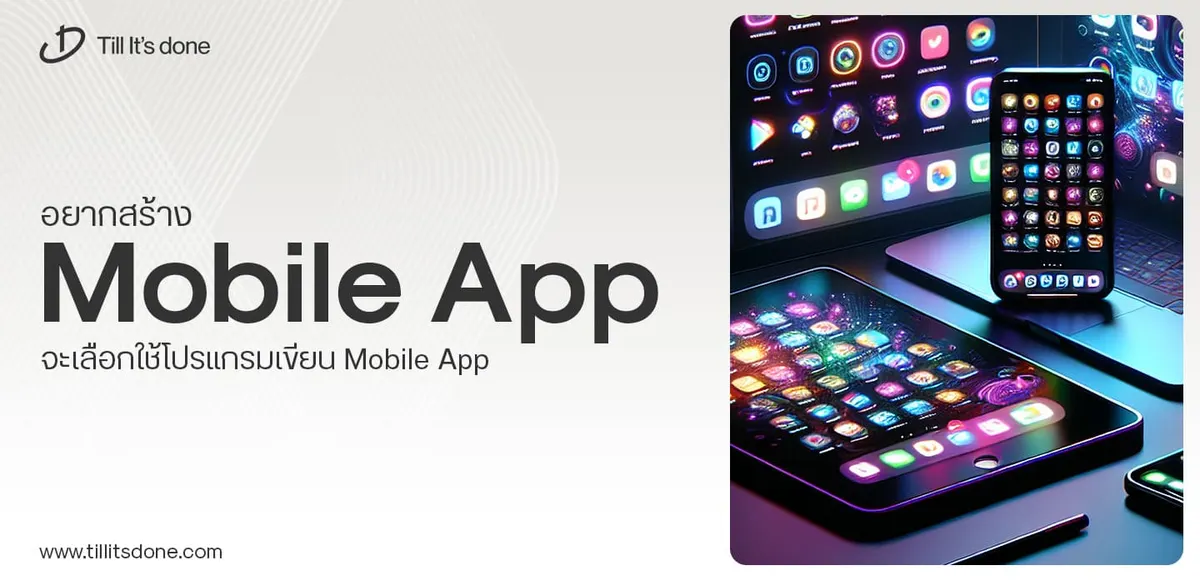
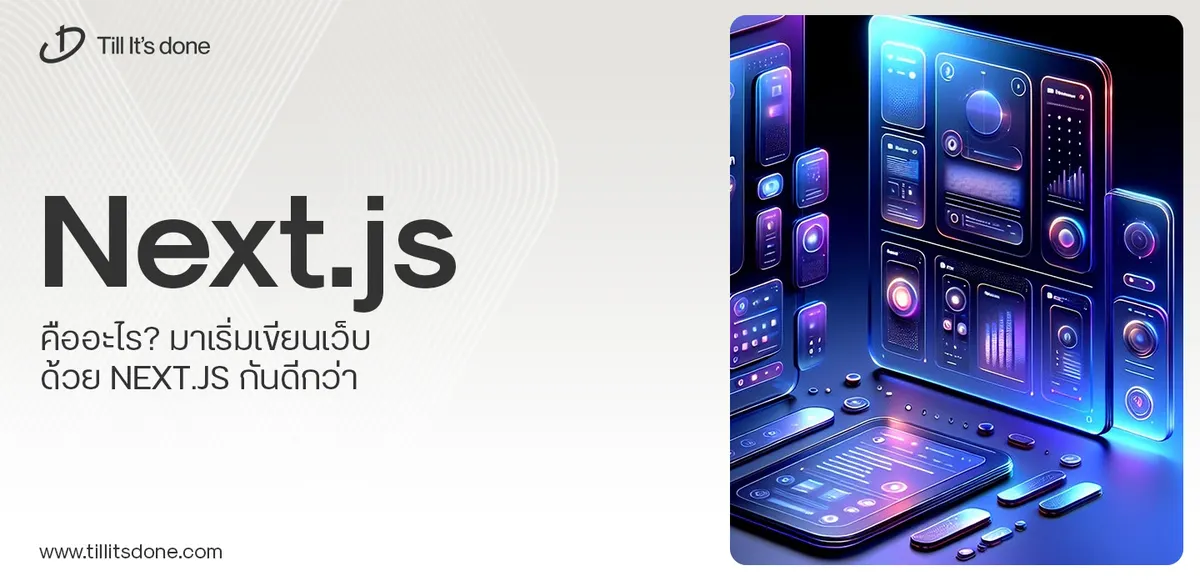
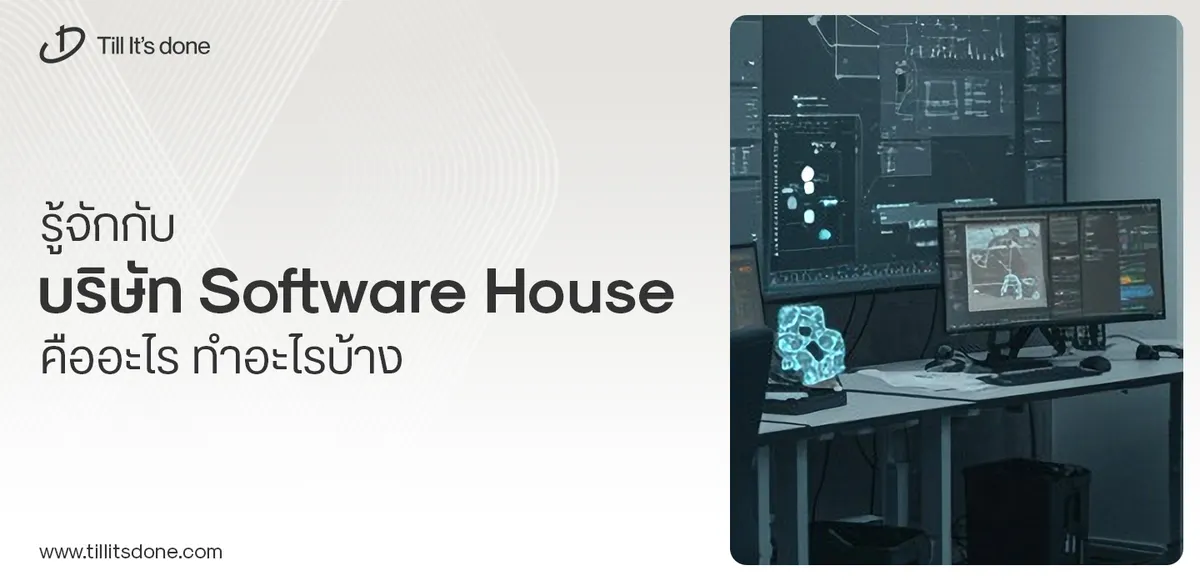
Talk with CEO
Ready to bring your web/app to life or boost your team with expert Thai developers?
Contact us today to discuss your needs, and let’s create tailored solutions to achieve your goals. We’re here to help at every step!
🖐️ Contact us 196 Articles
Explore Popular JavaScript library for building user interfaces with a component-based architecture.
160 Articles
Explore UI toolkit for building natively compiled applications for mobile, web, and desktop from a single codebase.
144 Articles
Explore JavaScript runtime for building scalable, high-performance server-side applications.
58 Articles
Explore React framework enabling server-side rendering and static site generation for optimized performance.
38 Articles
Explore Utility-first CSS framework for rapid UI development.
36 Articles
Explore Superset of JavaScript adding static types for improved code quality and maintainability.
126 Articles
Explore Programming language known for its simplicity, concurrency model, and performance.
67 Articles
Explore Astro is an all-in-one web framework. It includes everything you need to create a website, built-in.
38 Articles
Explore Versatile testing framework for JavaScript applications supporting various test types.
5 Articles
Explore 4 Articles
Explore 4 Articles
Explore 2 Articles
Explore 1 Articles
Explore 1 Articles
Explore 337 Articles
Explore CSS3 is the latest version of Cascading Style Sheets, offering advanced styling features like animations, transitions, shadows, gradients, and responsive design.
Let's keep in Touch
Thank you for your interest in Tillitsdone! Whether you have a question about our services, want to discuss a potential project, or simply want to say hello, we're here and ready to assist you.
We'll be right here with you every step of the way.
We'll be right here with you every step of the way.
Contact Information
rick@tillitsdone.com+66824564755
Address
9 Phahonyothin Rd, Khlong Nueng, Khlong Luang District, Pathum Thani, Bangkok Thailand
Social media
FacebookInstagramLinkedIn
We anticipate your communication and look forward to discussing how we can contribute to your business's success.
We'll be here, prepared to commence this promising collaboration.
We'll be here, prepared to commence this promising collaboration.
Frequently Asked Questions
Explore frequently asked questions about our products and services.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.