- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Master TypeScript Generics: Essential Tips & Code
Learn how to create type-safe APIs, build reusable components, and leverage advanced generic patterns for better code.
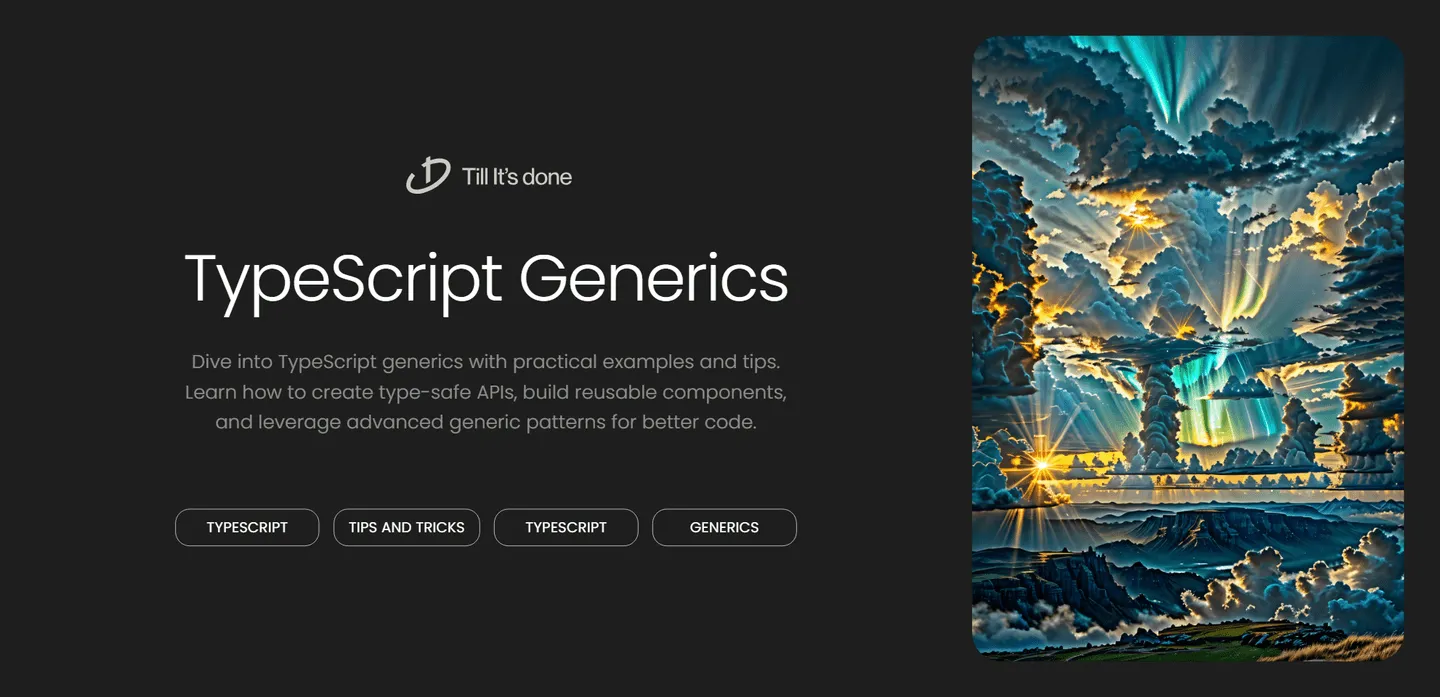
Mastering TypeScript Generics: Tips and Examples
If you’ve been working with TypeScript, you’ve probably encountered generics. They might seem intimidating at first, but once you grasp their power, they become an indispensable tool in your TypeScript arsenal. Let’s dive into some practical examples and tips that will help you level up your TypeScript game.
Understanding the Basics
Think of generics as placeholders for types that you’ll define later. Instead of committing to a specific type, you’re keeping your options open while maintaining type safety. It’s like having a box that can hold any type of item, but once you decide what goes in, everything stays consistent.
Real-World Applications
1. Type-Safe API Responses
One of the most common use cases for generics is handling API responses. Here’s how you can create a type-safe wrapper for your API calls:
interface ApiResponse\<T\> { data: T; status: number; message: string;}
async function fetchData\<T\>(url: string): Promise<ApiResponse\<T\>> { const response = await fetch(url); return await response.json();}
// Usageinterface User { id: number; name: string;}
const user = await fetchData<User>('/api/user/1');// user.data.name is now type-safe!
2. Smart Component Props
When building reusable components, generics help create flexible yet type-safe interfaces:
interface ListProps\<T\> { items: T[]; renderItem: (item: T) => React.ReactNode; keyExtractor: (item: T) => string;}
function List\<T\>(props: ListProps\<T\>) { return ( <ul> {props.items.map(item => ( <li key={props.keyExtractor(item)}> {props.renderItem(item)} </li> ))} </ul> );}
Advanced Tips and Tricks
Constraint Magic
Use constraints to make your generics more powerful and predictable:
interface HasId { id: number | string;}
function findById<T extends HasId>(items: T[], id: T['id']): T | undefined { return items.find(item => item.id === id);}
Generic Type Inference
Let TypeScript’s inference system work for you:
function pick<T, K extends keyof T>(obj: T, keys: K[]): Pick\<T, K\> { const result = {} as Pick\<T, K\>; keys.forEach(key => { result[key] = obj[key]; }); return result;}
// TypeScript infers everything!const person = { name: 'John', age: 30, email: 'john@example.com' };const nameAge = pick(person, ['name', 'age']);
Best Practices
- Keep it simple - don’t over-genericize your code
- Use meaningful type parameter names (T for type, K for key, V for value)
- Consider adding constraints when you know the type should have certain properties
- Use multiple type parameters when it makes sense, but don’t go overboard
Remember, generics are tools to help you write better, more maintainable code. They shouldn’t make your code more complicated - if they do, step back and reconsider your approach.
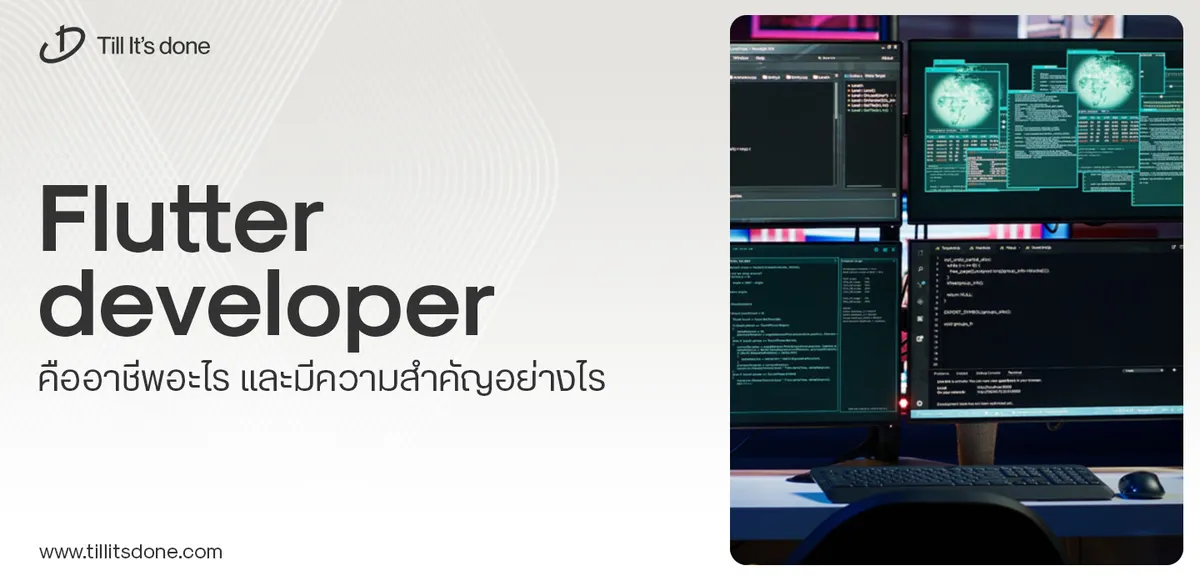
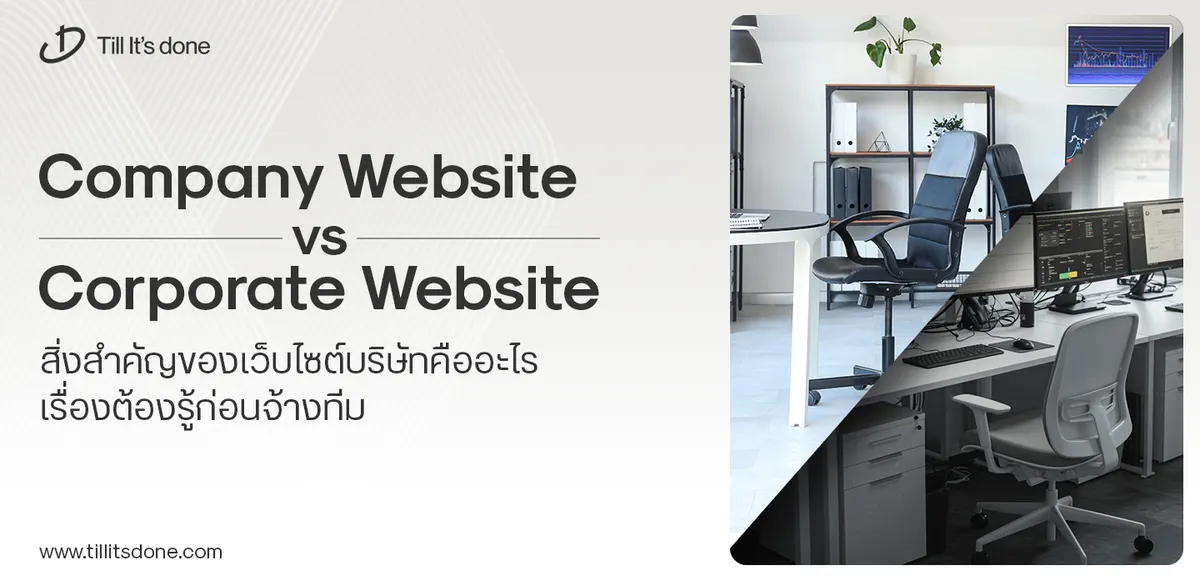
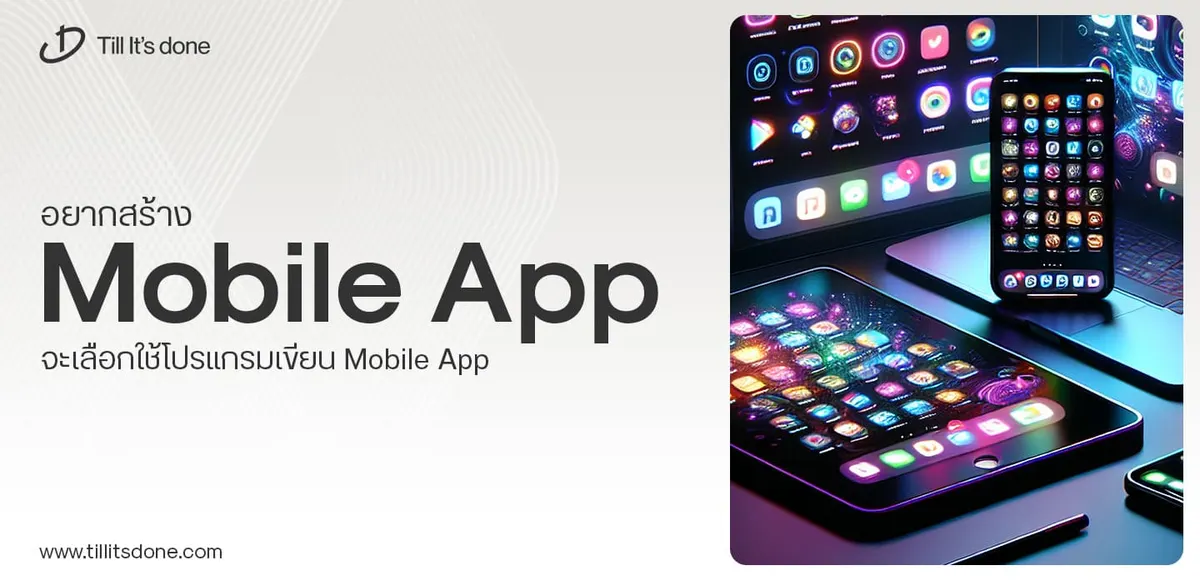
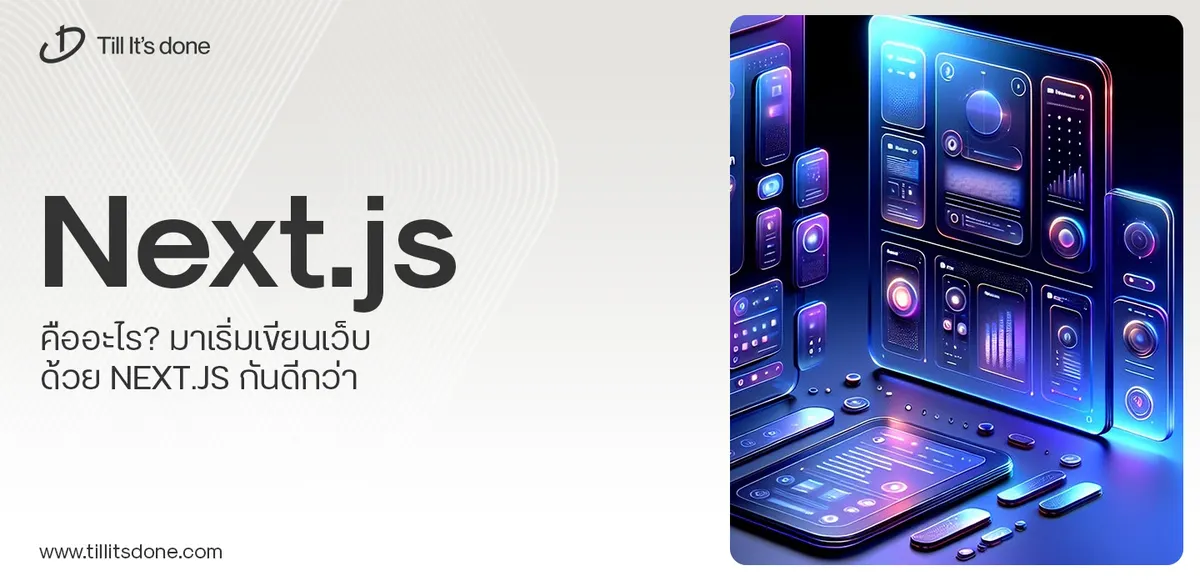
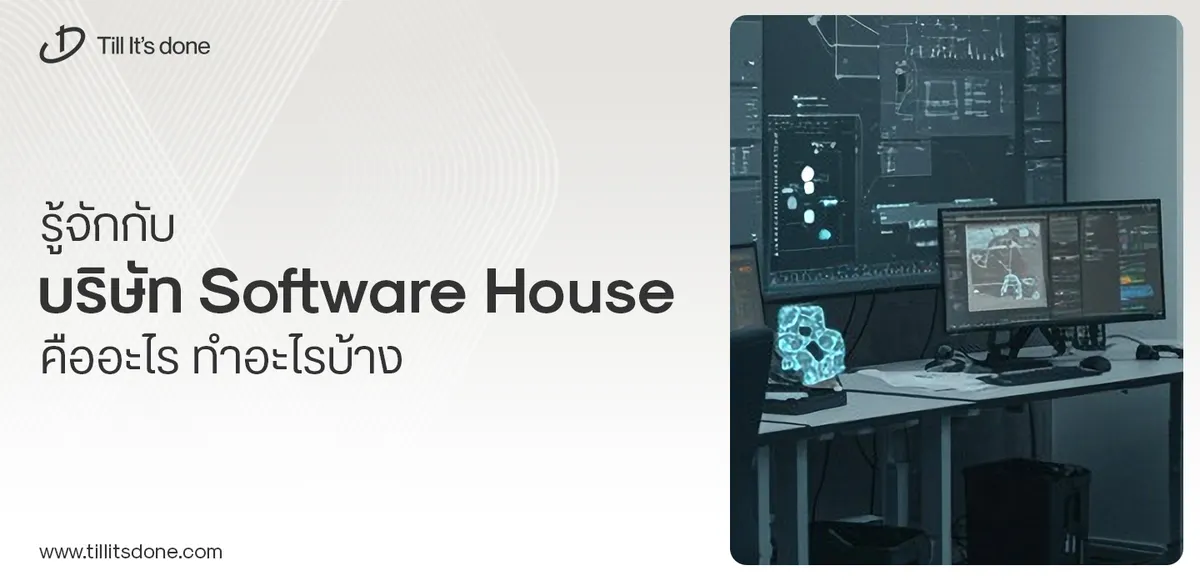
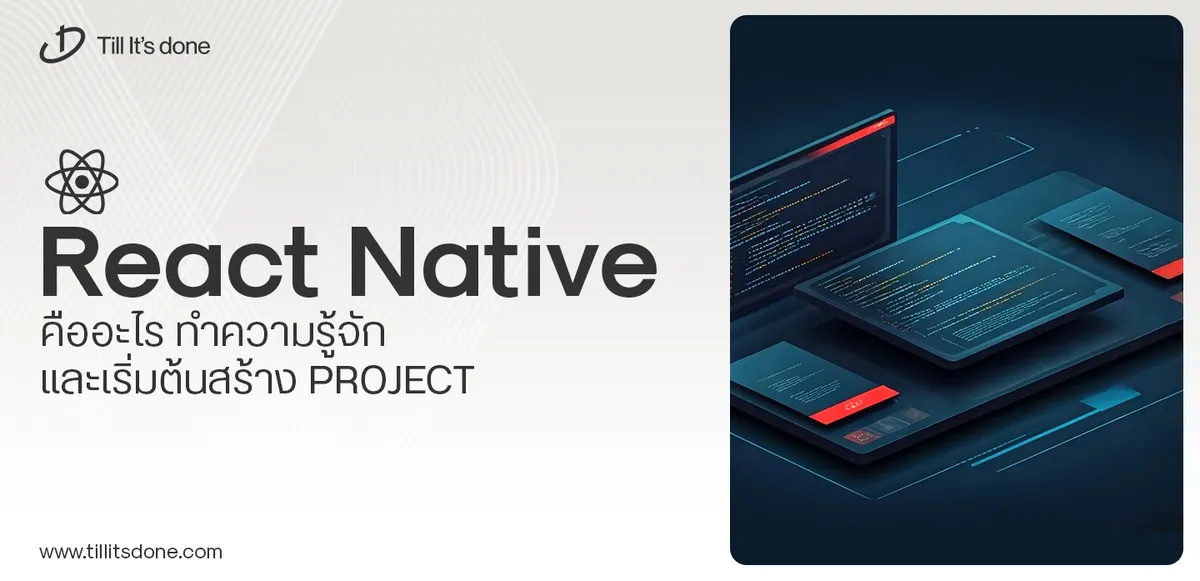
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.