- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
How to Write Maintainable TypeScript Code
Discover tips on configuration, interfaces, type systems, and design patterns to improve code quality and team collaboration.
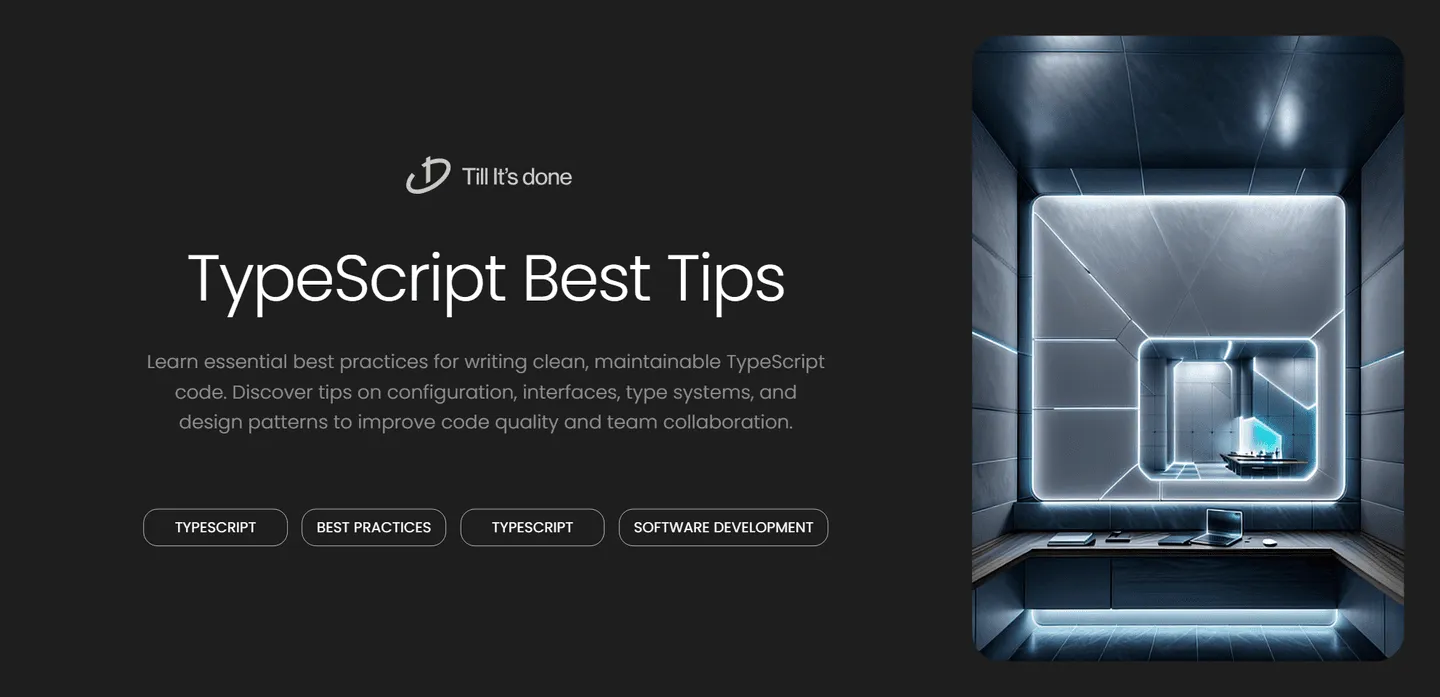
How to Write Maintainable TypeScript Code
Writing maintainable TypeScript code is crucial for long-term project success. As codebases grow and team members come and go, having clean, readable, and maintainable code becomes increasingly important. Let’s explore the best practices that can help you achieve this goal.
Start with Strong TypeScript Configuration
Your TypeScript configuration sets the foundation for your entire project. A strict configuration helps catch potential issues early and enforces better coding practices:
{ "compilerOptions": { "strict": true, "noImplicitAny": true, "strictNullChecks": true, "noUncheckedIndexedAccess": true }}
Define Clear Interface Contracts
Interfaces are your best friends in TypeScript. They help define clear contracts between different parts of your application:
// Badfunction processUser(user: any) { // ...}
// Goodinterface User { id: string; name: string; email: string; role: 'admin' | 'user';}
function processUser(user: User) { // ...}
Leverage TypeScript’s Type System
TypeScript’s type system is powerful and can help you write more maintainable code. Use utility types, discriminated unions, and generics when appropriate:
// Using utility typestype UserWithoutId = Omit<User, 'id'>;type ReadonlyUser = Readonly<User>;
// Discriminated unionstype Success\<T\> = { status: 'success'; data: T;};
type Error = { status: 'error'; message: string;};
type ApiResponse\<T\> = Success\<T\> | Error;
Organize Code with Design Patterns
Implement common design patterns to solve recurring problems in a consistent way:
// Singleton Patternclass Configuration { private static instance: Configuration; private constructor() {}
static getInstance(): Configuration { if (!Configuration.instance) { Configuration.instance = new Configuration(); } return Configuration.instance; }}
Best Practices for Maintainability
- Use meaningful variable and function names
- Keep functions small and focused
- Document complex logic with clear comments
- Implement proper error handling
- Write unit tests for critical functionality
- Use consistent code formatting
- Regularly update dependencies
Error Handling
// Badtry { doSomething();} catch (e) { console.log(e);}
// Goodclass CustomError extends Error { constructor(message: string, public readonly code: string) { super(message); this.name = 'CustomError'; }}
try { doSomething();} catch (error) { if (error instanceof CustomError) { // Handle specific error } else { // Handle unknown error }}
Conclusion
Writing maintainable TypeScript code is an ongoing process that requires attention to detail and consistent application of best practices. By following these guidelines, you’ll create code that’s easier to understand, debug, and maintain over time.
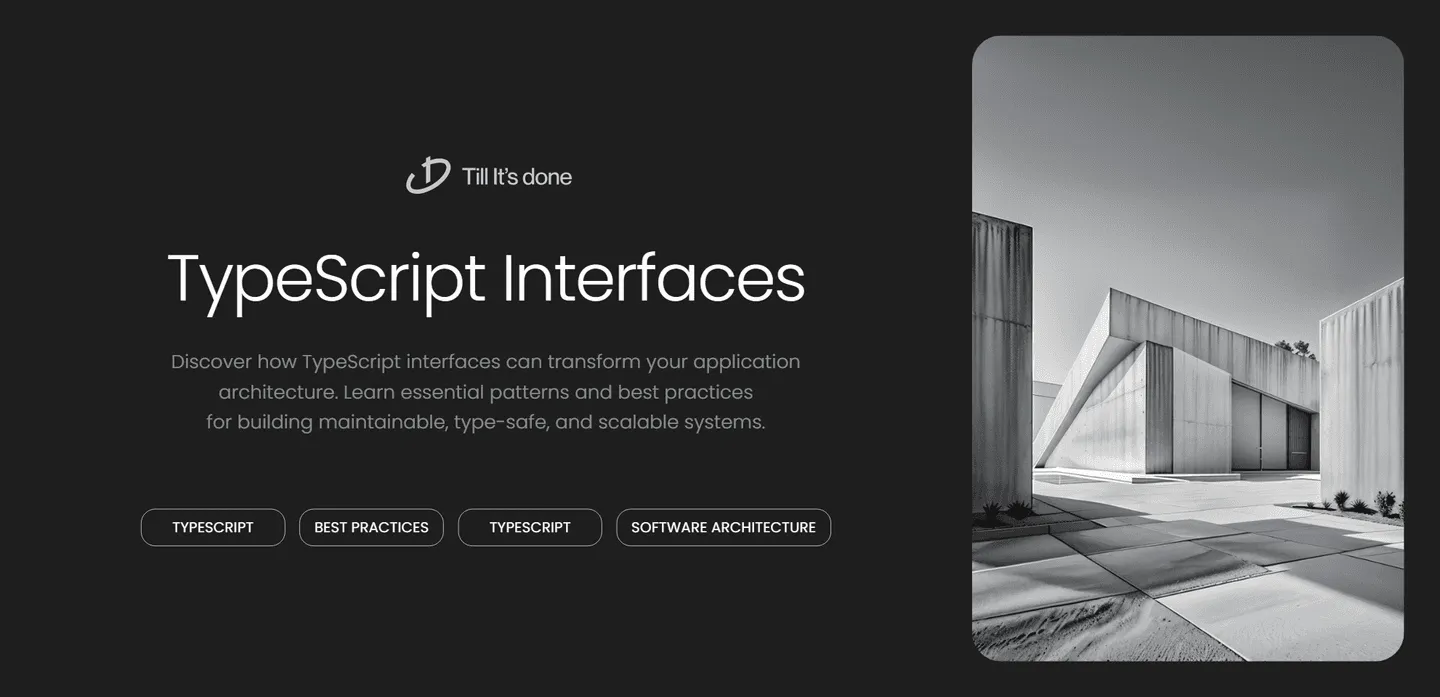
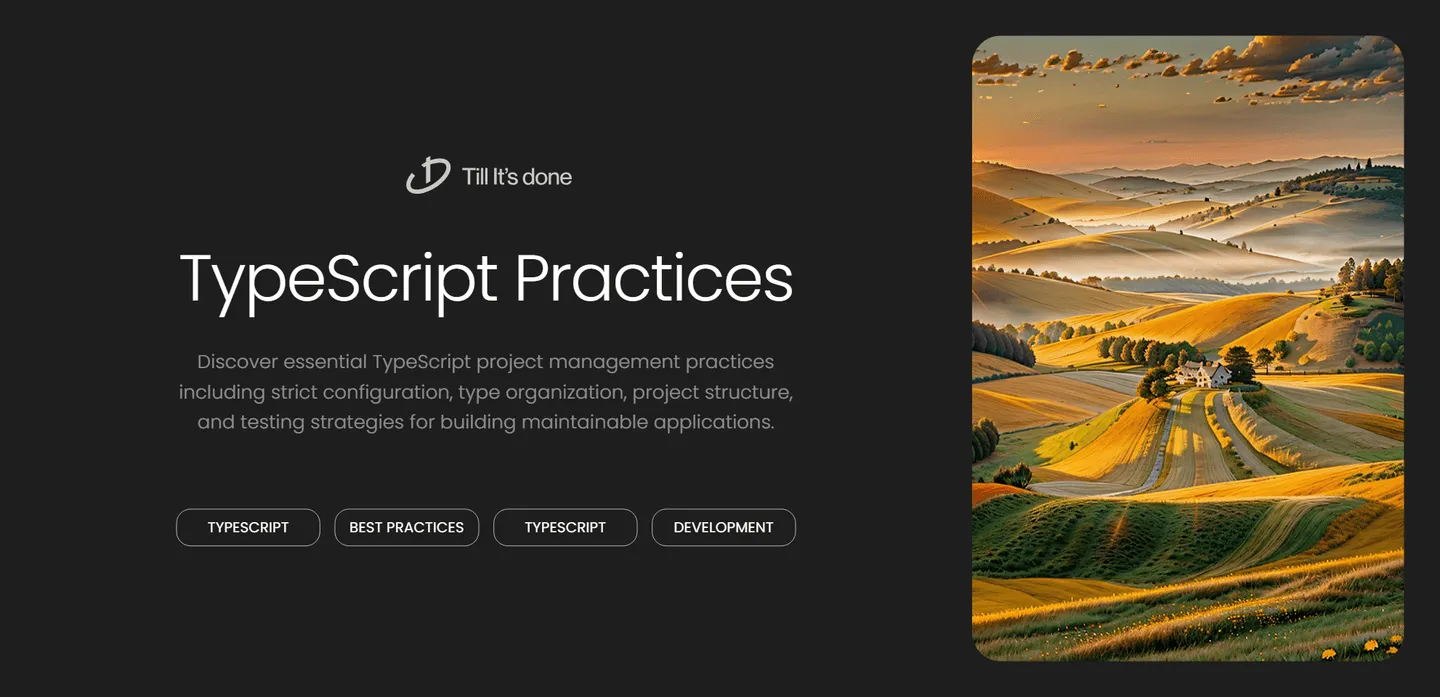
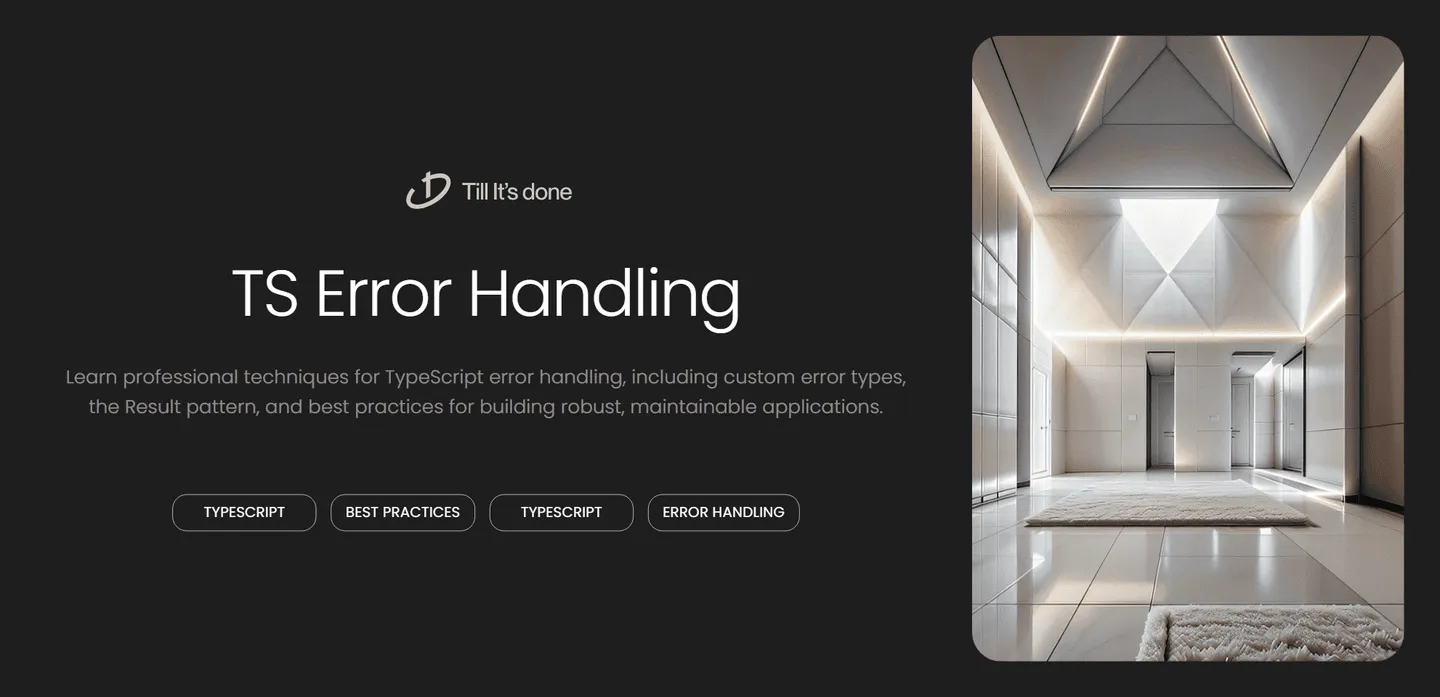
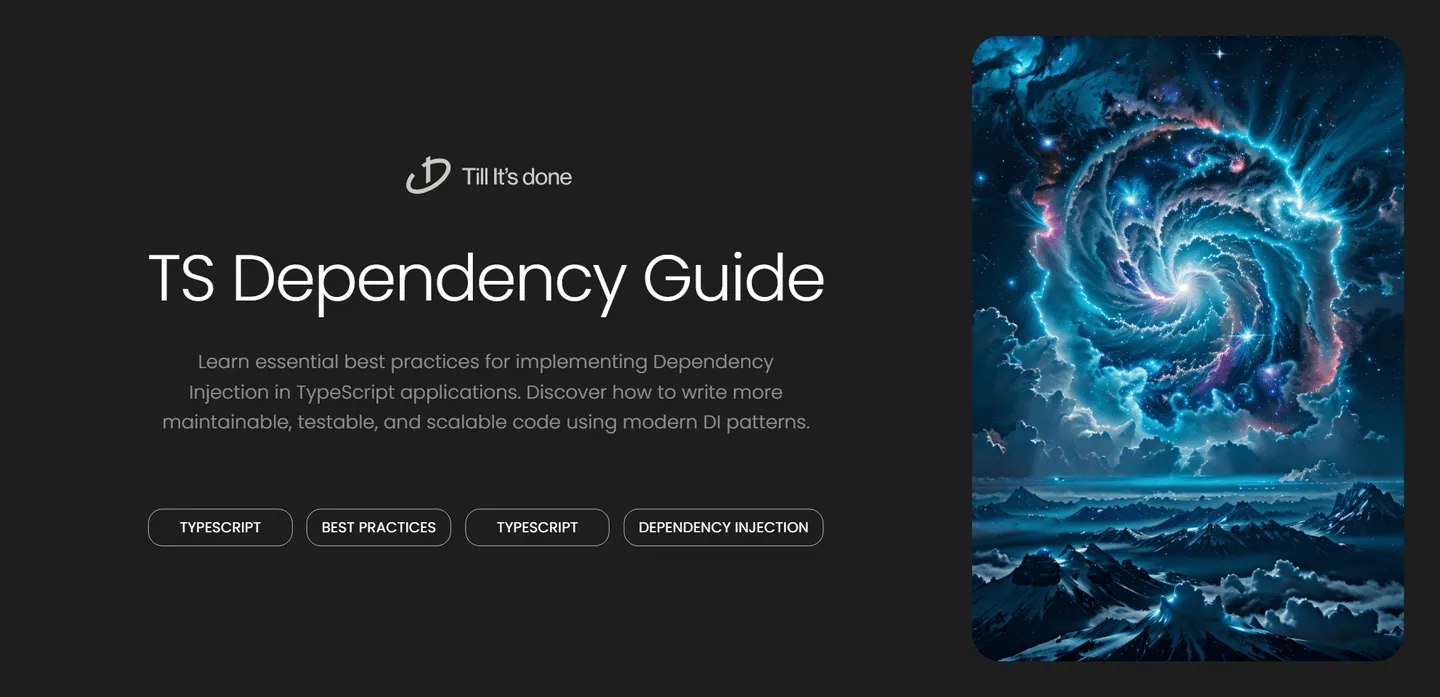
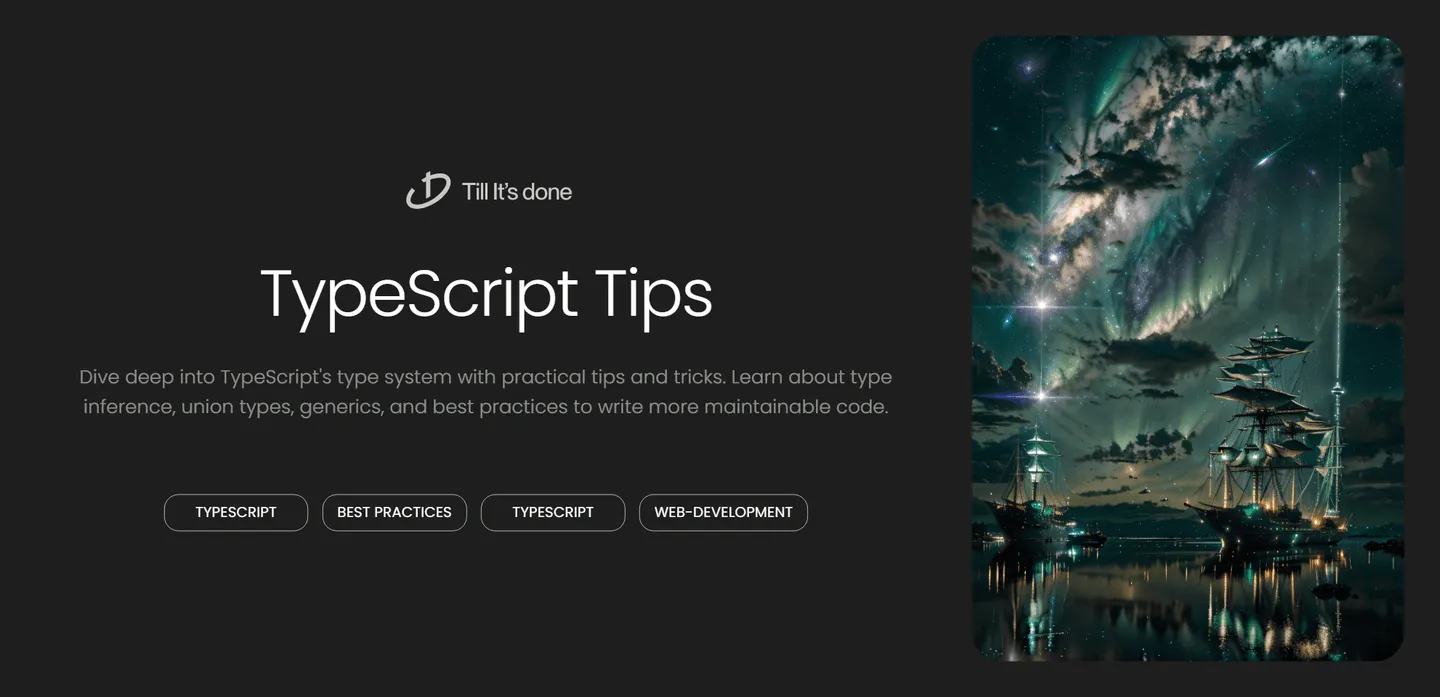
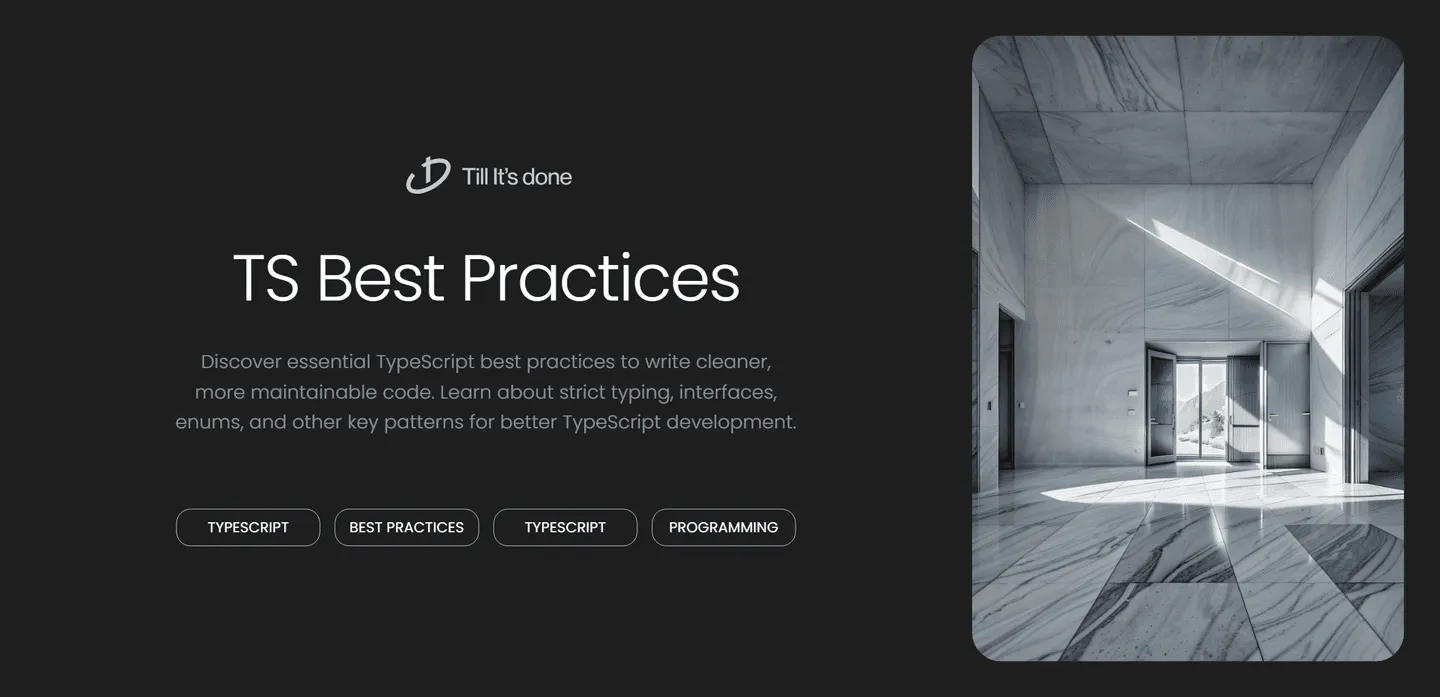
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.