- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Create Custom Middleware in Koa.js Guide
Master the middleware flow, understand best practices, and build powerful Node.js applications with practical examples.
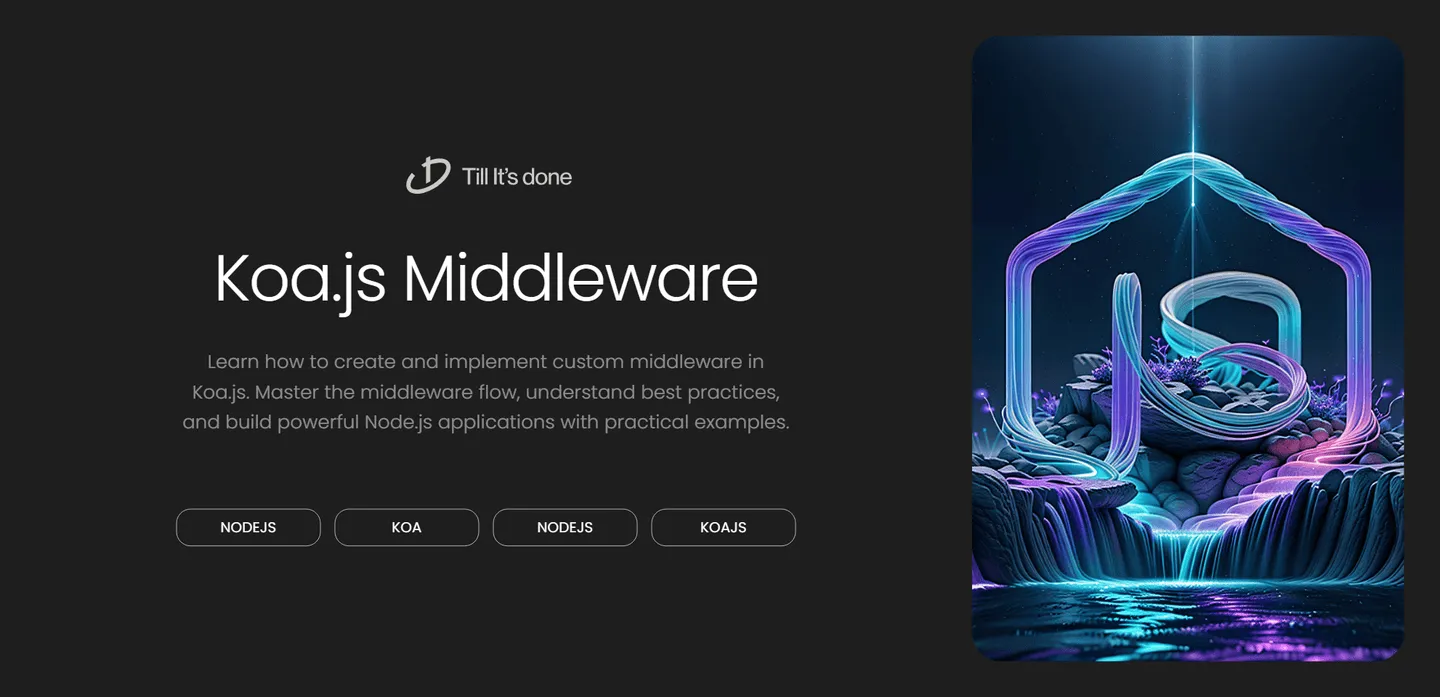
Middleware in Koa.js: How to Create and Use Custom Middleware
Middleware is the heart and soul of Koa.js. Think of it as a series of functions that your requests flow through, each adding its own magic to the process. Today, let’s dive into creating and using custom middleware in Koa.js - and I promise to keep it simple!
What is Middleware?
In Koa, middleware functions are like checkpoints that your request passes through before getting a response. Each middleware can do something with the request, pass it on to the next middleware, or even stop the process entirely.
Creating Your First Middleware
Let’s start with a basic example. Here’s how you create a simple logging middleware:
const logger = async (ctx, next) => { const start = Date.now(); await next(); const ms = Date.now() - start; console.log(`${ctx.method} ${ctx.url} - ${ms}ms`);};
app.use(logger);
Understanding the Middleware Flow
The real power of Koa middleware lies in its cascade structure. When a request comes in, it flows down through your middleware stack, then flows back up once it hits the end. This is often called the “onion model”.
app.use(async (ctx, next) => { console.log('Going down...'); await next(); console.log('Coming back up!');});
Practical Middleware Examples
Here’s a useful error-handling middleware:
const errorHandler = async (ctx, next) => { try { await next(); } catch (err) { ctx.status = err.status || 500; ctx.body = { error: err.message }; ctx.app.emit('error', err, ctx); }};
Best Practices
- Keep middleware focused on a single responsibility
- Use async/await for better flow control
- Always remember to call
next()
unless you’re ending the response - Handle errors appropriately
- Order your middleware logically - more generic first, specific last
Building custom middleware in Koa.js is straightforward once you understand these core concepts. With these tools in your belt, you can create powerful, maintainable applications that handle complex operations with ease.
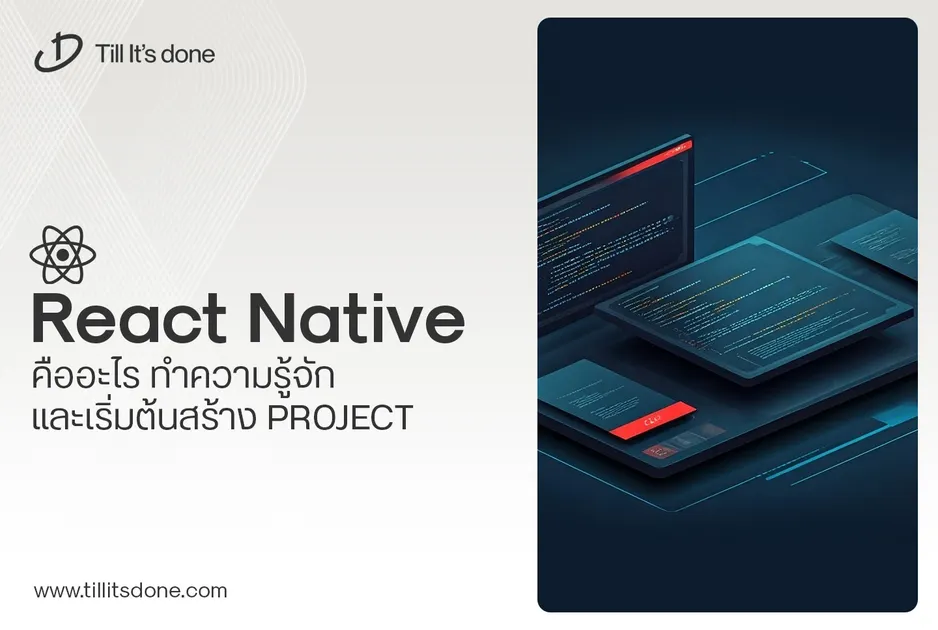
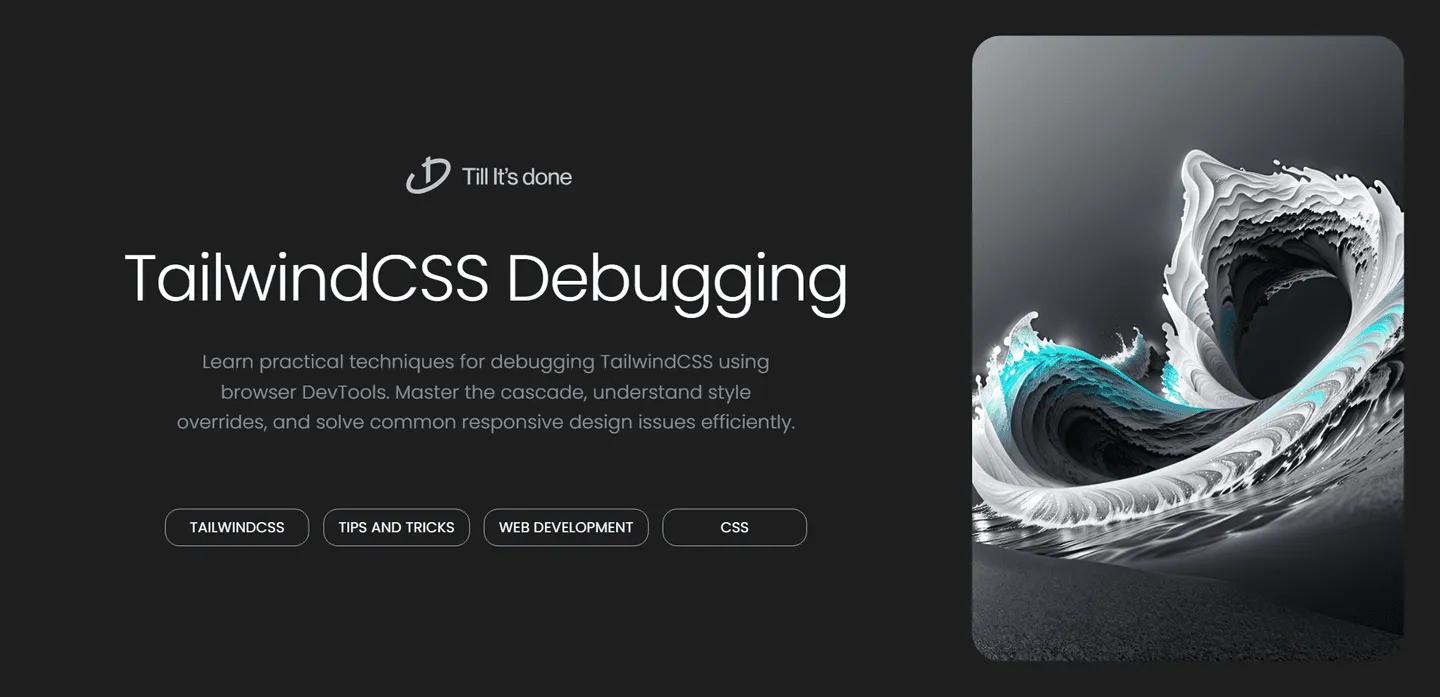
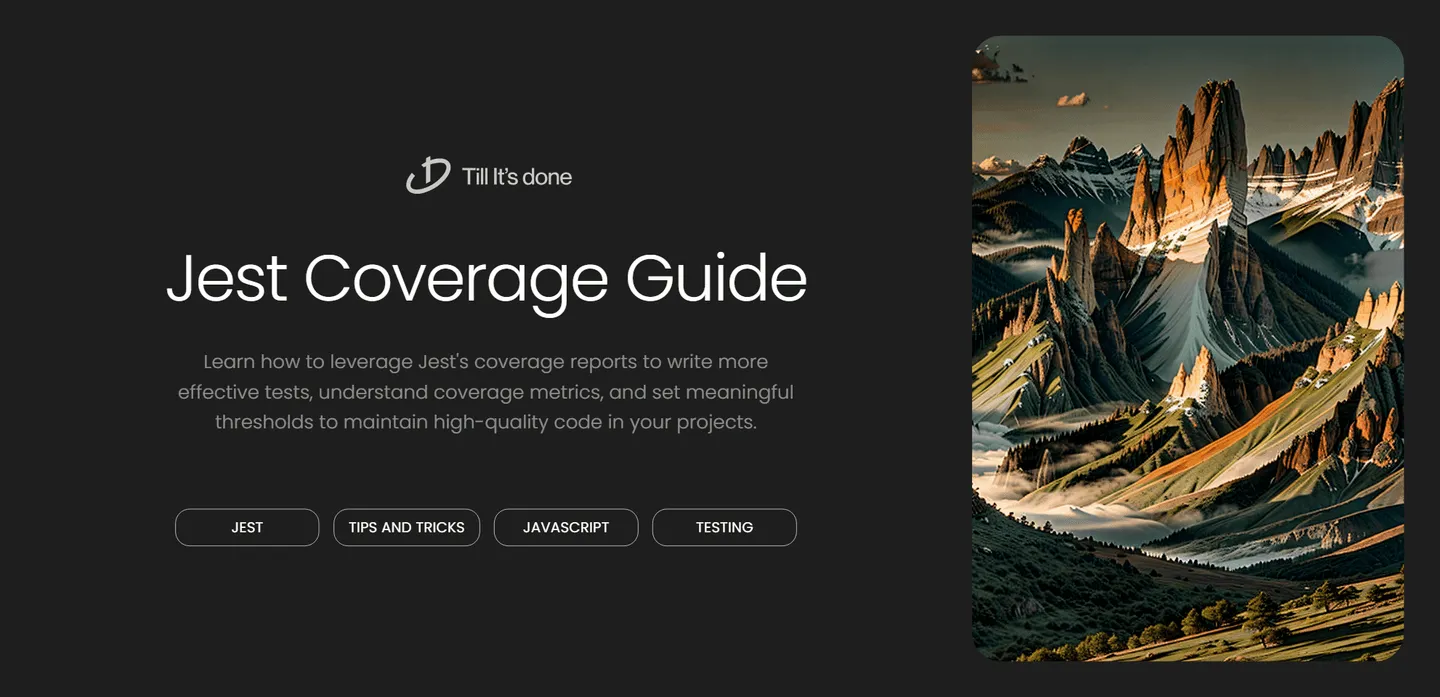
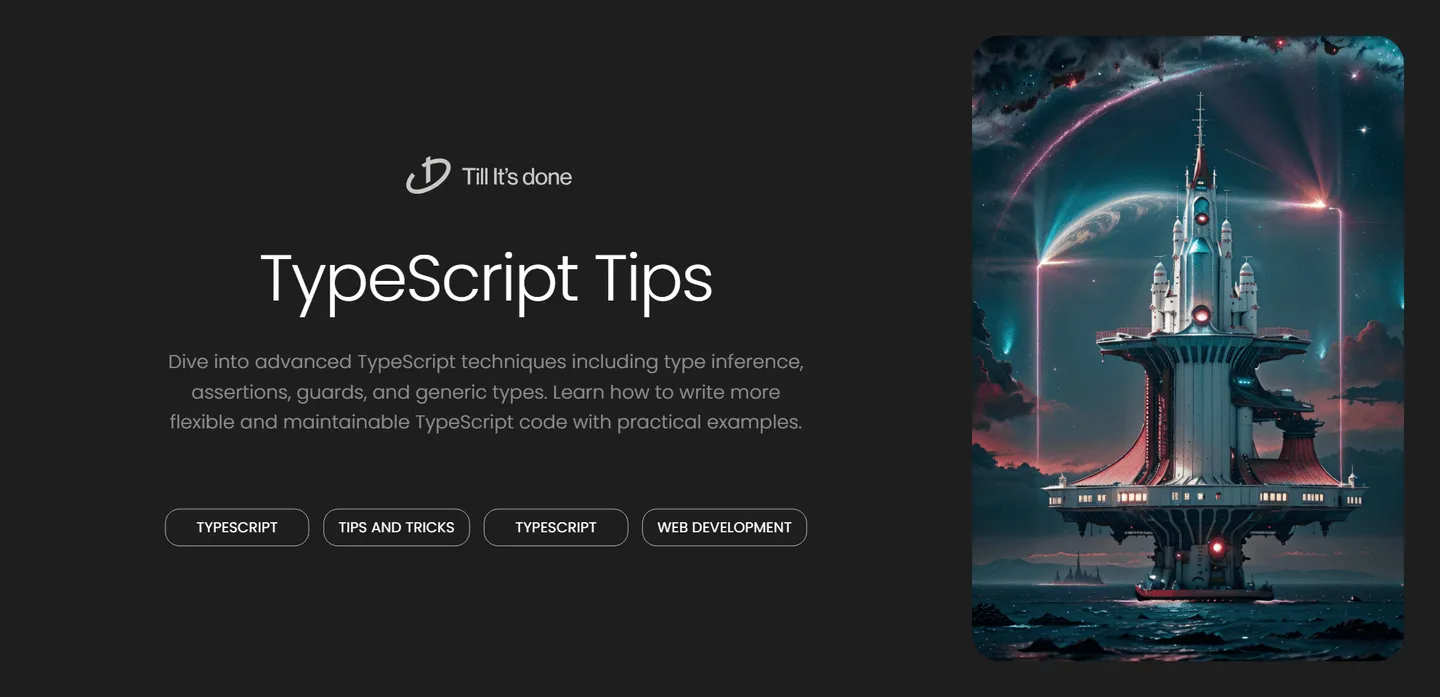
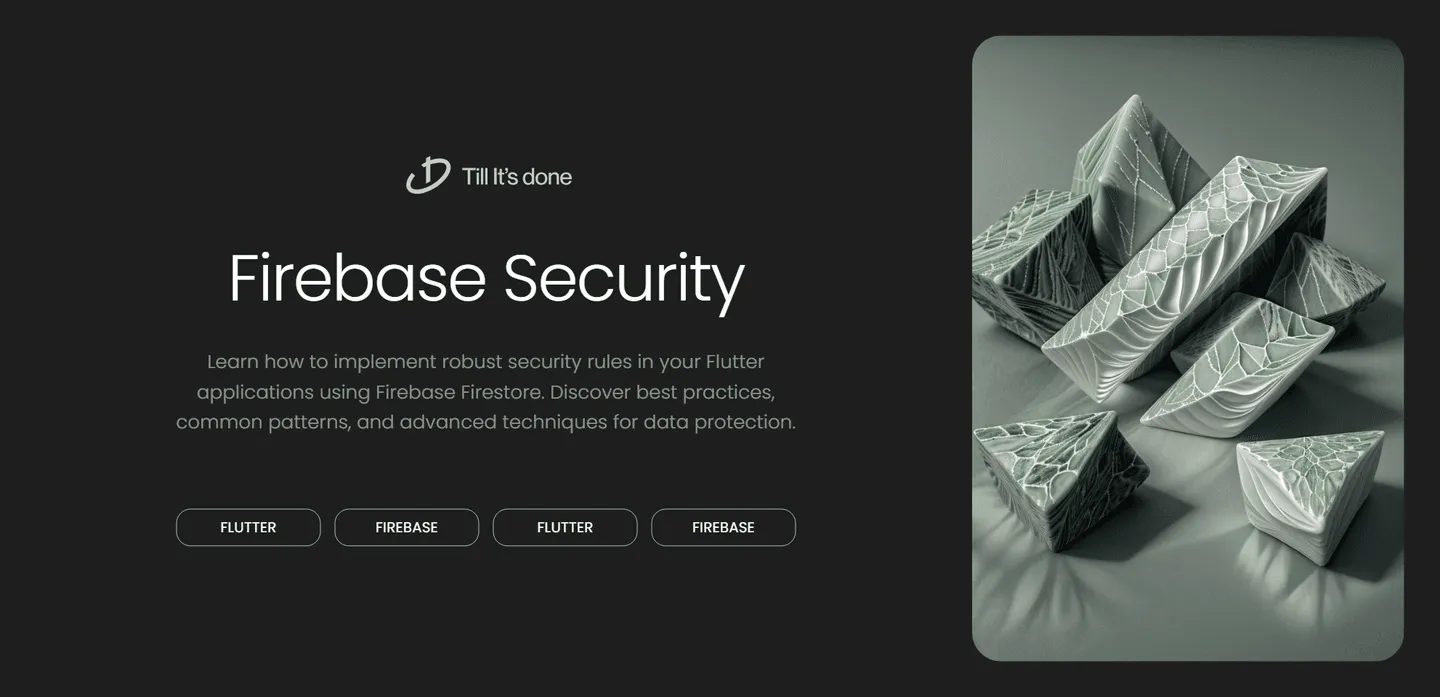
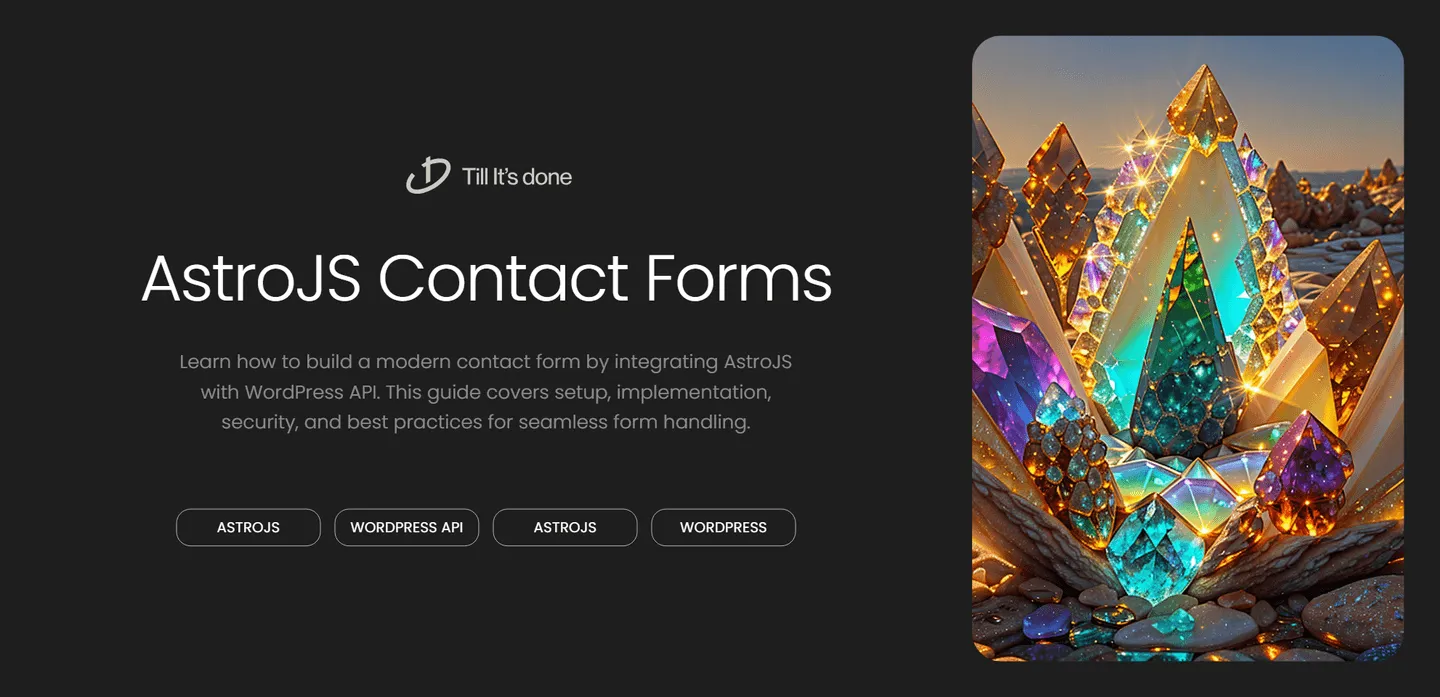
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.