- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Using Koa.js with TypeScript for Strong Typing
Explore middleware typing, error handling, and best practices for creating robust Node.js applications.
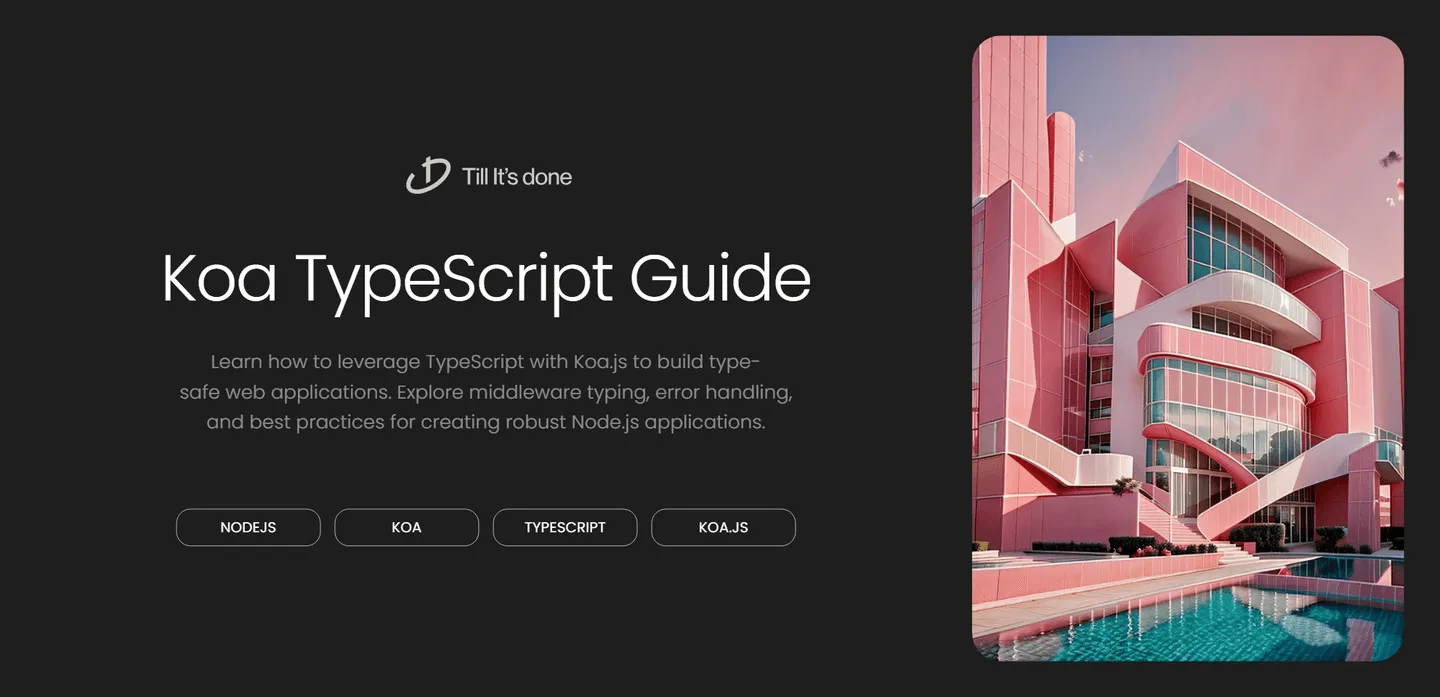
Using Koa.js with TypeScript for Strong Typing
TypeScript has become the go-to choice for developers seeking robust type safety in their Node.js applications. When combined with Koa.js, a modern and lightweight web framework, it creates a powerful foundation for building scalable and maintainable web applications. Let’s explore how to leverage TypeScript with Koa.js to create type-safe web applications.
Setting Up Your Project
First, let’s set up a new project with TypeScript and Koa. We’ll need to install the necessary dependencies:
npm init -ynpm install koa @koa/router koa-bodyparsernpm install --save-dev typescript @types/koa @types/koa__router @types/koa-bodyparser
Type-Safe Middleware
One of the biggest advantages of using TypeScript with Koa is the ability to create type-safe middleware. Here’s how we can define and use strongly-typed middleware:
interface CustomState { user: { id: number; name: string; };}
interface CustomContext extends Koa.Context { state: CustomState;}
const app = new Koa<CustomState>();
const authMiddleware: Koa.Middleware<CustomState> = async (ctx, next) => { ctx.state.user = { id: 1, name: "John Doe" }; await next();};
Error Handling with Type Safety
TypeScript helps us create more robust error handling by defining custom error types:
class ApplicationError extends Error { status: number; constructor(message: string, status: number = 500) { super(message); this.status = status; }}
app.use(async (ctx, next) => { try { await next(); } catch (err) { if (err instanceof ApplicationError) { ctx.status = err.status; ctx.body = { error: err.message }; } }});
Type-Safe Router Implementation
Implementing routes with TypeScript provides excellent autocompletion and type checking:
interface TodoItem { id: number; title: string; completed: boolean;}
const router = new Router<CustomState>();
router.get('/todos', async (ctx) => { const todos: TodoItem[] = [ { id: 1, title: 'Learn TypeScript', completed: false } ]; ctx.body = todos;});
Best Practices and Tips
- Always define interfaces for your request/response bodies
- Use type guards for runtime type checking
- Leverage TypeScript’s utility types for better type manipulation
- Keep your type definitions in separate files for better organization
- Use strict TypeScript configuration for maximum type safety
By following these practices, you’ll create more maintainable and reliable applications while catching potential errors during development rather than at runtime.
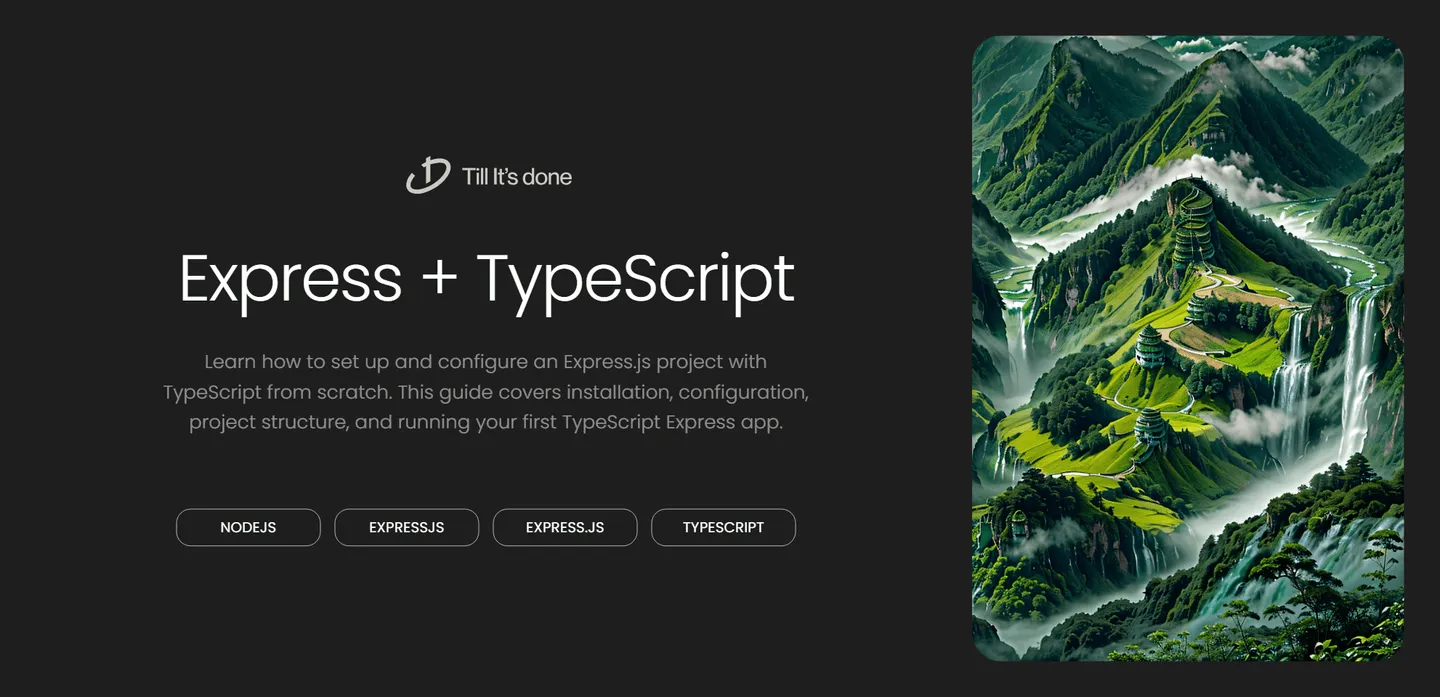
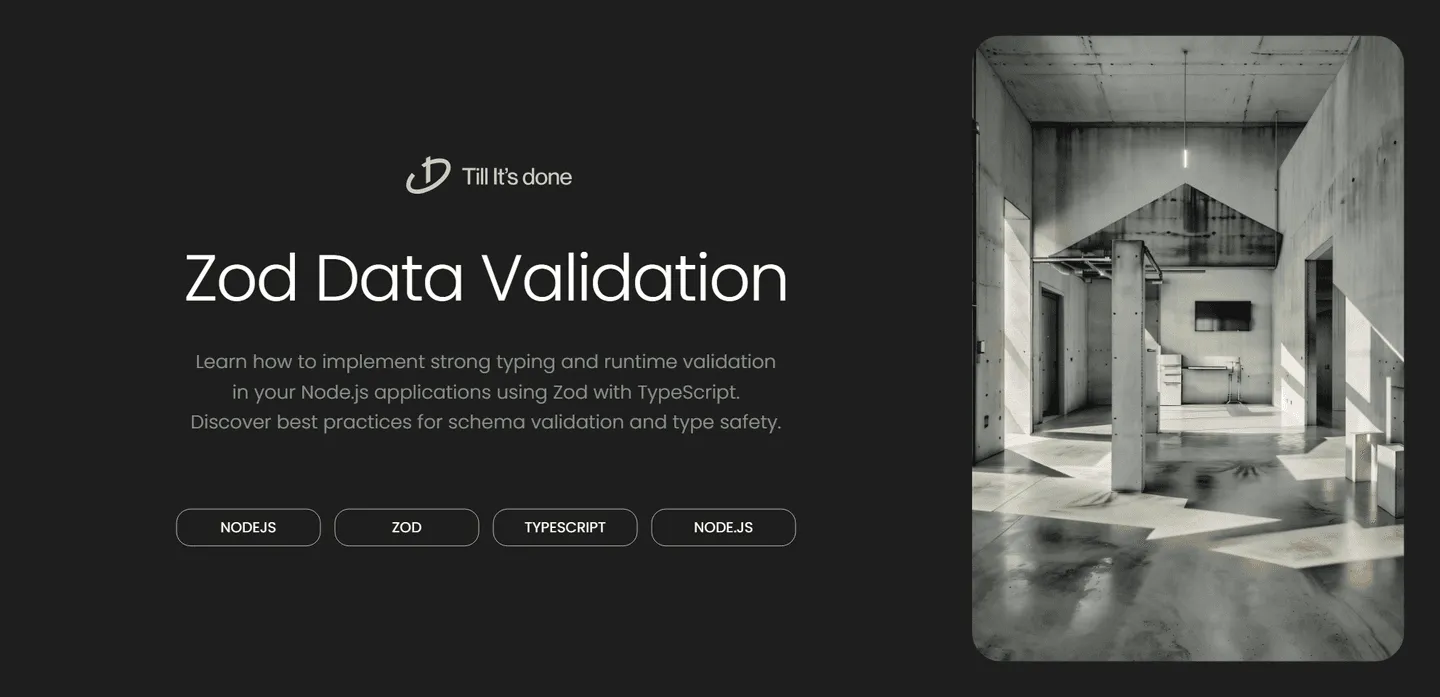

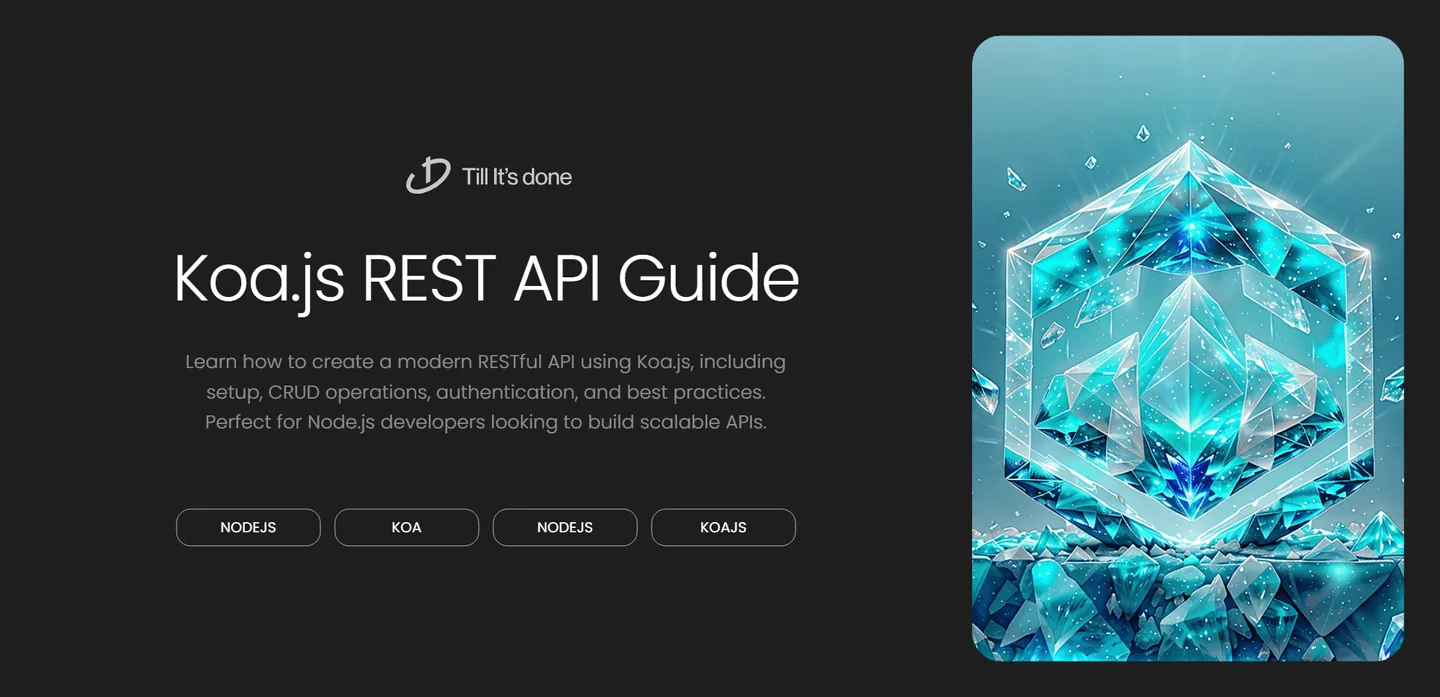


Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.