- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
EN
TH
rick@tillitsdone.com
+66824564755
How to Implement JWT Authentication in Chi API
Learn how to secure your Go API with JWT authentication using the Chi router.
This guide covers token generation, middleware creation, and best practices for implementing secure authentication.
This guide covers token generation, middleware creation, and best practices for implementing secure authentication.
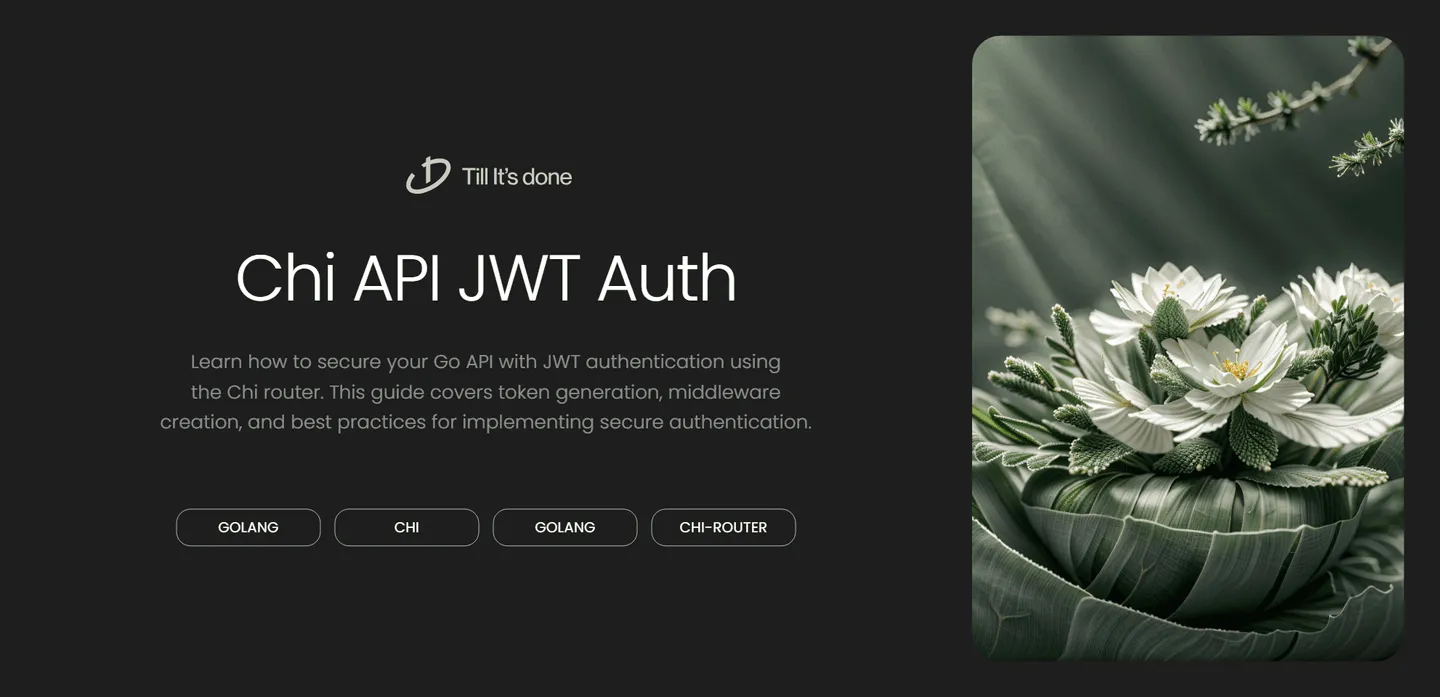
# Implementing JWT Authentication in a Chi API: A Practical Guide

Securing your Go web applications is crucial in today's digital landscape. In this guide, we'll walk through implementing JWT (JSON Web Token) authentication in a Chi router-based API. We'll create a robust authentication system that's both secure and scalable.
## Setting Up the Project
First, let's set up our project structure and install the necessary dependencies. We'll need the Chi router and a JWT package:
```gogo get -u github.com/go-chi/chi/v5go get -u github.com/golang-jwt/jwt/v5
Creating the JWT Middleware
The heart of our authentication system lies in the middleware. This code will verify incoming JWT tokens and protect our routes:
func JWTMiddleware(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { tokenString := r.Header.Get("Authorization") if tokenString == "" { http.Error(w, "Unauthorized", http.StatusUnauthorized) return }
token, err := jwt.Parse(tokenString, func(token *jwt.Token) (interface{}, error) { return []byte(os.Getenv("JWT_SECRET")), nil })
if err != nil || !token.Valid { http.Error(w, "Unauthorized", http.StatusUnauthorized) return }
next.ServeHTTP(w, r) })}
Implementing Login and Token Generation
When users authenticate, we’ll generate a JWT token containing their credentials:
func Login(w http.ResponseWriter, r *http.Request) { // Validate user credentials here
claims := jwt.MapClaims{ "user_id": user.ID, "exp": time.Now().Add(time.Hour * 24).Unix(), }
token := jwt.NewWithClaims(jwt.SigningMethodHS256, claims) tokenString, err := token.SignedString([]byte(os.Getenv("JWT_SECRET")))
if err != nil { http.Error(w, "Error generating token", http.StatusInternalServerError) return }
json.NewEncoder(w).Encode(map[string]string{ "token": tokenString, })}
Protecting Routes
Now we can protect our routes using the middleware:
func main() { r := chi.NewRouter()
// Public routes r.Post("/login", Login)
// Protected routes r.Group(func(r chi.Router) { r.Use(JWTMiddleware) r.Get("/protected", ProtectedHandler) })}
Best Practices and Security Considerations
- Always use environment variables for your JWT secret
- Set appropriate token expiration times
- Implement token refresh mechanisms
- Use secure password hashing for user credentials
- Consider implementing token blacklisting for logout
- Use HTTPS in production
By following these steps, you’ll have a secure JWT authentication system in your Chi API. The middleware pattern makes it easy to protect routes and manage user sessions effectively.
Discover our top articles, selected to support the growth of your business.
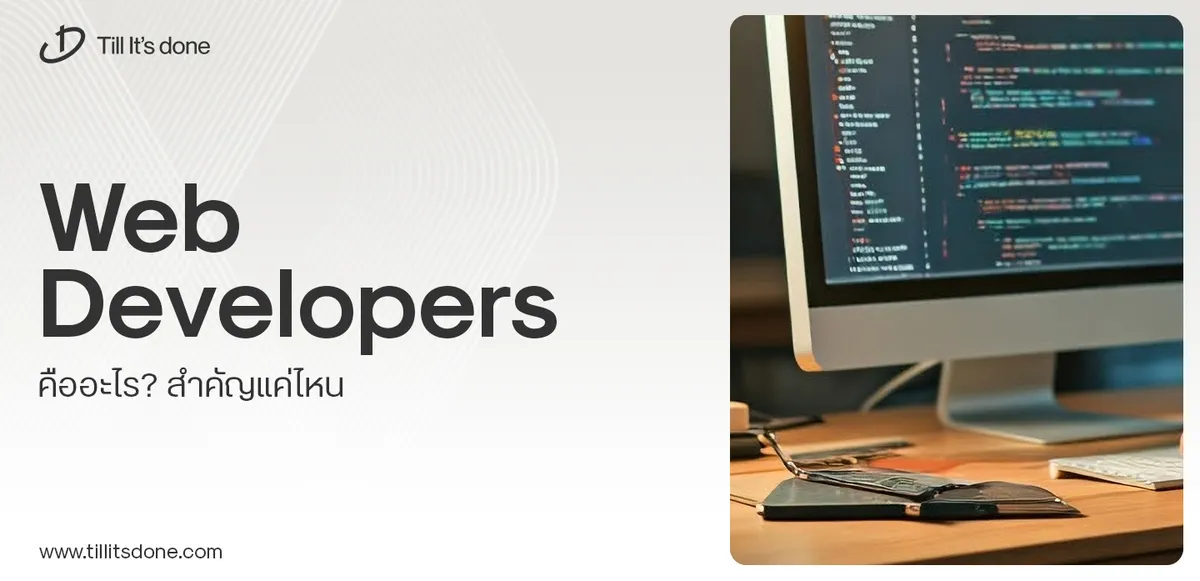
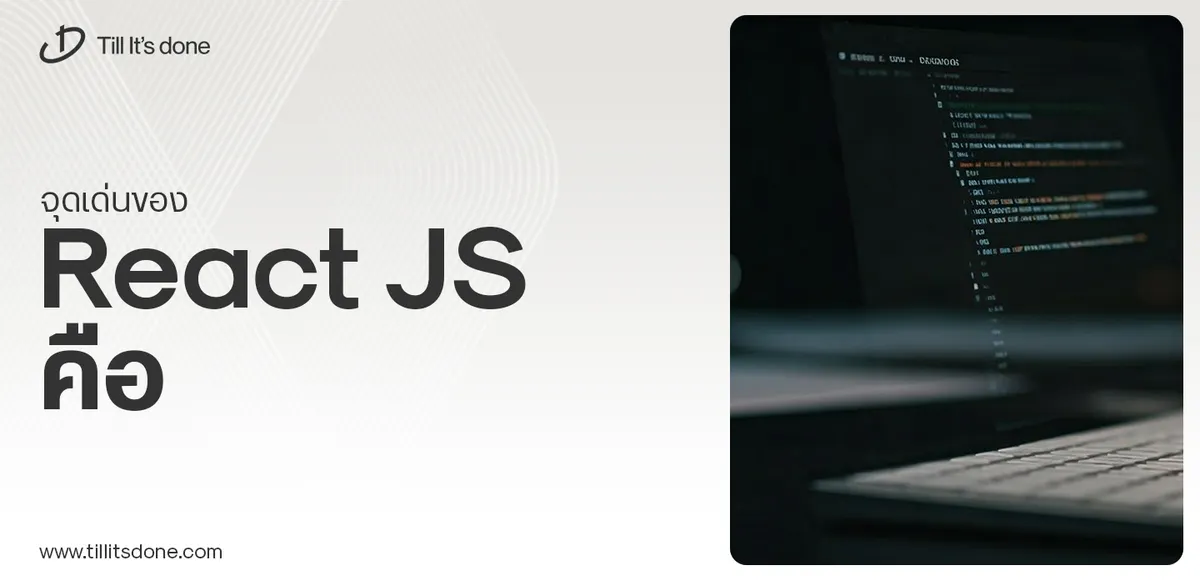
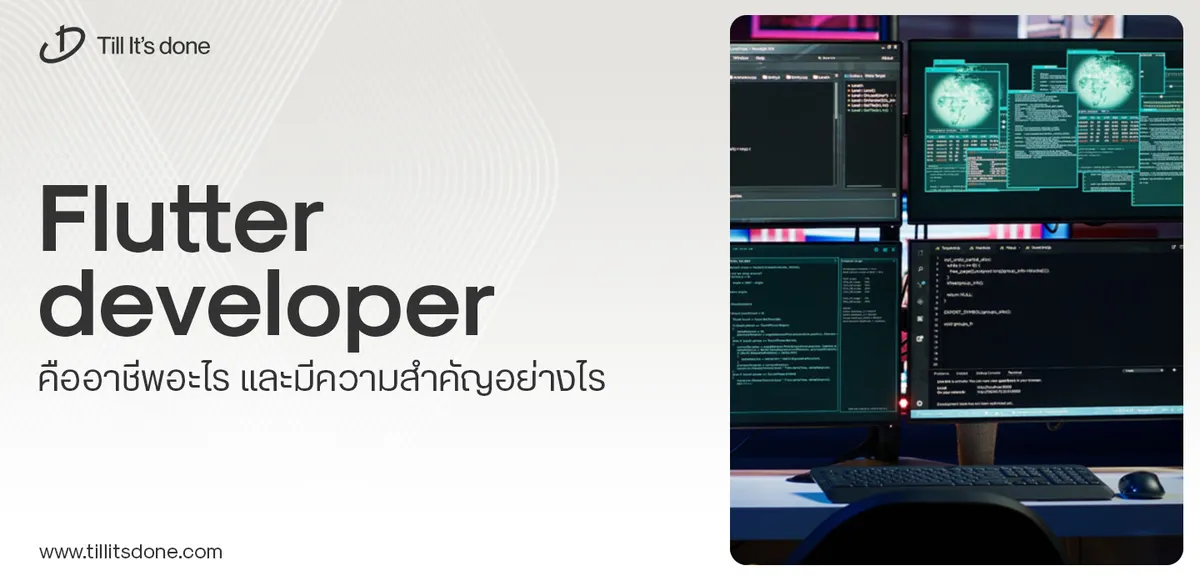
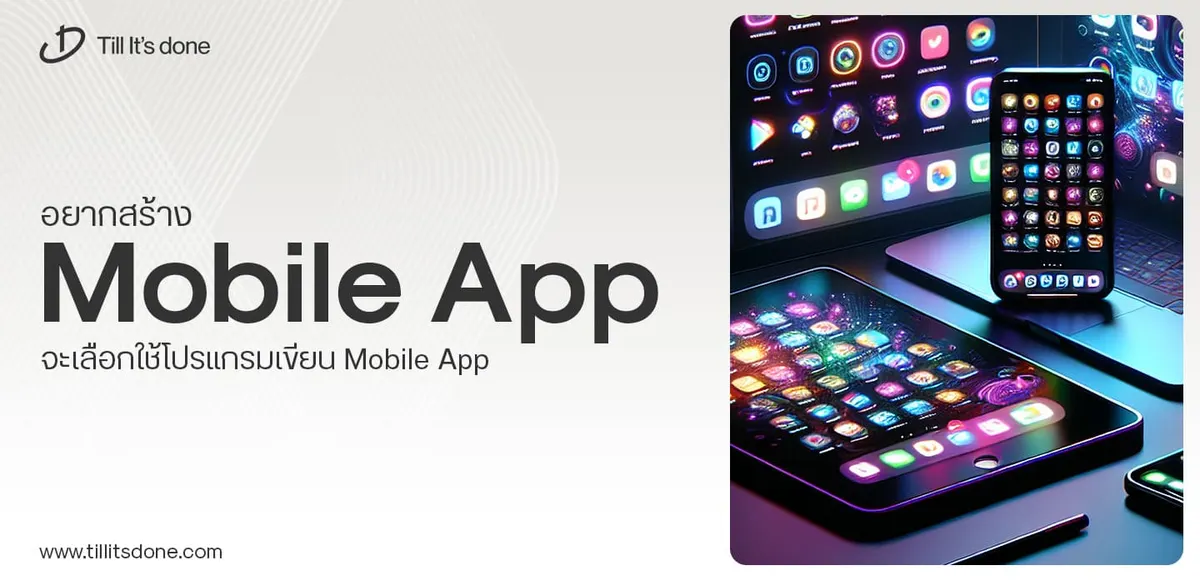
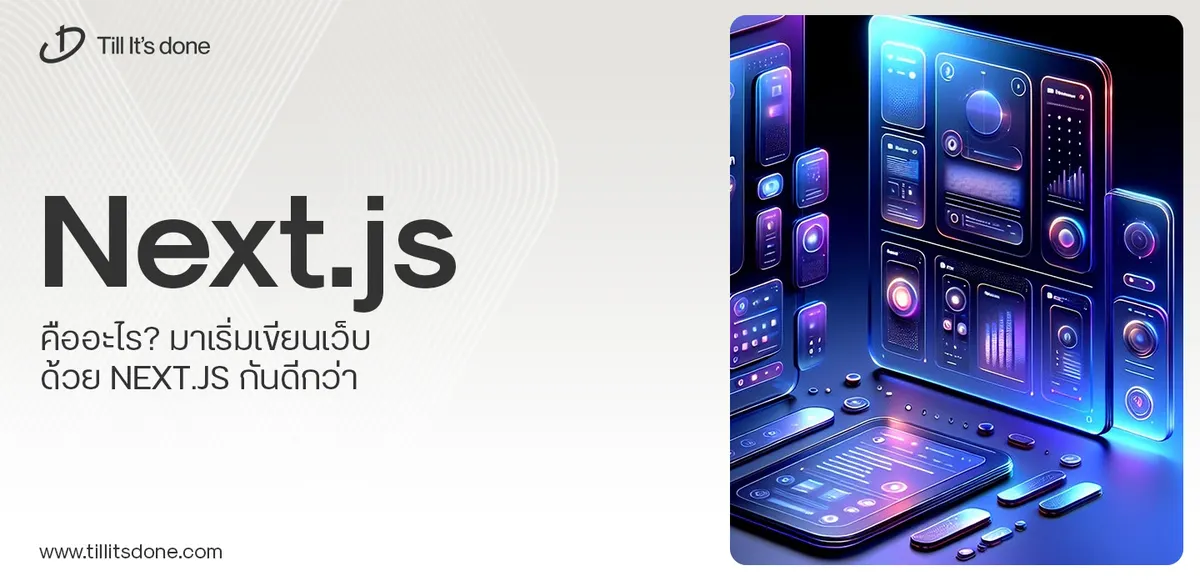
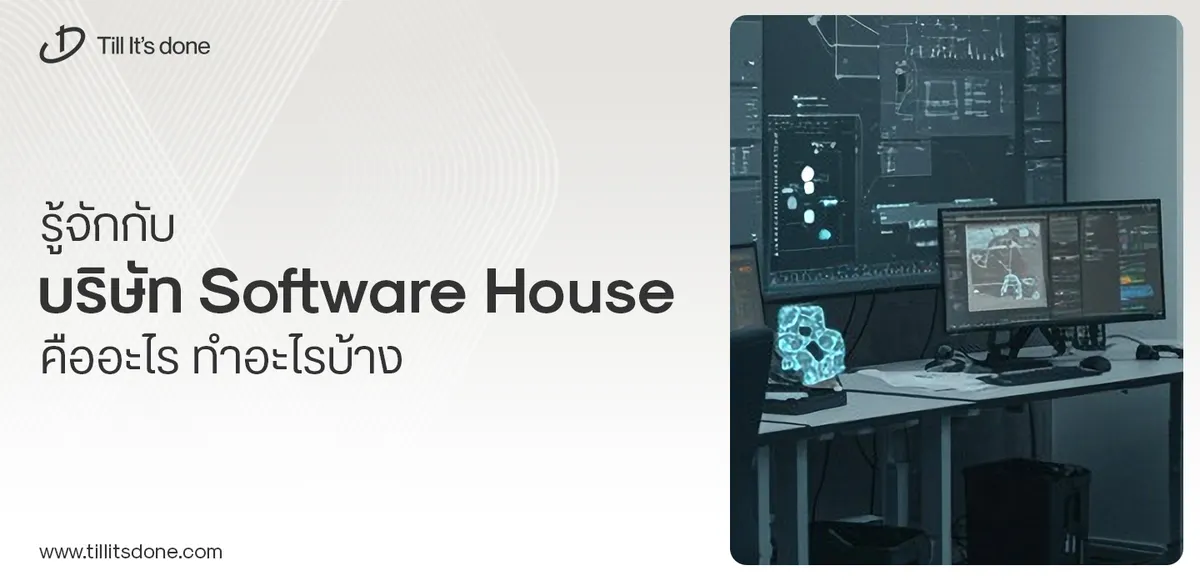
Talk with CEO
Ready to bring your web/app to life or boost your team with expert Thai developers?
Contact us today to discuss your needs, and let’s create tailored solutions to achieve your goals. We’re here to help at every step!
🖐️ Contact us 196 Articles
Explore Popular JavaScript library for building user interfaces with a component-based architecture.
160 Articles
Explore UI toolkit for building natively compiled applications for mobile, web, and desktop from a single codebase.
144 Articles
Explore JavaScript runtime for building scalable, high-performance server-side applications.
58 Articles
Explore React framework enabling server-side rendering and static site generation for optimized performance.
38 Articles
Explore Utility-first CSS framework for rapid UI development.
36 Articles
Explore Superset of JavaScript adding static types for improved code quality and maintainability.
126 Articles
Explore Programming language known for its simplicity, concurrency model, and performance.
67 Articles
Explore Astro is an all-in-one web framework. It includes everything you need to create a website, built-in.
38 Articles
Explore Versatile testing framework for JavaScript applications supporting various test types.
5 Articles
Explore 4 Articles
Explore 4 Articles
Explore 2 Articles
Explore 1 Articles
Explore 1 Articles
Explore 337 Articles
Explore CSS3 is the latest version of Cascading Style Sheets, offering advanced styling features like animations, transitions, shadows, gradients, and responsive design.
Let's keep in Touch
Thank you for your interest in Tillitsdone! Whether you have a question about our services, want to discuss a potential project, or simply want to say hello, we're here and ready to assist you.
We'll be right here with you every step of the way.
We'll be right here with you every step of the way.
Contact Information
rick@tillitsdone.com+66824564755
Address
9 Phahonyothin Rd, Khlong Nueng, Khlong Luang District, Pathum Thani, Bangkok Thailand
Social media
FacebookInstagramLinkedIn
We anticipate your communication and look forward to discussing how we can contribute to your business's success.
We'll be here, prepared to commence this promising collaboration.
We'll be here, prepared to commence this promising collaboration.
Frequently Asked Questions
Explore frequently asked questions about our products and services.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.