- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Using Jest with Babel for ES6+ Code Testing
This guide covers setup, configuration, and best practices for writing tests with modern syntax.
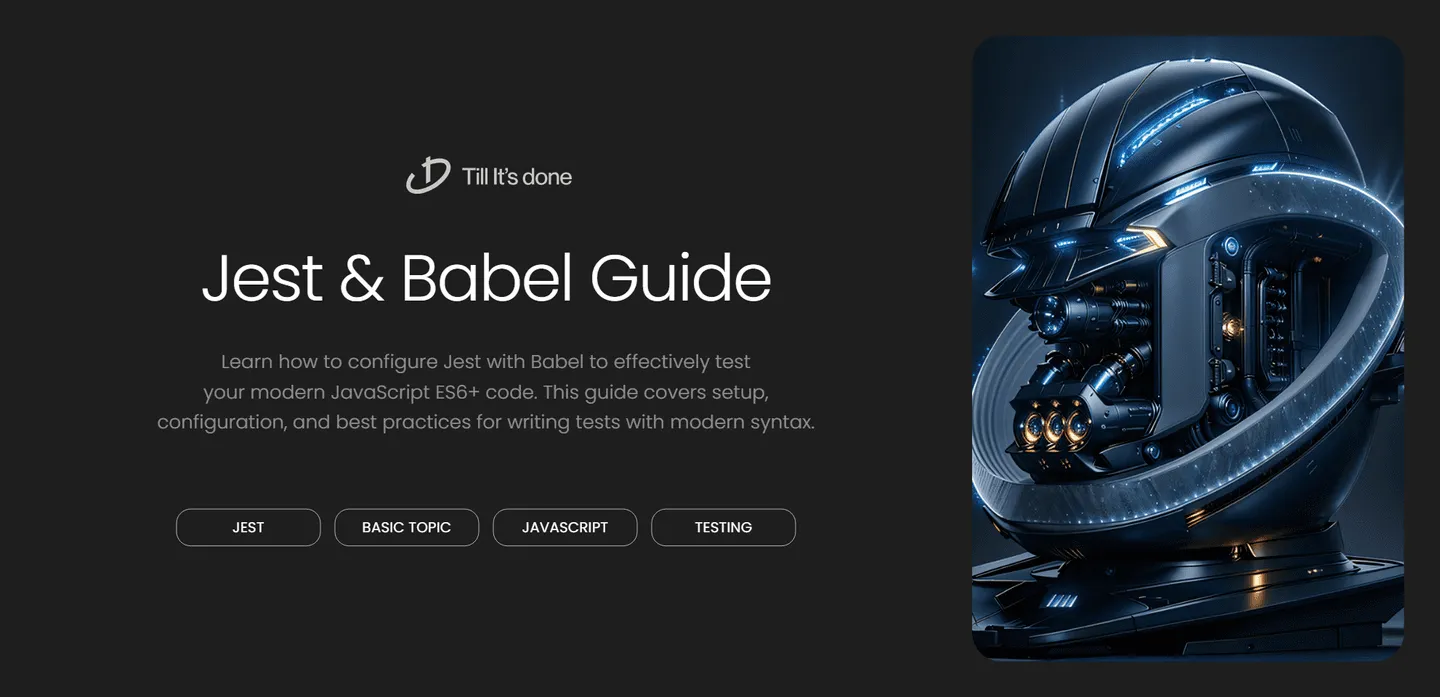
Using Jest with Babel for ES6+ Code
If you’ve been writing modern JavaScript, you’re probably using ES6+ features like arrow functions, classes, and modules. But when it comes to testing this code with Jest, you might run into some compatibility issues. Don’t worry – I’ll show you how to set up Jest with Babel to test your ES6+ code smoothly.
Why Do We Need Babel with Jest?
Jest runs in Node.js environment, which might not support all the latest JavaScript features out of the box. That’s where Babel comes in – it transforms our modern JavaScript code into a version that Jest can understand and execute.
Setting Up Your Project
First things first, let’s get our dependencies in order. You’ll need to install a few packages:
npm install --save-dev jest babel-jest @babel/core @babel/preset-env
Now, create a babel.config.js
file in your project root. This tells Babel how to transform your code:
module.exports = { presets: [['@babel/preset-env', {targets: {node: 'current'}}]],};
Writing Tests with ES6+ Features
With our setup complete, we can now write tests using modern JavaScript features. Here’s a simple example:
export class Calculator { add = (a, b) => a + b; subtract = (a, b) => a - b;}
// math.test.jsimport { Calculator } from './math';
describe('Calculator', () => { const calc = new Calculator();
test('adds numbers correctly', () => { expect(calc.add(5, 3)).toBe(8); });
test('subtracts numbers correctly', () => { expect(calc.subtract(10, 4)).toBe(6); });});
Advanced Configuration
Sometimes you might need more specific Babel configurations. For instance, if you’re using TypeScript or other experimental features, you can add more presets or plugins:
module.exports = { presets: [ ['@babel/preset-env', {targets: {node: 'current'}}], '@babel/preset-typescript', ], plugins: ['@babel/plugin-proposal-class-properties']};
Tips for Better Testing
- Use ES6 modules (
import
/export
) consistently - Take advantage of arrow functions for cleaner test syntax
- Utilize ES6+ features like destructuring and template literals in your tests
- Remember that async/await is fully supported with this setup
That’s it! You’re now equipped to write modern, clean tests for your ES6+ code. Happy testing! 🚀
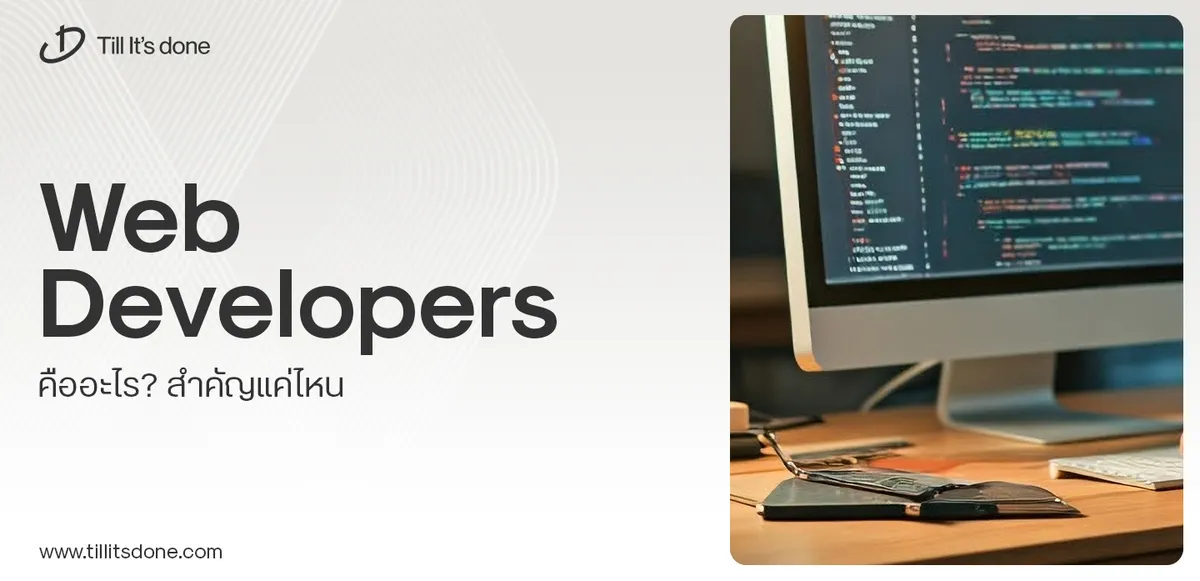
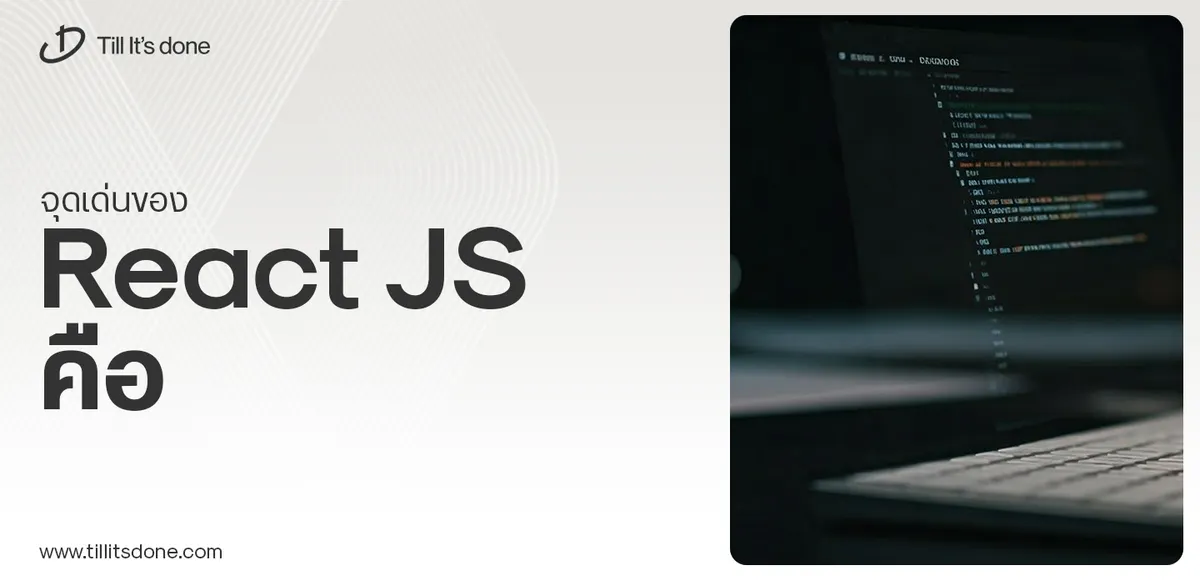
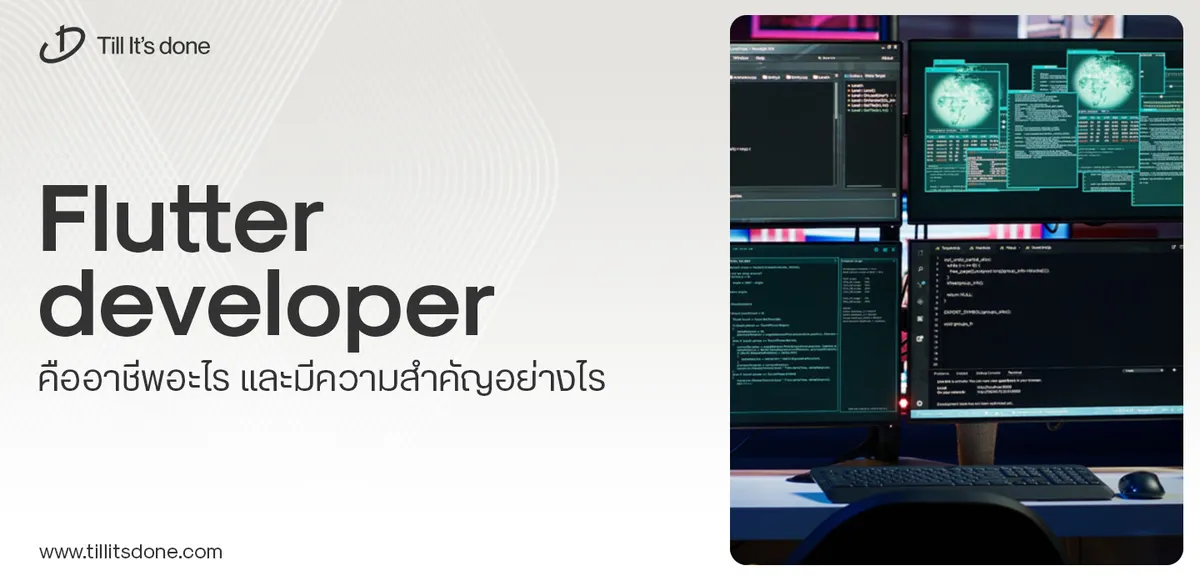
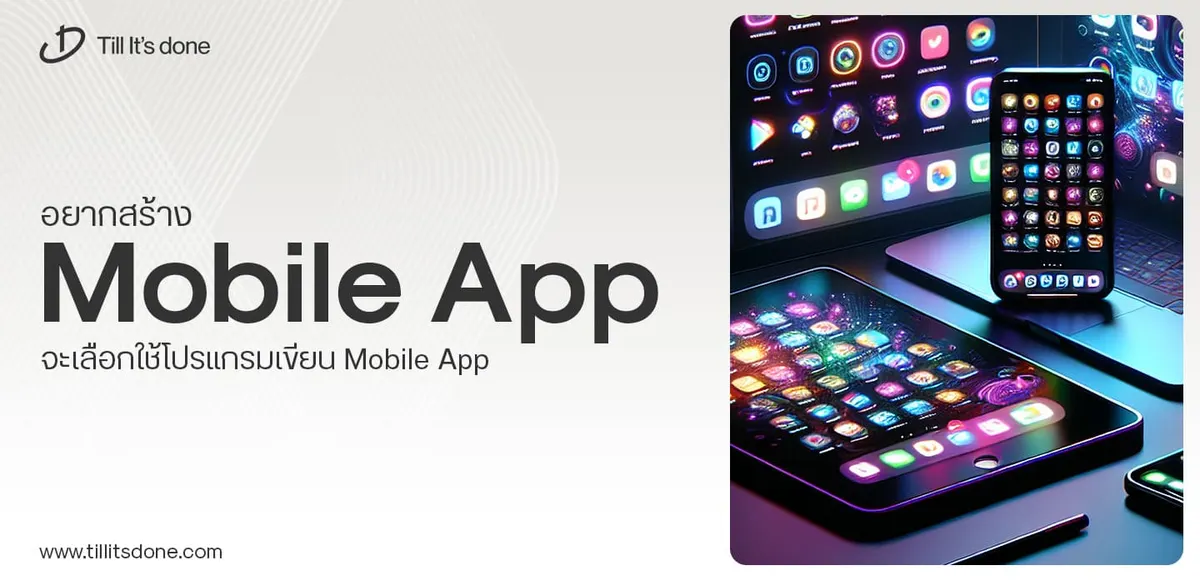
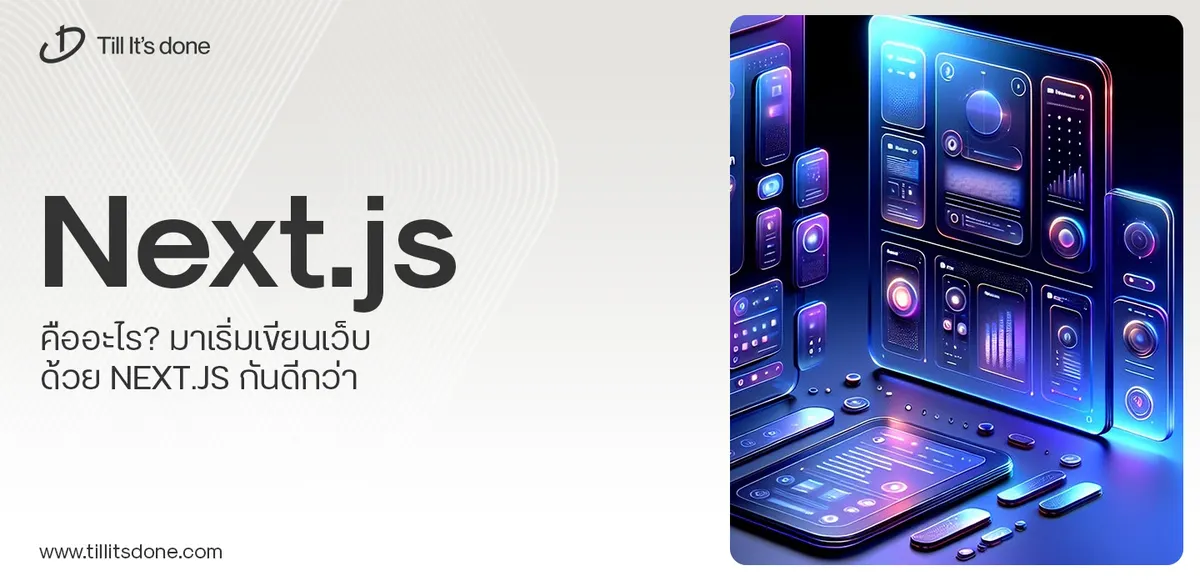
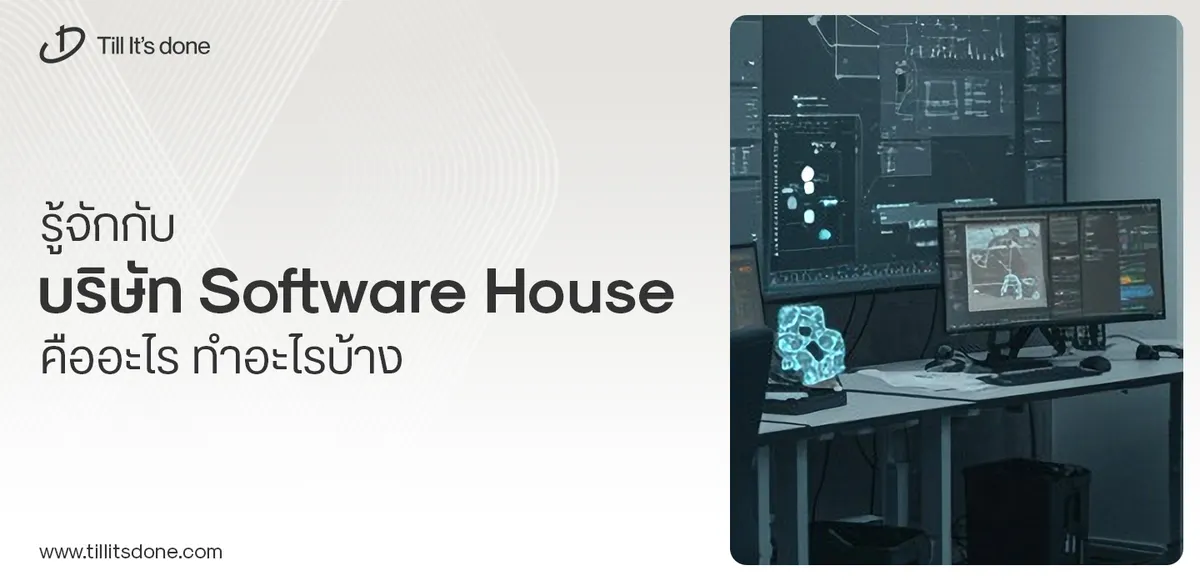
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.