- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Optimize Jest Performance for Large Codebases
Discover parallel execution, smart filtering, and advanced caching techniques to dramatically improve your testing workflow.
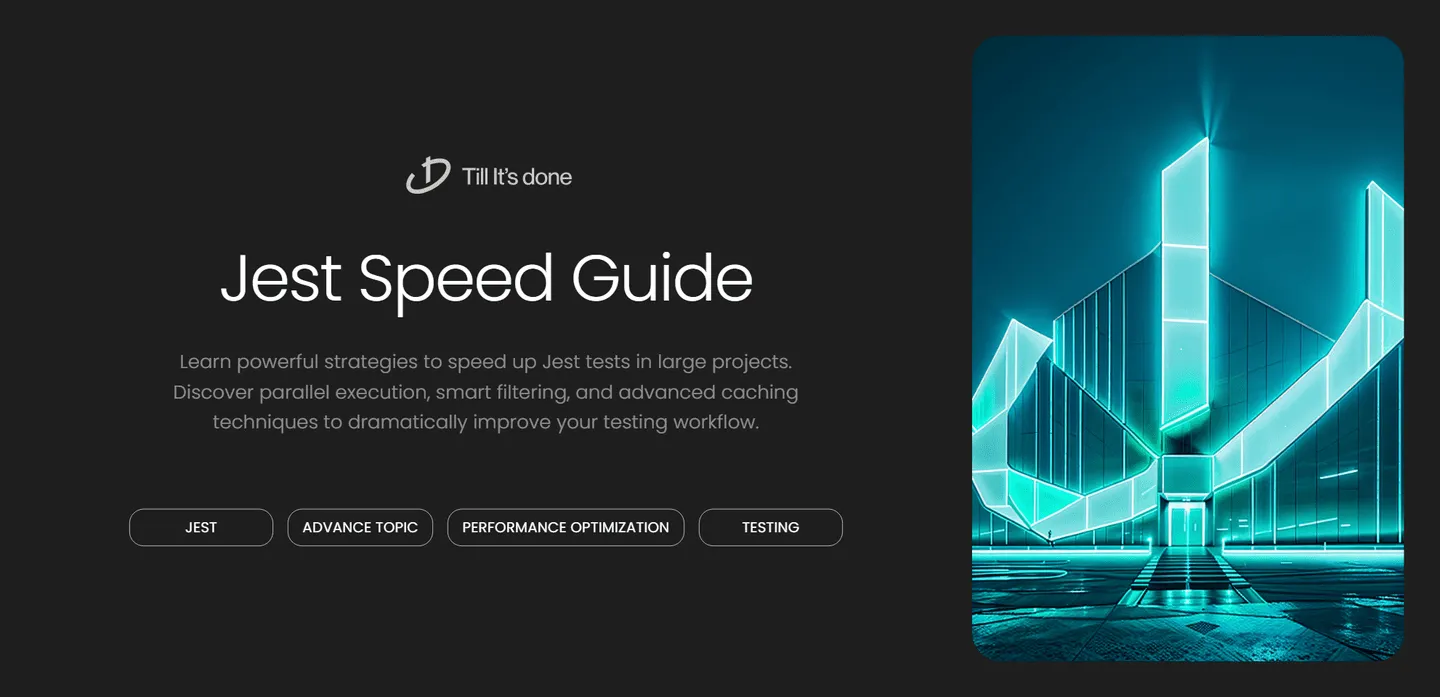
Optimizing Jest Performance for Large Codebases
As your codebase grows, running tests can become painfully slow. I’ve been there – watching Jest tests crawl by while your productivity grinds to a halt. After optimizing test suites across several large-scale projects, I’ve discovered some game-changing strategies to supercharge Jest’s performance.
Understanding the Performance Bottlenecks
Before diving into solutions, let’s identify what makes Jest slow in large codebases. The main culprits are usually:
- Large amounts of test files being processed
- Heavy setup/teardown operations
- Complex module dependencies
- Resource-intensive operations in tests
- Unoptimized configuration settings
Key Optimization Strategies
1. Parallel Test Execution
One of the most impactful changes you can make is maximizing Jest’s parallel execution capabilities. By default, Jest runs tests in parallel, but you can fine-tune this behavior:
module.exports = { maxWorkers: '50%', workerIdleMemoryLimit: '2GB'};
2. Smart Test Filtering
When working on specific features, running the entire test suite isn’t always necessary. I’ve found these approaches particularly useful:
# Run only changed filesjest --onlyChanged
# Run tests related to specific filesjest --findRelatedTests path/to/changed/file.js
# Run tests matching a patternjest -t "auth"
3. Optimizing the Test Environment
The test environment setup can significantly impact performance. Here’s what works well:
module.exports = { testEnvironment: 'node', setupFilesAfterEnv: ['./jest.setup.js'], timers: 'modern'};
4. Mocking Strategies
Strategic mocking can dramatically improve test execution speed. I’ve developed these best practices:
// Efficient module mockingjest.mock('./heavyModule', () => ({ heavyOperation: jest.fn().mockReturnValue('result')}));
// Using manual mocks// __mocks__/heavy-computation.jsmodule.exports = jest.fn().mockReturnValue('result');
5. Caching and Watch Mode
Leverage Jest’s built-in caching mechanisms:
module.exports = { cache: true, cacheDirectory: '.jest-cache'};
6. Resource-Intensive Operations
For operations that are expensive to set up:
// Use beforeAll instead of beforeEach when possiblebeforeAll(async () => { // Setup expensive resources once await setupDatabase();});
// Clean beforeEach hooksbeforeEach(() => { // Only reset what's necessary jest.clearAllMocks();});
Advanced Techniques
Custom Transformers
When dealing with non-standard files, custom transformers can be more efficient:
module.exports = { process(sourceText, sourcePath) { return { code: transformedCode }; }};
Test Sharding
For extremely large test suites, consider implementing test sharding:
# Running tests in shardsjest --shard=1/3
Monitoring and Maintenance
Regular performance monitoring is crucial. Use Jest’s built-in timing data:
jest --verbose
This helps identify slow tests that need optimization.
Remember, optimization is an ongoing process. Regular maintenance and monitoring will keep your test suite running efficiently as your codebase continues to grow.
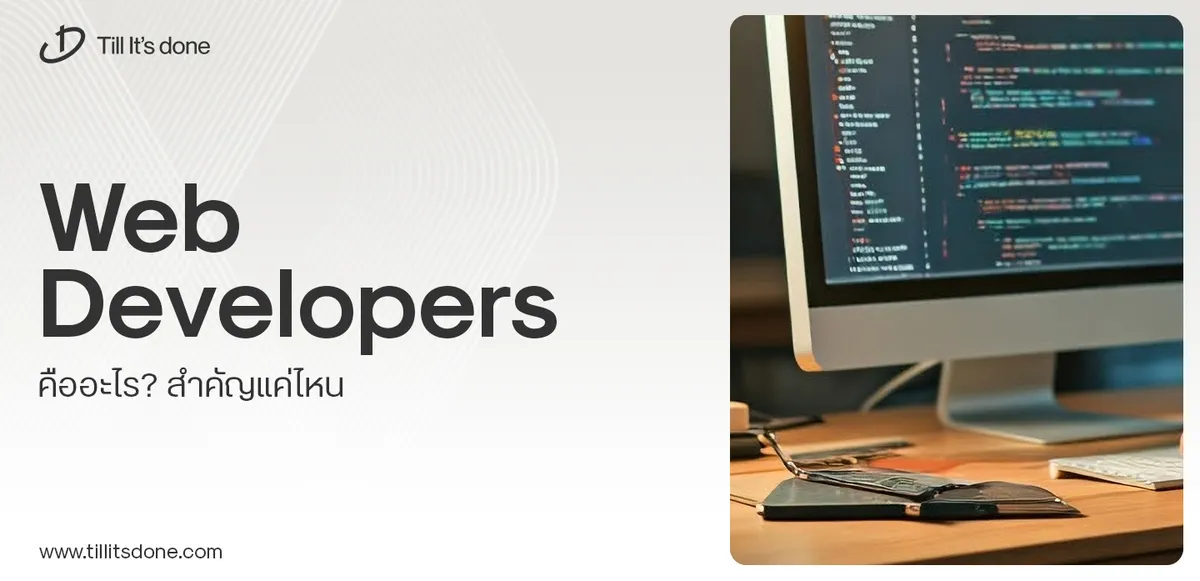
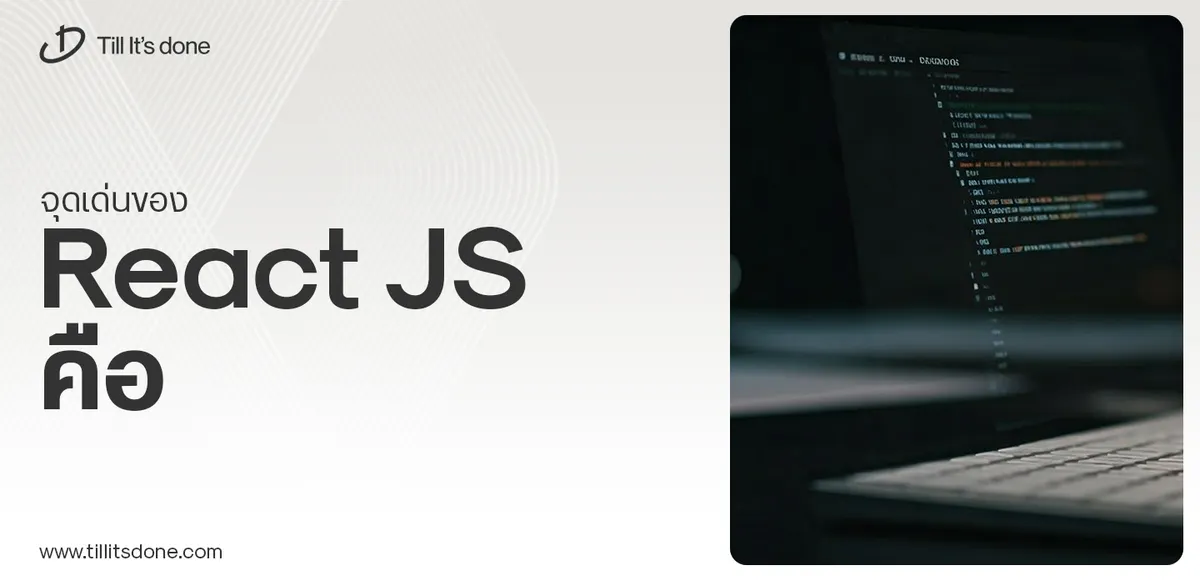
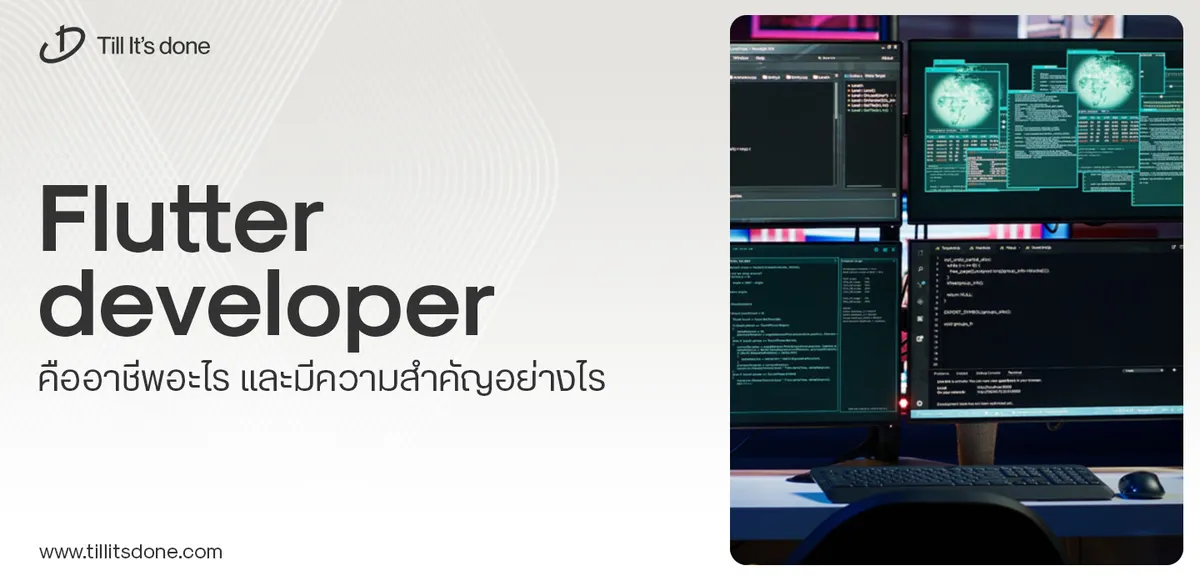
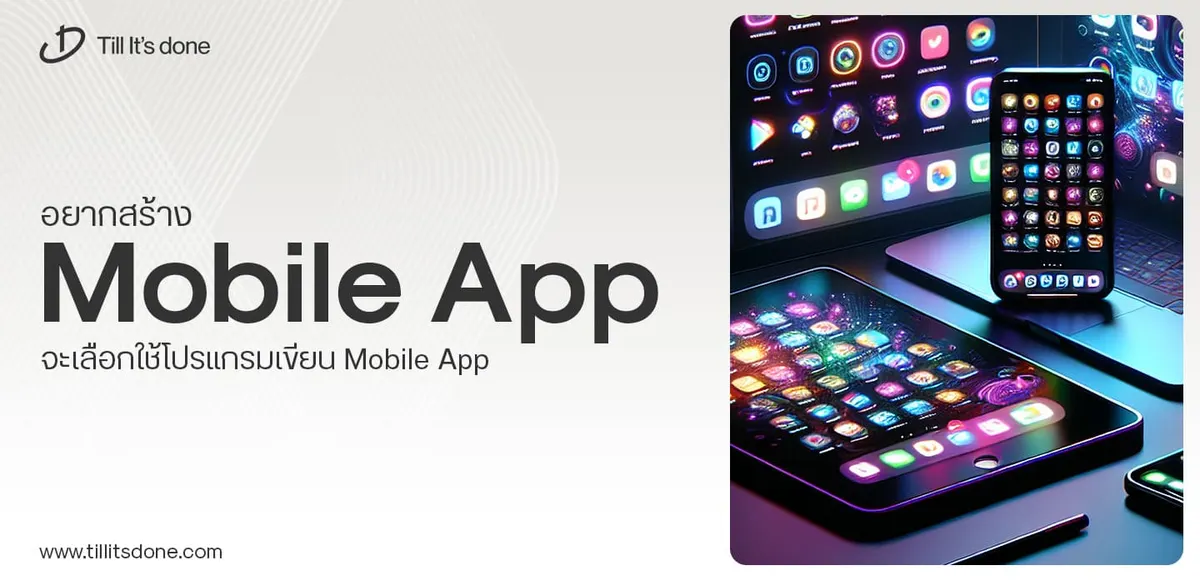
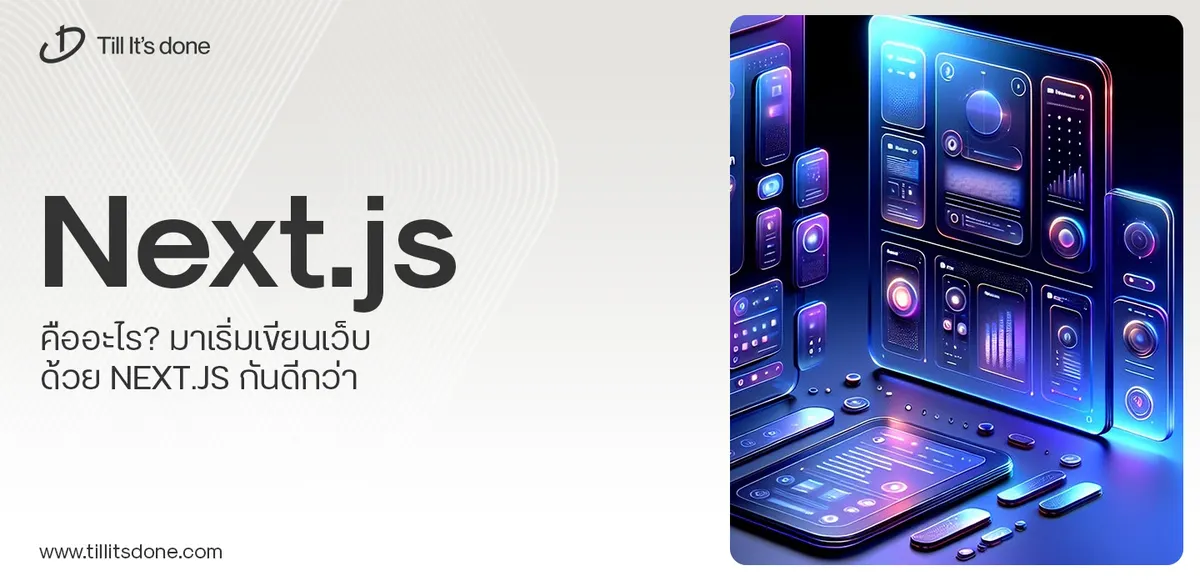
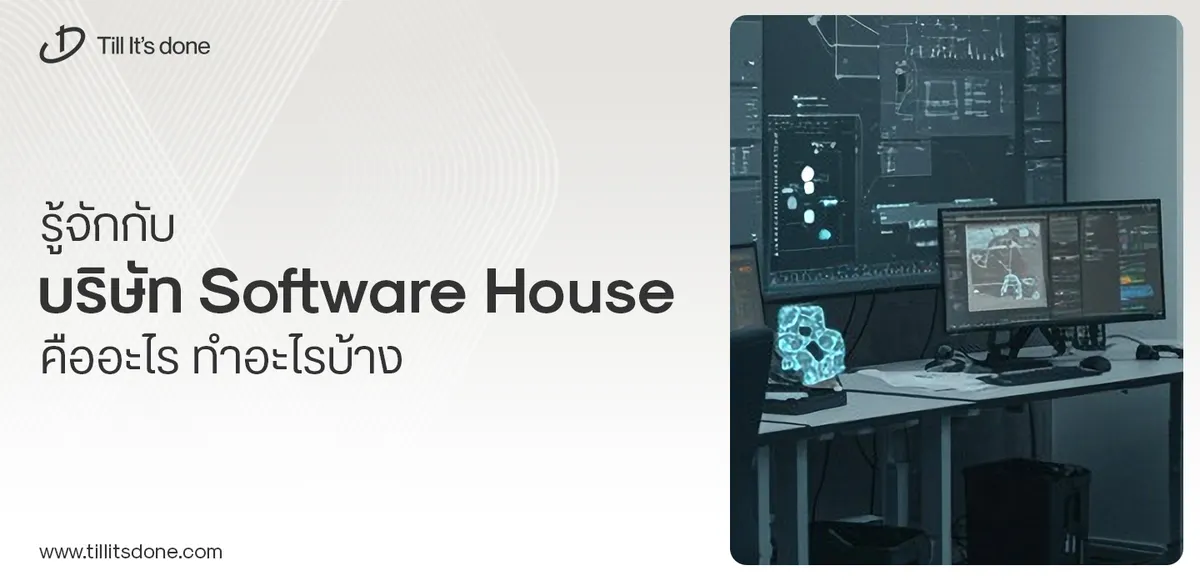
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.