- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Mastering Jest Hooks: Before and After Guide
Discover best practices, common patterns, and avoid pitfalls in test setup and teardown.
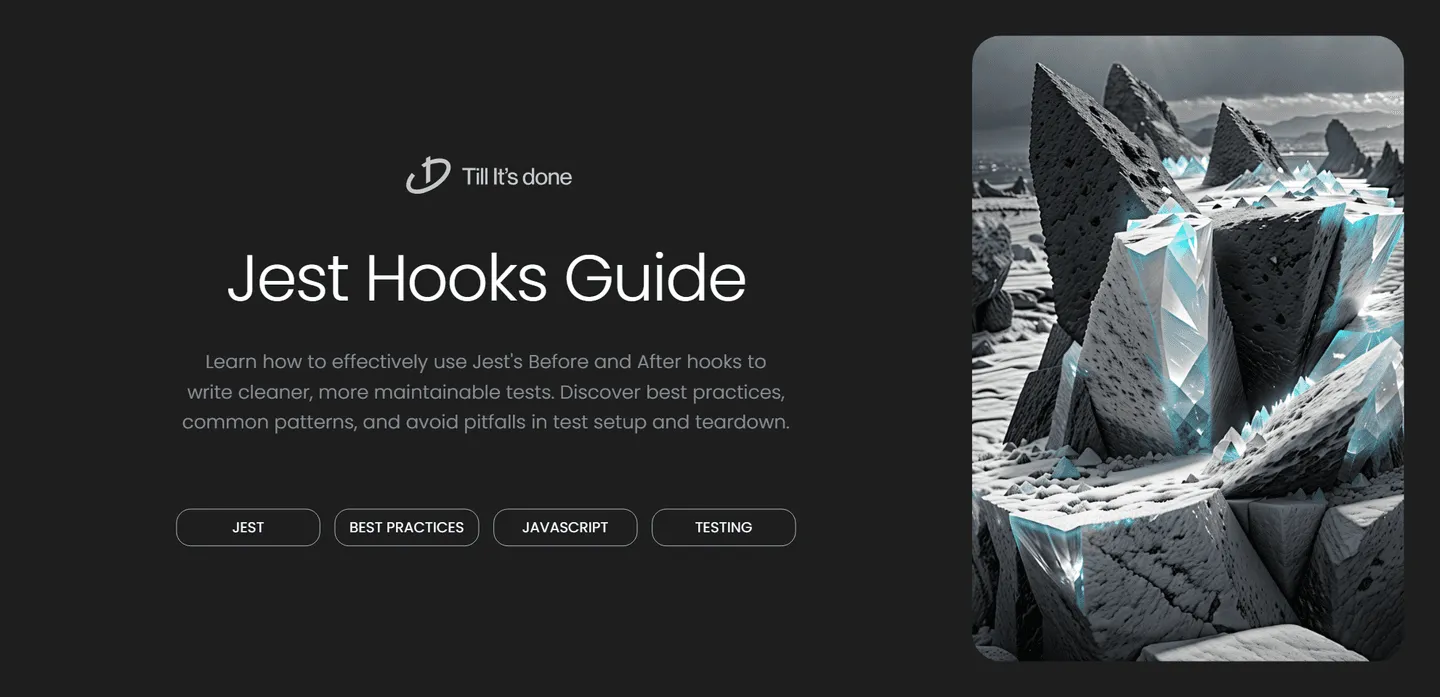
Effective Use of Before/After Hooks in Jest
Testing is an art, and like any masterpiece, it requires the right tools and techniques. One of the most powerful features in Jest is its suite of hooks - beforeAll, beforeEach, afterAll, and afterEach. Let’s dive into how we can leverage these hooks effectively to write cleaner, more maintainable tests.
Understanding the Basics
Before and After hooks in Jest are like the stagehands of a theater production - they set up and clean up behind the scenes, ensuring everything runs smoothly. These hooks help us maintain a clean testing environment and reduce code duplication.
Common Use Cases
Database Connections
One of the most common use cases for hooks is managing database connections. Here’s how you might structure your tests:
let db;
beforeAll(async () => { db = await connect();});
afterAll(async () => { await db.close();});
test('should save user to database', async () => { // Your test here});
State Reset
For tests that modify shared state, beforeEach and afterEach are your best friends:
let user;
beforeEach(() => { user = { name: 'John Doe', email: 'john@example.com' };});
test('should update user name', () => { user.name = 'Jane Doe'; expect(user.name).toBe('Jane Doe');});
test('should have original name', () => { expect(user.name).toBe('John Doe');});
Best Practices
-
Keep Hooks Simple Your hooks should be focused and straightforward. If you find yourself writing complex logic in hooks, consider moving that logic into helper functions.
-
Scope Your Hooks Appropriately Use describe blocks to scope your hooks. This helps maintain clarity about which setup and teardown code applies to which tests.
-
Clean Up After Yourself Always clean up any resources you’ve created. This includes closing database connections, clearing mocks, and resetting any modified global state.
-
Use Mock Restoration When working with mocks, take advantage of Jest’s automatic mock restoration in combination with hooks:
const mock = jest.fn();
beforeEach(() => { mock.mockClear();});
test('should call mock once', () => { mock(); expect(mock).toHaveBeenCalledTimes(1);});
Advanced Patterns
Sometimes you need more complex setup and teardown logic. Here’s a pattern for handling nested hooks:
describe('User API', () => { beforeAll(() => { // Setup for all user tests });
describe('authentication', () => { beforeEach(() => { // Setup specifically for auth tests });
test('should authenticate valid user', () => { // Test code }); });});
Common Pitfalls to Avoid
- Don’t make hooks dependent on test execution order
- Avoid sharing state between tests unless absolutely necessary
- Keep async operations properly handled with async/await
- Don’t skip cleanup in afterEach/afterAll hooks
Remember, the goal of hooks is to make your tests more maintainable and reliable. Use them wisely, and they’ll serve as powerful allies in your testing arsenal.
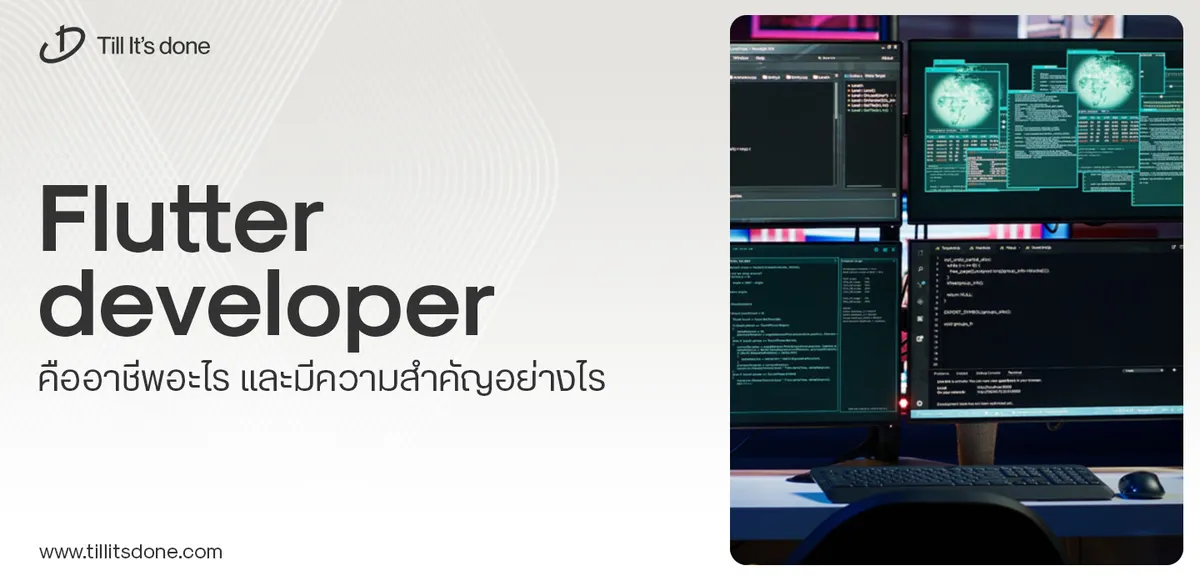
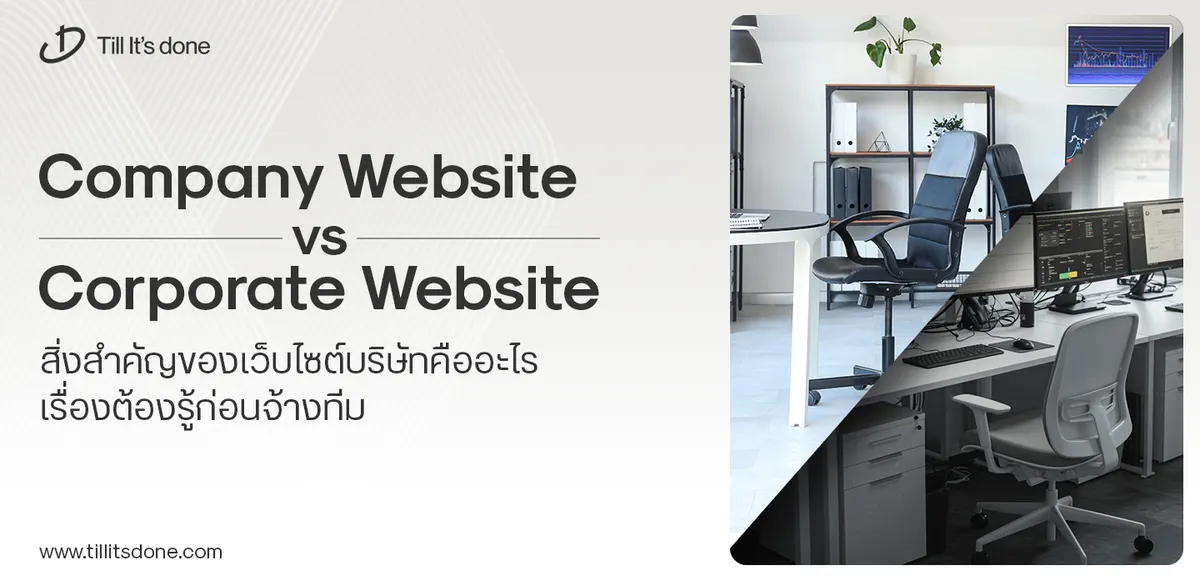
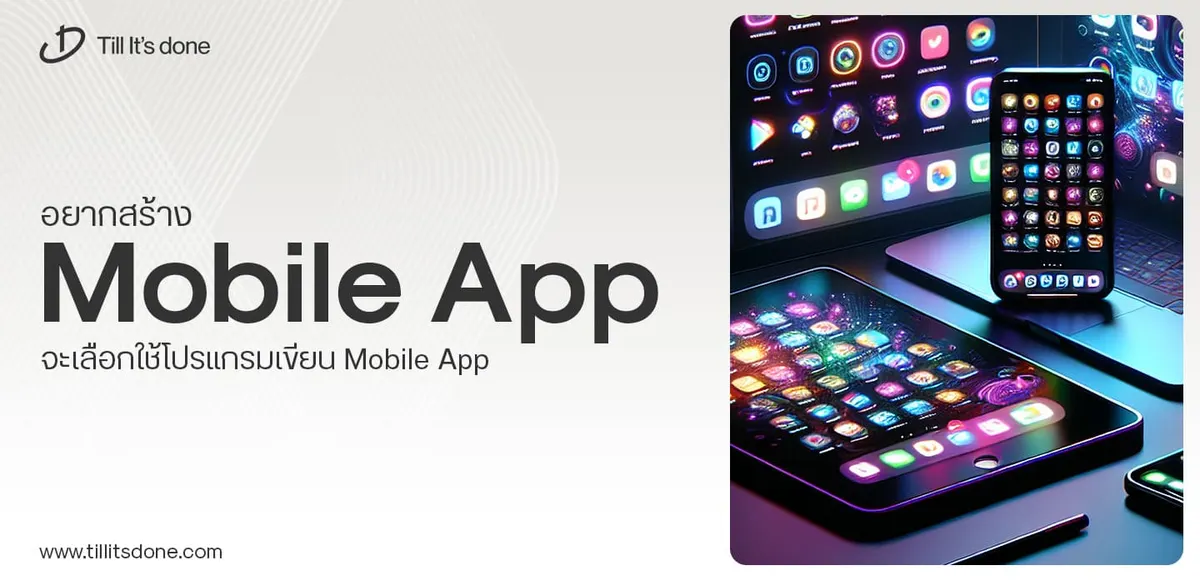
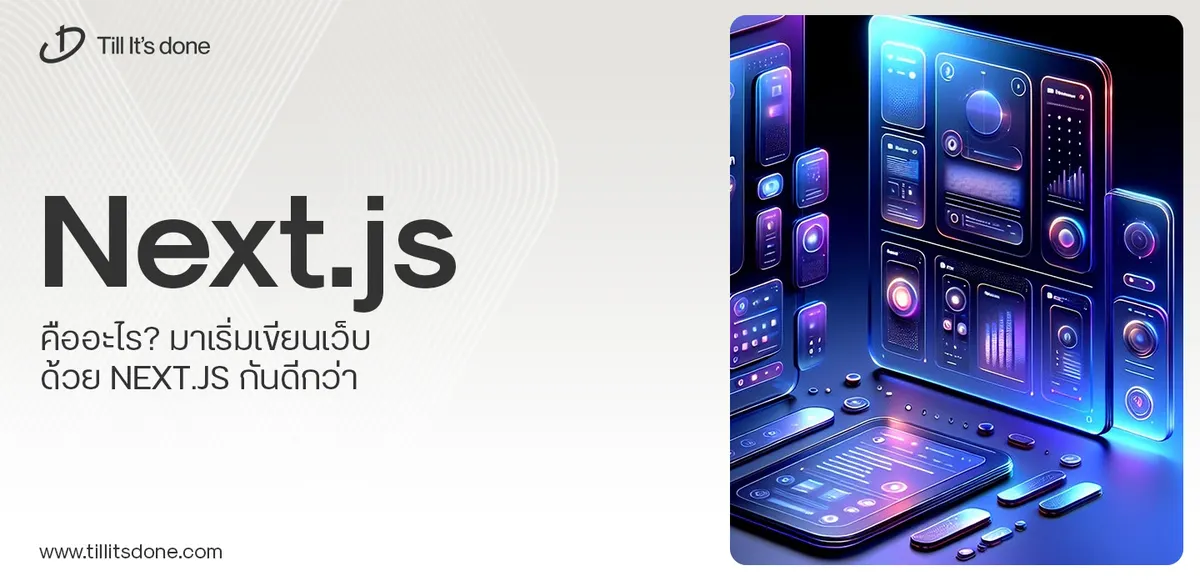
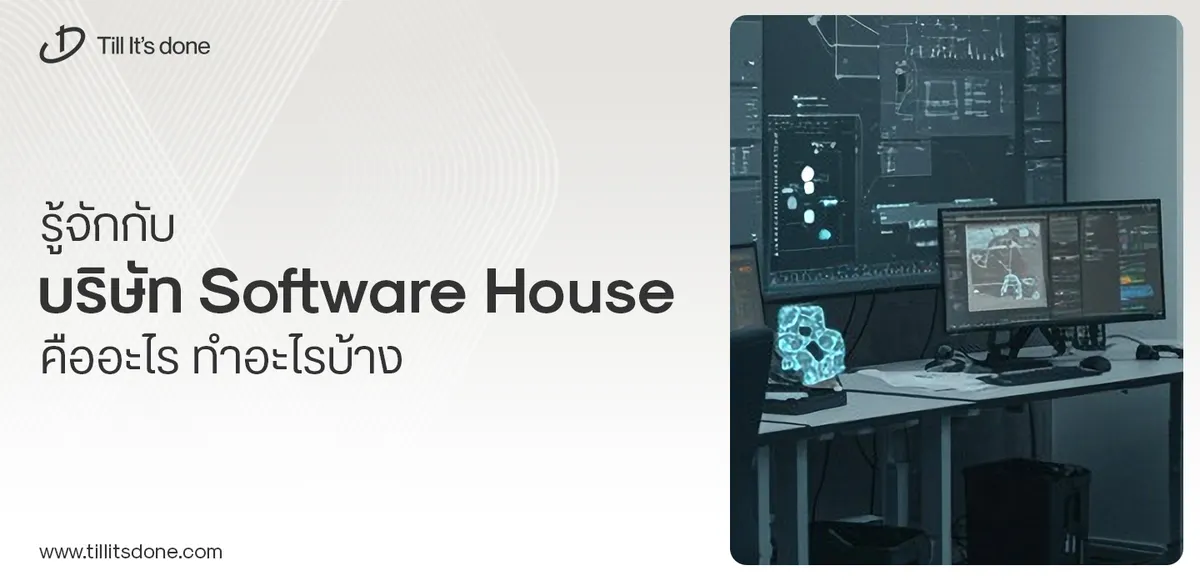
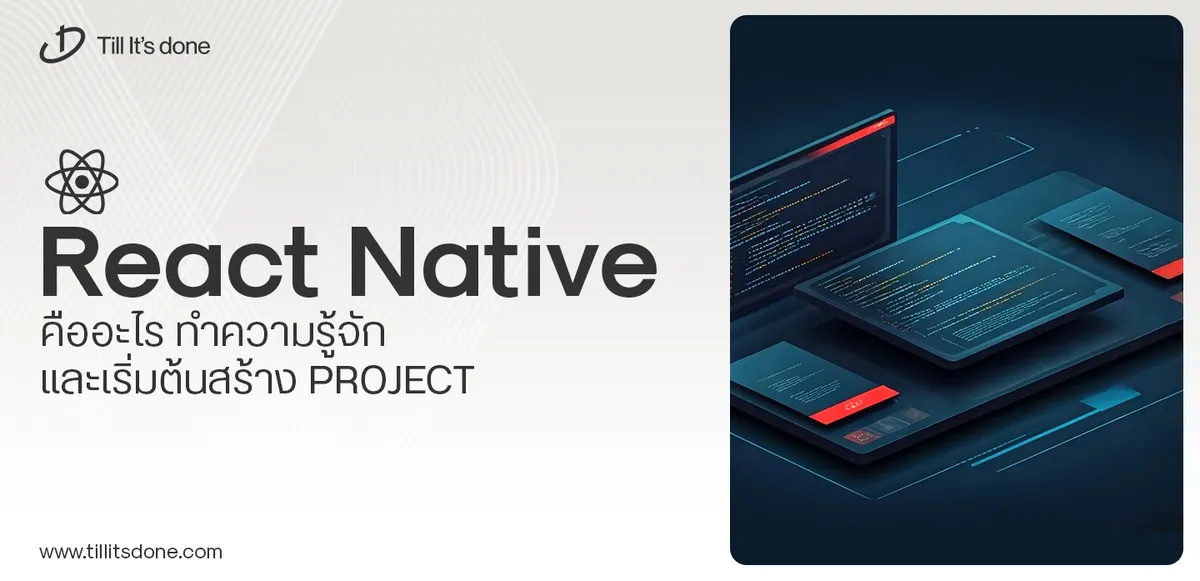
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.