- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Master Database Transactions with GORM
Explore basic and advanced transaction patterns, best practices, and common pitfalls to build reliable applications.

A Comprehensive Guide to Transactions in GORM
Database transactions are crucial for maintaining data integrity in modern applications. When working with Go and GORM, understanding how to properly implement transactions can make the difference between a robust application and one prone to data inconsistencies. Let’s dive deep into GORM transactions and explore their practical applications.
Understanding Transactions in GORM
Transactions ensure that a series of database operations either complete successfully as a single unit or fail entirely, leaving the database unchanged. Think of it as an all-or-nothing operation – perfect for scenarios where data consistency is paramount.
Basic Transaction Usage
GORM provides several ways to work with transactions. Let’s explore each approach with practical examples.
Method 1: Transaction Blocks
func CreateOrder(db *gorm.DB) error { return db.Transaction(func(tx *gorm.DB) error { order := Order{ UserID: 1, Items: []Item{ {ProductID: 1, Quantity: 2}, {ProductID: 2, Quantity: 1}, }, }
if err := tx.Create(&order).Error; err != nil { return err }
// Update inventory if err := tx.Model(&Product{}). Where("id = ?", 1). Update("stock", gorm.Expr("stock - ?", 2)).Error; err != nil { return err }
return nil })}
Method 2: Manual Transaction Management
func ProcessPayment(db *gorm.DB) error { tx := db.Begin()
defer func() { if r := recover(); r != nil { tx.Rollback() } }()
if err := tx.Error; err != nil { return err }
// Perform operations if err := tx.Create(&Payment{Amount: 100}).Error; err != nil { tx.Rollback() return err }
return tx.Commit().Error}
Advanced Transaction Patterns
Nested Transactions
While GORM doesn’t support true nested transactions, it provides SavePoint functionality:
func ComplexOperation(db *gorm.DB) error { return db.Transaction(func(tx *gorm.DB) error { // Main transaction operations if err := tx.Create(&MainRecord{}).Error; err != nil { return err }
// Savepoint tx.SavePoint("before_nested")
if err := riskyOperation(tx); err != nil { tx.RollbackTo("before_nested") // Continue with other operations }
return nil })}
Transaction Hooks
GORM allows you to register callbacks for transaction events:
db.Callback().Create().After("gorm:create").Register("after_create", func(db *gorm.DB) { if db.Statement.Table == "users" { // Perform additional operations after user creation }})
Best Practices and Common Pitfalls
- Always use transactions for operations that modify multiple records
- Keep transactions as short as possible to avoid long-running locks
- Handle errors properly and ensure proper rollback
- Be cautious with external API calls within transactions
- Use appropriate isolation levels for your use case
When to Use Transactions
- Payment processing
- Order management
- User registration with related records
- Inventory management
- Data migration operations
Remember that while transactions provide data consistency, they come with performance overhead. Use them judiciously and always test your transaction logic thoroughly in a staging environment before deploying to production.
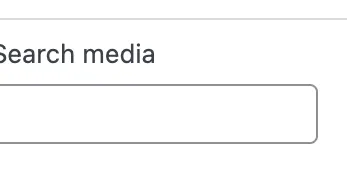





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.