- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Optimizing Performance with GORM: Fast Queries
From proper indexing to batch operations, discover how to make your database operations blazingly fast.

Optimizing Performance with GORM: Tips for Faster Queries
GORM is a fantastic ORM for Golang, but like any tool, it needs to be used wisely to achieve optimal performance. Let’s dive into some proven strategies to supercharge your GORM queries and make your applications blazingly fast.
Understanding Index Usage
One of the most fundamental aspects of database optimization is proper indexing. When using GORM, creating the right indexes can dramatically improve query performance. Instead of letting your database scan through every record, indexes act as shortcuts to find the data you need.
type User struct { gorm.Model Email string `gorm:"index"` Name string Age int `gorm:"index:idx_age"`}
Eager Loading to Prevent N+1 Problems
The N+1 query problem is a common performance killer. It occurs when you fetch a record and then execute additional queries for each related record. GORM provides elegant solutions through eager loading.
// Instead of this (N+1 problem)var users []Userdb.Find(&users)for _, user := range users { var orders []Order db.Model(&user).Association("Orders").Find(&orders)}
// Do this (Eager loading)var users []Userdb.Preload("Orders").Find(&users)
Batch Operations for Better Performance
When dealing with multiple records, batch operations can significantly reduce database load and improve performance.
// Create records in batchesvar users []Userdb.CreateInBatches(users, 100)
// Update in batchesdb.Table("users").Where("age > ?", 18).Updates(map[string]interface{}{"verified": true})
Query Optimization Techniques
Select Specific Fields
Only retrieve the fields you need instead of fetching entire records:
var users []Userdb.Select("name", "age").Find(&users)
Use Caching Wisely
Implement caching for frequently accessed data that doesn’t change often:
type CachedUser struct { *User cache *Cache}
Raw SQL When Needed
Don’t be afraid to use raw SQL for complex queries that are difficult to express through GORM:
var result []Resultdb.Raw("SELECT users.name, COUNT(orders.id) as total FROM users LEFT JOIN orders ON orders.user_id = users.id GROUP BY users.id").Scan(&result)
Connection Pool Tuning
Properly configuring your connection pool can prevent bottlenecks:
db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{ SkipDefaultTransaction: true, PrepareStmt: true,})
sqlDB, err := db.DB()sqlDB.SetMaxIdleConns(10)sqlDB.SetMaxOpenConns(100)sqlDB.SetConnMaxLifetime(time.Hour)
Remember, performance optimization is an iterative process. Always measure the impact of your changes using benchmarks and monitoring tools to ensure your optimizations are actually improving performance.
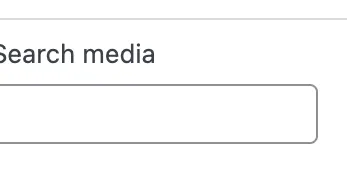





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.