- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Error Handling in GORM: Debug Common Issues
Learn effective debugging strategies and best practices for robust database operations.
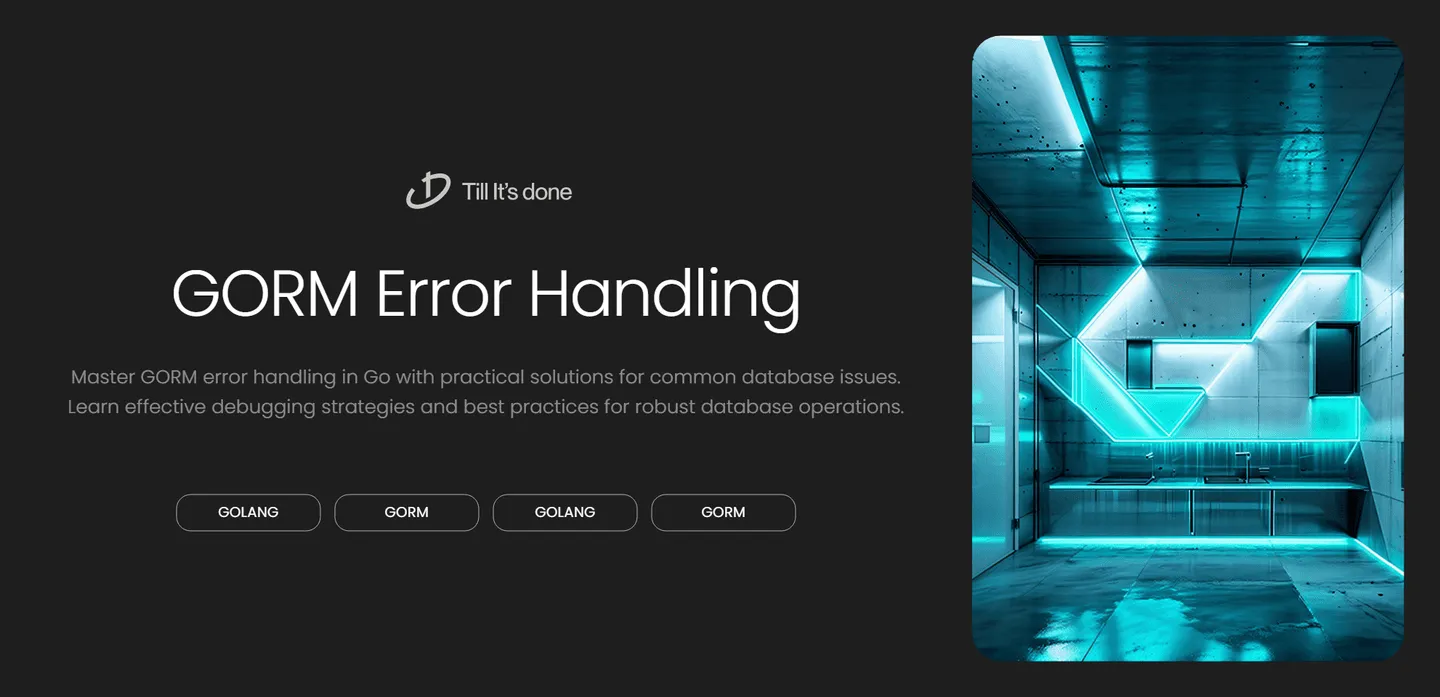
Error Handling in GORM: Debugging and Fixing Common Issues
When working with GORM in Go applications, proper error handling is crucial for maintaining robust and reliable database operations. In this guide, we’ll explore common GORM errors and learn effective strategies to debug and resolve them.
Understanding GORM Error Types
GORM provides several ways to handle errors, and understanding these patterns is essential for writing maintainable code. The most basic form is checking the error returned by GORM operations:
result := db.Create(&user)if result.Error != nil { // Handle the error}
However, real-world applications require more sophisticated error handling approaches. Let’s dive into common scenarios and their solutions.
Common GORM Errors and Solutions
1. Record Not Found
One of the most frequent issues developers encounter is handling missing records. GORM provides a specific error type for this:
var user Userresult := db.First(&user, 1)if errors.Is(result.Error, gorm.ErrRecordNotFound) { // Handle missing record}
2. Foreign Key Constraints
Foreign key violations can be tricky to debug. Here’s how to handle them effectively:
if result := db.Create(&order); result.Error != nil { if mysqlErr, ok := result.Error.(*mysql.MySQLError); ok && mysqlErr.Number == 1452 { // Handle foreign key constraint error }}
3. Validation Errors
GORM supports built-in validations, but handling validation errors requires attention:
type User struct { Name string `gorm:"not null"` Email string `gorm:"unique"`}
Best Practices for Error Handling
- Always check for errors after database operations
- Use custom error types for better error handling
- Implement proper logging for database errors
- Create middleware for common error patterns
- Use transactions for complex operations
Here’s an example of implementing these practices:
func CreateUser(db *gorm.DB, user *User) error { tx := db.Begin() defer func() { if r := recover(); r != nil { tx.Rollback() } }()
if err := tx.Create(user).Error; err != nil { tx.Rollback() return fmt.Errorf("failed to create user: %w", err) }
return tx.Commit().Error}
Debugging Tips
- Enable GORM’s debug mode during development
- Use proper logging levels
- Implement request tracing
- Monitor database performance
Remember that effective error handling is not just about catching errors – it’s about providing meaningful feedback and maintaining data integrity.
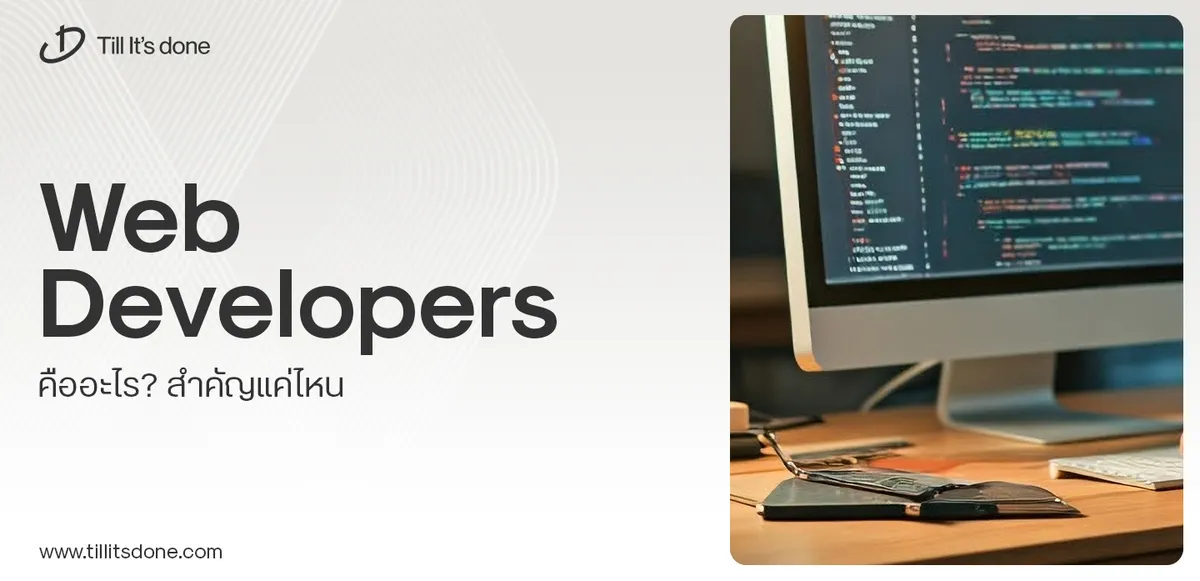
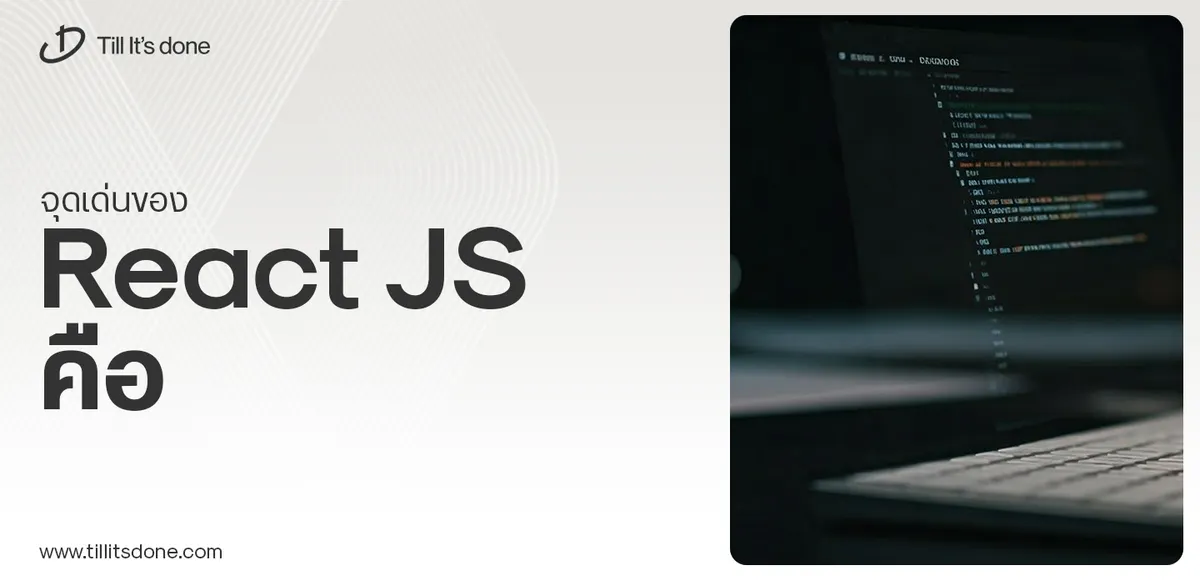
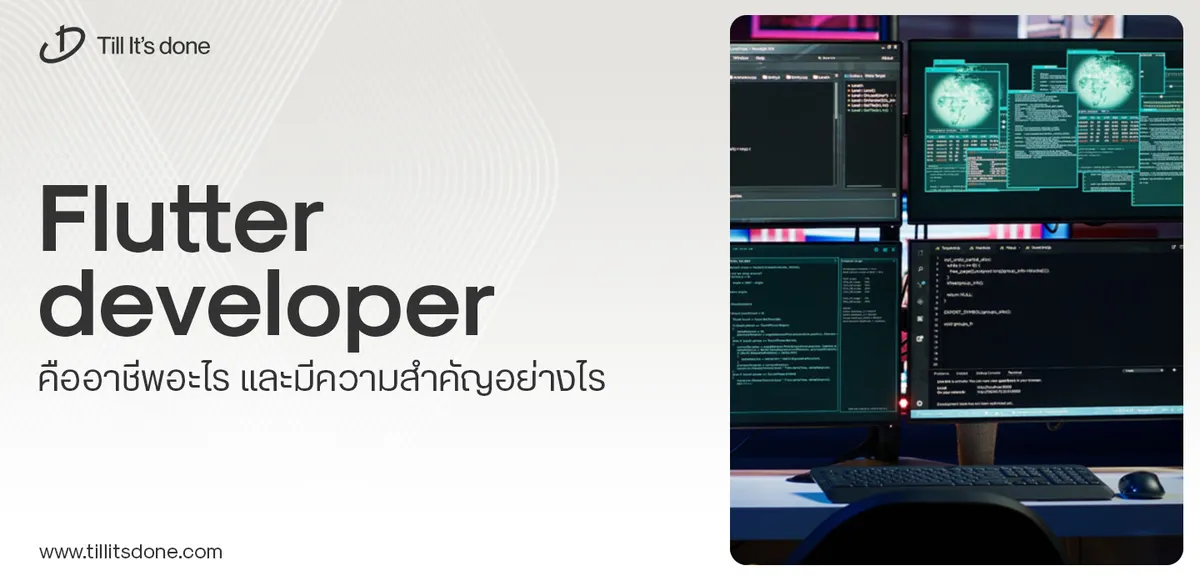
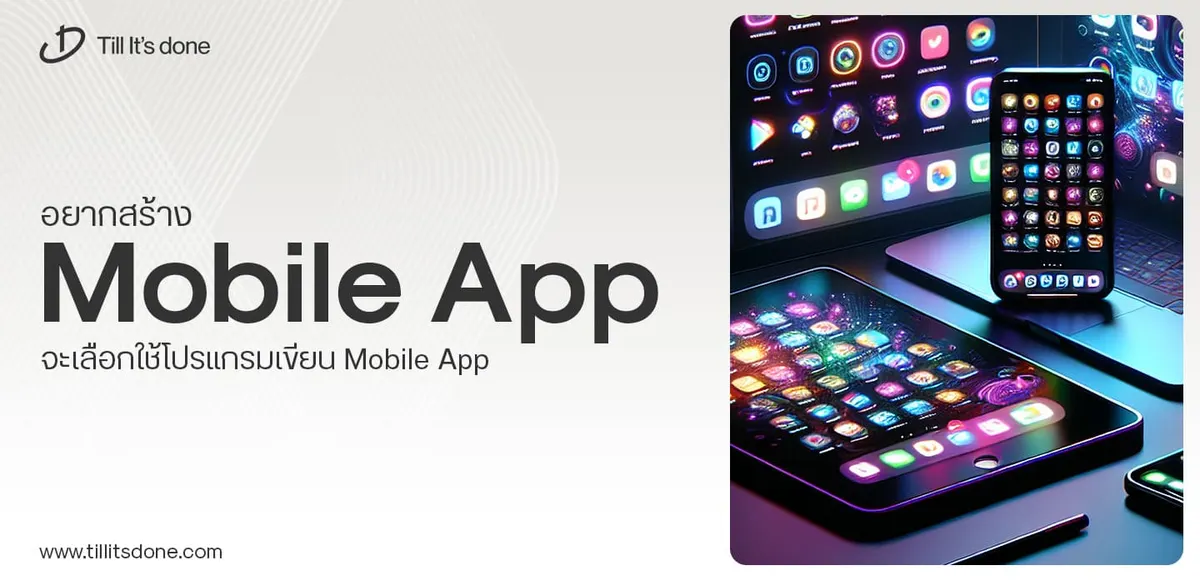
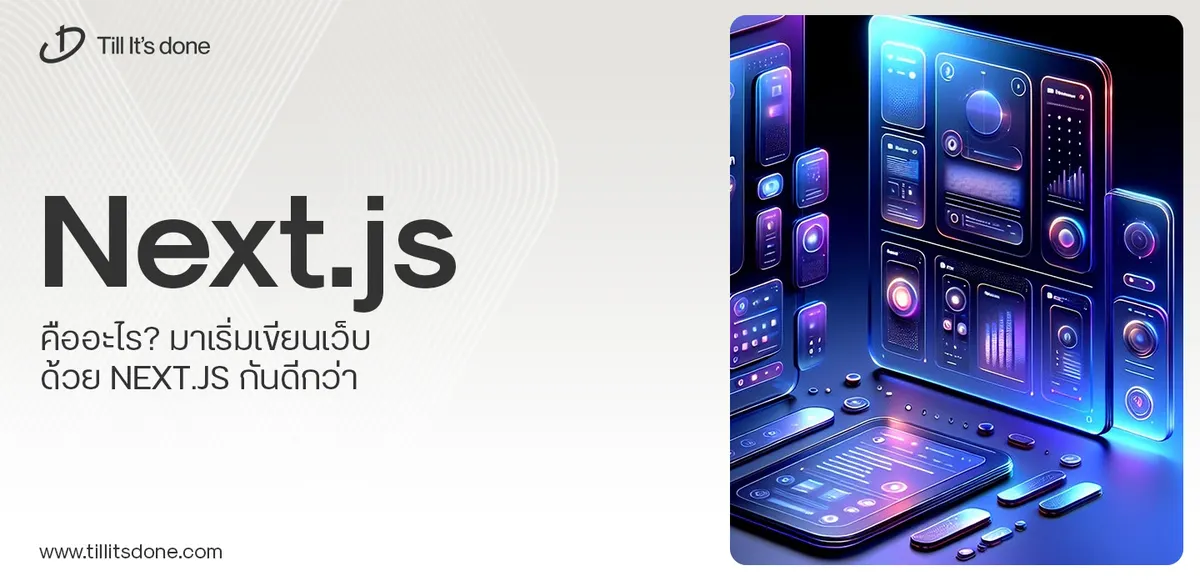
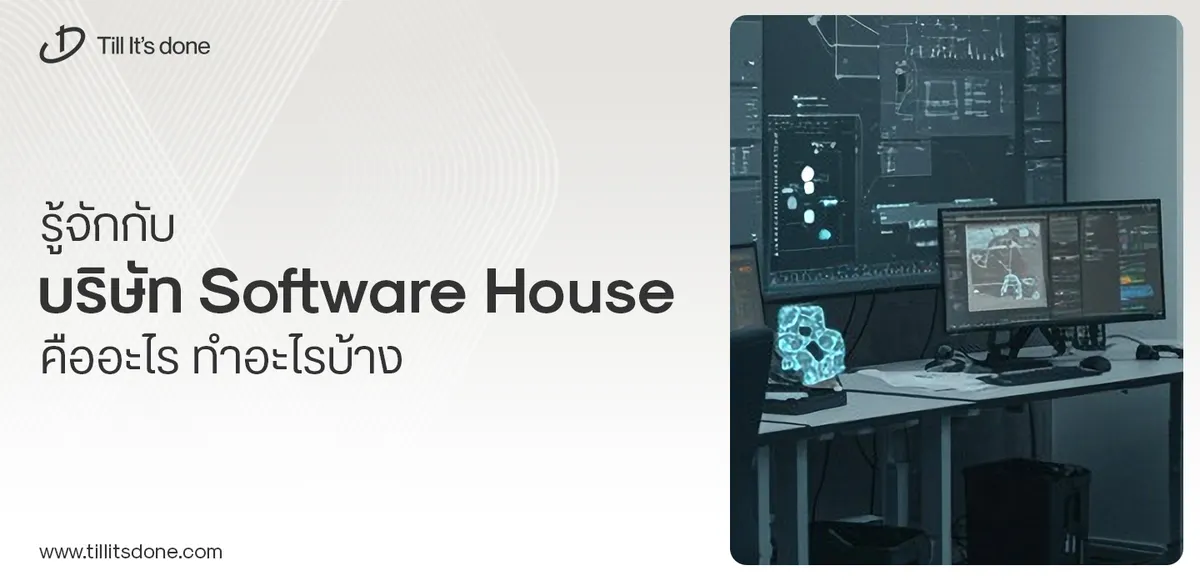
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.