- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Testing WebSocket Apps in Go: Debug Guide
Discover best practices, tools, and techniques for building reliable real-time applications.

Testing and Debugging WebSocket Applications in Golang
Building real-time applications with WebSocket in Go is exciting, but when things go wrong, debugging can feel like finding a needle in a haystack. Let’s dive into practical strategies and tools for testing and debugging WebSocket applications effectively.
Understanding WebSocket Testing Fundamentals
Testing WebSocket applications differs significantly from traditional HTTP endpoint testing. Unlike REST APIs, WebSocket connections maintain a persistent connection, making it crucial to test both the initial handshake and the ongoing message exchange.
Essential Testing Strategies
Unit Testing WebSocket Handlers
Here’s a practical approach to unit testing WebSocket handlers in Go:
func TestWebSocketHandler(t *testing.T) { server := httptest.NewServer(websocket.Handler(WebSocketHandler)) defer server.Close()
url := "ws" + strings.TrimPrefix(server.URL, "http") ws, err := websocket.Dial(url, "", server.URL) if err != nil { t.Fatalf("Dial failed: %v", err) }
message := []byte("Hello, WebSocket!") if err := websocket.Message.Send(ws, message); err != nil { t.Fatalf("Send failed: %v", err) }
var received []byte if err := websocket.Message.Receive(ws, &received); err != nil { t.Fatalf("Receive failed: %v", err) }
if string(received) != "Hello, WebSocket!" { t.Fatalf("Expected message %s, got %s", message, received) }}
Integration Testing
When testing WebSocket applications end-to-end, consider these key aspects:
- Connection establishment and handshake
- Message sending and receiving
- Connection termination
- Error scenarios and recovery
- Load testing with multiple concurrent connections
Debugging Techniques
Logging Strategies
Implement comprehensive logging to track WebSocket lifecycle events:
func (c *Connection) handleMessages() { defer func() { log.Printf("Connection closed for client %s", c.ID) }()
for { messageType, message, err := c.ws.ReadMessage() if err != nil { log.Printf("Error reading message: %v", err) break }
log.Printf("Received message type %d: %s", messageType, string(message)) // Handle message }}
Common Issues and Solutions
-
Connection Drops
- Implement heartbeat mechanisms
- Monitor connection states
- Handle reconnection gracefully
-
Memory Leaks
- Properly close connections
- Implement connection timeouts
- Monitor goroutine counts
-
Performance Issues
- Use connection pools
- Implement message buffering
- Monitor system resources
Tools and Utilities
Several tools can help in debugging WebSocket applications:
- WebSocket clients like wscat or Postman
- Network analyzers like Wireshark
- Performance monitoring tools like pprof
- Load testing tools like Artillery or k6
Best Practices
- Always implement proper error handling
- Use structured logging
- Set appropriate timeouts
- Implement retry mechanisms
- Monitor connection health
- Use connection pools for scalability
Remember, thorough testing and debugging are crucial for building reliable WebSocket applications. By following these practices and using the right tools, you can create robust and maintainable real-time applications in Go.
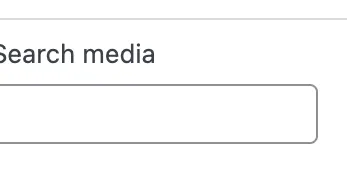





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.