- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Go Performance Tips: Profiling & Benchmarking
Learn how to identify bottlenecks, optimize memory usage, and implement effective testing strategies.

Tips for Improving Go’s Performance with Profiling and Benchmarking
Performance optimization is a crucial aspect of Go development that can make or break your application. While Go is already known for its impressive performance out of the box, there’s always room for improvement. In this article, we’ll dive into practical tips and techniques for profiling and benchmarking your Go applications to squeeze out every bit of performance.
Understanding Go’s Built-in Profiling Tools
Go provides powerful built-in profiling tools that can help you identify bottlenecks in your code. The runtime/pprof
package and go tool pprof
command are your best friends when it comes to performance analysis.
Let’s start with the basics of profiling:
import "runtime/pprof"
func main() { f, _ := os.Create("cpu.prof") defer f.Close()
pprof.StartCPUProfile(f) defer pprof.StopCPUProfile()
// Your application code here}
Essential Benchmarking Practices
Benchmarking in Go is straightforward thanks to the testing package. Here’s how you can create meaningful benchmarks:
func BenchmarkMyFunction(b *testing.B) { for i := 0; i < b.N; i++ { MyFunction() }}
Remember these benchmarking tips:
- Always run benchmarks multiple times for consistent results
- Use realistic data sets that match your production environment
- Run benchmarks on the same machine state for fair comparisons
Memory Profiling and Optimization
Memory optimization is crucial for long-running applications. Use the heap profiler to identify memory leaks and unnecessary allocations:
import "runtime"
func main() { f, _ := os.Create("mem.prof") defer f.Close()
runtime.GC() pprof.WriteHeapProfile(f)}
Advanced Performance Tips
- Use sync.Pool for frequently allocated objects
- Consider using buffered channels appropriately
- Implement proper error handling without excessive allocations
- Utilize string builders for string concatenation
- Profile your application under real-world conditions
Remember, premature optimization is the root of all evil. Always profile first, then optimize based on data, not assumptions.
Continuous Performance Monitoring
Integrate performance testing into your CI/CD pipeline to catch performance regressions early. Tools like benchstat
can help you compare benchmark results across different versions of your code.
Don’t forget to document your performance requirements and regularly review them as your application evolves.



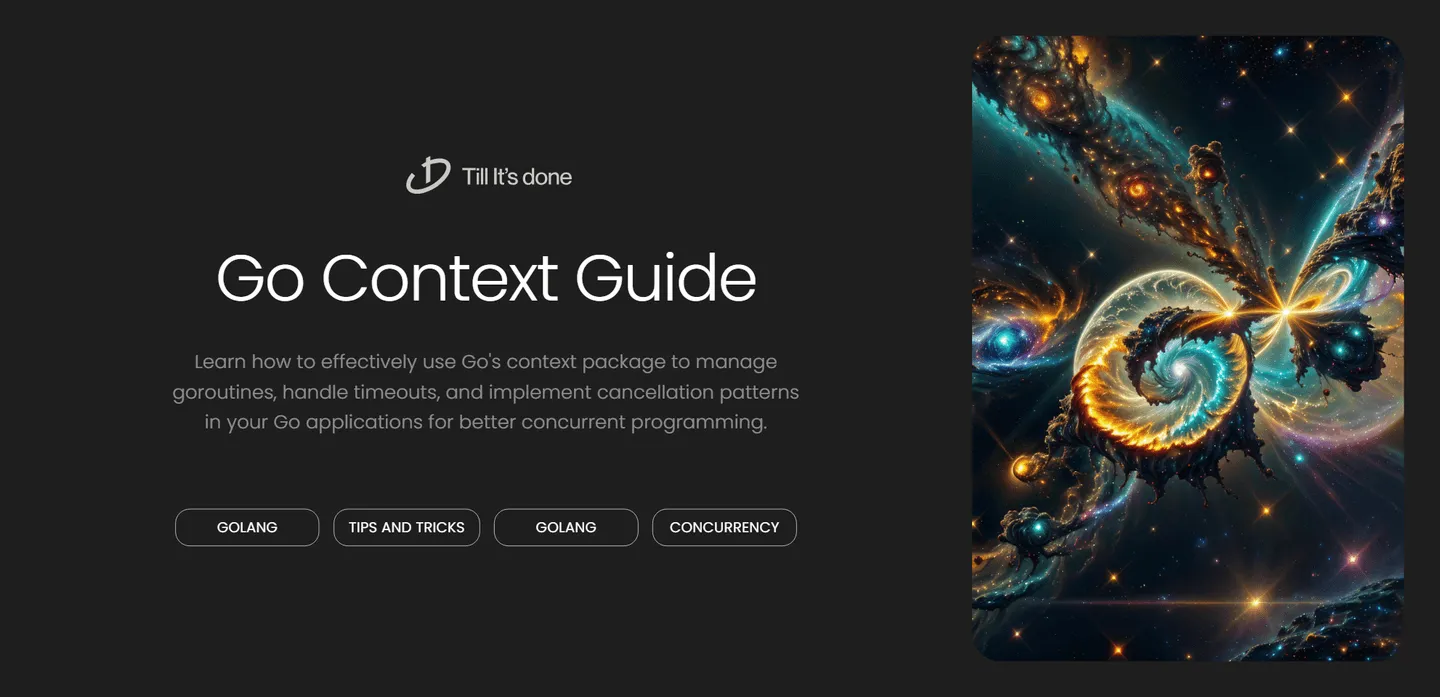


Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.